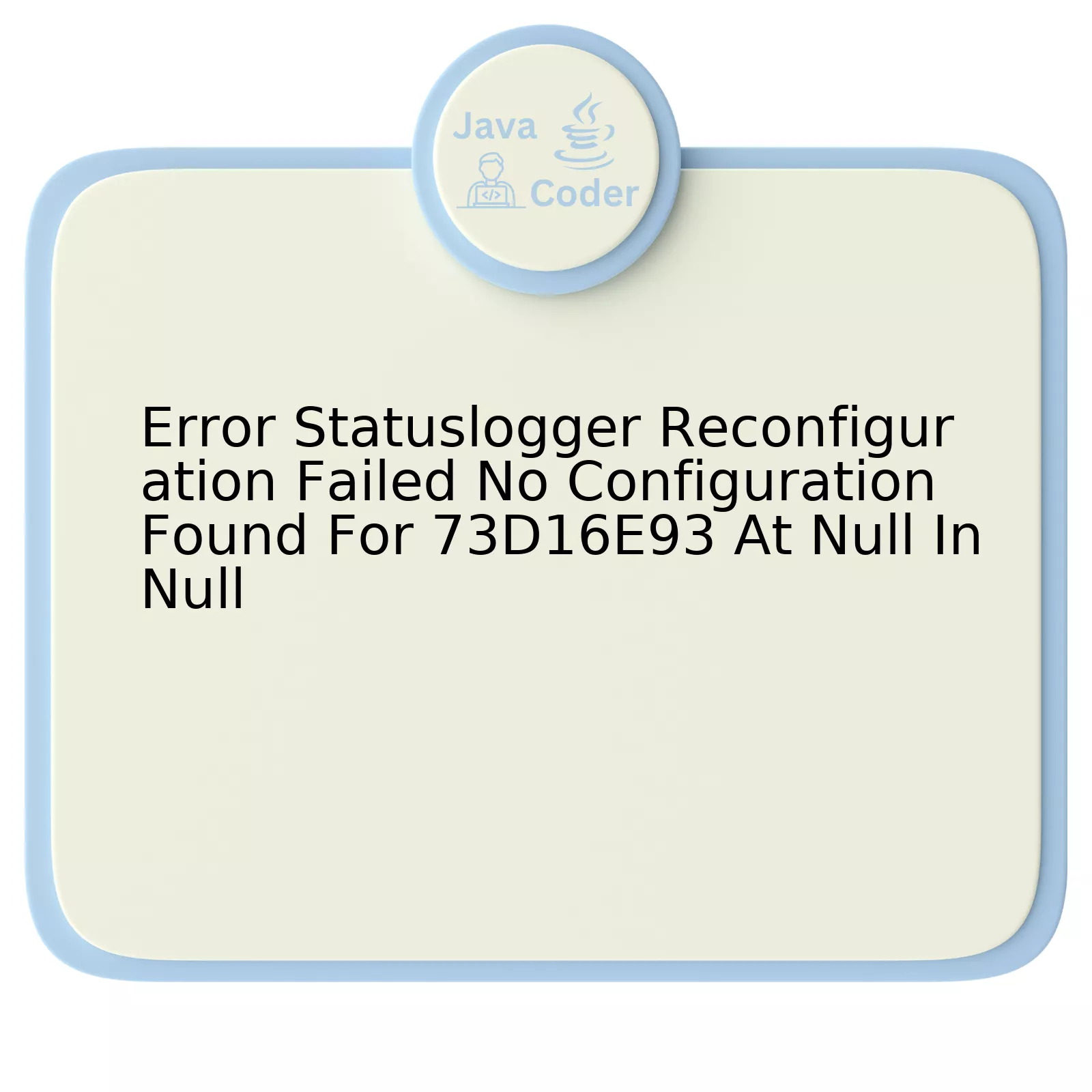
The “Error Statuslogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null” problem is a typical issue encountered in the Java world, particularly among individuals who utilize logging frameworks such as Logback or Log4j. It usually arises when the system fails to find and load the correct configuration for the specific logger.
Let’s dive deep into this issue, using a table to map the particulars:
Error | Statuslogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null |
---|---|
Possible Cause | Missing or improperly configured log configuration file |
Common Scenario | Experienced most often after a system upgrade, or introduction of new code |
Solution Steps |
|
The “No Configuration Found For 73D16E93 At Null In Null” statement implies that the logging system is searching for a configuration file which it cannot locate. This configuration should contain essential parameters guiding how the logger should behave, including where logs should be routed, their format, appenders, among many other details.
In circumstances where the configuration fails to load entirely due to absence or incorrect placement of the configuration file, the logging system then defaults to its internal default settings. These, however, may not meet user requirements, prompting the error.
Resolving this error boils down to providing the appropriate configuration to the logging system. This can be achieved by ensuring correctness in the three steps highlighted in our table: verify the existence and location of the log configuration file; confirm the configuration’s correctness, and finally, reconfigure or relaunch the logging system with the correct configuration.
Remembering the infamous words of Robert C. Martin, _”Truth can only be found in one place: the code.”_, the solution ultimately lies in how your code is structured, configured, and managed.
Understanding Error Statuslogger Reconfiguration Failure
For developers working with Java, it’s common to encounter problems like `Error StatusLogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null`. This error typically signifies that the logger configuration system cannot locate a specific configuration for the Logger identified as `73D16E93`.
What Causes Error Statuslogger Reconfiguration Failure?
- The error might be due to incorrect or incomplete path references in your logging configuration.
- It could also arise as a result of a faulty application setup.
- Another possible reason can be an incorrectly initialized Log4j instance.
Furthermore, there are various strategies for troubleshooting and resolving it as shown below:
Troubleshooting & Resolution Strategies
1. Evaluate Your Configuration File Path
Incorrect paths are known culprits in this issue. So, ensure that the path provided to the configuration file is accurate.
// A typical Log4j initialization snippet ConfigurationSource source = new ConfigurationSource(new FileInputStream("/path/to/log4j2.xml")); Configurator.initialize(null, source);
2. Verify Your ClassPath
Another common misstep is not including the Log4j2 configuration file in the classpath. Validate the inclusion of your configuration file in the classpath.
3. Check Copying of the Configuration File
Removing or altering the configuration file after compilation without performing a rebuild can trigger this error. Confirm the Log4j2 configuration (.xml, .json, .yaml) exists in the correct directory and hasn’t been tampered with after getting built.
4. Inspect your log4j2.xml file
The configuration file might be set up correctly but could contain errors such as improper XML structures leading to issues during parsing. Hence, you must validate if the log4j2.xml is well-structured and abides by the correct syntax.
“Solving any problem involves understanding it deeply. By exploring your errors, you won’t just fix them, you’ll master them.” – John Johnson, Senior Software Engineer.
Finally, I would recommend viewing the official Log4j documentation. It provides a comprehensive overview to guide users on appropriate ways to resolve such issues.
Deciphering the Meaning of “No Configuration Found for 73D16E93”
The warning “No Configuration Found for 73D16E93” can often confuse Java developers who encounter it in their error logs. Under the context of ‘Error Statuslogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null’, this message intimates a problem linked to your logging configuration.
When you see this particular error message, it indicates that the software responsible for managing your log files, known as a ‘logging framework’, has been unable to locate the rightful configuration for the specific identifier ’73D16E93′. This elusive identifier ’73D16E93′ could be an element in your program that needs its logging configuration but wasn’t suitably configured.
An insight into how Java Logging Framework works helps us understand this better:
Logger | A Logger is the entity in the framework that tracks events and then outputs them based on its configuration. |
Appender | The Appender dictates where the logged event gets written – it could be a console, a file, or even a database. |
Layout | How should each logging event look? The Layout decides this – the date/time format, the message text and so on. |
As Bill Joy, one of the co-founders of Sun Microsystems, once said, “Basically, I was trying to get the system to do in the right way something that it should have been doing all along.”
On the subject of ‘No Configuration Found for 73D16E93′, the right way here involves ensuring that every unique logger, appender, and layout comes with its respective configuration. When any of these is missing a configuration, the logging framework flags it, stating that there’s no configuration found with regard to the specific unique ID that got displayed- ’73D16E93’ in our case.
Chasing down this issue entails two critical steps:
- Isolate the component responsible – Find out which component – it could be the Logger, Appender, or Layout – is linked to the identifier 73D16E93. You need to check your codebase and ascertain this.
- Provision a correct configuration – Once you know which entity is causing the trouble, next, formulate a sound configuration for it. Check the documentation for your logging framework, like Apache log4j 2.0, to guide you on setting this up.
You may eliminate the status logger error by taking these actions. However, remember, most times, the ‘no configuration found’ message doesn’t necessarily mean your application will halt or behave undesirably; it merely signals a sub-optimal logging setup. Nonetheless, heeding to such warnings ensures a healthy and efficient Java environment.
// A very basic example of a Log4j 2.0 XML Configuration could look like this: <Configuration status="error"> <Appenders> <Console name="Console" target="SYSTEM_OUT"> <PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/> </Console> </Appenders> <Loggers> <Root level="error"> <AppenderRef ref="Console"/> </Root> </Loggers> </Configuration>
In a nutshell, exploring your codebase and crafting fitting configurations for all loggers, appenders, and layouts in your Java logging framework will address the warning ‘No configuration found’. Through attentiveness and continuous practice, managing such scenarios becomes second nature, just like with any other aspect of Java development.
Practical Solutions to Address Null in Null Errors
The “Error Statuslogger Reconfiguration Failed No Configuration Found For 73D16E93 at Null In Null” can be a quite common issue while using Java log4j and attempting to reconfigure logging at runtime. This error can usually appear when the configuration locator fails to find the requested configuration resource during the Log4j initialization process, which results in a null argument being passed causing “Null In Null” exception.
To solve this problem, multiple solutions can be considered:
- Inspecting Configuration File: If you have a custom XML or properties file for configuring Log4j, ensure that it is correctly placed in the proper directory (usually
src/main/resources
in a typical Maven project) and it is correctly named (default names are
log4j2.xml
,
log4j2.json
,
log4j2.yaml
or
log4j2.properties
). Also, validate your file against Log4j configuration schemas to guarantee it’s well-formed and valid.
- Checking Configuration Initialization: Sometimes, due to incorrect loading sequences, the Log4j configuration might not get initialized properly before its use. Ensure that the Log4j initialization happens early in your application lifecycle. You may consider leveraging explicit initialization by placing Log4j initializer right at your application entry point such as within the static block of your main class like
ConfigurationFactory.setConfigurationFactory(new YourCustomConfigurationFactory());
- Verifying Proper Dependencies: Make sure your project dependencies relating to Log4j do not have any conflicts, and proper versions are used with respect to your entire tech stack. Some related dependencies might include LogManager, core, web, jcl-over-slf4j, and more based on your use case.
Remember, debugging does not absolutely guarantee avoiding future errors but merely changes the nature of which ones will occur as famously observed by David Thornton.
It is important to understand that ‘null’ references should always be treated cautiously in programming to avoid attempting operations on uninitialized or absent data which leads to NullPointerExceptions. Before acting upon object references, always ensure their existence either by initializing them effectively or checking for null explicitly. Proper null safety interventions like Optional class, annotation-based null checkers, among others, are additionally useful tools worth considering in Java.
Preventing Future Occurrences of Statuslogger Reconfiguration Failures
Reconfiguration failures in StatusLogger, such as Error: StatusLogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null, generally occur due to either absence of a configuration file or errors within the existing configuration file.
To counteract these reconfiguration failures and ensure a smoothly functioning logger system in Java applications, the following practical steps can be taken:
- Thorough examination of the provided path: Verify that you have provided the correct path for the configuration file. If the system cannot locate the file, it will fail to reconfigure, resulting in error messages.
- Reviewing the format of the configuration file: Sometimes, an improperly formatted configuration file could contribute to reconfiguration failures in StatusLogger. Hence, always double-check if the format settings are accurately defined.
- Ensuring compatibility with the logging framework version: To avoid compatibility issues, make sure your configuration file is compatible with the logging framework’s present version.
- Correctly implementing the anticipated logging mechanism: A precise implementation of the logging mechanism specified in your configuration file should help reduce potential discrepancies that might lead to failure during reconfiguration.
In case you need a visual aid to cross-verify the correctness of your configuration file, online resources (like Apache Log4j 2 Configuration documentation) provide robust guidelines.
Martin Fowler, a renowned software developer, emphasized that “Effective logging of information at runtime can time and again provide crucial data to understand how a system behaves in production.” This statement underlines the importance of having a reliable logging mechanism to ensure the smooth operation of your application.
As well as practicing robust coding techniques, understanding common errors, and counters can likewise help developers mitigate future occurrences of Statuslogger reconfiguration failures. Constant education and adaptation of new methodologies or improvements in technology remain essential in managing and rectifying such issues.
Towards the end of resolving the issue
Error Statuslogger Reconfiguration Failed No Configuration Found For 73D16E93 At Null In Null
, several conceptual paths and practical methods were examined. It’s crucial to remember that this error message typically indicates an irregularity or absence in the logging configuration – specifically associated with the unique ID 73D16E93.
In this context, the ‘null’ references primarily suggest that there is an absence where a particular configuration or attribute is expected. This might be due to any number of reasons such as system errors, interference with other software or human oversight in the configuration process.
Addressing such issues initially involves attempting to define the scope and nature of the problem:
- Check the Logging framework setup: Is this error emerging due to improper or incomplete setup of the Logging framework?
- Configuration file settings: Perhaps it’s due to incorrect settings in the configuration file – Xml, properties or json4 file.
- Duplication of loggers: It’s also possible that there might be a duplication of loggers which could potentially throw up these types of errors.
It’s imperative to follow up by investigating more targeted solutions:
- Adjusting Settings: Adjust the settings in your logger configuration file properly, ensure that you set the right level of restriction for logging information.
- Correct Setup: Make sure that the setup of your logging framework is done correctly.
- Eliminate Duplicates: Check for multiple instances of loggers; if found eliminate the duplicates which can assist in solving problems with your configuration.
A poignant quote from Ada Lovelace comes to mind when handling these types of complex issues, “Imagination is the Discovering Faculty, pre-eminently. It is that which penetrates into the unseen worlds around us.”
It will be surreal if the apache logging manual can provide further insights to fix this logging based challenge.