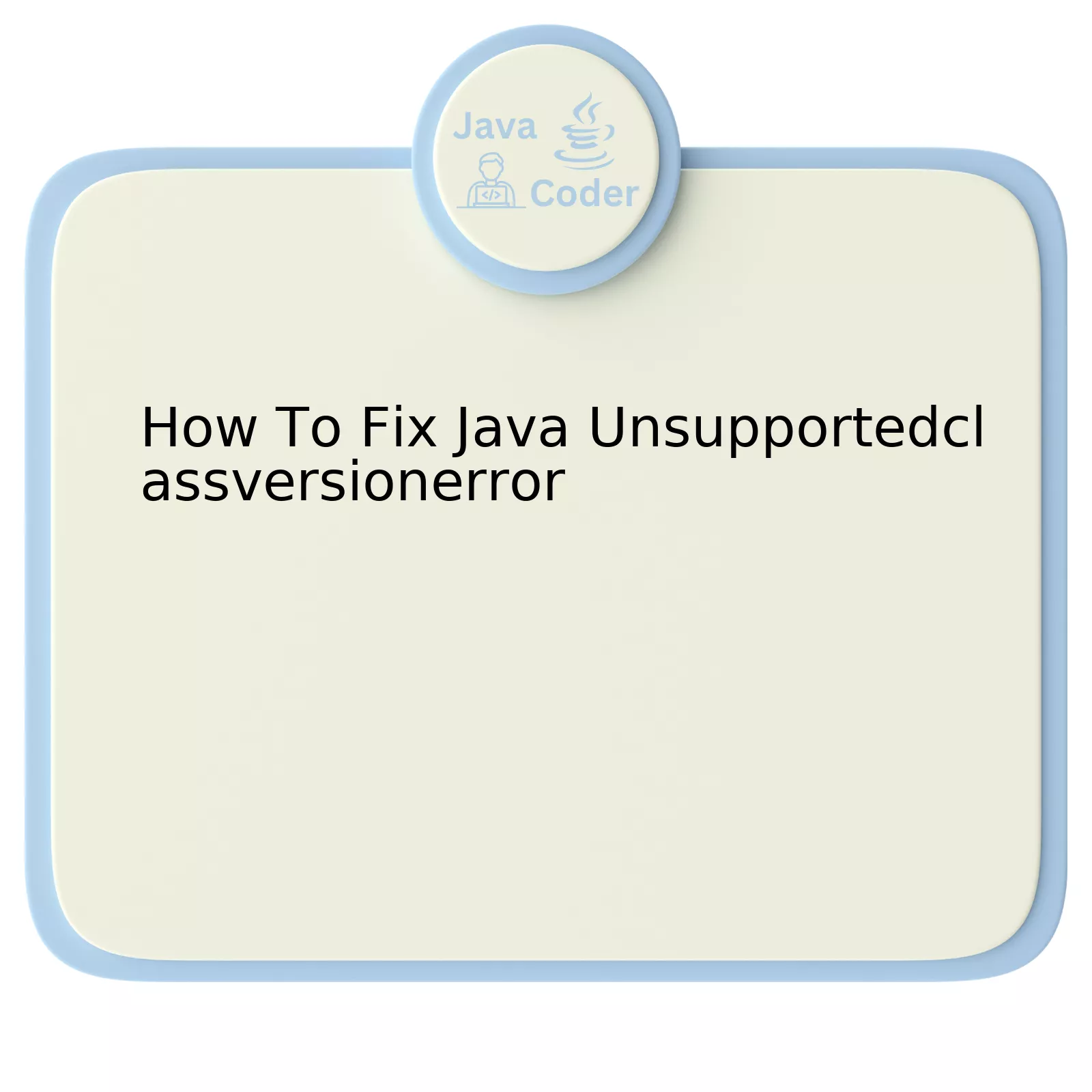
Without question, the Java UnsupportedClassVersionError arises when a class was compiled using a newer version of the Java Development Kit (JDK) and attempting to execute it on an older JVM (Java Virtual Machine). This is to say, for instance, you are trying to run a class that was compiled in JDK 11 environment on a JRE 8 or JRE 10.
Action | Description |
---|---|
Identify Your Java Version | Use terminal command: <code>java -version</code> |
Recompile Your Class in Target Environment | You need to find out which JDK was used to compile your class file. Use the JDK corresponding to the JVM where you are planning to run your code. This can be done by compiling the class with the command: <code>javac -target 1.8 MyNewClass.java</code>. Here, ‘1.8’ is an example target version. |
Upgrade Your Java Runtime Environment (JRE) | If you have no access to the class source code or cannot recompile it, consider updating your JRE or JVM installation to match the JDK version of the class causing problems. |
Allow me to expound on the table above detailing how to fix Java UnsupportedClassVersionError:
– **Identify Your Java Version**: It’s crucial to know which version of Java your system is currently using. To learn this information, enter the following command in the terminal: `java -version`. The output will show you the Java version present on your system.
– **Recompile Your Class in Target Environment**: When porting code between environments running different Java versions, it is generally advisable to indicate the lower target JVM version during compilation. You can define the `-target` option in the `javac` command as such: `javac -target 1.8 MyNewClass.java`. Note that the given ‘1.8’ here is only an example. This should correspond to the JVM version of your runtime environment.
– **Upgrade Your Java Runtime Environment (JRE)**: This is the last resort if you have no access to the source code, or you can’t recompile it due to some restrictions. You can upgrade the existing JRE or JVM installation to the required version.
As programmer Gary Bernhardt said, “Make it work, make it right, make it fast”. Debugging errors such as the Java UnsupportedClassVersionError require patience, careful exploration and even revisiting the basics of how Java compiles and executes code.
In reference to the online resources that will help explore Java UnsupportedClassVersionError more can be found here.
Understanding Java UnsupportedClassVersionError: Causes and Implications
Undoubtedly, encountering a
Java UnsupportedClassVersionError
can be frustrating. This error typically signifies that a higher Java Development Kit (JDK) version was used to compile the bytecode than the Java Runtime Environment (JRE) that is currently attempting to run it. For example, if you compiled your program on a system with JDK 11 installed, it would be incompatible with a JRE 8 environment.
To dive deeper, the major causes of this error are:
- Outdated JDK: If the bytecode is intended for a newer JDK and the present runtime environment is an older one.
- Multiple JDKs: You might be having more than one JDK version on your machine, and inadvertently using different versions for compiling and executing.
When analyzing the repercussions of the
UnsupportedClassVersionError
, they primarily revolve around application functionality disruptions. The inability to execute bytecode hampers the working of the application causing development, testing, or even production outages if unseen in earlier stages.
Now, let’s uncover strategies on how to fix
Java UnsupportedClassVersionError
.
- Check your JDK and JRE versions: By running
java -version
and
javac -version
on your command line, you can quickly verify what versions of the JRE and JDK you’re currently using. Make sure they align, particularly if using a multi-JDK set-up.
- Compile to a lower version: If feasible, change your project settings to compile your code into a lower JDK version by setting target bytecode version that matches your runtime environment.
- Upgrade your JRE: Conversely, you could also adjust your JRE to match the JDK version with which the bytecode was compiled for.
According to Dennis Ritchie, “The only way to learn a new programming language is by writing programs in it.” This holds true when troubleshooting errors as well. Navigating through them not only improves our understanding but also fine hones problem-solving skills.
To further familiarize yourself, here’s an example considering scenario with two JDK versions (8 & 11):
Updated alternatives for multiple JDK: sudo update-alternatives --config java Compilation with specific javac from JDK 8: /usr/lib/jvm/java-8-openjdk-amd64/bin/javac SampleApp.java Execution using JRE from JDK 11: /usr/lib/jvm/java-11-openjdk-amd64/bin/java SampleApp
In these commands, we switch between the versions, check, and ensure that the right version is used for the right task to avoid the
UnsupportedClassVersionError
. This kind of error handling further solidifies the fact that “problems are nothing but wake-up calls for creativity” in words of Gerhard Gschwandtner.
For more detailed information, turn to official Oracle Java Documentation.
Implementing Solutions for Java UnsupportedClassVersionError
The Java
UnsupportedClassVersionError
typically manifests when you’re running an application with a Java version that is below the minimum-required version. This error can often occur when your development environment is using a different Java Runtime Environment (JRE) from the one on your deployment system.
An example of such an error message would be
java.lang.UnsupportedClassVersionError: com/test/MyClass : Unsupported major.minor version 52.0
This example implies that your MyClass was compiled with Java 8 (version 52.0), but an earlier version of Java is being used to run it.
To resolve this issue, it’s imperative that you align the Java version across all platforms where the application is developed, tested, and deployed.
Step 1: Verify Java Version
Firstly, establish what version of Java each environment is using by executing the command:
java -version
You will see output similar to this:
java version "1.8.0_271" Java(TM) SE Runtime Environment (build 1.8.0_271-b09) Java HotSpot(TM) 64-Bit Server VM (build 25.271-b09, mixed mode)
Here, the version is 1.8 which corresponds to Java 8.
Step 2: Re-compile with Lower JDK Version
Assuming you have control over the development environment, but not the production environment, the straight-forward solution would be to compile your code with the same or lower version of Java as compared to the runtime environment.
Using the target keyword for the javac compiler, your compile command should look like:
javac -target 1.7 MyProgram.java
In the above case, you are specifically compiling to run on Java 7.
Step 3: Update the JRE Version
Alternatively, if you have access to update the JRE in the deployment environment, consider downloading and installing the appropriate version to match the development environment.
Oracle’s official website is a reliable place to download the required Java version.
Upgrading the JDK & Managing Multiple Java Versions
There may be scenarios where you need to manage multiple versions of Java. For instance, several projects could require different versions. A version management tool like SDKMAN! might prove useful. It helps you switch between different Java versions smoothly and effectively.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
When a common language version is established across all systems, it eliminates misunderstandings. In programming too, it ensures that all involved machines can understand the commands given, enabling smooth operation of the Java application without running into an
UnsupportedClassVersionError
.
The Role of JVM and JDK in Fixing UnsupportedClassVersionError
The Java Virtual Machine (JVM) and the Java Development Kit (JDK) play instrumental roles in addressing an `UnsupportedClassVersionError`. However, to fully comprehend their function in resolving such an error, we must first understand what leads to this error.
UnsupportedClassVersionError
is an issue that arises when attempting to load a class compiled with a version of Java that is superior to your JVM’s version. For instance, if a class was compiled using JDK 10, but your runtime environment utilizes JVM for Java 8, an `UnsupportedClassVersionError` will ensue.
The JVM, essentially, interprets the bytecode and executes it. Each version of the JVM understands the bytecode generated by the same version of the JDK. If a bytecode produced by a higher version of the JDK is presented, the older JVM won’t understand it, resulting in an `UnsupportedClassVersionError`.
This knowledge paves the way towards feasible solutions. It’s pertinent to note two distinct but commonly implemented approaches:
1. Updating your JVM: Firstly, consider aligning your JVM version to the JDK version used to compile the `.class` file. This could involve updating your current JVM or downloading a newer release from Oracle’s official website.
2. Recompiling the Java Class: Alternatively, source code files (`*.java`) can be recompiled using a lower version of the JDK, matching your runtime environment. To achieve this, you need to set your system’s `JAVA_HOME` to point to the desired JDK version before recompiling your code. The resulting bytecode will be compatible with your current JVM.
Regarding the JDK’s role, it provides `javac`, the tool that translates Java source files into platform-independent bytecode. Each new release of the JDK introduces support for more advanced features, some of which are not supported by previous versions. Therefore, compatibility between the JDK used for compilation and the JVM used for execution is vital.
A relevant quote in context here comes from James Gosling, the father of Java himself: “Java is an attempt to update the computer science concepts in one safe, high-performance language.” Recognizing these mechanisms within the JVM and JDK is indeed part of grasping Java’s ‘updated’ safety and performance measures.
In essence, the role of JVM and JDK in addressing an `UnsupportedClassVersionError` revolves around ensuring compatibility. The versions of JDK (used for compiling) and JVM (used for the running application) must align harmoniously. Naturally, their related tools then aid in either updating the JVM or recompiling source files with an appropriately matched version of the JDK. Utilizing these methods strategically serves as a tangible response towards fixing `UnsupportedClassVersionError`.
Case Study: Real-time Scenarios of Resolving the Java Unsupportedclassversionerror
When it comes to Java development, encountering errors can be a common theme. One such error is the
UnsupportedClassVersionError
. This error typically occurs when you are trying to run a class that was compiled using a more recent version of the Java Development Kit (JDK), on an older version of Java Runtime Environment (JRE).
Studying real-time scenarios can provide invaluable insight into resolving this issue. Let’s delve into the steps for troubleshooting and rectifying the Java
UnsupportedClassVersionError
.
Held in High Regard: Identifying the Error
The first crucial step towards fixing any problem is accurately identifying its existence. Whenever the JVM attempts to read a class file, it verifies the major and minor version numbers in the file. If these numbers indicate that the class was compiled with a later version of Java than the JVM, it throws an
UnsupportedClassVersionError
. The error description might look something like:
java.lang.UnsupportedClassVersionError: HelloWorld : Unsupported major.minor version ...
.
Tabulating the version compatibility makes easy to track down the source of this error:
Java SE | JDK version | major.minor version |
---|---|---|
12.0 | 12 | 56.0 |
11.0 | 11 | 55.0 |
10.0 | 10 | 54.0 |
Tidying Up: Aligning JDK and JRE Versions
To fix the
UnsupportedClassVersionError
, one simple strategy would be to ensure that your compiler (JDK) and runtime environment (JRE) versions align. When compiling your code and running your compiled classes, you should do so on the same version of Java or use a newer version of JRE than JDK for execution.
Here is how :
If you have multiple Java versions installed, you can specify which one to use by setting the
JAVA_HOME
environment variable. For instance, prior to compiling or running your java application, you can set the path to the JRE as follows:
On Windows:
set JAVA_HOME=path to your JRE path %JAVA_HOME%\bin;%path%
On Linux/Mac:
export JAVA_HOME=path to your JRE export PATH=$JAVA_HOME/bin:$PATH
In Full Swing: Recompiling Source Code
Another method includes involving recompiled code using an older version of JDK to match our JRE. Run the
javac
command line tool with the
-target
option to generate class files compatible with earlier JVM versions.
For example:
javac -target 1.8 HelloWorld.java
These are some strategies every Java developer can apply in a real time scenario to resolve the Java
UnsupportedClassVersionError
. As renowned coder Phil Karlton once said, “There are only two hard things in computer science: cache invalidation and naming things”, but fortunately, fixing the
UnsupportedClassVersionError
isn’t one of them!
To address the issue of Java UnsupportedClassVersionError, it is imperative to understand what this error means and its root cause. An UnsupportedClassVersionError in Java typically occurs when a higher JDK has been used to compile the .class file which a lower JVM is interpreting.
Specifically, Key reasons for such errors include the following:
– Incorrect Environment Variable Settings: When the JAVA_HOME variable points to an older version of Java, the error may occur.
– Version Mismatch between JDK and JRE: If your Java Development Kit (JDK) and Java Runtime Environment (JRE) are not synchronised with each other, or if they are from different versions, these issues can commonly appear.
To fix this UnsupportedClassVersionError, systematic approaches should be adapted:
1. Consistency in using JDK and JRE Versions: One must ensure that both JDK and JRE belong to the same version. This can control the compilation and execution processes effectively, reducing the chance of version conflict.
2. Proper Set-Up of Environment Variables: Adjust JAVA_HOME environment variable so that it points correctly towards the updated Java version.
Lastly, it’s beneficial to leverage integrated development environments (IDEs) that automatically manage these configurations and handling version compatibility issues effectively. IDEs like IntelliJ IDEA or Eclipse ensure the seamless production environment set-up, sparing developers from the manual error-fixing hassle.
An effective route to dodging the Java UnsupportedClassVersionError is dispatching regular updates on your build paths and configurations, therefore, tackling potential version discrepancies before they could trigger any errors.
“Coding is simply about taking conscious actions today to avoid tomorrow’s issues.” – A sound wisdom to adhere by in the realm of Software Engineering.