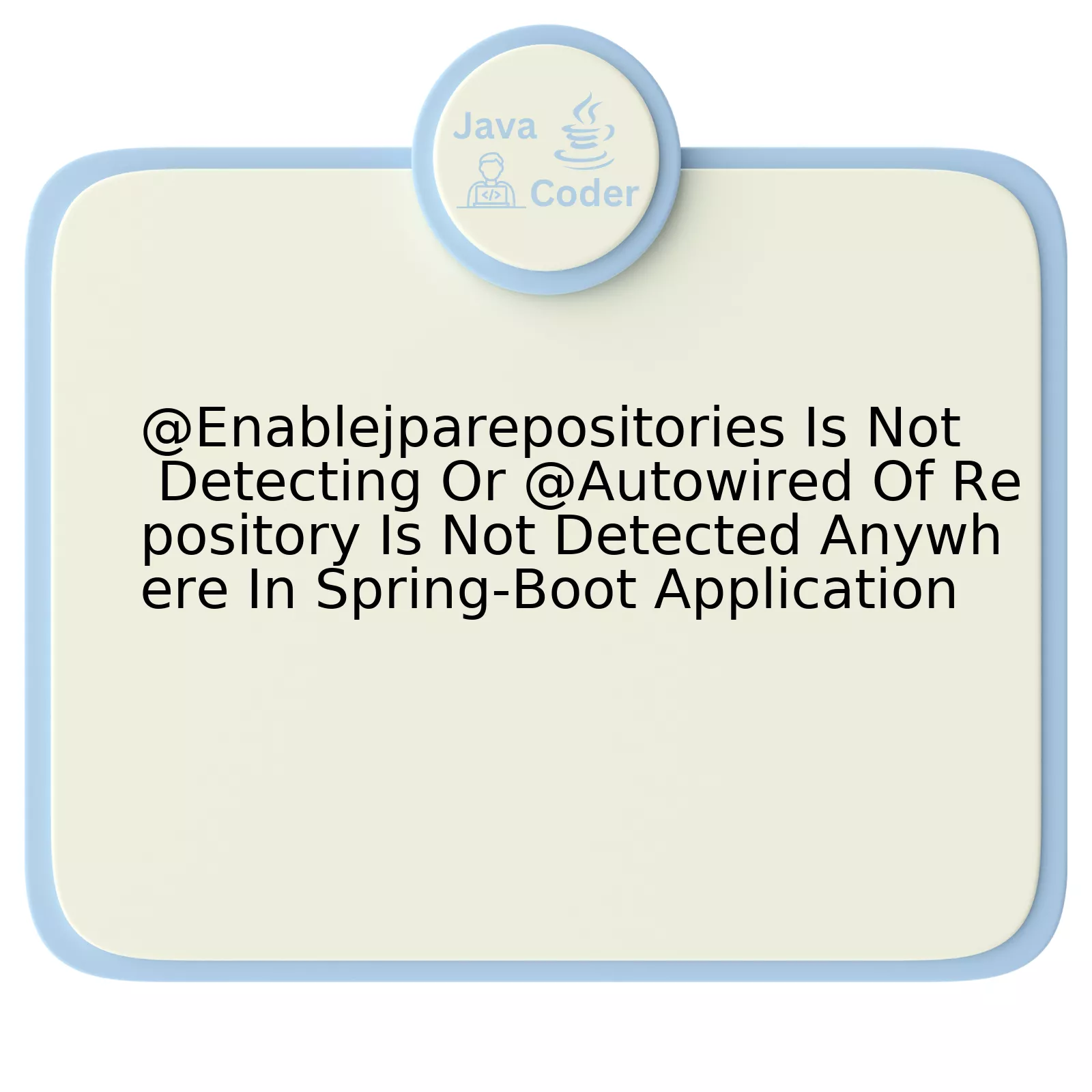
This issue of `@EnableJpaRepositories` not being detected, or `@Autowired` of Repository not being detected anywhere in a Spring-Boot application is a common problem Java developers encounter. Let’s first understand the key components involved:
Annotation | Description |
---|---|
@Autowired |
This annotation allows Spring to resolve and inject collaborating beans into your bean. |
@EnableJpaRepositories |
This annotation activates the JPA repositories. It’s mandatory if you’re using JPA repositories. |
Now, what could possibly lead to the non-detection of these annotations? Well, several reasons could contribute to this conundrum:
- Scan setting mismatch: Spring Boot relies heavily on classpath scanning to auto-configure components. If for any reason, the repository package falls outside the scan range of the main Spring Boot application class, or if the setup for component scanning isn’t done correctly, then it’s likely that the Repository isn’t being detected.
- Improper configuration:
Whether you are utilizing a traditional Spring setup or more modern Spring Boot setup, getting the paths configured incorrectly in your `@Configuration` file can lead to null instances of your repository, even when the `@Autowired` annotation is utilized as intended. - Naming conventions: Java, much like any other language, has its own naming conventions. Your Repository interface must end with the “Repository” suffix, otherwise Spring Data JPA may not recognize the interface as a Repository.
- Incorrect import: Occasionally, an incorrect import such as importing `javax.transaction.Transactional` instead of `org.springframework.transaction.annotation.Transactional` could also lead to such an issue.
As Martin Fowler famously said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This emphasis on understanding means carefully checking our configurations, paths, and conventions to ensure all components are functioning together in a well-understood manner.
To rectify these issues, here’s what you can do:
- Ensure proper package structuring and do make sure your main Spring Boot application class is placed in the root package above other classes.
// Ensure Spring Boot Application Class is in Root Package package com.example; @SpringBootApplication public class MainApplicationClass { ... }
- You can explicitly define the base packages to be scanned using `@ComponentScan` or `@SpringBootApplication(scanBasePackages = {“com.package”})`. Alternatively, utilize `@EnableJpaRepositories(basePackages ={“repository.class.path”})` to help Spring locate your repository interfaces.
- Check your naming conventions. The Repository interfaces should end with “Repository” suffix.
- Make sure you have imported the correct dependencies in your project’s build automation tool (like Maven or Gradle). To use Spring Data JPA for example, the spring-boot-starter-data-jpa dependency needs to be included.
By rectifying these simple oversights, we can help Spring locate and identify the repositories without fail, ensuring that our application works as expected.
Understanding the Role of @EnableJpaRepositories in Spring-Boot Applications
The
@EnableJpaRepositories
annotation is an essential feature that is found in Spring Boot applications. It serves as the pivotal point for integrating the Java Persistence API (JPA) with Spring, thereby enabling your application to interact seamlessly with your database.
The primary role of
@EnableJpaRepositories
is to activate the creation of Spring Data JPA repository implementations. To elaborate, these repositories are interfaces you define for each entity, and Spring Data JPA automatically generates the implementation at runtime. This means you don’t have to write the boilerplate code related to data access layer operations, making your code more concise and efficient.
However, within the stipulated condition of “@EnableJpaRepositories Not Detecting or @Autowired Of Repository Not Detected Anywhere In Spring-Boot Application”, the issue may likely be a misconfiguration situation. Let’s delve into the possible reasons:
– Incorrect Base Package Specification: You might not have specified the base package(s) correctly where your repositories are located. The annotation needs this information to scan these specific packages for repository interface definitions.
@SpringBootApplication @EnableJpaRepositories("com.example.demo.repo") public class DemoApplication { ... }
In this case, under the
@EnableJpaRepositories
, all your repositories should be located under the package “com.example.demo.repo”.
– Unattended Auto Configuration: While Spring Boot automatically configures your application based on the dependencies you have added, if you have custom configurations (like
@EnableJpaRepositories
) but also have spring-boot-starter-data-jpa dependency on classpath, then remove the unnecessary manual configurations or just add
@EnableJpaRepositories
without any arguments.
– No Repository Marker Interface: The repositories need to extend the Repository marker interface provided by Spring Data. If they don’t, Spring won’t create proxy instances for those.
public interface UserRepository extends JpaRepository{ ... }
– Misplaced Annotation or Application Entry Point: Another common mistake is that your
@EnableJpaRepositories
is not in the same place or package as your
@SpringBootApplication
or sometimes it’s not even in a higher level package. Spring-boot uses this entry point as root to start its recursive component scanning process.
To put it simply, the correct use and configuration of
@EnableJpaRepositories
plays an important role in ensuring the smooth operation of your Spring Boot application. As Rod Johnson said, “Good design is key for long term maintainability. It’s like getting regular oil maintenance done on your car. If you don’t do it, you might find yourself stuck somewhere.”source. So, keep checking and reviewing your configurations and codebase to ensure it conforms to desired design practices.
Investigating Cases Where @Autowired Repository is Not Detected in Spring-Boot
Navigating through instances where Spring Boot does not detect the
@Autowired
repository or the
@EnableJpaRepositories
annotation is not locating the repositories can often be addressed by focusing on three key areas:
• Package Scanning:
By default, Spring Boot performs a package scan starting from the base package where the main application class exists. If your repositories are placed in a package that is not a subpackage of the base location, they might not be picked up. Consequently, it recommends nesting the repository packages under the main application package. Packages should be organized as follows:
mainApp.package |_mainApp.package.controller |_mainApp.package.repository |_mainApp.package.service
In cases where the structure cannot be reorganized, an alternative would be specifying the base packages to scan explicitly using the
@ComponentScan
annotation:
@SpringBootApplication @ComponentScan(basePackages = {"com.organization.repository", "com.organization.other.package"}) public class Application { }
• Repository Detection:
Occasionally, even when package scanning seems correct, Spring Boot might not detect the
@Autowired
repository, possibly due to missing annotations on the repository interface. For optimal Spring Data JPA interaction, you should annotate repository interfaces as
@Repository
and extend
JpaRepository
or its superclasses. Here’s a sample interface:
@Repository public interface SampleRepository extends JpaRepository<SampleEntity, Long> { //define additional methods here }
• Application Configuration:
Cases where
@EnableJpaRepositories
doesn’t seem to detect the repositories may stem from inadequate or incorrect configuration information provided in the application properties file. Cross-check with the connectivity information and entity model settings to ensure everything is correctly configured.
Remember, the goal is compatibility and smooth interfacing between Spring Boot, Spring Data JPA, and the underlying database. Alan Kay, one of the foremost thinkers in technology iterates, “Simple things should be simple, complex things should be possible.”. It’s about bringing this ethos to any roadblocks encountered – tackling them systematically, making debugging simpler, thus paving the way for more complex explorations.
Common Issues and Solutions when using @EnableJpaRepositories & @Autowired Repository in a Spring-Boot Application
The issues you’re experiencing with
@EnableJpaRepositories
and
@Autowired Repository
in your Spring-Boot application may come from several factors. Do note that these elements are crucial for establishing relationships between the database layer and your application using JPA (Java Persistence API).
Common Issues
- The primary issue generally lies in package scanning, which might be leading to ‘Repository’ beans not getting detected within the Spring context.
- Rarely, there could be a naming convention mistake or wrong use of annotations, which causes the developer’s intended functionality to go amiss.
- A third common error happens when the Repository isn’t an interface. JPA repositories must always be interfaces rather than classes.
- Lastly, configuration problems in the build file can also cause
@EnableJpaRepositories
or
@Autowired Repository
to function improperly.
Solutions
To resolve the above issues, consider the following actions:
Correct Package Scanning
Ensure that
@EnableJpaRepositories
is set properly. By default, it scans the current package and sub-packages. If your Object-Relational Mapping (ORM) resides outside, specify the base packages to include them.
Thus, instead of using:
@EnableJpaRepositories
Use:
@EnableJpaRepositories(basePackages={"com.example.package1", "com.example.package2"})
Replace ‘com.example.package1’ and ‘com.example.package2’ with your actual package names.
Naming Conventions & Annotation Usage
Check to make sure your repository interface name follows Spring’s convention, i.e., [EntityName]Repository. Ensure correct use of
@Autowired
and
@EnableJpaRepositories
. A general rule of thumb is to use
@Autowired Repository
only in Service or Controller layers, but not inside the entity model.
Interface Implementation Problem
Confirm whether your repository is implemented as an interface, not a class, and extends JpaRepository
For example:
public interface UserRepository extends JpaRepository{}
Adjust Build Configuration
Lastly, check your ‘pom.xml’ or ‘build.gradle’ for any discrepancies. Are all necessary dependencies included? Is the Spring Boot version compatible with the Java version used?
Remember Mark Twain’s wise words – “The secret of getting ahead is getting started. The secret to getting started is breaking your complex overwhelming tasks into smaller manageable tasks, then starting on the first one.”
Spring Data JPA – Reference Documentation
IntelliJ cannot Autowire spring repository – Stack Overflow
Improving Detectors for Repositories: A Deeper Look at Annotations in Spring Boot
Detecting repositories in Spring Boot applications is a consistent aspect of constructing efficient and dependable software. Such configurations are typically employed in the structuring of numerous applications. However, developers frequently face dilemmas when the `@EnableJpaRepositories` annotation declines to recognize repositories or `@Autowired` of the repository isn’t detected anywhere within the Spring-Boot application. This situation frequently results from subtle inconsistencies in your project structure or misunderstanding how Spring Boot deploys annotation-based configurations.
Understanding Annotations in Spring Boot
Your Spring Boot application leverages different annotations for implementing various roles:
- Firstly, the `@EnableJpaRepositories` annotation is engineered for enabling JPA repositories. This instruction ought to be placed on a configuration class that will kick-start your background persistence layer.
- Next up, we have the `@Autowired` annotation. This enables you to inject object dependencies seamlessly. When you employ Spring’s dependency injection, this annotation guides the framework to utilize the setter approach, constructor, a field, or strategies for injecting dependency on beans.
Unfortunately, at times, your Spring Boot application might not detect these annotations – and this can present significant issues.
Addressing Annotation Detection Issues in Spring Boot
If your Spring Boot application is encountering difficulties detecting these annotations, there are methodical tactics that you could deploy to troubleshoot:
• Package Structure:
Ensure your main application file exists in a root package above other classes. When `@SpringBootApplication` (a combination of `@Configuration`, `@ComponentScan` and `@EnableAutoConfiguration` annotations) is set on your main class, Spring Boot performs an automatic scan on the package containing the class and its sub-packages.
Below is an illustration of a correct package hierarchy in Spring Boot:
com +- example +- Application.java +- domain +- Customer.java +- CustomerController.java +- CustomerService.java +- CustomerRepository.java
This package structure aids Spring Boot’ automatic component scanning feature, thus ensuring that your repositories and services are properly detected.
• Manually Defining Repository Base:
In unique instances where automatic detection fails, manually defining the repository base for `@EnableJpaRepositories` can resolve the problem. You would do this by indicating the comprehensive package to scan for repositories.
Here’s an instance showcasing such usage:
@EnableJpaRepositories(basePackages = "com.example.project.repository")
This directs Spring Boot to exclusively scan the designated package for repositories, consequently augmenting the chances of the repository being detected.
According to James Gosling (creator of Java), “So much of what software development is, is surfing right on the edge of complexity”. Struggling with annotation detection may initially appear overwhelming, but with the correct understanding of Spring Boot and its operation procedures, troubleshooting becomes markedly more simplified. It always involves performing diligent diagnostics while bearing in mind the core dynamics that drive annotation deployments in your application. However, comprehending the annotation intricacies in the Spring framework, and, indeed, tackling the rebellious behaviour of the `@EnableJpaRepositories` or `@Autowired` annotations, illustrates the reality of Gosling’s point – possibly serving as another wave for us to conquer in our quest to better surf the complexity inherent in developing software.
Addressing the subject of @Enablejparepositories not being detected or @Autowired of Repository not being identified in a Spring Boot Application entails discussion regarding the accurate configuration, placement, and usage of these annotations in the context of this framework.
Hosting the Annotations Incorrectly:
@SpringBootApplications public class Application { }
Spring Boot applications typically follow the annotation-based configuration approach. The @SpringBootApplication is a convenience annotation that adds all of the following:
- @Configuration: Designates this class as a configuration class (i.e., Beans are instantiated, assembled, and managed by Spring IoC container).
- @EnableAutoConfiguration: Instructs Spring Boot to start including beans based on classpath settings, other beans, and various property settings.
- @ComponentScan: Tells Spring to look for other components, configurations and services in the corresponding package.
It’s important to place the main application class in a root package above other classes.
Mishandled Configuration:
The @EnableJpaRepositories annotation is used only when you want to customize the base repository otherwise, it’s optional. This annotation will configure the base packages where Spring should scan to find the interface, which extends JpaRepository.
Ensuring the Correct Use of @Autowired:
In order for the @Autowired of Repository not being detected issue, understanding the fundamental working principle of Spring’s dependency injection is crucial. @Autowired works by Injecting collaborating beans into our bean. If you have properly defined beans and your Application class files sit appropriately within the classpath, then it should work as expected.
Tim Berners-Lee, the inventor of the World Wide Web once said, “You affect the world by what you browse.” Coding could be paralleled with this statement implying that, just as how browsing affects a larger ecosystem, how annotations are used in coding can make significant impacts in the outcome of an application.
For future reference or enhancement of your knowledge on Spring Boot Application and its annotations, the official guidelines from Spring Data JPA documentation might come handy.
Effective utilization and proper coding posture aimed at @Enablejparepositories and @Autowired can substantially increase productivity and efficiency, clearing any hurdles related to non-detection that this Spring-Boot application project might encounter.