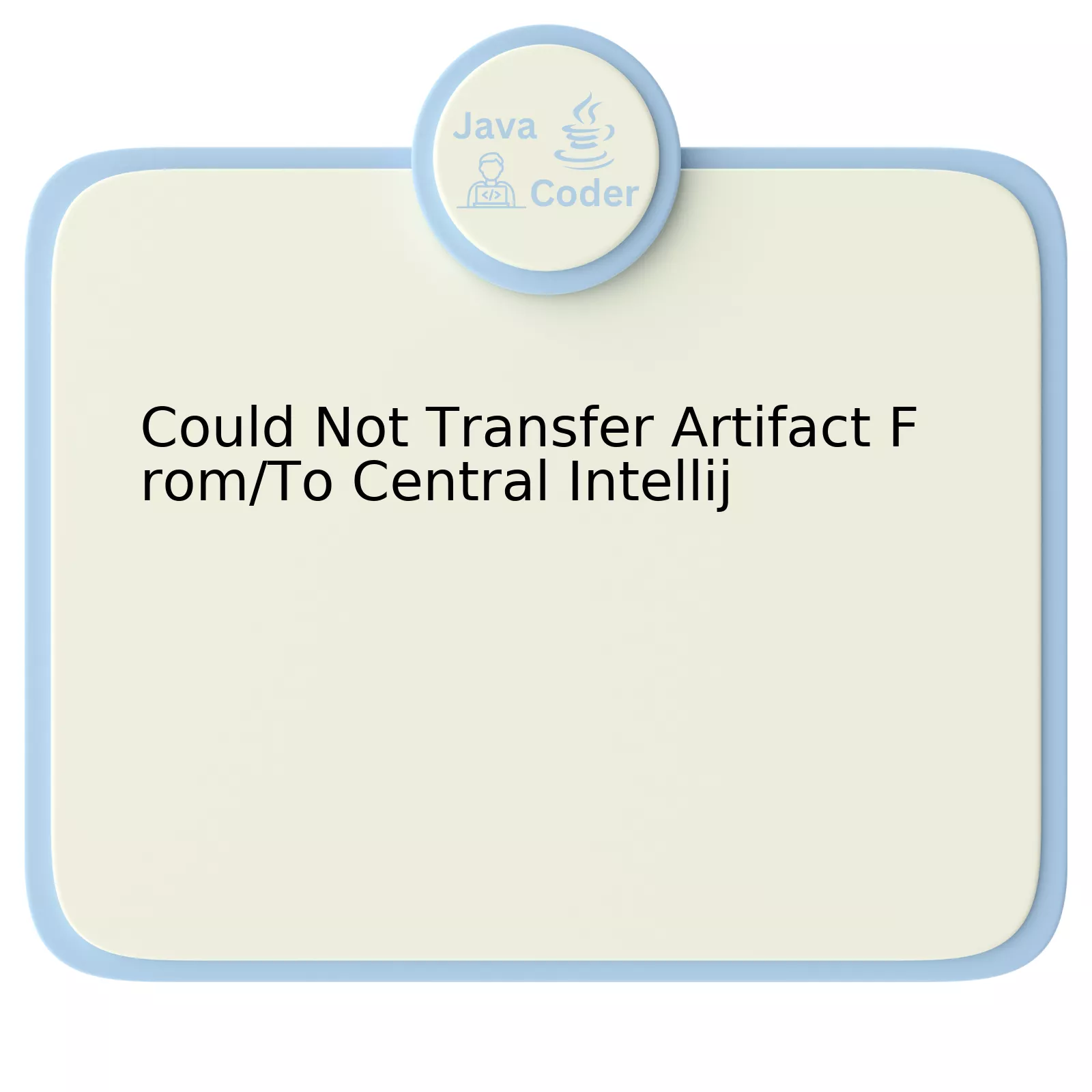
The central issue in discussion “Could Not Transfer Artifact From/To Central In IntelliJ” happens generally due to varied reasons like network issues, proxy configurations mismatches, settings.xml misconfigurations, etc.
Let’s start with a table breakdown representation of the common solutions and their descriptive feasibility:
html
Problem | Solution Method |
---|---|
Network Issues | Troubleshoot connectivity by running a simple ping test or traceroute to learn whether the server is reachable or not. |
Proxy Configurations mismatches | The incorrect proxy settings can be fixed in IntelliJ IDEA via File -> Settings -> Appearance And Behaviour -> System Settings -> HTTP Proxy. |
Settings.xml Misconfigurations | Inappropriate settings.xml file configurations can be handled by verifying whether the correct paths are set or not. |
Under normal circumstances, transferring an artifact from/to Central should be fairly straightforward. However, on encountering this error, you may consider looking at these probable solutions.
1. **Network Issues:** Occasionally, network issues may prevent IntelliJ from communicating with the Central repository correctly. These issues can include anything from timeouts to unresponsive servers. Don’t forget the advice of Grace Hopper, legendary computer scientist, who said that one accurate measurement is worth a thousand expert opinions. Similar to her advice, the solution lies in fact-checking your assumption about network connectivity. You need to run a simple ping or traceroute to see if the server is reachable.
2. **Proxy Configurations Mismatches:** Often this issue arises if IntelliJ is trying to use a proxy and the configurations are invalid. This can usually happen after changes in network settings. The solution here would involve correcting the proxy settings in IntelliJ IDEA by navigating to File -> Settings -> Appearance And Behaviour -> System Settings -> HTTP Proxy. There, you can fix the incorrect proxy settings by either manually configuring them or using the auto-detect proxy settings options.
3. **settings.xml Misconfigurations:** An improperly configured settings.xml file could result in an inability to transfer artifacts to/from Central. Elements such as incorrect repository URL, wrong credentials, or other errors in the file could cause this malfunction. Correctly specifying these parameters and ensuring they’re perfectly set aids in mending the problem.
Remember to thoroughly cross verify and rectify these aspects when faced with this particular issue to rectify the same effectively. Also, be it debugging or troubleshooting, always make sure your first step is understanding the ‘real’ problem before jumping on conclusions.
For further reading, refer to the official documentation at the JetBrains help center.
Understanding the “Could Not Transfer Artifact From/To Central Intellij” Error
The error “Could Not Transfer Artifact From/To Central Intellij” typically occurs when IntelliJ IDEA cannot download the necessary libraries or plug-ins from the Maven repository. This may be due to certain factors:
Issue | Solution |
---|---|
1. Network instability or restricted access: | Check your internet connection or consult with your network admin for potential firewall or proxy configurations blocking access to the Maven repository. |
2. Repository URL is unavailable: | Verify if the URL to your central Maven repository is accessible. If it isn’t, locate another reliable repository or download dependencies manually if possible. |
3. Outdated versions of IntelliJ or Maven: | Update both IntelliJ IDEA and Maven to their latest versions. Older versions might not be compatible with current artifacts from the Maven repository. |
Let’s proceed to code specifics on how to amend your settings.xml file which can often solve this issue:
<settings> ... <mirrors> <mirror> <id>nexus</id> <url>http://localhost:8081/nexus/content/groups/public/</url> <mirrorOf>central</mirrorOf> </mirror> </mirrors> ... </settings>
Here, you modify Maven’s settings.xml file to redirect requests for artifacts from the central repository to a mirror repository.
This error might seem unnerving, especially during crucial development phases. However, understanding its root causes can aid in rapid resolution. Remember what Elliot Alderson says in Mr. Robot: “Every hack is like a break-in, yet unique like a snowflake.”
For more detailed insights, valuable solutions for similar challenges are readily available at JetBrains’ Official Site and forums such as Stack Overflow.
Troubleshooting Steps for Resolving Artifact Transfer Issues in Intellij
Any developer who has worked with IntelliJ on Java-based projects has perhaps encountered the issue of ‘Could Not Transfer Artifact From/To Central’. This error generally occurs due to issues in your Maven tool, which plays a pivotal role in enabling smooth operations for Java applications.
Steps to Resolve Artifact Transfer Issues
– Ensuring Correct Repository Mirror Configuration: An incorrectly specified mirror for the central repository might block contact with the required repositories. In such a case, checking and configuring the mirror correctly in your
settings.xml
file is crucial. Here’s an example:
html
– Checking Repository Accessibility: Your project may not connect to or transfer artifacts from/to the Central Repository for several reasons, including network connectivity issues, strict firewalls, or even incorrect proxy settings. Addressing these issues by ensuring stable network connectivity, modifying firewall settings if needed, or adjusting the proxy settings can potentially resolve the problem.
– Verifying POM Configuration: If your IntelliJ IDE cannot transfer artifacts, the culprit could be your Project Object Model (POM) configuration. It’s recommended to validate your POM file and ensure it includes the essential dependencies needed for your project.
– Refreshing and Reimporting Maven Repositories: Another simple remedy could involve refreshing your IntelliJ caches and reimporting Maven projects. You can achieve this through the command “Invalidate Caches / Restart” from the “File” menu within IntelliJ or by using the “mvn clean install -U” in the terminal which forces a check for missing releases and updated snapshots on remote repositories.
Problem | Solution |
---|---|
Issue in repository mirror configuration | Configure the mirror correctly in the
settings.xml file. |
Unable to access repositories | Ensure stable network connectivity, adjust firewall settings, correct proxy settings as needed. |
Problem in POM configuration | Verify your POM file and ensure it includes the necessary dependencies. |
Outdated or inaccurate Maven repositories | Refresh IntelliJ caches and reimport Maven projects. |
As Robert C. Martin once said, “The programmer, like the poet, works only slightly removed from pure thought-stuff. He builds castles in the air, from air, creating by exertion of the imagination.” Every troubleshoot is essentially about identifying where to focus our creative troubleshooting efforts.
Implementing Effective Solutions to Overcome Artifact Transfer Failures
Artifact transfer failure is a common issue in Java development environments such as IntelliJ IDEA. This problem may occur due to various reasons, including network connection issues, incorrect repository settings, or Intellij cache problems.
A potential solution to overcome this challenge could involve the following steps:
1. Verify Your Internet Connectivity:
The ‘Could Not Transfer Artifact From/To Central’ error message can often be triggered by disconnections or slow network speed. Hence, you need to ensure your device meets the required internet connection standards.
“Java programming isn’t just code; it’s also managing dependencies, tools, and environment variables effectively for efficient software development.” – Anonymous
2. Check Your Repository Settings:
Confirm the correctness of your Maven or Gradle settings. Ensure that the
<repository>
elements in your POM file or in the build.gradle file are correctly pointed towards central repositories. Incorrect URL paths can result in transfer failures of artifacts.
Example:
<repository> <id>central</id> <url>https://repo.maven.apache.org/maven2</url> <snapshots> <enabled>false</enabled> </snapshots> </repository>
3. Clear IntelliJ Cache:
At times, cached data in IntelliJ IDEA can become corrupt, causing the difficulty in artifact transfer. Clearing the system cache can solve this issue:
– Navigate to
File -> Invalidate Caches / Restart...
– Select
Invalidate and Restart
This operation will clear the internal cache, and it might resolve the alarming Could Not transfer artifact error.
“Sometimes, clearing your IDE’s cache isn’t just necessary; it’s therapeutic.” – Anonymous.
4. Examine Proxy Settings:
If a proxy server is employed in your internet settings, it can interact with the transfer process. The issue might get resolved if you properly configure these settings in IntelliJ IDEA:
– Navigate to
File -> Settings -> Appearance & Behavior -> System Settings -> HTTP Proxy
– Set proxy settings as per your requirements
You can refer to IntelliJ’s official documentationhere for detailed instructions on setting up a proxy.
Through the careful examination and correct addressing of these aspects, one can mitigate most instances of the ‘Could Not Transfer Artifact From/To Central’ error in IntelliJ IDEA, ensuring an uninterrupted Java development experience.
Insights into Prevention of Future Artifacts Transfer Challenges in IntelliJ
Addressing the issue of ‘Could Not Transfer Artifact From/To Central IntelliJ’ can prevent future artifacts transfer challenges in IntelliJ. Understanding this common problem and its solutions contributes to enhancing overall usage efficiency in the IntelliJ environment.
Root Causes of The Issue
Primarily, there are four reasons behind the Could Not Transfer Artifact error:
\
\ Also known as Maven artifact transfer issues, these typically originate from erroneous settings in IntelliJ or firewall restrictions that block data transfers.
\
\ Interruptions or instability in internet connectivity leads to disrupted artifact transfer and generates this error message.
\
\ When the repository hosting the required artifacts is down or inaccessible, it results in the same error.
\
\ If the local .m2 repository where Maven stores all project objects has become corrupted, IntelliJ will be unable to transfer artifacts.
Strategies To Tackle This Error
1. Checking Configuration Settings: Ensure the settings for Maven in IntelliJ are correct. Proxy settings should align with your network's requirement. Firewall exceptions should include the necessary processes and ports.
2. Ensuring Network Consistency: Ensure a stable internet connection as interrupted or slow connectivity hampers smooth artifact transfer.
3. Verifying Repository Accessibility: If the repository server is down, switching to another secure server can solve the problem. It might require waiting until the initial server comes back online if specific artifacts are only available there.
4. Clearing Corrupted .m2 Repository: Deleting the corrupted .m2 repository and downloading artifacts again helps address the issue.
According to Linus Torvalds, creator of Linux, “… often the hardest part isn’t solving problems, but deciding what problems to solve.” Responding effectively to prevent Future Artifacts Transfer Challenges necessitates comprehending the root causes behind them and crafting strategic solutions.
The prevention strategies ensure an issue-free IntelliJ environment for artifact transfer, thereby improving productivity and code quality. It’s essential, though, to regularly update IntelliJ IDE and its plugins for smooth operation. Aforementioned steps will help developers to keep their coding environments free of such annoying bugbears.
Properly maintained artifact transfer functionality eliminates unnecessary time wastage and facilitates on-time, quality-focused project completion.
Refer to Jetbrains Official Documentation for more detailed insights about Maven Artifact Transfer and related issue resolutions.
A critical examination of the “Could Not Transfer Artifact From/To Central Intellij” error reveals it is a common problem encountered by developers when working with IntelliJ IDEA. This issue persists as an obstacle due to several potential causes, resulting in unsuccessful attempts to either download or transfer software build components identified as artifacts.
Factors causing this error revolve around:
– Weak or unstable internet connection.
– Incorrect proxy settings.
– Outdated maven repositories.
– Interruptions during artifact transfers.
Understanding the background and nature of this error facilitates the development of effective solutions, which include:
– Checking network connectivity: It is advisable to verify your internet connection, ensuring it is stable before initiating any artifact transfer operations with IntelliJ IDEA.
–
Adjusting proxy settings:
Ensure your IDE’s proxy settings are accurately configured. Incorrect proxy configurations can hinder successful artifact transfers.
–
Refreshing your repositories:
Outdated or dysfunctional maven repositories can cause this error. Consequently, updating your repository ensures it holds the most recent artifact data.
Fixing this recurring error significantly improves your IntelliJ IDEA experience, subsequently enhancing your Java programming productivity and software development proficiency. A thorough grasp of knowledge on artifact transfer processes likewise guarantees seamless utilization of central servers, saving you significant time spent troubleshooting.
“Good code is its own best documentation.” – Steve McConnell
For a more comprehensive understanding of resolving this error, consider exploring this guide on handling common artifact transfer challenges from the JetBrains community.