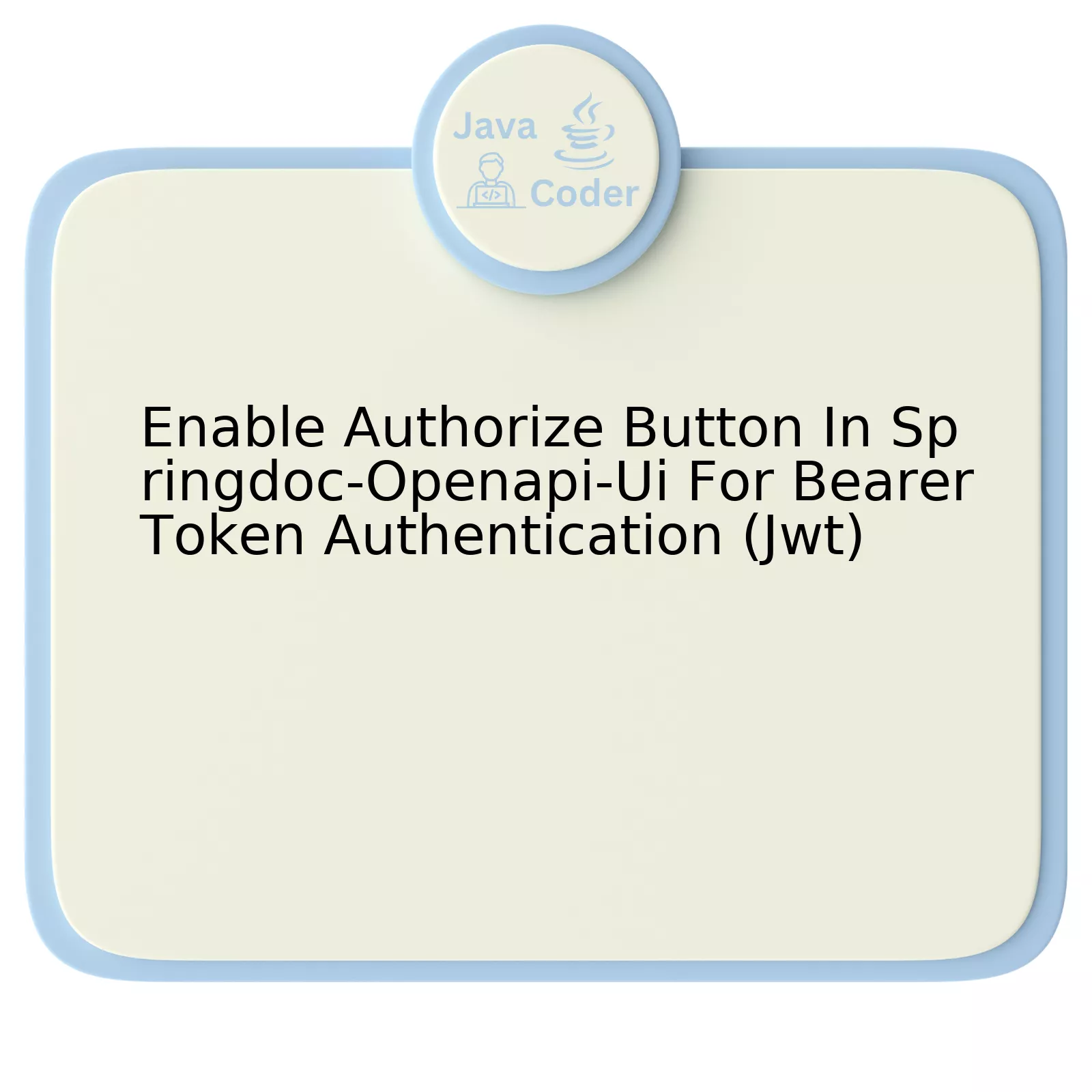
To enable the “Authorize” button in springdoc-openapi-ui for Bearer token authentication (JWT), we need to follow a series of steps which involve working with Spring Security and setting a Security Scheme for our OpenAPI documentation. Below is a detailed tabular representation of the process:
html
Step | Description |
---|---|
Add Springdoc OpenAPI UI dependency | Ensure to add the springdoc-openapi-ui dependency in your project’s build tool. |
Configure Spring Security | Your application should be configured to use Spring Security, allowing you to handle security aspects such as Authorization and Authentication. |
Set a Security Scheme for OpenAPI | Establish a SecurityScheme of type HTTP bearer in the OpenAPI configuration. This scheme will act as a layer showing how API keys are sent and from where they are read. |
Create & Link Security Requirement | Create a SecurityRequirement object and link it to the previously defined SecurityScheme within your OpenAPI customizer bean. It informs that a specific operation or entire API uses applied Security Schemes. |
The first step requires the integration of springdoc-openapi-ui dependency into your Java project. This feature is typically included in pom.xml in Maven or build.gradle in Gradle accordingly.
On properly configuring Spring Security, JWT Bearer token authentication will be enabled and this lays the groundwork enabling the ‘Authorize’ button in the OpenAPI User interface.
Next, implementing OAuth2 on top of Spring Security becomes important as you have to establish a `SecurityScheme` setting. Termed ‘HTTP bearer’, this protocol adds another layer to ensure that the API keys are secure and cannot be misused.
SecurityScheme securityScheme = new SecurityScheme().type(SecurityScheme.Type.HTTP).scheme("bearer").bearerFormat("JWT");
For the final step toward activating the ‘Authorize’ button, you create a `SecurityRequirement` object linking it to the previously defined SecurityScheme. How do you go about this? An instance of this requirement needs to be added to the list of global security requirements. Once accomplished, this communicates that an individual operation or the complete API has successfully implemented predefined Security Schemes.
SecurityRequirement securityItem = new SecurityRequirement().addList("JWT");
By detailing these concepts, we can see insights from popular tech personalities like Bill Gates who stated, “With great power comes great responsibility”. This speaks volumes especially in the context of handling secure user data and ensuring appropriate measures are taken to safeguard information as well.
Understanding JWT and Springdoc-openapi-ui Integration
Web token authentication, particularly that of JSON Web Tokens (JWTs), comes in handy for securing web applications and APIs. JWT technology allows a server to create a signed token upon user login, which is subsequently authenticated throughout the session until it expires. This approach eliminates the need to maintain and check client sessions on the server-side, increasing scalability.
When integration springdoc-openapi-ui and JWT happens correctly, an “Authorize” button shows up on the UI, facilitating Bearer token authentication.
Following are the concise and specific steps you’ll need to enable the “Authorize” button in springdoc-openapi-ui:
Firstly, configure Spring Security and integrate JWT into your Java Spring Boot project. Here’s a basic example from jwt.io:
@Configuration @EnableJwtTokenValidation public class JwtConfig { @Bean public IJwtProvider jwtProvider() { return new DefaultJwtProvider(); } }
This setup validates incoming JWTs. For inclusive details on setting up Spring Security with JWT, view this guide on devglan.com.
Secondly, add the appropriate “security scheme” to OpenAPI configuration using the `springdoc-openapi` library. ‘bearerFormat’ should be ‘JWT’ and ‘scheme’ should be ‘bearer’. Here is a code example:
OpenAPI customOpenApi() { Info applicationApiInfo = new Info().title("Application API").description("Detailed description"); Components components = new Components() .addSecuritySchemes("bearer-key", new SecurityScheme().name("bearer-key") .type(SecurityScheme.Type.HTTP) .scheme("bearer") .bearerFormat("JWT")); SecurityRequirement securityItem = new SecurityRequirement().addList("bearer-key"); return new OpenAPI().info(applicationApiInfo) .components(components) .addSecurityItem(securityItem); }
Lastly, remember these actions generate a Swagger UI “Authorize” button, which expects a Bearer token whenever you attempt to access a secured endpoint. Once you’ve entered the JWT into the required field of the Authorization dialog box, the resulting secured API requests will include the necessary Authorization header, verifying the token before providing access.
To quote Linus Torvalds, “Talk is cheap. Show me the code.” Indeed, it is the implementation that verifies the strength and efficacy of this method, paving the way for secure and scalable web applications powered by JWT and openAPI documentation.
Strategies for Enabling Authorize Button in Springdoc-openapi-ui
Active and expected implementation of JWT token authorization in Springdoc-openapi-ui is a significant task that involves a series of strategic steps. While enabling the ‘Authorize’ button, configurations towards authentication mechanisms like Bearer Token Authentication become required to facilitate adequate user interactions with the Swagger UI.
JWT Authorization Setup
The first part of enabling JWT token authorization in Swagger UI using Springdoc-openapi-ui calls for setting up JWT with the Spring Boot application.
Using a library like Java JWT (jjwt), you can build applications that can generate and verify JSON Web Tokens.
For instance, the following blocks of code illustrate how tokens might be created and validated:
public String createToken(String username) { return Jwts.builder() .setSubject(username) .setIssuedAt(new Date()) .signWith(SECRET_KEY) .compact(); }
Validation could potentially entail something similar to this:
public Boolean validateToken(String token, UserDetails userDetails) { final String username = extractUsername(token); return (username.equals(userDetails.getUsername()) && !isTokenExpired(token)); }
It’s pertinent to note that “SECRET_KEY” represents your selected JWT signature key.
Configuring Spring Security
Post JWT setup, configuring Spring Security ensures secure endpoint access. Implementation typically encompasses an Authentication Filter to authenticate User Requests, an Authorization Filter to establish if authenticated users have specific role access, and a JWT Util class to handle token creation, validation, etc., as initially discussed.
Adding Swagger Documentation for JWT
Following security configurations, integrating JWT with Swagger requires enhancing the API documentation to let users enter their JWT tokens. It involves two critical steps:
– Firstly, define the `SecurityScheme` as shown below:
@Bean public OpenAPI customOpenAPI() { final String securitySchemeName = "bearerAuth"; return new OpenAPI() .addSecurityItem(new SecurityRequirement().addList(securitySchemeName)) .components( new Components() .addSecuritySchemes(securitySchemeName, new SecurityScheme() .name(securitySchemeName) .type(SecurityScheme.Type.HTTP) .scheme("bearer") .bearerFormat("JWT"))); }
– Secondly, apply the `SecurityScheme` to all operation paths you wish to secure by annotating the controller methods or classes with `@SecurityRequirements`, referencing the security requirement/scheme you have set up in your OpenApiCustomizer Bean.
Enabling the Authorize Button
Conclusively, upon successfully nailing the earlier steps, your Swagger UI should display an ‘Authorize’ button. Clicking on the ‘Authorize’ button should activate a user prompt for entering their JWT token—the entered token authenticates subsequent requests to the secured endpoints from Swagger UI.
Remember, these strategies capture IoT trends where they “have taught us anything; it’s that securing such systems isn’t just about protecting data, but life and property too.” – Bruce Schneier on Technology. Thus, highlighting the importance of security measures, like JWT token implementation, in modern applications.
Best Practices for Bearer Token Authentication with Springdoc-openapi-ui
Bearer Token Authentication is an acclaimed method within the classification of HTTP Authentication schemes. It transfers the credentials through HTTP headers from the client to inspect the access rights of the caller over the resources provided by the server-side application. The case in point we will be focusing on here involves Springdoc-openapi-ui’s authorization button which facilitates Bearer Token Authentication.
One of the widely applied methods for accomplishing this purpose involves Java Web Tokens (JWT). In tighter security scenarios, JWT has proven its effectiveness by providing an authorize button immediately accessible from the Swagger UI rather than sending it manually with each API request.
Springdoc-openapi-ui integration with Spring Security, particularly for JWT Authorization, complies with a step-by-step progression of instructions:
Integration of Spring Security
Core initial stage pertains to the integration of Spring Security into your Spring Boot application, a prerequisite for JWT implementation. Maven dependencies set requirement:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
This dependency will load all requested details about user authentication and role-based-custom access rights over defined URLs.
JWT Configuration
Setting up the JWT configuration includes validating the JWT token and retrieving user claims from the token. This process assimilates subsequent steps:
- First, define the secret key – JWTS “JSON web token signature”- in your configuration file.
- Next, illuminate how to extract user information from the token in a Filter implementation. As part of this extraction, perform a check to ensure the token is valid via SignatureAlgorithm.HS256.
Swagger Configuration
To unlock the Authorize button on your Swagger UI, adjustments are mandatory through Swagger Configuration files bearing multiple influencing elements. These include:
- An instance of OpenAPI
- SecurityScheme components
- SecurityRequirement around operations
Lastly, as per Bill Gates’ assertion, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.”
To embody this tenet, utilize Springdoc-openapi-ui’s Bearer Token Authentication strategy to automate and boost your project’s efficiency. Albeit intricate, a proficient alignment between Springdoc-openapi-ui and JWT ensures streamlined security measures without undermining the system’s speed or flexibility. More detailed information on setting up these configurations can be found on the official Springdoc documentation page .
Do keep in mind that while implementing these practices, it is critical not to expose sensitive information like passwords and tokens unintentionally and ensure strict access controls are implemented.
Addressing Common Challenges in Implementing JWT Bearer Token Authentication
JWT (JSON Web Token) Bearer Token Authentication is a widely used method for securing web services. However, there are common challenges faced in implementing JWT Bearer Token Authentication that developers have a keen interest in overcoming.
JWT Bearer Token Authentication Challenges | Solutions |
---|---|
Expired Tokens | Tokens should be timely renewed or token expiry time should be monitored closely. |
Loss of Token | Use HTTPS to ensure tokens are transmitted securely over networks and vulnerabilities are reduced. |
Token Storage | Store tokens securely using HTTP-only cookies to prevent Cross-Site Scripting(XSS) attacks. |
The relevance of these challenges becomes apparent when you work with the Springdoc-Openapi-Ui library, which is utilized for documenting RESTful APIs and offers interactive features including an Authorize button for JWT.
To Enable the Authorize Button in Springdoc-Openapi-Ui for Bearer Token Authentication (JWT), make use of the OpenAPI functionality provided by the Springdoc library. Follow this simple guide:
1. Add Springdoc’s library to your project dependencies.
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-ui</artifactId> <version>{springdoc-version}</version> </dependency>
2. Using the @OpenAPIDefinition annotation, you can define your API info and configure a SecurityScheme for JWT. Specify type as “http”, scheme as “bearer”, and bearer format = “JWT”.
@OpenAPIDefinition(info = @Info(title = "My API", version = "v1"), security = @SecurityRequirement(name = "bearerAuth")) @SecurityScheme( name="bearerAuth", type = SecuritySchemeType.HTTP, scheme = "bearer", bearerFormat = "JWT" )
Overcoming these challenges and building atop this knowledge broadens your programming expertise horizon. As Steve Jobs once said: “‘Innovation distinguishes between a leader and a follower.'”
You may refer to this comprehensive online resource of springdoc-openapi documentation here.
Springdoc-openapi-ui is a fantastic library that helps to automatically generate an OpenAPI documentation for Spring Boot applications. Importantly, it also aids in enabling the Authorize button for Bearer Token Authentication, commonly known as JSON Web Tokens (JWT).
When dealing with JWT, the key is ensuring a secure method of transmitting sensitive information between parties. It encodes this information using a secret, and can be signed either using a secret with the HMAC algorithm, or a public/private key pair using RSA or ECDSA.
@Override public void addResourceHandlers(ResourceHandlerRegistry registry) { registry.addResourceHandler("swagger-ui.html") .addResourceLocations("classpath:/META-INF/resources/"); registry.addResourceHandler("/webjars/**") .addResourceLocations("classpath:/META-INF/resources/webjars/"); }
Springdoc-openapi-ui equips you with the ability to enable the “Authorize” button through its robust customization capabilities, thereby assisting developers in testing their APIs while staying within the security confines of JWT.
For JWT token incorporation into your resource requests:
– Add the following dependency to your project:
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-ui</artifactId> <version>{version}</version> </dependency>
– Normalize the paths, grouping them by path packages and not by tags, providing a more compact view of operations.
Keep in mind to store the JWT securely so it doesn’t leak, resulting in unauthorized access to confidential data. Also, make sure to refresh these tokens periodically to maintain an active user session and protect against token theft.
As Brian Behlendorf, one of the primary developers of the Apache HTTP Server once said, “The only truly secure system is one that is powered off, cast in a block of concrete and sealed in a lead-lined room with armed guards.” Therefore, our pursuit of systems and processes should be to come as close to this ideal state without sacrificing usability.
While handling JWT authentication with Springdoc-openapi-ui, remember to prioritize security and regularly review configurations. With this, you will facilitate a secure, smooth user experience, while enriching your OpenAPI documentation.Reference