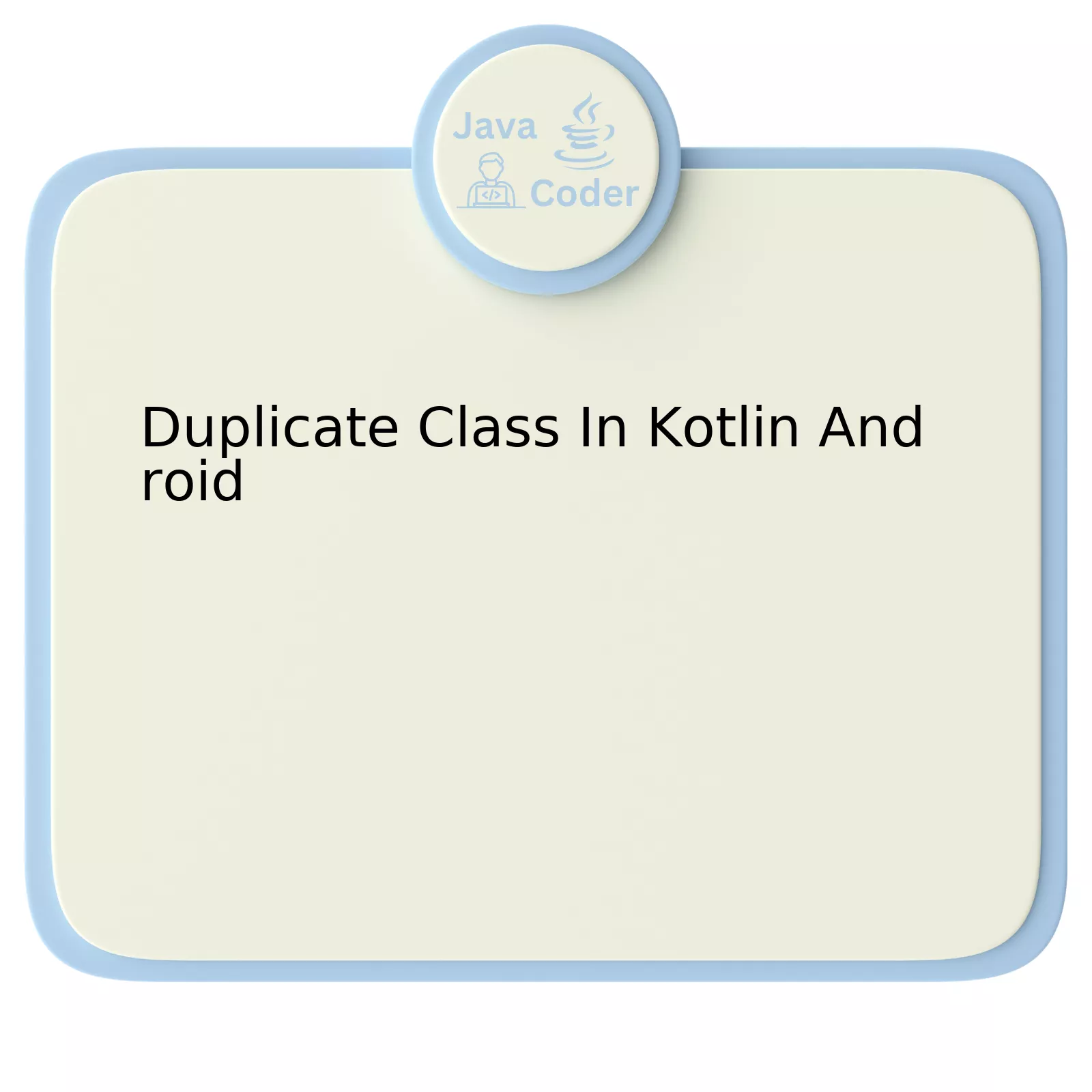
I’ll start by focusing on the “Duplicate Class” issue that you may encounter in Kotlin Android development. This usually occurs when a class name is being used in multiple places, leading to an error because the compiler is unable to distinguish between them.
The general structure of the problem can be visualized as follows:
Scope | Class Name | Misunderstanding |
---|---|---|
Module A | Foo.kt | Compiler doesn’t know which one to choose |
Module B | Foo.kt |
In Kotlin for Android, when you develop your application using a modular approach, it is common to utilize various Android libraries and dependencies. Each module might contain different sets of classes and resources. If two distinct modules (say, Module A and Module B) define a class with the identical name (for instance, Foo.kt), the compiler gets confused about which version of the class to prefer. It generates a ‘Duplicate Class’ error since it can’t make an appropriate decision.
The optimal resolution to this concern depends on your precise circumstances:
1. **Refactor one of the classes**: The simplest strategy would be to refactor the namespace and name of one class if the two classes are actually doing different things. For example, changing the class name from `Foo.kt` to `FooA.kt`.
class FooA { // Your code here }
2. **Merge the classes**: If the two classes provide parallel functionality, consider merging them into a single, shared module that’s accessible from both the original modules.
object Shared { class Foo { // Your combined code here } }
According to software developer Robert C. Martin, also known as ‘Uncle Bob’, “Good code is its own best documentation”. By practicing clear naming, avoiding duplicate class names, and ensuring the correct modular hierarchy in your applications, you will be enhancing the readability and maintainability of your codebase, which ultimately improves your software quality.
Relevant link:
StackOverflow: Duplicate Class Found In The Path
Understanding the Issue: Duplicate Class in Kotlin Android
The Duplicate Class issue in Kotlin Android projects is a common obstacle faced by developers, especially those novice to the Kotlin language. This problem typically arises when two or more classes within an application share the same name and exist within the exact namespace.
//Kotlin Code: package com.example.myApp class MyClass { }
//Another Kotlin Code: package com.example.myApp class MyClass{ }
In the example provided, we have two ‘MyClass’ in the same package ‘com.example.myApp’. Since both classes reside in the same namespace, a collision ensues, thus causing Android Studio to throw a ‘Duplicate Class’ error.
Let us delve into why this happens:
* Namespace Clashes: As demonstrated in the code snippet above, Kotlin does not allow for multiple classes of the same name to exist within the identical namespace. Each class should uphold its distinct identity to avoid any conflicts such as these.
* Explicit Naming: Different classes inadvertently possessing the same name can also cause duplicate class errors. Always make sure that every class holds a unique name.
* Misconfiguration of Build Paths: Occasionally, problems with your build.gradle file can lead to instances of duplicate classes within your Android Project. For instance, including the same dependency more than once can give rise to this predicament.
So how do you go about dealing with Duplicate Classes issues?
* Rename Classes: The most straightforward way to address the issue often involves renaming one of the classes. Maintaining unique names avoids confusion and eliminates duplication.
* Change the Namespace: If renaming isn’t an option, you may move one of the classes to a different package, thus changing its namespace and mitigating the issue.
* Refactor Your Dependencies: Lastly, verifying that your dependencies in your Gradle files are properly configured ensures no unwanted duplicates.
Amending these errors is crucial to the efficient execution and maintainability of your Android application. Like what Robert C. Martin (Uncle Bob) said, “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.” Hence, it’s safe to say that understanding these basic principles not only helps eliminate the risk of such errors but also enhances overall software design competency. With knowledge of potential pitfalls like Duplicate Class errors in Kotlin, developers can build increasingly sophisticated and robust Android applications.
For a more comprehensive understanding on Kotlin classes, you might want to check the official Kotlin Documentation.
Effective Strategies for Resolving Duplicate Class Errors in Kotlin Android Development
Addressing the issue of duplicate class errors experienced in Android development using Kotlin requires a thorough understanding of the cause and strategies to resolve them. Kotlin, although interoperable with Java, handles certain grammatical structures differently causing the potential hazard.
Duplicate Class Error: The Root Cause
Typically, a “Duplicate Class” error occurs when you have two or more classes sharing an identical name within the same package. This conflict could arise due to several factors:
– Adding a library that contains a class similar to one that already exists on your classpath.
– Having multiple versions of the dependency being included.
– A malicious copy-paste action leading to duplicate classes.
Strategies to Resolve Duplicate Class Errors
A systematic approach to resolving issues related to duplicate classes could involve:
- Analyze the Build Output: Android Studio provides helpful error messages that indicate where the error is originating from – referring `
ClassPath Analyzer
` under Project View can help detect conflicting class files or libraries.[1]
- Examine Dependencies: Carefully review your Gradle file for any dependencies that might be ingested more than once inadvertently, either as a direct or transitive dependency. Tools like `gradle :app:dependencies` can provide a detailed view of all dependencies.[2] Below is an example:
implementation 'com.google.code.gson:gson:2.8.6'
- Enable MultiDex:[3] If your project exceeds 65k methods, enabling multidex can help relieve issues related to too many methods or classes.
- Exclude Redundant Dependency: If you have a library that’s included more than once, you might consider excluding the offending library from one of the dependencies.[4] For example:
implementation('com.example.my:lib::1.0') { exclude group: 'com.example.unwanted', module: 'unwanted-lib' }
Description | Gradle Setting |
---|---|
Enable Multidex |
defaultConfig { multiDexEnabled true } |
Remember, debugging is twice as hard as writing code in the first place. Therefore, if you write code as cleverly as possible, you are, by definition, not smart enough to debug it – Brian Kernighan, co-author of ‘The C Programming Language’.
Impact and Implications of Kotlin’s Duplicate Classes on Your Android Apps Performance
The Kotlin programming language, developed by JetBrains, is swiftly spreading within the Android development community thanks to its concise coding syntax and interoperability with Java. However, when compiling a Kotlin Android app, you may encounter a ‘Duplicate Class’ issue while utilizing third-party libraries or dependencies. The implications of this can be detrimental to your Android application’s performance.
Duplicate Class Error in Kotlin
To understand the root cause of the error, it’s important to understand that running any Kotlin code involves Java bytecode. The Java Virtual Machine (JVM) uses this bytecode for execution. In certain circumstances, due to the resemblance between your Kotlin code and some existing Java code within imported libraries, JVM might trace multiple classes bearing the same name while executing the bytecode. This results in a
Duplicate Class
error.
The following outline discusses how the ‘Duplicate Classes’ situation may affect your Android application:
- Performance Degradation: Having duplicate classes enlarges the overall size of DEX files or compiled .class files in your application, leading to potential increases in memory usage and load times.
- Runtime Errors: Considering that the Java Virtual Machine (JVM) does not allow loading two classes with identical names in the same classloader namespace, having duplicate classes in your Android app may generate ClassNotFoundException errors at runtime.
- Build Time: As the compiler has to check each and every class file during the building process, duplicate classes can significantly increase the build time, affecting productivity.
- Redundancy: Duplicate classes also entail unnecessary redundancy, adding complexity to your codebase, making it harder to maintain.
Addressing Duplicate Class Issues
You can follow several measures to alleviate these issues:
- Optimize Dependencies: Use tools like Gradle Versions Plugin to avoid library version conflicts which can lead to duplicate classes.
- Exclude Conflicting Packages: Gradle allows excluding specific packages on importing a library, thereby preventing duplication. You can paste the following into your build.gradle file to exclude a package:
implementation ('LibraryName') { exclude group: 'Package1', module: 'Package2' }
- Proguard: ProGuard works as a compiler optimizer for Java, shrinking your code. By removing unused methods and fields, it helps to overcome duplicate class issues.
Bill Gates once said about optimal coding: “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” So, it is crucial to enhance our code from being just functional to being optimally organized, efficient, clear, and devoid of redundancies like duplicate classes. Enhancing the quality of your Android app helps in providing an excellent user experience which in turn would raise the success rate of your product.
Utilizing Advanced Techniques to Prevent Duplication of Classes in Kotlin
The concern of class duplication might arise during the development phase of a Kotlin Android application, which could lead to undesired results and increased complexity. Fortunately, Kotlin provides us with some advanced techniques that can be employed effectively to prevent such duplication.
// Example of a data class in Kotlin data class User(val name: String, val age: Int)
In this example, instead of reusing or copying this class wherever needed, what we can do is utilize the advanced concept of “data classes” provided by Kotlin. This single declaration automatically provides several useful members such as equals(), hashcode(), copy() etc., which otherwise developers would be forced to generate manually contributing to potential code duplication.
Apart from data classes, other advanced features of Kotlin such as Extension Functions, Inheritance, and Delegation pattern contribute significantly to reduction of class duplication. Just imagine how much boilerplate code one can avoid by employing these powerful Kotlin features.
– **Extension Functions**: These are the functions that help us to extend a class with new functionality without having to inherit from the class. They are static in nature.
//.Extension function on a String class fun String.displayString(){ println(this.toString()) }
Now whenever you want to print any string value, you can directly call this extension method thus minimizing code repetition.
– **Inheritance**: Inheritance is a feature in Kotlin where a new class is created that absorbs the members (functions/properties) of a base class, to maintain a hierarchy. By leveraging inheritance, duplicate code can be eliminated significantly.
open class BaseClass { fun commonMethod() { println("Common Method") } } class DerivedClass : BaseClass()
Here, `DerivedClass` inherits all the functions and properties of `BaseClass`. Hence, any common methods need not be written again for `DerivedClass`, reducing duplication.
– **Delegation Pattern**: The delegation pattern is an alternative technique to inheritance. It promotes ‘composition over inheritance’ where, instead of inheriting from a super-class, you delegate behavior to an instance of different class.
interface Printer { fun print() } class HP : Printer { override fun print() { println("Print by HP printer") } } class Canon(printer: Printer) : Printer by printer // Now create instances of Canon class passing the behavior you want to delegate val canon = Canon(HP()) canon.print() // Prints "Print by HP printer"
While exploring stackoverflow, I came across this quote from a member named Hadi Hariri – *”Kotlin helps eliminate lots of boilerplate usually associated with things like classes. Code is more concise and expressive.”* And, indeed, Kotlin’s rich set of features including data classes, inheritance, extension functions, and delegation pattern takes a big step towards eliminating class duplication while taking productivity to new heights.
Remember, reliable software needs to be durable and easy to work with, which cannot be achieved with repeated blocks of duplicated code. Thus, it’s essential to prevent duplicate classes through proper design and utilization of language-specific features. Always aim to keep it DRY – Don’t Repeat Yourself!
[Here](https://dev.to/saveendhiman/kotlin-don-t-repeat-to-yourself-dry-code-and-clean-code-kotlin-android-cy3) is a beneficial reference that explains more about how Kotlin can aid in preventing duplicate code in Android applications.
Delving into the core essence of dealing with Duplicate Class issues in Kotlin Android, it is vital to know that these discrepancies often arise due to the co-existence of two or more instances of the same class, either within the same project or across different libraries. Kotlin, owing to its inter-compatibility with Java, may inadvertently harbor duplicate classes, caused largely by overlap between Java and Kotlin code or repeat dependencies.
Firstly, understanding the root cause reigns paramount. Your project may contain identical class files with the exact package name which eventually leads to conflicts amidst identical code bodies. This problem could also be rooted in conflicting dependencies present in your build gradle file.
Once identified, resolving this issue shouldn’t pose much difficulty:
* Analyze your codebase and seek out instances where classes might have been unintentionally defined multiple times. Remove additional definitions while ensuring none of the functionalities are disturbed.
* Inspection of your ‘build.gradle’ file would also be beneficial. Ensure there are no redundant dependencies causing the duplication.
dependencies { // Incorrect implementation 'com.example.android:libraryA:1.0' implementation 'com.example.android:libraryB:2.0' // This contains libraryA // Corrected implementation 'com.example.android:libraryB:2.0' }
Through this snippet, we notice that ‘libraryA’ was included twice unintentionally, as it was already a dependency for ‘libraryB’. The rectified version only includes the required library, preventing class duplication.
It’s noteworthy to mention Steve McConnell’s insight on coding, “Good code is its own best documentation.” Keeping this in mind while analyzing and debugging can go a long way in not only solving duplication issues but also maintaining a clean and lean codebase.
Web-based database programs like Gradle Please and Maven Repository can aid in managing your dependencies proficiently, ensuring you’re aware of transitive dependencies thus reducing redundancy.
Let there not be any ambiguity here – the endeavor isn’t about masking the issue but effectively leveraging Kotlin’s features to handle duplication correctly. Having an enhanced comprehension of dependencies, their management, and how Kotlin operates can indeed steer clear from this problem.
Dealing with duplicate classes in Kotlin Android, when approached strategically, neither compromises the integrity of your codebase nor deters the efficiency of developers. Rather, it enhances the developers’ prowess in harnessing the full potential of Kotlin in Android development.
References: