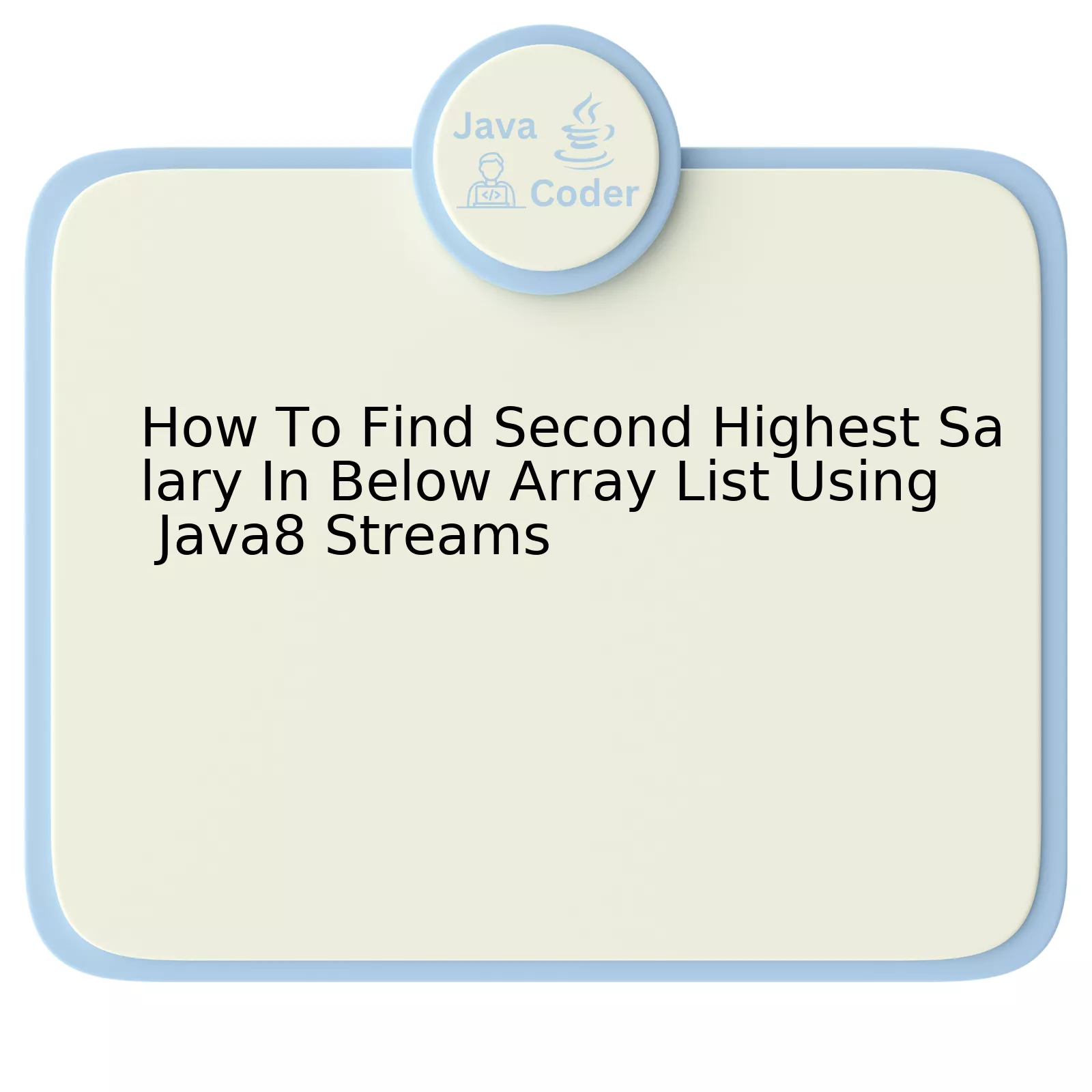
Sure, below is the methodology for finding the second highest salary from a given ArrayList using Java 8 Streams with an illustrative table representation.
Step | Action |
---|---|
Initialize array list of salaries | First, create an object of ArrayList in Java and populate it with salaries. |
Use Stream API | Pipeline the ArrayList through the Stream API offered by Java8. |
Sort the stream | Arrange the data stream in descending order using the sorted operation in combination with Comparator.reverseOrder(). |
Skip elements | Skip the first element as it represents the maximum salary utilizing the skip() function. |
Retrieve second highest | The next element is our target – the second highest salary. |
Let’s take this explanation to code:
ArrayListsalaries = new ArrayList<>(Arrays.asList(10000,20000,30000,40000,50000)); int secondHighestSalary = salaries.stream() .sorted(Comparator.reverseOrder()) .skip(1) .findFirst() .get(); System.out.println("Second Highest Salary is: " + secondHighestSalary);
In this snippet, we start with an ArrayList ‘salaries’ holding integer values representing different salary figures. Then, we convert our ArrayList into a Stream and sort it in descending order. Next, we want to exclude (or skip) the first value which invariably is the highest salary after sorting. The second highest comes in line next. With the aid of findFirst() and get(), we are able to retrieve this second highest salary. We then print it out.
For understanding streams, Bill Joy, one of the co-founders of Sun Microsystems and a key contributor to the development of the Java programming language was quoted saying “if I were to pick a language to use today other than Java, it would be Scala“. Even though he points towards another language, but Stream API added in Java8 provides functionalities similar Scala which reinforces the competitiveness and continuity of Java language.
Therefore, leveraging Java8 Streams can efficiently solve problems related to computing values from collections such as finding the second highest salary. After all, programming languages like Java are meant to make complex computations easy and efficient.
Leveraging Java8 Streams for Salary Analysis
Leveraging the power of Java8 streams, we can conduct an analytical expedition into numerous data-driven tasks, like performing salary analysis. One intriguing task in this arena would be finding the second highest salary in a given array list.
Here’s how we could perform this using Java8 Streams:
java
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.Optional;
public class SalaryAnalyzer {
public static void main(String[] args) {
List
Integer secondHighestSalary = findSecondHighestSalary(salaries);
System.out.println(“The second highest salary is: $” + secondHighestSalary);
}
private static Integer findSecondHighestSalary(List
Optional
.sorted(Comparator.reverseOrder())
.skip(1)
.findFirst();
return secondHighest.orElse(null);
}
}
In above Java8 example, all salaries from the list are streamed, sorted in reversed or descending order using `Comparator.reverseOrder()`, and then the first element (which is now the second-highest salary owing to reverse sorting) is fetched using `findFirst()` after skipping one salary with `skip(1)` which actually is the highest payment.
“When code and comments disagree,” warns Robert C. Martin, “both are probably wrong.” This reflects the importance of writing effective code that reinforces what it sets out to achieve. Using the stream API in Java provides an efficient method to deal with aggregate operations on collections, like finding the second highest salary, expressing complex data processing queries in a simple form.
Note that if the salaries’ list does not contain at least two distinct elements, null will be returned as there is no second-highest value. Therefore, you need to ensure apposite data validity and input checks for production-level codes.
Employing Java8 streams is a compelling methodology in crafting clear, concise, yet tenacious solutions which isn’t only confined to salary investigations, but an extensive range of data analysis applications.
Additional details regarding Java Stream API usage can be accessed on Oracle’s official guide.
Unfolding the Process to Find Second Highest salary
In order to find the second highest salary in an array list using Java 8 Streams, we can follow the beneath process. This will entail three major steps, namely creating a List, sorting it in reverse order, and grabbing the index position.
To start, let’s initially create an
ArrayList
of Integer representing salaries:
List<Integer> salaryList = Arrays.asList(20000,30000,25000,35000,40000);
Secondly, after successfully establishing a list collection with our hypothetical data set on the inclusive salaries, we’ll have to sort that list in a descending order. In Java8’s Stream API, to achieve this we have the power of the integrated sorted function which caters for both natural and custom ordering of data structures like arrays or more importantly, lists. To perform a descending sort on the list, the code syntax would look something like this:
salaryList = salaryList.stream().sorted(Collections.reverseOrder()).collect(Collectors.toList());
Our ArrayList will now look like [40000,35000,30000,25000,20000].
Lastly, the next step requires us to grasp the second highest salary from our newly organized list. Here comes the benefit of zero-based indexing in Java language. The top record, being first from the sorted list sits on position zero (0) and therefore, the second-highest salary is pending at the index one (1). Note, this approach is only good for small datasets as it becomes inefficient with larger collections due to having to sort the entire sequence first; advanced strategizing would be essential for accomplishing efficiency with larger data sets. Check out this highlighted code snippet for the final operation:
int secondHighestSalary = salaryList.get(1);
As Don Knuth, the famed computer scientist, once mentioned, “We should forget about small efficiencies, say, about 97% of the time: premature optimization is the root of all evil.” Despite the inefficient property of the sorting method here with vast data sets, this approach is still fulfillingly valid given that, in this scenario, the salary collection is fairly small.
For more detailed information refer to the official Oracle [Java Documentation](https://docs.oracle.com/javase/8/docs/api/java/util/stream/package-summary.html) to dive deeper into Streams in Java 8.
Java Coding Concepts in Detail: Retrieving Second Maximum Salary
Uncovering the concept of retrieving second maximum salary using Java streams is an engaging task. Powerful and productive features of Java8, especially Streams, have simplified many complex operations including sorting and filtering data in collections. Resolving your query involves understanding the working and usage of these concepts.
Let’s begin by explaining ‘Arrays’ and ‘Streams’:
* Java Arrays: An array is a data structure used to store homogeneous elements at contiguous locations. The size of an array must be specified at time of declaration.
* Java Streams: Introduced in Java 8, the Stream API is used to process collections of objects. A stream is a sequence of objects that supports various methods which can be pipelined to produce the desired result. The key abstraction of java.util.stream package is `Stream
List<Employee> employees = new ArrayList<>(); employees.add(new Employee("John Doe", 20000)); employees.add(new Employee("Jane Doe", 30000)); employees.add(new Employee("Joe Doe", 40000)); employees.add(new Employee("Donald Trump", 50000));
The above snippet describes a list of employees with their respective salaries. To determine the second highest salary from this list, follow these steps:
* First, sort the employee list in descending order on the basis of salary.
ListsortedEmployees = employees.stream() .sorted((e1, e2) -> e2.getSalary() - e1.getSalary()) .collect(Collectors.toList());
In the code snippet above, Java Stream is first converted into a sorter stream of employees based on salary. Next, it is collected back into a list.
* After that, you need to pick the second element from the sorted list.
Employee secondHighestPaidEmployee = sortedEmployees.get(1);
Note that indexes start at 0 with Java, thus, the second element is at position 1.
These are the basics needed for obtaining the second maximum salary utilizing Java8 streams. It’s important to remember that although technology continuously updates, these fundamental concepts will not change.
As widely revered computer scientist Donald Knuth has said,
“People think that computer science is the art of geniuses but the actual reality is the opposite, just many people doing things that build on each other, like a wall of mini stones.” Similarly, Java programming includes understanding numerous small but interconnected concepts that yield powerful functionality.
For detailed exploration, visit Java 8 documentation: Java 8 Stream
Practical Applications of Java8 Streams for Payroll Evaluations
Surely, Java 8 streams provide myriad applications that include the evaluation of payrolls. A key focus for this section is handling an array list containing employee salaries and using Java 8 Streams to determine the second highest salary.
Understanding the Concept
Java 8 Streams introduce a new abstraction – streaming data. This API allows programmers to perform operations on data (like database queries) in a declarative style, known as functional programming.
Streams work by maintaining no internal storage but instead taking input from collections, arrays, I/O channels and generating sequence of elements on demand.
Applying this concept to the task at hand—finding the second highest salary in an array list—we can leverage various methods provided by Java 8 Stream API such as
stream()
,
sorted()
, and
limit()
.
Analyzing the Solution Approach
The approach to find the second highest salary using Java 8 Streams consists of these steps:
1. First, call the
stream()
method on the ArrayList. This converts the collection into a Stream.
2. Next, use the method
sorted(Comparator.reverseOrder())
. This sorts the salaries in descending order. By default, it’ll sort in ascending, so passing Comparator’s reverseOrder() method will sort it in descending order.
3. Now, apply the
distinct()
method. This ensures there are no duplicate values in the stream.
4. Use the
skip(1)
method to skip the first (highest) salary.
5. Finally, the
findFirst()
method will give the second highest salary. If it exists,
Optional.get()
returns the value, else it throws a NoSuchElementException.
Here is example of applying these steps with some sample data:
ArrayList<Double> salaries = Arrays.asList(5000.0,6000.0,3000.0,7000.0,9000.0, 4000.0, 2000.0, 4000.0); |
---|
double secondHighestSalary = salaries.stream().sorted(Comparator.reverseOrder()).distinct().skip(1).findFirst().get(); |
However, keep in mind the trade-off with using Java 8 Streams: they may increase code readability but can also be more complex when debugging or performing stack traces.
“Before software can be reusable, it first has to be usable.” – Ralph Johnson
The process of obtaining the second-highest salary in a given ArrayList using Java 8 streams can effectively be simplified and made very efficient. Employing the concept of Java Streams can result in cleaner, more readable and optimized code which parallels our ultimate goal in any programming task.
To perform this operation we’d use the
stream()
method on our ArrayList. Upon the creation of a Stream object, two essential steps follow: sorting the Stream in reverse order with salaries as the basis for comparison through the method
sorted(Comparator.reverseOrder())
, and then slicing out the desired element – the second highest salary – via combination of the methods
skip(1)
and
findFirst()
. Here is a simple coding illustration:
ArrayListsalaries = new ArrayList<>(Arrays.asList(2000, 5000, 7000, 10000)); int secondHighest = salaries.stream() .sorted(Comparator.reverseOrder()) .skip(1) .findFirst() .get(); System.out.println(secondHighest);
This will print “7000” since it is the second highest salary from the list. The implementation of Java streams in such cases is similar to SQL-based solutions for problems where ranking among data elements is necessary.
Java8 streams are not only efficient but also improve readability and maintainability of code while performing complex operations like finding the second highest salary from a list of salaries. As renowned software engineer Robert C. Martin (Uncle Bob) once said, “The readability of your code can be a matter of life or death”, signifying the importance of readable and maintainable code in any application development [Ref].
It’s crucial to realize that whole power of modern Java features, like Stream API, for data manipulation and transformation. It makes coding much easier and faster, but one has to understand and grasp it fully first. Through the magic of Java 8 Streams, we unlock an approachable pathway to handle large amounts of data without the hassle of traditional methods.
Overall, understanding how to utilize Java8 Streams to find the second highest salary within an ArrayList is immensely useful. It provokes thought around computational efficiency, teaches a great deal about Java’s powerful stream operation, and inspires developers to produce leaner, more effective code.