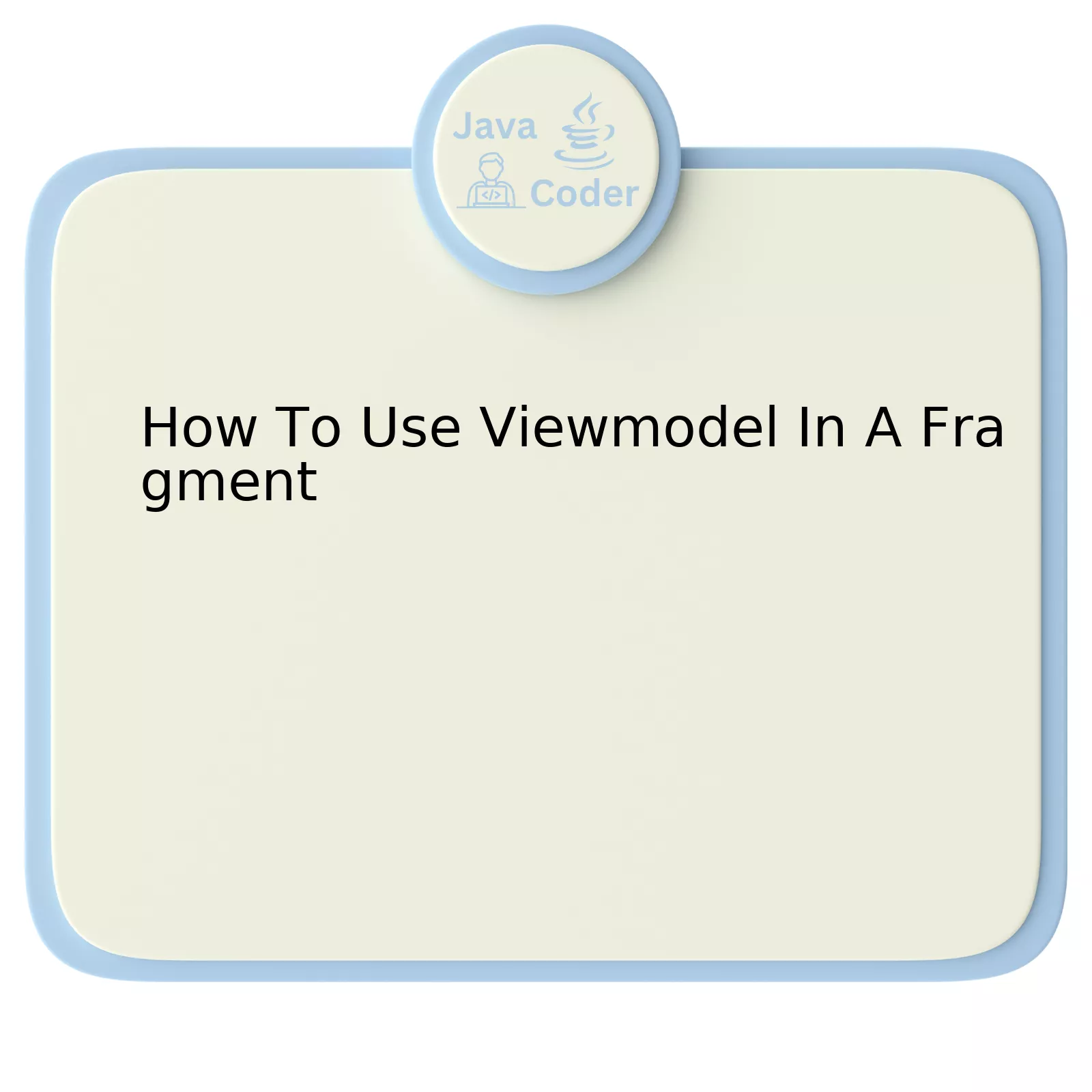
A critical aspect of Android development is maintaining robust and performant apps. One element that aids in this pursuit is the ViewModel, particularly in fragments. This tool is instrumental in structuring code effectively.
Steps | Description |
---|---|
Create a ViewModel class | This class will hold and manage UI-related data in a lifecycle conscious way |
Initialize the ViewModel | A ViewModel is initialized in an associated fragment using the ViewModelProvider |
Use LiveData | Live Data is an observable data holder class. When used with ViewModel, it allows for automatic updates in data across the lifespan of a given activity or fragment. |
Add necessary data | In the ViewModel add methods for providing necessary data to the UI controller |
Creating a ViewModel provides numerous benefits – primarily being able to retain data in configuration changes such as screen rotations.
A ViewModel is explicitly created by extending the ViewModel class.
public class SharedViewModel extends ViewModel { private final MutableLiveData- selected = new MutableLiveData
- (); public void select(Item item) { selected.setValue(item); } public LiveData
- getSelected() { return selected; } }
Here, consider the `SharedViewModel` class which holds the information related to a particular Item. The `LiveData` inside this ViewModel reacts to data change overtime thus making any changes immediately visible to the observers listening to it.
We instantiate our ViewModel inside the fragment with:
viewModel = new ViewModelProvider(this).get(SharedViewModel.class);
ViewModelProvider being a utility class provides the ViewModel and makes sure that it survives configuration changes.
Incorporating LiveData into your ViewModel ensures continuous data updates during active lifecycles. You just need to observe the LiveData instance:
viewModel.getSelected().observe(getViewLifecycleOwner(), new Observer- () { @Override public void onChanged(@Nullable Item item) { // Update the UI. } });
Great developers utilize these tools to achieve efficient, state-of-the-art application design. As Joshua Bloch mentioned; “Good code is its own best documentation”. Exploring advanced tools such as ViewModel, keeps you closer to writing ‘Good Code’.
Understanding the Role of ViewModel in Fragment Operations
ViewModel plays a significant role in managing UI-related data in the lifecycle of fragments in Android applications. Acting as a store for view-based data, ViewModel empowers developers to maintain consistent features and experiences for users, even through changes such as screen rotations or navigation between fragments.
In context of using ViewModel within a Fragment:
Data retains across fragment instances.
Through its architected design, ViewModel ensures that data remains unaffected by fragment lifecycle events such as creation, pause, resume or destruction. This is beneficial in scenarios where background tasks are running concurrently with UI-User interactions, ensuring a smooth user experience.
Leverage on the ‘lifecycle-aware’ nature.
ViewModel’s “lifecycle-aware” state automatically resolves common issues associated with handling UI data, including memory leaks or crashes due to stopped activities. This promotes safer and more reliable code within your application.
To illustrate how ViewModel can be utilized in a fragment, here’s an example in Java:
public class MyFragment extends Fragment { private MyViewModel mViewModel; @Override public void onActivityCreated(@Nullable Bundle savedInstanceState) { super.onActivityCreated(savedInstanceState); // Initialize ViewModel mViewModel = new ViewModelProvider(this).get(MyViewModel.class); // Observer for data change mViewModel.getSomeData().observe(getViewLifecycleOwner(), new Observer() { @Override public void onChanged(String s) { // Update UI } }); } }
Above, we create a new instance of `MyViewModel` in the `onActivityCreated()` method, which only execute once per the entire lifecycle of the Fragment. Then we use the `getSomeData().observe()` method to update the UI when the underlying data changes.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, author of Refactoring: Improving the Design of Existing Code paints a vivid picture of why leveraging these modern architectural components like ViewModel, should be considered in the process of crafting our code.
Overall, using ViewModel fosters a clean and modular architecture by providing a robust and streamlined manner of managing UI data within Fragments thus resulting in applications being more resilient and efficient.
Leveraging ViewModels for Enhanced Data Management in Fragments
ViewModels are powerful tools that take data management in fragments to the next level by providing an efficient way to manage your UI data while also separating it from your UI controller. They were introduced as a part of Android Architecture Components, a collection of libraries that helps you design robust, testable, and maintainable apps. To fully grasp what makes them such a boon for developers, let’s delve further into their specifics.
Why Use ViewModels?
A ViewModel serves multiple purposes but its principal function revolves around the concept of data ownership. It owns and preserves data across lifecycle changes. Here are some of the additional benefits:
-
Survives Lifecycle Events:
The data in the ViewModel survives configuration changes like screen rotations. So, you don’t lose data.
-
Separation of Responsibilities:
It assists in achieving separation of concerns by removing data handling capabilities from the UI controller.
-
Avoids Memory Leaks:
Data stored in the ViewModel is automatically cleared when it’s no longer needed, preventing memory leaks.
In the context of fragment usage specifically, a ViewModel ensures consistency and retains the information even when the fragment is destroyed and recreated.
How to use a ViewModel in a Fragment?
To leverage a ViewModel in a Fragment, there are certain steps to be followed. Note that using ViewModel requires adding the dependency to your app-level build.gradel file. Below is a general overview defining the typical flow.
- First, define a ViewModel class. This is done by extending the ViewModel class and creating the necessary variables or LiveData objects for storing your data. For example:
public class MyViewModel extends ViewModel { private final MutableLiveData
- selectedItem = new MutableLiveData
- (); public void selectItem(Item item) { selectedItem.setValue(item); } public LiveData
- getSelectedItem() { return selectedItem; } }
- selectedItem = new MutableLiveData
- Next, associate the ViewModel with your fragment. To do this, you initialize a ViewModel instance in your fragment using ViewModelProvider. For example:
public class MyFragment extends Fragment { private MyViewModel model; public void onActivityCreated(Bundle savedInstanceState) { super.onActivityCreated(savedInstanceState); model = new ViewModelProvider(this).get(MyViewModel.class); model.getSelectedItem().observe(this, item -> { // update UI }); } }
By incorporating these steps into your fragment development workflow, you can efficiently enhance data management from start to finish.
“Bugs come from untested corners. Using a ViewModel drives your code towards making sure that those corners get tested.” – Marcin Moskala, author of “Effective Kotlin”. Keep this in mind when dealing with data management within your Android applications.
For more detailed and specific knowledge about ViewModels and fragments in Android, you may want to check this official documentation.
Step-by-step Guide: Implementing ViewModel within a Fragment
Implementing ViewModel within a Fragment provides an efficient way to retain data even when your Android application goes through life-cycle states such as recreations due to transformations or settings modifications.
The initial step for integrating ViewModel into a Fragment is the inclusion of dependencies in the gradle file:
dependencies { def lifecycle_version = "2.2.0" implementation "androidx.lifecycle:lifecycle-viewmodel:$lifecycle_version" // ViewModel }
It’s worth noting that, rather than hard-coding a specific version, it’s a good practice to store the lifecycle_version you’re using as a variable. This eases maintenance and promotes modularity.
Focusing on implementing the ViewModel, define a ViewModel class for your specific use-case. Every distinct data situation could require its unique ViewModel class. Consider this example of a ‘ProductViewModel’:
import androidx.lifecycle.ViewModel; public class ProductViewModel extends ViewModel { // private instance variables here e.g., a list of products public void setProducts(Listproducts) { // update the local list } public List getProducts() { // return current product list } }
In this code snippet, our `ProductViewModel` manages a list of Products, the fundamental elements of the app.
Subsequently, you have to associate the ViewModel with your Fragment. Here’s how you can initialize the `ProductViewModel` within the Fragment:
public class ProductFragment extends Fragment { private ProductViewModel productViewModel; @Override public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) { super.onViewCreated(view, savedInstanceState); // Get a reference to the ViewModel for this screen. productViewModel = new ViewModelProvider(this).get(ProductViewModel.class); // Observe changes to the products held by the ViewModel productViewModel.getProducts().observe(getViewLifecycleOwner(), new Observer>() { @Override public void onChanged(List
products) { // update UI // for example, update a RecyclerView adapter with the updated product list } }); } }
In this preparation, an instance of the ViewModelProvider generates the ViewModel i.e., `productViewModel`. It connects lifecycle information with the specified ViewModel. The `getViewLifecycleOwner()` function retrieves the relevant lifecycle owner from the Fragment’s vision hierarchy. Last but not least, an observer monitors changes in data( product list).
As Bill Gates, co-founder of Microsoft, once stated, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Hence, including ViewModel in your Fragments is one way to foster seamless data management in your applications [source](https://www.goodreads.com/quotes/6526-the-advance-of-technology-is-based-on-making-it-fit).
Common Pitfalls and Best Practices when Using ViewModel with Fragments
The ViewModel is a class from the Android Architecture Components, designed to store and manage UI-related data in a lifecycle-conscious way. Specifically used with fragments, it empowers developers to manage screen-oriented data which can outlive changes like configuration changes during fragment lifecycle transitions.
However, using ViewModel with Fragments has its pitfalls if certain guidelines aren’t accurately followed:
Incorrect Usage of ViewModel:
A common mistake among quite a few developers occurs when creating ViewModels. The creation should require no parameters in the constructor, and the ViewModel’s instance should be retrieved via methods. If created improperly, it may lead to crashes or loss of data.
// ViewModel creation to avoid MyViewModel viewModel = new MyViewModel();
Duplicating ViewModel Instances:
Another frequent pitfall is the mistake of creating duplicate instances of ViewModel in different components/fragments causing data inconsistency.
How to successfully incorporate ViewModel within a Fragment requires following best practices:
Instantiation Using ViewModelProvider:
ViewModels should always be instantiated using ViewModelProvider. This ensures correct scope management and discourages the wrong assumption of recreating an existing ViewModel as every call to,
new ViewModelProvider(this).get(MyViewModel.class);
will provide you with the same ViewModel instance across the component or fragment lifecycle.
Updating UI Based on ViewModel State:
On the event that a ViewModel alters any observed LiveData object, the system automatically triggers any observer interfaces on the main thread, hence there is no need for manual synchronisation.
Here’s an example of updating the UI based on ViewModel state change:
myViewModel.getData().observe(this, myData -> { // Update the ui. });
Refer to the official android documentation here.
“These architecture components are about taking care of your app for you,” as Mike Cleron Linux Kernel Committee Chair said, “they’re about making it easier to write robust apps.”
By adhering strictly to these guidelines and understanding how ViewModels work, continuous updates on real-time values across different states becomes seamless with minimal error margin.
To extract the essence of ‘How To Use ViewModel In A Fragment’, it’s imperative to first conceptualize how a Fragment and ViewModel work together in a consolidated software architecture.
ViewModel has been engineered for managing and storing UI-related data in a lifecycle aware manner. The ViewModel class is architected to hold and manage UI-related data in a lifecycle conscious way and enables data to survive device-configuration changes such as screen rotations.
In a simplified outline, Using ViewModel in a Fragment involves these key steps:
- Creation of a ViewModel class.
- Initializing the ViewModel in a Fragment.
- Utilizing LiveData for observing changes in ViewModel from the Fragment.
Moreover, utilizing the ViewModel class allows data changes to be observed within the UI components based on lifecycle states. This reciprocal association between ViewModel and Fragments enhances code efficiency and boosts performance.
Let’s visualize this interaction with a code snippet:
public class MyViewModel extends ViewModel { private final MutableLiveData- selectedItem = new MutableLiveData
- (); public void selectItem(Item item) { selectedItem.setValue(item); } public LiveData
- getSelectedItem() { return selectedItem; } }
Here, we can observe how the ViewModel manipulates LiveData instance that represents an Item and provides methods to alter its state. Now, for the Fragment section, the ViewModel is initiated in the following way:
public class MyFragment extends Fragment { public void onViewCreated(@NonNull View view, Bundle savedInstanceState) { super.onViewCreated(view, savedInstanceState); MyViewModel model = new ViewModelProvider(this).get(MyViewModel.class); model.getSelectedItem().observe(getViewLifecycleOwner(), item -> { // Update the UI. }); } }
Evidently, adopting ViewModel within Fragments proffers ease of manipulation, efficient handling, and lifecycle-aware data management making your application resilient and streamlined.
As Turing Award winner Tony Hoare said, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies. The first method is far more difficult.” ViewModel encourages the first approach, maintaining simplicity and effectiveness throughout.
For further reading, The official Android Developer’s Guide provides comprehensive insights.