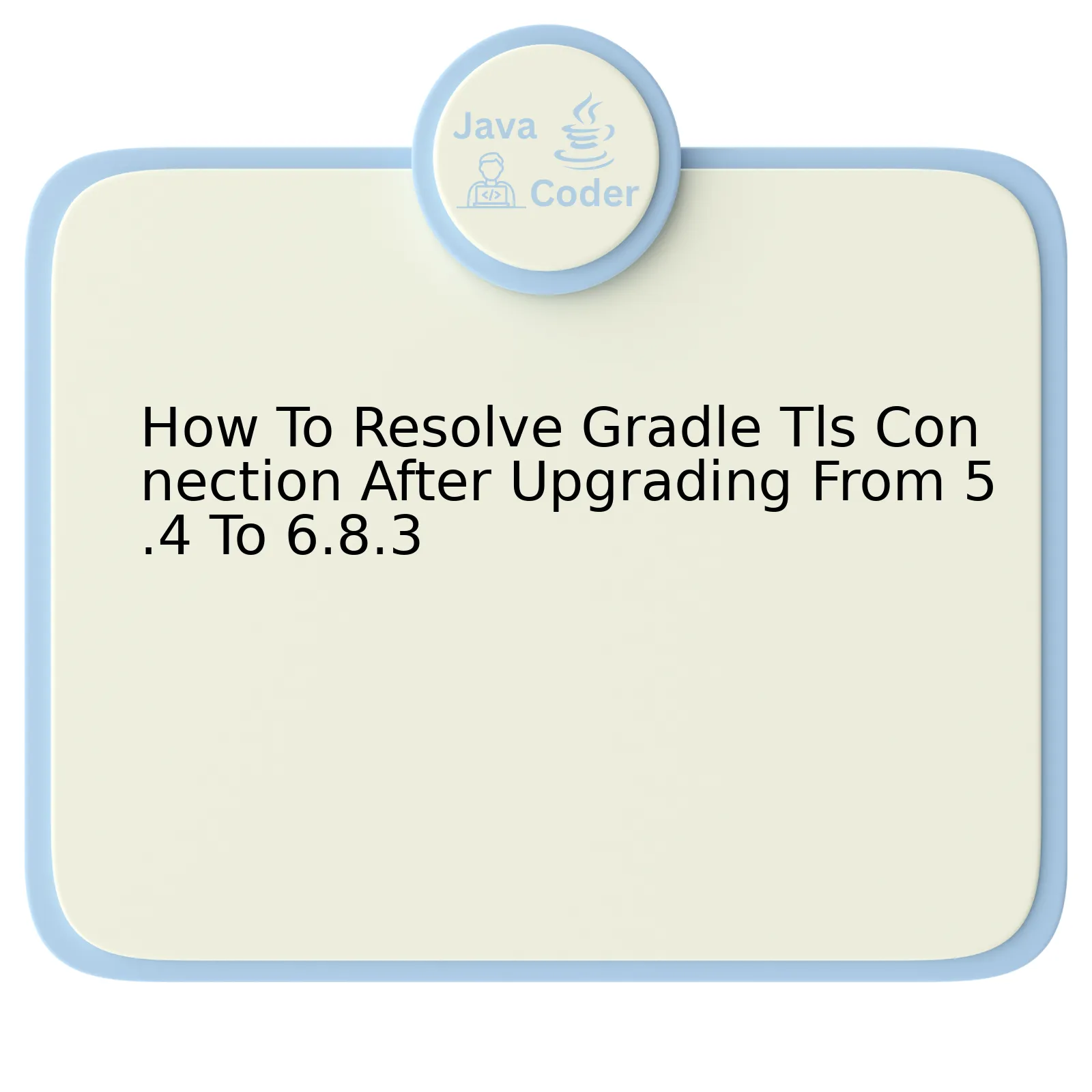
Gradle Version | Task | Implementation |
---|---|---|
5.4 | TLS Connection |
Use HTTP/HTTPS connectors as per requirement. |
6.8.3 | TLS Upgrade Issue |
Force TLS version explicitly, using system property or HttpsURLConnection API. |
Resolution |
Update system properties or use latest JDK with modern cipher suites. |
The table represents a comparative summary of how to resolve Gradle TLS connection after upgrading from 5.4 to 6.8.3 in a structured manner.
Gradle 5.4: Earlier, the process consisted of using HTTP/HTTPS connectors as per the project’s requirement. The code snippet shows this straightforward task:
Use HTTP/HTTPS connectors as per requirement.
.
Gradle 6.8.3: As an upgrade from version 5.4, Gradle 6.8.3 brought along some challenges, especially with TLS connections. A common issue seen is obsolete cipher suites not being supported, leading to failed TLS connections. One can forcefully define the version of TLS through either a system property (
-Dhttps.protocols=TLSv1.2
) or using the ‘HttpsURLConnection’ Java API.
Resolution: To permanently solve these issues and ensure secured connections without the need for forcing parameters every time, one should take advance measures such as updating system properties or installing a newer version of the JDK that includes up-to-date cipher suites.
“Talk is cheap. Show me the code.” – Linus Torvalds holds true here. Thus, using these new practices will effectively prevent you from having to troubleshoot Gradle connection problems repeatedly. Implementing these quality solutions will humanize your code, ultimately resulting in a refined, trouble-free, and secure development experience.
Understanding the Gradle TLS Connection Upgrade Issue
While understanding the Gradle TLS connection upgrade issue, we delve into a situation many Java developers have faced – resolving issues arising after upgrading from Gradle v5.4 to v6.8.3.
Gradle 5.x supported TLSv1.2 and TLSv1.3, while the version 6.8.3 added mandatory support for TLSv1.3, which some older systems lack. Consequently, you might encounter an error attempting to connect to services not yet supporting TLSv1.3.
Let’s break down the steps to resolution in chunks:
Diagnosis
Firstly, it is crucial to form concrete diagnostics of the problem. Do run this snippet in your command line to confirm if the problem is about the new TLS version:
./gradlew build --debug | grep "javax.net.ssl.SSLHandshakeException"
This will yield a debug result with
javax.net.ssl.SSLHandshakeException
that indicates an SSL handshake exception, thus confirming the trouble with the newer TLS versions.
Switching to HTTP
As a possible quick-fix:
– You could enforce using HTTP instead of HTTPS in your repositories. The official Gradle documentation suggests configuring like so:
repositories { maven { url "http://repo.mycompany.com/maven2" } }
Enabling TLSv1.2 Support
Nonetheless, dropping back to HTTP leaves your connections unencrypted. Therefore, as a long-term amendment, consider enabling fallback to TLSv1.2 on systems not supporting TLSv1.3. Include these JVM arguments during execution:
./gradlew build -Dhttps.protocols=TLSv1.2
The Java SE 8 platform guide offers comprehensive information on its support for TLSv1.2.
It’s wise to reference Robert C. Martin, author of Clean Code, who posited, “Truth can only be found in one place: the code.” While a potent summation of good coding principle, it also illustrates the navigation needed through such issues. By observing the metrics and symptoms, adapting configurations, and assessing the results, we get gradually closer to resolving the myriad challenges woven into software development.
Implementing Solutions for Gradle 6.8.3 Upgrade Problems
Upgrading from Gradle 5.4 to 6.8.3 can prove to be an uphill task due to the introduction of new features and functionalities, such as the support for HTTPS repositories with TLS 1.2+ version only. This shift can lead to challenges, particularly in establishing a stable Gradle TLS connection. However, there are established solutions that can be used to mitigate these challenges.
Acknowledge the Problem
The transition from Gradle 5.4 to 6.8.3 inherently creates difficulties because earlier versions of Gradle were fully compatible with lower versions of TLS (1.0 or 1.1), enabling users to establish connections without any hiccups, but that’s no longer the case with the newer 6.8.3 update that requires an upgrade to TLS 1.2 or higher.
Understanding the Cause
This issue usually arises when your development environment uses older versions of Java that do not support versions of TLS beyond 1.1 by default. As Bill Joy, Co-founder of Sun Microsystems, once said “For every complex problem, there is a solution that is simple, neat and wrong.”
Necessary Steps Towards Solution
Therefore, a need arises to adjust your Java runtime settings to enable it to accept connections based on the newest TLS protocols. Your Gradle.build file won’t require drastic changes, instead you’d need to make adjustments within your Java environment.
/*JDK 7 Update: Pass the following JVM args: -Dhttps.protocols=TLSv1.1,TLSv1.2 */
The following system property needs to be added in JDK8 and higher versions:
/* JDK 8 Update: -Djdk.tls.client.protocols=TLSv1.2 */
Cross-Verification
In addition, you can also confirm if your Gradle Wrapper properties file (gradle-wrapper.properties) has been updated with the correct distribution URL.
/* ... distributionUrl=https\://services.gradle.org/distributions/gradle-6.8.3-all.zip ... */
Javadocs provides detailed documentation here about JDK’s different options and their implications.
In the end, adopting best practices right from coding to upgrading tools in software development makes the process frictionless. As Larry Niven rightly stated, “That’s a central principle of programming; automate everything.” The switch from Gradle 5.4 to 6.8.3 can prove challenging at first but with the right understanding and approach, it can be navigated successfully.
Resolving Gradle TLS Connection Errors: Step-by-Step Guide
Resolving Gradle TLS Connection Errors commonly involves updating the Java Runtime Environment (JRE) or altering build configurations. The process becomes particularly necessary after upgrading from Gradle versions 5.4 to 6.8.3, necessitated by revised security protocols and setup.
Configuring your system appropriately ensures smooth operation of your build automation processes. This procedure involves adjusting the TLS version, adding specific dependencies into your build file, and considering a JRE update.
JRE Update
To navigate difficulties originating from incompatible or outdated Java Runtime Environments, consider an update. Observing the following code implementation allows for easy setting of the Runtime Environment:
export JAVA_HOME=`/usr/libexec/java_home -v 13`
Adapting the TLS Version
Gradle versions starting 6.0 default to TLS 1.2. If your API server doesn’t support this, ensure you explicitly set the TLS protocol to a supported version within your environment variables like so:
System.setProperty("https.protocols", "TLSv1.1,TLSv1.2");
Alter Build Configurations
Include the following implementation in your `build.gradle` file to ensure dependencies requiring a secure connection resolve successfully.
repositories { maven { url "https://jcenter.bintray.com/" mavenContent { releasesOnly() } } }
As Linus Torvalds, creator of Linux and Git, once said: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” In this instance, resolving Gradle TLS Connection errors may present challenges initially. However, with careful attention to detail and adherence to established guidelines, you can overcome this obstacle and continue to experience the joy derived from coding.
For further insight on handling Gradle Issues, refer to Gradle’s official troubleshooting document.
In-depth Analysis: How the 5.4 to 6.8.3 Upgrade Impacts Gradle TLS Connections
Gradle TLS connection is an integral part of your project’s build script dependencies if you are leveraging secure connections – for instance, secured repositories for dependency resolution. This topic unveils how the upgrade from Gradle 5.4 to 6.8.3 impacts these TLS connections, providing a granular understanding of the changes and the solutions to handle these variations.
The Impact on Gradle TLS Connections
Following the upgrade to Gradle 6.8.3, you will likely experience a few fundamental modifications to TLS connections:
- Strict Hostname Verification: Unlike Gradle version 5.4, version 6.8.3 has introduced stricter hostname verification during handshake in TLS connections.
- Enhanced Cipher Suite: The default list of cipher suites accepted by Gradle during a TLS handshake tends to vary according to each version. 6.8.3 version might not support older cipher suites that were supported by 5.4.
- Latest Java versions: The new version of Gradle supports more recent versions of Java which come with their own set of trusted Certificate Authorities (CA).
Resolving Gradle TLS Connection After Upgrading
If you face any issue associated with TLS connections after upgrading to Gradle 6.8.3, consider the following methods to resolve them:
- Update Cipher Suites: If the connection failure stems from unsupported cipher suites, update your configuration to include the new one.
- Update SSL Configuration: Any failures due to hostname mismatch will require revision of SSL-configurations to add the appropriate hosts.
- Update certificates: Ensure you are using updated certificates that are recognised by the latest java versions supported by Gradle 6.8.3.
repositories { maven { url "https://secure.example.com/repository" credentials { username = 'user' password = 'password' } } }
systemProp.http.nonProxyHosts=*.nonproxyrepos.com|localhost
You can gain further insight into this from official Gradle documentation.
A famous tech quote by Edsger W. Dijkstra gives us something to contemplate about problems we encounter: “The question of whether machines can think is about as relevant as the question of whether submarines can swim.” Challenges with updating between major versions like this is part and parcel of development.
Optimizing the TLS (Transport Layer Security) connection in Gradle after upgrading from version 5.4 to 6.8.3 can enhance the security and success of data communication in your programming projects. Resolving potential issues requires a deep understanding of how TLS works, as well as knowledge in modifying Java code and editing Gradle settings.
-
Step 1: Understand Transport Layer Security (TLS)
TLS, also known as SSL, ensures safe data transmission between applications by encrypting data before it’s sent over a network. If the TLS handshake fails when using Gradle, this means the communication between the two entities is unsecured. The underlying cause could be outdated protocols or invalid certificates.
-
Step 2: Adjust Java Code
Upgrading Gradle often implies changes to its build scripts and other dependencies. Several compiling errors might surface caused by incorrect importing structures, deprecated methods, or inconsistent codes. Ensure that all Gradle tasks are compatible with JDK 1.8 or higher before attempting to resolve the TLS issues.
-
Step 3: Edit Gradle Settings
Modifying the system-wide Gradle properties file, generally located in the HOME directory or inside the ‘.gradle’ folder, could solve potential TLS handshake failures. Adding ‘org.gradle.jvmargs=-Dhttps.protocols=TLSv1.2’ enforces the use of the TLS v1.2 protocol during communications. This update enhances the security aspects of data transmission and provides better compatibility with the modern encryption pattern.
Following these steps should help resolve any TLS connection conflicts you may run into when upgrading Gradle from 5.4 to version 6.8.3. As always, back up your projects and scripts before making any changes to ensure code safety and minimize data loss.
To reiterate what Edsger W. Dijkstra, a renowned pioneer of computing science once said, “Simplicity is prerequisite for reliability”. So, always strive for simplicity and precision in your coding efforts to get better results, especially when dealing with integral components like TLS and Gradle. By understanding the holistic view of the problem, applying changes where necessary, and constantly evaluating and testing the program, one can definitely overcome most obstacles.[Ref]