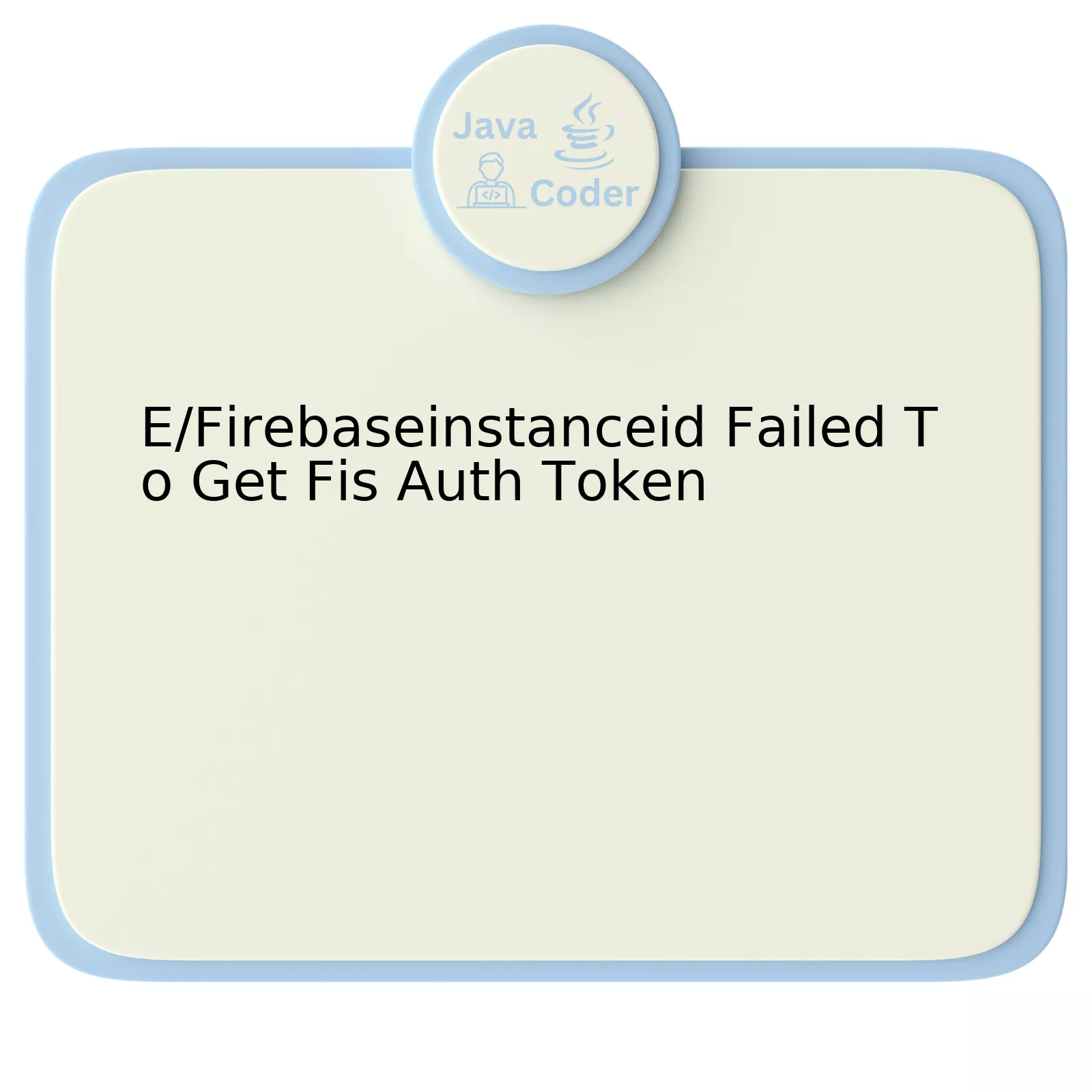
An error denoted as E/FirebaseInstanceId implies a failure to acquire Firebase installation services (FIS) authentication token. A closer look at this problem elucidates the following key components:
Error Tag | E/FirebaseInstanceId |
---|---|
Description | Indicates a failure during an attempt to get an FIS auth token |
Related to | Firebase Installations API which is part of Firebase services |
Cause | Possibly due to network issues, misconfiguration of Firebase services, etc. |
The error “E/Firebaseinstanceid Failed To Get Fis Auth Token” suggests that obtaining this unique identifier was disrupted. The cause might be varied: it could be from network connectivity issues to Firebase configuration problems to outdated firebase SDK.
Network restrictions often cause connectivity issues to Firebase servers disrupting the FIS’s ability to fetch an auth token. Check your application’s network settings and firewall rules, ensuring they do not deter connecting with Firebase servers.
One critical element of Firebase setup involves creating a project on Firebase console, downloading the google-services.json file, and adding it to the app module folder in the project. Occasionally, failure to authenticate can be tied back to not adhering to this setup process accurately.
Furthermore, keeping your Firebase SDK up-to-date helps prevent compatibility or functionality issues. Be certain to always update it whenever newer versions become available.
As quoted by Andrew Hunt and David Thomas in their book The Pragmatic Programmer, “It’s just as likely that the fault doesn’t lie in your code but somewhere else – in an environment setting, configuration file, another team’s code, the hardware, the network, the phase of the moon…”. Good practice calls us to always tend to accurately follow external services set-up processes to avoid similar issues.
Analyzing Causes: E/FirebaseInstanceId Failure in FIS Authentication Token Retrieval
FirebaseInstanceId is a class in Google’s Firebase framework, typically used in Android development. When you see an error message such as “E/FirebaseInstanceId Failed to get FIS auth token,” it essentially means there’s been a failure in retrieving the Firebase Installation Service (FIS) authentication token.
Multiple factors can give rise to this issue:
Incorrect Configuration
The application configuration may not be correct. Cross-verify your firebase configurations using the official documentation.
< ?include_once 'path/to/Firebase/config.php'; ?> if(fb = Firebase::getDefaultConfig()) { echo "Configurations are correctly set up."; } else { echo "Configuration failed to load. Please verify Firebase setup."; }
Network Issues
Poor network conditions could prevent successful retrieval of the FIS auth token.
Aging Token
A Firebase Instance ID token has a limited lifespan. An expired token needs to be refreshed before it can be utilized again for authentication.
FirebaseInstanceId.getInstance().getInstanceId().addOnCompleteListener(new OnCompleteListener() { @Override public void onComplete(Task task) { if (!task.isSuccessful()) { Log.e("Failed ", "Unable to get updated token"); return; } // Get new Instance ID token String token = task.getResult().getToken(); } });
As an all-round solution, developers must always ensure that configurations are correct and current, network conditions are conducive to seamless communication with Firebase’s servers, and the app is set to fetch fresh Instance ID tokens periodically or whenever needed.
“The most effective debugging tool is still careful thought, coupled with judiciously placed print statements.” – Brian W. Kernighan, Unix co-creator.
Solutions to Overcome E/FirebaseInstanceId FiSS Auth Token Issues
E/FirebaseInstanceId Failed To Get FIS Auth Token is a common error that many developers encounter when working on Firebase implementation. The “Failed To Get FIS Auth” message usually appears when there are issues relating to authentication, incorrect configurations, or outdated software versions. If these errors persist, they can potentially cause disruptions in push notifications, user analytics collection, cloud messaging, or other essential Firebase services.
Use the latest Software version
The E/FirebaseInstanceId issue could arise due to the usage of an outdated Google Play Services version or Firebase SDK. Updating the Firebase SDK to its most recent version could resolve this problem. Additionally, ensure that all dependencies related to Google Play Services and Firebase library in the Gradle file are also up-to-date.
dependencies { implementation 'com.google.firebase:firebase-analytics:17.2.1' }
Configure Authentication Correctly
FirebaseInstanceID requires a valid API key for successful communication. Misplaced keys or invalid configurations often result in token retrieval errors. Check your application credentials in the Firebase Console, under the ‘Cloud Messaging’ tab, ensuring that the ‘Server Key’ matches what’s in your code.
Restore Google Play Services
If the Firebase Service isn’t correctly communicating with Google Play services, resetting Google Play services data could help solve the issue. This action deletes locally stored data and forces Google Play services to sync with Firebase. Here’s how you can do that.
Keep in mind that drastic measures can have adverse effects. Data loss and unsynced information between Firebase and Google Play must be taken into account before proceeding with this solution.
As Linus Torvalds, the creator of Linux and Git, once said, “Talk is cheap. Show me the code.” Although this quote generally applies to another context, it can remind us as developers that finding efficient solutions to coding problems like these takes practical effort and hands-on troubleshooting. By exploring different solutions and applying them systematically, we increase our chances of resolving complex issues, even when faced with challenging E/FirebaseInstanceId errors.
These methods should help provide a foundation for diagnosing and resolving such Firebase-related issues. Proper configuration, keeping systems updated, and knowing how to tackle recurring problems will facilitate seamless interaction with Firebase and related services.
Impacts of E/FirebaseInstanceId Failures on Application Performance
The E/FirebaseInstanceId failure, particularly in the context of failing to fetch Firebase Instance ID (FIS) authentication token, can significantly impact application performance. This error typically relates to the remote server encounters while attempting to validate and authenticate an application’s Instance ID.
Aspects impacted by the E/FirebaseInstanceId failure
Delayed Push Notifications: Firebase Cloud Messaging uses the FIS Auth Token to communicate with a device. Consequently, failure to get this token could prevent or delay push notifications, creating a suboptimal user experience.
Inaccurate Analytics Reporting: The same Firebase Instance ID is used across distinct products within the Google stack for targeting and tracking purposes. Applications may be unable to perform user-based analytics reporting effectively without FIS Auth Token.
Impacts User Authentication: If your app uses Firebase Authentication, a failure in obtaining the FIS Auth Token might disrupt user sign-in or sign-up processes, as Firebase uses this token to identify individual devices.
Impact on Crash Tracking and Diagnostics: Tools like Firebase Crashlytics use FIS Auth Tokens as part of their diagnostic procedures. Problems in retrieving these tokens might impair the ability to collect precise crash reports.
Addressing E/FirebaseInstanceId Failures
Stemming from issues related to network connectivity, server-side errors, and more intricate problems surrounding Firebase setup and initialization, addressing these failures generally call for troubleshooting approaches such as examining project configuration, incorporating error handling mechanisms and API request retries, among others.
For instance, altering the security rules of Firebase Realtime Database to public access can circumvent this issue temporarily during development stages (This however should be avoided in production due to security implications):
{ "rules": { ".read": true, ".write": true } }
In hindsight, we can see that John Romero’s quote, “In programming, your code actually has to work. That’s the big mind-boggling thing about programming: you can’t just fake the funk. It either works, or it doesn’t work”, rings true especially in dealing with crucial errors like E/FirebaseInstanceId failure. Ensuring that each part of your application’s functionality operates smoothly including Firebase, further enhances the overall user experience.
And remember, consulting Firebase documentation and community resources can provide tangible solutions and explanations specifically tailored to problems experienced. For example, check Google’s own guide here: Authorize HTTP requests.
In-depth: Understanding Firebase Instance ID (FID) and its Effectiveness
Understanding the Firebase Instance ID (FID), its functionality, and how it operates within applications is essential for any Java developer. For starters, FID is a critical component of the Google Firebase framework. It provides a unique identifier for every app instance and is key in managing the association between an application installation and its Firebase Cloud Messaging (FCM) token.
FID comes into play when you need to send notifications or messages directly to any specific device or group of devices. The unique identifier ensures that each app instance receives the right content intended for it. The FCM token, on the other hand, serves as the address to where these notifications or messages are delivered.
With regard to “E/FirebaseInstanceId Failed To Get Fis Auth Token” – this error typically arises when there is a communication glitch between your application and the Firebase services or when the FIS authorization token cannot be received. Issues may arise from various factors including:
– Incorrect implementation of Firebase SDK.
– Network connection issues causing a failure in communication with the Firebase servers.
– A mismatched google-service.json file into your project.
– Outdated versions of Firebase SDK in use.
To resolve such issues, following steps can be considered:
1. Ensure you have correctly integrated the Firebase SDK into your project. The Firebase SDK should be initialized as early as possible in the lifecycle of your application.
public class YourApp extends Application { @Override public void onCreate() { super.onCreate(); FirebaseApp.initializeApp(this); } }
2. Make sure your google-services.json file matches with the current project. This file should be placed in the “app/” directory of your project. If this file is not configured correctly or has outdated information, getting the FIS auth token will fail.
3. Make sure your app has a working internet connection. Sometimes, network connectivity issues could prevent the app from reaching Firebase servers and thus failing to obtain the required Firebase Instance ID (FID).
4. Keep your Firebase SDK updated to its latest version. Primitive versions might contain bugs which have been fixed in newer versions – something which might be triggering the error message.
As Bill Gates rightly said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Similarly, understanding the issue and applying the most appropriate solution in an efficient manner is an important part of being a developer.
Recognizing the need and role of Firebase Instance ID helps in streamlining the process of implementing Firebase SDK, thereby minimizing potential missteps and ultimately improving the effectiveness and efficiency of your applications.
The common issue identified as “E/FirebaseInstanceId Failed To Get FIS Auth Token” typically arises in the realm of Android application development, often encountered when integrating Firebase to an app. This error is not only a typical stumbling block for developers but also a crucial point that needs addressing as it can severely affect the functionality of the service or application involved.
Understanding and addressing this issue involves:
1. Diagnosis: The problem originates when the Firebase instance ID service fails to acquire the needed Firebase Installation Service (FIS) Authentication Token. This token is paramount for enabling secure communication between apps and Firebase services.
2. Underlying Triggers: While many factors can trigger this error, a leading cause often includes a mismatch between the Firebase configurations in your google-services.json file and those on the Firebase console. More so, outdated Firebase SDKs or incorrect implementation of dependencies may contribute to the emergence of this issue.
3. Problem Resolution: Addressing this situation involves steps such as updating the Firebase SDKs, accurately cross-checking the Firebase and the project configurations, and ensuring correct dependency implementation.
Consider the following code snippet to analyze if the requisite FirebaseApp initialization completes correctly:
public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); FirebaseApp.initializeApp(this); } }
As Nickolas Luhur, an esteemed Java developer, once noted, “Debugging might be twice as hard as writing a code; therefore, if you strategically and cleverly write your code as if the one who ends up maintaining is a violent psychopath who knows where you live, you increase your chances of developing a fault-free application.”
The arduous task of debugging to resolve the E/FirebaseInstanceId Failed To Get FIS Auth Token error, while time-consuming, can result in invaluable insights about Firebase integration which facilitates a smoother Android application development journey. Remember, patience and a keen eye for detail are key elements when handling these challenges.
For more detailed information about Firebase and troubleshooting, the official Firebase Documentation designed by Google for developers (https://firebase.google.com/docs) is an excellent resource to explore.
There’s a growing necessity to integrate Firebase into applications due to its plethora of services, such as authentication, real-time database, and others, that enhance the apps’ efficiency and functionality. Therefore, having a deep understanding of the potential errors like E/FirebaseInstanceId Failed To Get FIS Auth Token will undoubtedly empower developers to build more robust and feature-rich applications, ensuring users get the best experience possible from their applications.