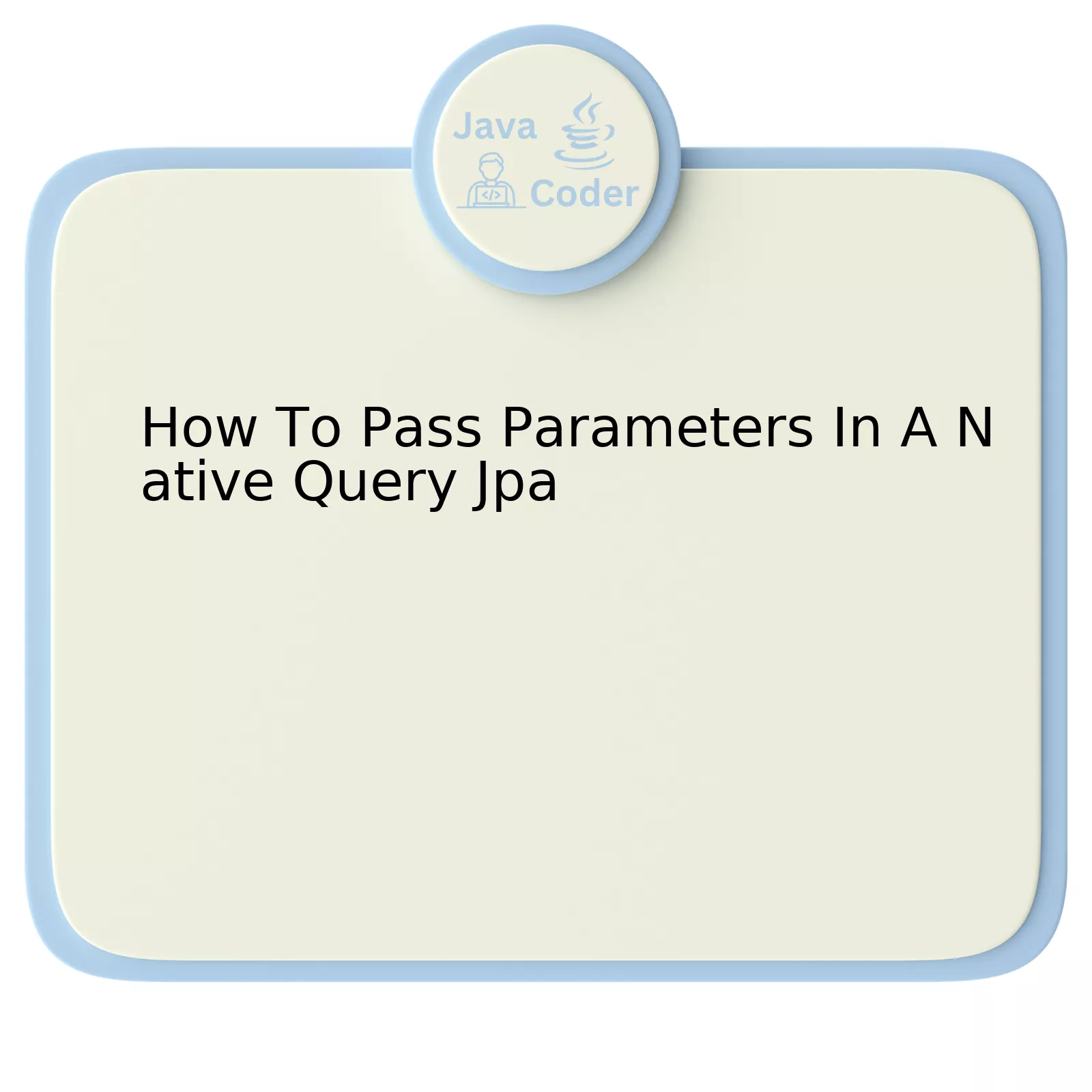
Passing parameters in Native Query JPA is a common requirement in many programming scenarios. An optimal method of passing these parameters is by using the setParameter method provided by the Query interface.
Consider that we have a “Book” class, and we need to fetch a book’s details by its “id”.
String sql = "SELECT * FROM Book WHERE id = :id"; Query query = entityManager.createNativeQuery(sql, Book.class); query.setParameter("id", 1);
The createNativeQuery function generates an SQL query, indicating that we are working with a ‘Book’ entity. We then use the setParameter method, where the first argument is the parameter name, and the second one is the parameter value.
This coding approach allows us to build dynamic and efficient queries that pass parameters safely and effectively. Setting parameters in this manner, rather than manually building the query string, helps in preventing SQL injection attacks.
Below is a table representation of the process:
Method | Action |
---|---|
createNativeQuery |
Generates SQL query, specifying we work with the ‘Book’ entity. |
setParameter |
Sets the id parameter for the query. In our case, the ‘id’ is 1. |
As Bill Joy, co-founder of Sun Microsystems said, “Basically, I’m not interested in doing research and I never have been. I’m interested in understanding, which is quite a different thing. And often to understand something you have to work it out yourself because no one else has done it.” This highlights the importance of truly grasping new concepts within the practicality of code.
Seamlessly blending the comprehension of setParameter methods when using JPA can greatly influence the effectiveness of your code, making database communications streamlined, safe, and dynamically adept to change.
Understanding JPA Native Queries and Parameter Passing
Java Persistence API (JPA) supports the concept of Native SQL, granting developers the ability to write SQL queries that are directly executed in the database. This can be quite useful when complex data operation cases arise, exceeding the JPQL or Criteria API’s capabilities.
To interact with the database using Native queries, you use the
createNativeQuery
method on the
EntityManager
interface or its subclass.
So, how do we pass parameters in a native query with JPA? There exist two methods for parameter passing in JPA native queries:
1. Positional Parameters:
Using positional parameters, input values are passed into the SQL statement based on their position. In JPA, this is accomplished by the use of question mark
?
as
placeholders followed by an integer designating position. For instance:
String sql = "SELECT * FROM users WHERE name = ?1"; Query q = entityManager.createNativeQuery(sql, User.class); q.setParameter(1, "Alex");
In the given example, ‘?1’ is the placeholder for the first parameter which stores the name. We then set the value for this parameter using the
setParameter()
method.
2. Named Parameters:
Named parameters provide another way of passing arguments. It could make your code more readable and self-explanatory. They are similar to placeholders, but they use specific names instead of a question mark. View the example below:
String sql = "SELECT * FROM users WHERE name = :name"; Query q = entityManager.createNativeQuery(sql, User.class); q.setParameter("name", "Alex");
In this code snippet, ‘:name’ is the placeholder for the parameter which stores the name. Then we assign the actual value using the same
setParameter()
method.
While handling JPA native queries, developers should be cautious about possible SQL injection attacks. Always remember to sanitize user inputs before including them in your SQL strings.
As Donald Knuth, a renowned computer scientist once said, “Premature optimization is the root of all evil.” Avoid over-optimizing your native queries; keep them simple and efficient. For online references consider checking the Oracle Java documentation on the use of PreparedStatements, which provide similar features in pure JDBC.
Applying Positional Parameters in JPA Native Queries
JPA, or the Java Persistence API, provides a robust platform for translating between object-oriented domains and relational databases. When it comes to executing native SQL queries using JPA, parameters can certainly be leveraged to make the processes more efficient and secure.
Positional Parameters in JPA Native Queries
While developing applications using JPA, quite often, developers prefer to utilize named parameters in their queries. However, positional parameters instead of named parameters can be employed in some situations.
Implementing positional parameters within JPA Native Queries involves signifying the parameters with “?” symbol followed by a number indicating the position of the parameter in relation to others; it starts from 1. It’s worth mentioning that the positioning counts only those placed in the WHERE clause. To exemplify, take a look at this hypothetical SQL query below:
em.createNativeQuery("SELECT * FROM Employee e WHERE e.name = ?1 AND e.age = ?2");
In the above code snippet, “?1” and “?2” are positional parameters. The first represents the name, and the second refers to the age in the database query.
No Room For Duplicated Positions in Positional Parameters
One point to note is that unlike named parameters, duplicated positions are not permissible in positional parameters. This restriction demands special attention during coding to avoid redundancy in specifying positions.
Passing Positional Parameters
Passing positional parameters to a native JPA query enables one to feed information into the query dynamically and safely, protecting against SQL injection attacks. Here’s the way parameters are set in the query using EntityManager’s setParameter method:
Query query = em.createNativeQuery("SELECT * FROM Employee e WHERE e.name = ?1 AND e.age = ?2"); query.setParameter(1, "John Doe"); query.setParameter(2, 25);
The setParameter method allows you to change the placeholders (?1, ?2) with actual values (“John Doe”, 25). It utilizes two arguments: the first stands for the placeholder number in the query, and the second presents the value to replace the placeholder.
While discussing the prospect of passing parameters in programming paradigms, Edsger W. Dijkstra once said, “The use of COBOL cripples the mind; its teaching should, therefore, be regarded as a criminal offense.”. Similarly, when misused, improper handling of parameters could result in SQL injections, crippling the safety of your application
Always remember, when utilizing JPA Native Queries, appropriately managing positional parameters can help enhance the security and efficiency of your data access layer.
For more insight on JPA positional parameters, delve deeper with this reference.
Using Named Parameters Effectively in JPA Native Query
Passing parameters in a JavaScript Persistence API (JPA) native query can be achieved using two different methods: positional parameters and named parameters. While both have their advantages, using named parameters efficiently can considerably improve the readability and maintainability of your queries. Below you will find an in-depth comparison and useful tips on how to employ these methods effectively in your JPQL or SQL code.
Positional Parameters Vs Named Parameters
Positional parameters, specified as ? followed by the parameter index starting from 1 (
?1, ?2, etc.
), are simple to use but they can make complex queries hard to read and manage. Refactoring queries or adding new parameters can easily lead to errors if all indices aren’t updated correctly.
Contrastingly, named parameters, defined as : followed by a unique parameter name (
:name, :age, etc.
), enhance the legibility of your queries. They give additional context about what each value represents, making it easier to understand the purpose of each parameter. This is especially useful in larger team environments where the codebase is shared among various developers.
Using Named Parameters Effectively
To deploy named parameters productively in your JPA native queries ensure the following:
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler.
– Names should be self-explanatory: Ensure that names honestly and clearly represent the values they hold. For example, for a user’s age, prefer
:userAge
instead of something generic like
:param1
.
– Don’t repeat parameter names: Each named parameter within a single query should be unique.
– Use them consistently: If you decide on using named parameters, it’s crucial to utilize them uniformly throughout your project for constancy.
Here’s an example of how to set named parameters within a native query using EntityManager:
String sql = "SELECT u FROM User u WHERE u.name = :name and u.age = :age"; Query query = em.createNativeQuery(sql, User.class); query.setParameter("name", "John Doe"); query.setParameter("age", 35); Listusers = query.getResultList();
The setQueryParameter methods binds an argument to a named parameter.
Advantages of Using Named Parameters
Named parameters bring a multitude of benefits that enable you to increase the performance and cleanliness of your code:
– Better Readability and Maintainability: As previously discussed, named parameters provide context and meaning to the query statement thereby improving understandability for those who are debugging or maintaining the code.
– Simplified Query Tuning: Addition or removal of parameters becomes easier, mitigating the issues of indexing expenses as witnessed with positional parameters.
– Decreased Error Potential: Misalignment of binding parameters is less likely since specific keywords relay their exact intent.
Mastering and effectively implementing named parameters in JPA Native Queries can elevate the quality of your coding practices, enhancing efficiency in communication among your teammates, and bettering the debugging process.
Expert Tips for Debugging and Troubleshooting Parameter Issues in JPA
Delving into the dynamics of Java Persistence API (JPA), a pivotal subset of it is managing and handling parameters in a native query. This segment includes imparting tips to debug and troubleshoot any potential parameter-related issues that may arise when using JPA.
Tips for Debugging and Troubleshooting Parameter Issues:
1. Parameter Syntax:
While setting up parameters, ensure they adhere to JPA’s paramount syntax rules. For instance, if a parameter is erroneously defined or absent, an ‘IllegalArgumentException’ would surface. Therefore, always validate your parameter’s syntax for positional or named parameters. For instance, to set a parameter in a native query:
Query q = em.createNativeQuery("SELECT m FROM MyTable m WHERE m.age > ?1"); q.setParameter(1, 18);
In this example, “?1” signifies a positional parameter.
2. Data Type Mismatch:
Another common yet subtle error is the mismatch between column data type and the parameter data type. An ‘IllegalArgumentException’ gets thrown if the data types do not align, leading to an inaccurate parameter value. Hence, to prevent debugging difficulties later on, scrutinize your column and data type consistency.
3. Ensure Transaction Boundaries:
A transaction should embolden every JPA modification, including setting parameters. You must call begin() prior to modifying the database and end transaction with either commit() or rollback(). Sporadic attempt of working outside the boundaries of a transaction often triggers unanticipated behavior and trouble in debugging.
4. Managing Null Values:
When dealing with queries, always make a provision for null values. When left unmanaged, unassuming null value could lead to `NullPointerException` errors. Always ensure that appropriate null checks are in place before executing the query.
5. Error Handling:
Implement effective exception handling to make debugging easier. JPA provides different types of exceptions like `NoResultException`, `NonUniqueResultException`, which can guide you to pinpoint issues. Use try-catch blocks effectively to handle these exceptions.
In debugging, one should heed what former IBM programmer Wietse Venema once said, “Debugging is twice as hard as writing the code in the first place. So if you’re as clever as you can be when you write it, how will you ever debug it?”
6. Familiarize with Logging and Profiling:
Profiling tools can prove to be invaluable resources when troubleshooting quirky bugs. Considering employing Hibernate’s extensive logging facilities or JPA’s vendor-independent properties, which aid in inspecting SQL statements behind-the-scenes and identifying if parameters are rightly placed or not. It also allows you to track performance metrics and detect potential bottlenecks in your application.
Lastly, practice is crucial. Native queries offer more control but equally demand an understanding of SQL. Experiment with different SQL commands to understand their nuance and effect, be attuned to potential exceptions, and optimally structure your queries to promote maximum results.
Reverberating Thomas C. Gale’s thoughts, “Perfection is not attainable, but if we chase perfection we can catch excellence.” As a developer, constantly refining our understanding of parameters within JPA native queries aids not just in producing efficient code but also lowers time spent debugging issues.
Let’s delve into the intricate plot of passing parameters in a native query JPA. Thoughtful usage of parameters in native queries can enhance not only the readability and maintainability of your code but also its security against SQL injection attacks.
Anatomy of Passing Parameters in a Native Query JPA
In traditional SQL, we transfer data to our queries through explicit string concatenation. This becomes a breeding ground for SQL injection attacks as it treats all inputs as direct parts of the query.
If we replace this with Java Persistence API(JPA)’s native queries, we gain better secure, maintainable, and readable code.
For instance, consider this typical example:
Query q = em.createNativeQuery("SELECT m FROM MyTable m WHERE m.column = :value"); q.setParameter("value", "myValue"); Listresults = q.getResultList();
This code introduces us to two important actions:
• Using a ‘:’ rather than ‘?’ for parameter specification
• Implementing ‘setParameter’ function to provide an actual value for the mentioned parameter
Benefits Galore- Why Pass Parameters in Jpa?
The benefits involve:
Safety First:
The prevention of SQL injection attacks attributes to the foremost benefit. Parameterized queries treat input values as literal and independent from the query, thereby denying any chance of malicious modification in the query.
Clean Code:
Instead of lengthy string concatenations, you have a more streamlined, comprehensible code leading to improving debug and maintenance.
As Grady Booch, an American software engineer, once said, “Clean code reads like a well-written prose.”
Reusability:
Parameters within native queries also usher in higher reusability. You can reuse the same query for different sets of parameters countless times, thereby increasing your code efficiency.
Ensuring efficient and safe handling of data should remain an uncompromised priority for any developer. By harnessing the power of passing parameters in Native Query JPA, you are securing your applications and reducing code complexity. Remember, safety and simplicity in coding will always keep you one level ahead.