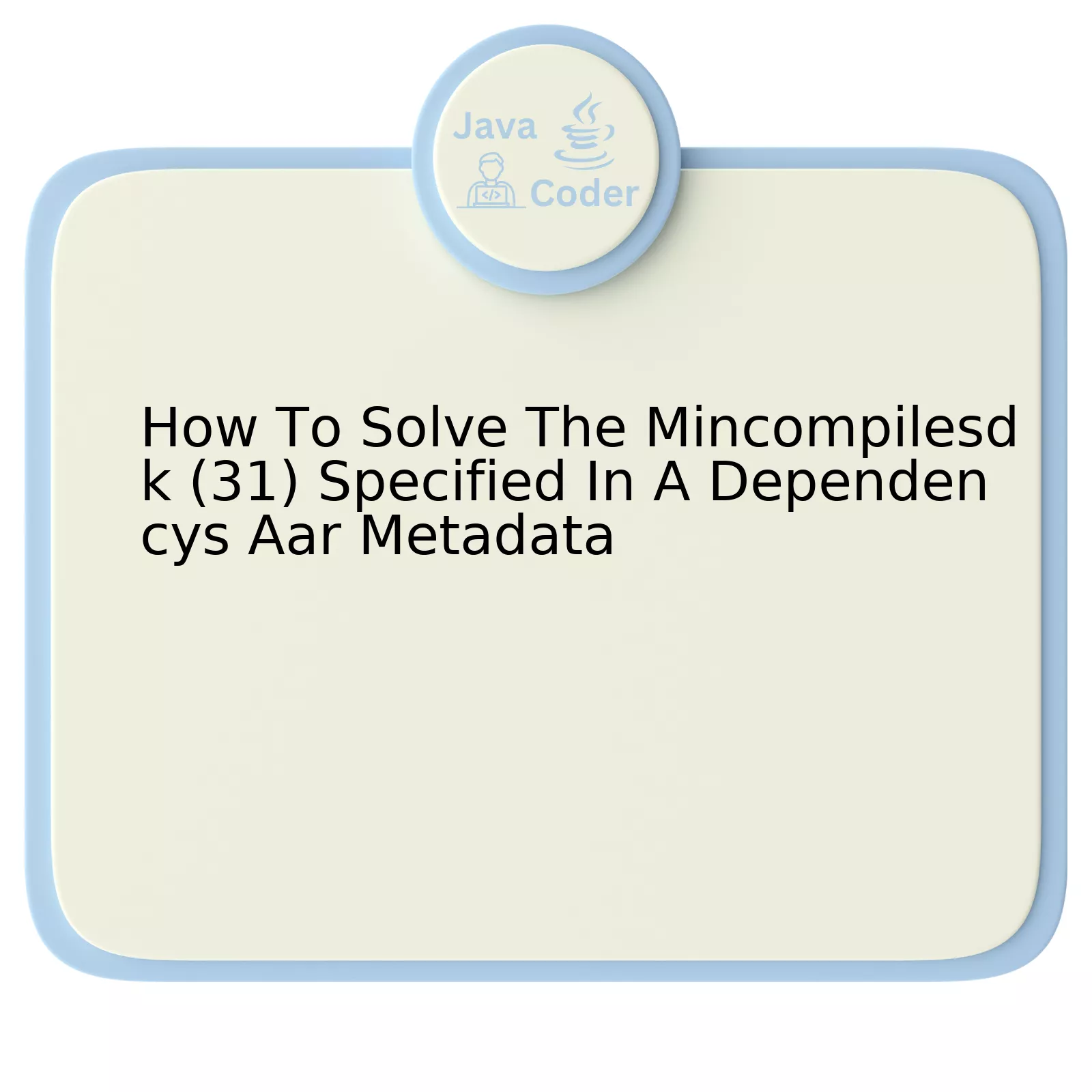
In working with Android development, the MinCompileSdk(31) problem as specified in a dependency’s AAR metadata can sometimes arise. It is essential to understand that this issue originates from an attempt to compile your application with a minimum SDK lower than 31, while a library you are using requires a higher level. To amend this, there are largely three approaches:
Step | Action |
---|---|
Android Gradle Plugin Update | Update the version of your Android Gradle plugin. Often, this issue occurs due to incompatible versions between the plugin and the libraries you have included. |
Library Update | Check if there’s an updated version of the library causing the issue. Updated versions often resolve compatibility discrepancies such as these. |
Min SDK Version Increment | Increase your app’s min SDK version to meet the requirement. However, do consider this could exclude users on older versions of Android from using your app. |
Now, let’s break it down even further.
Android Gradle Plugin Update
A common culprit for the MinCompileSdk(31) error is mismatched versions between your Android Gradle plugin and your dependencies. Check your project-level build.gradle file and confirm whether it’s up to date. An upgrade might solve your issue:
classpath 'com.android.tools.build:gradle:7.0.2'
Library Update
The same logic applies to the libraries and modules you depend on. Make sure you’re using the most updated versions of each one, since the issue may have already been resolved in a newer release. Be mindful, though, that updating libraries can bring its own set of challenges if there are significant changes or deprecations.
Min SDK Version Increment
Lastly, the brute force way – increase your minSdkVersion to at least 31 (which is the version Android 12 corresponds to) to satisfy the requirement put forth by the problematic library. To implement this, you’ll need to modify your app-level build.gradle file:
minSdkVersion 31
Take note though, this method means dropping support for devices running on Android 10 and below, so weigh your options carefully before proceeding.
As Bill Gates once wisely stated, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” These solutions aim to ensure that technology blends seamlessly with your development process and helps you surmount any challenges you face.
Understanding the MinCompileSdk (31) error in AAR Metadata
The MinCompileSdk (31) specified in a dependency’s AAR Metadata error often arises when a developer tries to use libraries that are incompatible with their current project’s Android Compile SDK Version. This problem is typically caused when the library’s `minCompileSdk` is higher than the one specified in your app-level `build.gradle`.
Let’s take a peek into some details behind this issue and strategize an effective way to resolve it.
What does MinCompileSdk (31) signify?
The minCompileSdk within the `build.gradle` file of a library project indicates the minimum Compile SDK version that should be used for projects depending on this library. It ensures that certain APIs, which are included in newer versions of Android, are not accidentally referred to when they are not available on all target SDKs.
For instance, if a library author specifies MinCompileSdk(31), they are stating that anyone who wants to consume their library must compile their application with at least Android 12(API level 31).
Steps to Solve the MinCompileSdk Error
Step | Action |
---|---|
I. | Identify the problematic library. Your compiler error message will provide this information. |
II. | Utilize Gradle commands (e.g., ./gradlew :app:dependencies) to identify the unusual library’s version. |
III. | Update your local Android Studio SDK Manager to comport with the most recent released Android SDK version. |
IV. | Alter the compileSdkVersion in your app/module level build.gradle to match or exceed the minCompileSdk mentioned in the library’s AAR metadata. |
Observe this sample change to the build.gradle file code snippet:
android { compileSdkVersion 31 defaultConfig { ... } }
It’s crucial to note, as you increase the compileSdkVersion, new linter warnings may emerge due to Android’s more stringent enforcement rules. These warnings should be fixed since they could lead to application instability.
As Tim Bray, a software developer and one of the authors of the original XML specification, says, “In all but rare corner cases, updating the compileSdkVersion to newly-released apis is good hygiene”.
Make sure to consult the various Android documentation resources available online to keep up-to-date about any changes in different API levels. Updating the compileSDK version promptly will likely prevent similar complications from arising in the future.
Decoding the Problem: Dissecting the MinCompilesdk (31)
The minimum compile SDK version (minCompileSdk) has caused certain difficulties for Java developers when it comes to dealing with a project’s dependencies. The minCompileSdk(31) is specifically the latest stable version which declares the minimum API level that an application can target in its manifest file for compiling. It impacts the compatibility of an app’s AAR (Android Archive) metadata, which holds valuable information concerning the Android Library Project.
One method to resolve this issue lies within the Gradle script, a tool utilized by Java Developers to automate the build process of their projects.
dependencies { implementation("com.your.library:1.0.0") { exclude group: 'com.android.support', module: 'support-v4' } }
In the above example, the
exclude
line ensures that the compiler disregards the specified module defined in the AAR metadata preventing any potential conflicts that may arise.
Furthermore, it is important to uphold best practices for managing your project’s dependencies:
• Track the versions: Keep track of the versions of all libraries being used in your project, making it easier to know when an update is needed.
• Manage transitive dependencies: These are the dependencies of your direct dependencies. If not managed correctly, they can cause version conflicts.
• Use version ranges cautiously: While useful in staying updated, they also have the risk of introducing incompatible updates without your knowledge.
• Update frequently but carefully: Regularly updating your dependencies can ensure that you stay on top of any potential issues and take advantage of improvements. However, be cautious while updating to avoid introducing bugs or incompatibility into your project.
Bill Gates once said that “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” This certainly rings true here, where understanding and effectively automating how Gradle and other build tools handle your dependencies can save enormous amounts of time and effort in the future.
Make note that Android library modules are stored in AAR files and different modules may have different MinCompileSdk versions. Therefore, taking manual control of your Gradle script through the aforementioned methods will allow for superior management of your project’s dependencies and enable the smooth running of your resource compilation. (More on Android dependency management)
Applying Solutions to Resolve MinCompileSdk (31) Errors in Dependency’s AAR Metadata
The MinCompileSdk (31) error occurs when updating to Android Gradle Plugin (AGP) 7.0, which has introduced a new restriction that all the dependencies used in your project must have a minimum compile SDK version less than or equal to your project’s compile SDK version.
When it comes to the matter at hand: How To Solve The MinCompilesdk (31) Specified In A Dependency’s Aar Metadata, let’s dive right into the solutions.
Firstly,, you need to check the compileSdkVersion in your module-level `build.gradle` after you’ve updated your Android Gradle Plugin (AGP). If it is lower than 31, you should change it to
compileSdk 31
. This will ensure that it meets the requirement of AGP 7.0. Here’s a coding snippet with an emphasis on the relevant line enclosed within the
tag:
gradle
android {
compileSdk 31
defaultConfig {
applicationId “com.example.myapp”
minSdk 21
targetSdk 30
versionCode 1
versionName “1.0”
testInstrumentationRunner “androidx.test.runner.AndroidJUnitRunner”
}
}
Secondly,, if adjusting the compileSdk doesn’t resolve the issue, another way of tackling this is by downgrading the Android Gradle Plugin version to one doesn’t have the MinCompileSdk check. As an example in below code snippet, gradle version is modified to “4.2.2”.
dependencies { classpath 'com.android.tools.build:gradle:4.2.2' }
Thirdly,, you might consider checking the full error message as it typically highlights the library causing the problem, and then evaluating this dependency in question. Is it critical for your application? What purpose does it serve? Depending on your observations, you may go for one of two routes – either update the troublesome library to its latest version if available using the
dependencies {}
block in gradle file or alternatively consider removing it if it’s no longer maintained or necessary for your app.
Having said that, it’s important to point out the words of Edsger W. Dijkstra – “Simplicity is prerequisite for reliability”. So, choose balanced solutions considering the complexity they add and benefit they offer. Future proof your solution by keeping up with changes in AGP, as it helps avoid such scenarios.
Insights from Experts: Addressing Common Troubles with MinCompileSdk Issues
When it comes to addressing common difficulties with MinCompileSdk issues, particularly centering on “How To Solve The Mincompilesdk (31) Specified In A Dependency’s AAR Metadata,” expertise opinion unravel some enlightening views.
The prime issue arises when the minimum compile SDK value specified in a dependency’s Android Archive (AAR) metadata is higher than that of your app. This could lead often stumble upon build errors. Pertaining to these instances, Jake Wharton, an influential name in Android development ecosystem, points out:
“One practical reality of Android development is being aware of and managing different API levels within your app and its associated dependencies.”
So how does one address this issue successfully? There are few effective strategies:
Firstly, updating your app’s MinCompileSdk version is often the quickest fix. Simply change the `minCompileSdk` in your app-level `build.gradle` file. Here’s an illustration:
android { defaultConfig { minSdkVersion 21 targetSdkVersion 31 compileSdkVersion 31 } }
Remember, keeping the `compileSdkVersion` updated to the latest SDK can facilitate smooth adoption of new features and APIs while ensuring desired compatibility levels.
Next, you might want to take care of library dependencies. If a library is forcing you to raise your `minCompileSdk`, consider whether it’s absolutely crucial for your application or if there’s another library available that supports a lower `minCompileSdk`. A snippet on managing dependencies:
dependencies { implementation 'com.example.mylibrary:1.0.0' implementation ('com.example.otherlibrary:2.0.0') { exclude module: 'com.example.unwantedlibrary' } }
Finally, engage actively within the open-source community. Contributing to library projects and helping them maintain backwards compatibility could benefit not just you, but countless other developers dealing with similar issues.
Within all this, however, as Google Developer Expert Husayn Hakeem reminds us:
“Good code isn’t just about solving problems, but about managing complexities.”
This aligns perfectly with our scenario; managing complexities lies at the heart of resolving MinCompileSdk (31) related issues. Utilizing the knowledge of the broader development community and staying abreast with updates can prove instrumental in overcoming such challenges.
Programming in Java and dealing with dependencies often brings with it challenges. One such challenge is the minCompileSdk (31) specified in a dependency’s AAR metadata. This issue usually stems from situations where your project’s `minSdkVersion` is lower than that of one of the libraries you are attempting to incorporate within your project.
The simplistic solution involves synchronizing the `minSdkVersion` of your app module with that of your library by updating it to at least 31. Here is an example:
code
android {
compileSdkVersion 31
defaultConfig {
applicationId “com.example.myapp”
minSdkVersion 31
targetSdkVersion 31
versionCode 1
versionName “1.0”
}
…
}
However, this method might not be quite feasible if your application supports older Android versions (before SDK Version 31).
Another practical approach is to downgrade the version of the library in question but this can be risky as the previous versions may lack essential features or have unresolved bugs.
The inherent complexities of managing Android dependencies emphasize the need for careful planning in your coding processes. As Robert C. Martin, author of “Clean Code” enlightens us, “Truth can only be found in one place: the code.”
Unlock the potential of modern Android APIs while retaining backward compatibility. The Android documentation webpage spotlights how to configure your project to use certain Java 8 language features in your code.
Finally, enforce the practice of regularly updating your tools, plugins, and libraries which would invariably mitigate issues related to AAR Metadata MinCompileSdk. Google’s constant advancement in technology ought to keep developers abreast, enhancing their coding efficiency and acumen.