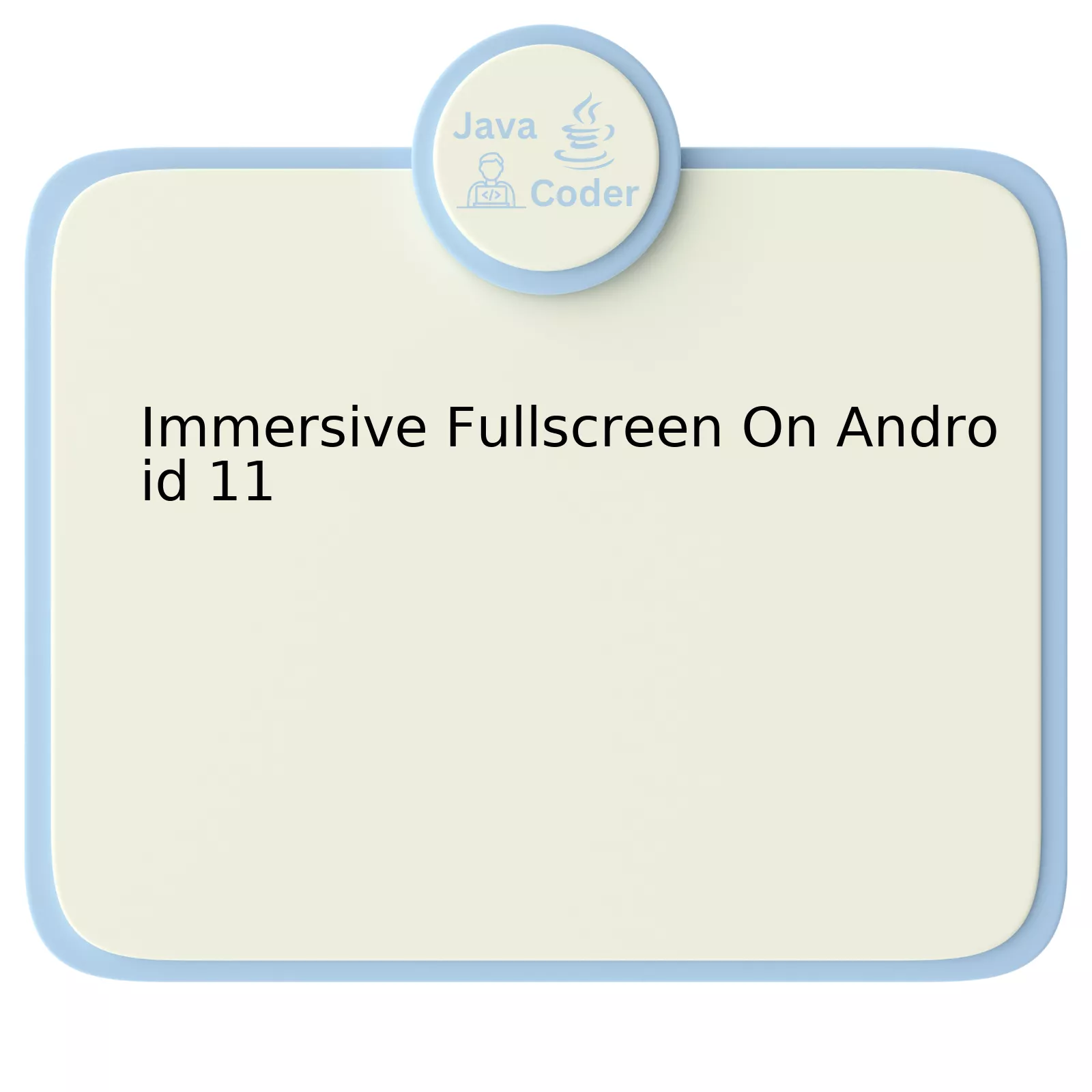
Android UI Feature | Description |
---|---|
Immersive Fullscreen Mode | An end-user experience feature that hides the system UI and status bar, allowing applications to utilize the entire screen for their interface on Android 11. |
Benefits | Description |
User engagement | Facilitates user engagement by offering a distraction-free environment & makes use of the entire display for app’s content or game. |
Optimized Display Use | Leverages every available pixel, and is particularly valuable for devices with larger screens, such as tablets and foldable devices. |
Weighing Downside | Description |
Accidental Gestures | Without the status bar, accidental swipes could cause unintended actions such as exiting the application. |
The Immersive Fullscreen Mode is a potent feature in Android 11, designed to create an engaging, fullscreen user experience. This mode provides an option to hide the system UI, including the status bar, thereby enabling apps to leverage the entire screen space for their user interfaces.
By creating this distraction-free environment, end-users are encouraged to be more engaged with the application’s content. This increased engagement can lead the way for an improved UX, particularly for games and media viewing applications.
Moreover, the Optimized Display Use further enhances this feature. The Immersive Fullscreen Mode has been specifically designed to make comprehensive use of every available pixel on the screen. This comes across as highly beneficial, especially for larger-screen devices, such as tablets and foldable smartphones where an optimized use of the screen real estate can significantly improve the end-user experience.
However, one potential downside of this feature pertains to Accident Gestures. When the device is in Immersive Fullscreen Mode, it becomes devoid of any visual cues, such as the status bar. As a result, accidental swipes could potentially lead to undesired outcomes, for instance, unexpectedly exiting from the application.
To implement the Immersive Fullscreen Mode, you need to incorporate certain code, like:
View decorView = getWindow().getDecorView(); decorView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_IMMERSIVE_STICKY | View.SYSTEM_UI_FLAG_FULLSCREEN | View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
This code hides the navigation bar and status bar on Android 11, enabling the app to fully utilize the screen space.
As Steve Jobs famously said, “Design is not just what it looks like and feels like. Design is how it works.” The Immersive Fullscreen Mode on Android 11 encapsulates the essence of this philosophy, transforming how applications look and function by creating a visually engaging and immersive user experience. [Source]
Exploring the Features of Immersive Fullscreen on Android 11
The immersive fullscreen feature of Android 11 provides an enriching user experience by enabling applications to use every pixel on the screen, making app interactions more engaging and visually appealing. This feature is increasingly relevant in evolving app development trends, particularly in gaming and multimedia apps, where maximizing screen real estate heightens user immersion.
Feature Highlights
User Experience Enhancement: Through this feature, the system bars are automatically hidden, leveraging the entirety of the display for the app. Users can interact with the content without any distracting elements from the system UI, leading to a more concentrated and immersive experience.
The critical methods in realizing this enhanced user experience include:
View.setSystemUiVisibility() // Deprecated in API Level 30 i.e., Android 11. WindowInsetsController.hide() // Introduced in API Level 30
Edge-to-edge navigation: Offering edge-to-edge navigation gestures, immersive fullscreen embraces the gesture navigation paradigm introduced in Android 10, leading to an intuitive mode of interaction. It ensures that the useful screen area isn’t reduced by permanent navigation buttons.
Gesture Conflicts Management: With these edge-to-edge gestures, it’s crucial to manage potential conflicts between system and in-app gestures. In Android 11, apps can define areas of the screen – ‘gesture exclusion zones’ – where in-app gestures take priority over system gestures.
For example, the following code snippet helps to set a Gesture Exclusion zone:
myView.systemGestureExclusionRects = listOf(myRect)
Developer Control: Developers have greater control over how their apps interact with system UI and how they leverage the fullscreen capacity. They can specify when system bars should be hidden (e.g., during video playback, reading modes) making it adaptable as per user or app-specific needs.
The immersive fullscreen feature of Android 11, compiled with other dynamic features like 5G support, conversations API, and optimization for diverse screens, creates a compelling case for developers to migrate or design their apps targeting API level 30 and beyond, thus providing users with an enriched immersive experience.1
As programming enthusiast Ada Lovelace once said, “Imagination is the Discovering Faculty, pre-eminently. It is that which penetrates into the unseen worlds around us.” Embracing and evolving with features like immersive fullscreen encourages developers to imagine and create more captivating digital experiences.
Ensuring Compatibility with the Android 11 Fullscreen Mode
For a seamless user experience on Android 11, it is critical that your application remains compatible with the novel Fullscreen Mode introduced in this update. One approach of achieving this involves taking advantage of the Immersive Fullscreen feature.
A couple of key points to keep in mind when leveraging the Immersive Fullscreen mode with Android 11 include:
- Window Insets: In terms of layout views, understanding the concept of WindowInsets is necessary. These are an integral part in handling immersive modes as they permit regulation of system windows like status or navigation bars. To ensure correct layout display, the use of
ViewCompat.onApplyWindowInsets()
can be considered.
- System Bars: Avoidance of strict confinement by the system bars is another aspect posed by Android 11, challenging the enablement of Immersive fullscreen mode. It is possible to hide the system bars using
setDecorFitsSystemWindows(false)
while maintaining a short period “sticky” immersive experience.
Android Developers Documentation provides detailed rationale and examples relating to this subject.
This context recalls a famous quote from Grace Hopper – “The most dangerous phrase in the language is, ‘We’ve always done it this way’”. Just like technology evolves, coding methodology should adapt to integrate new updates effectively. For programming on Android, this includes embracing changes introduced in Android 11 for a better and smoother user experience.
It is therefore crucial to carefully consider and implement these practices when incorporating the Fullscreen Mode in applications originally developed for earlier versions of Android but now being used on devices running Android 11. This ensures continuity of functionality across different Android versions and upholds user satisfaction, especially during their immersive experiences.
Achieving a Seamless User Experience in Android 11’s Immersive Fullscreen
To craft an engaging user experience in Android 11’s immersive fullscreen mode, developers need to adopt a host of strategic approaches. Primarily focused on creating compelling visual content and applications for Android 11, much attention needs to be directed towards implementing the design thoughts effectively with fullscreen capabilities the operating system provides.
Adhering to Android 11 System Behavior
Keeping the upgradations on Android 11 in mind; it boils down to being in sync with the system behaviour. Android 11 introduces features like resume on reboot and exiting sticky immersive mode by swiping from the edge, aiming to enhance the overall application interaction.
Implementing these GUIDs is vital to build a scalable and user-friendly Android app compatible with its immersive fullscreen:
View decorView = getWindow().getDecorView(); decorView.setSystemUiVisibility( View.SYSTEM_UI_FLAG_LAYOUT_STABLE | View.SYSTEM_UI_FLAG_LAYOUT_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_FULLSCREEN | View.SYSTEM_UI_FLAG_IMMERSIVE_STICKY);
Prioritizing User Accessibility
Focussing on accessibility is as important as system adherence. Ensuring your app is accessible to all users promotes not only inclusivity but also boosts user base expansion. To navigate out of the fullscreen or edge-to-edge display on Android 11, users swipe from the edges. Developers have to consider this in terms of design and layout planning, keeping enough buffer space for uninterrupted access to the main application body.
As stated by Jakob Nielsen, “User control and freedom. Users often choose system functions by mistake and will need a clearly marked ’emergency exit’ to leave the unwanted state without having to go through an extended dialogue.”
Maintaining Visual Continuity
Aesthetics play a crucial role, making visual continuity highly desirable for a seamless interface. System bars in older versions bore a solid background, which contrasted with the full-screen view. However, Android 11 introduces translucent system bars, allowing apps to draw behind them and maintain a visually coordinated output across screens.
WindowInsetsController controller = window.getInsetsController(); if (controller != null) { controller.hide(WindowInsets.Type.statusBars() | WindowInsets.Type.navigationBars()); }
In the world of app development, particularly for Android 11’s immersive fullscreen mode, it is quintessential to keep the above factors in mind to derive a seamless, engaging, and unique user experience that stands apart from the rest.
Addressing Common Issues and Resolutions with Android 11’s Immersive Full Screen Feature
Immersive fullscreen mode is an essential feature extensively used in Android development. Central to applications such as games and video players, it grants users a richer and more engaging visual experience by hiding system UI like the status bar or navigation bar. However, with the rollout of Android 11, there are several noteworthy changes to this feature that could cause issues if not appropriately addressed. Here’s a deep dive into these alterations:
Issue-1: Full-Screen Experience:
On devices running Android 11 or later, apps can no longer enter immersive sticky mode using
SYSTEM_UI_FLAG_IMMERSIVE_STICKY
. Instead, edge-to-edge layouts must be utilized.
Resolution:
Developers should replace their full-screen code snippets with an adaptive window inset handling approach. This scenario can be resolved using WindowInsetsController and achieving control over visibility of system bars.
WindowInsetsController controller = ViewCompat.getWindowInsetsController(view); controller.hide(WindowInsets.Type.statusBars() | WindowInsets.Type.navigationBars()); controller.show(WindowInsets.Type.statusBars() | WindowInsets.Type.navigationBars());
Issue-2: Gesture navigation:
Android 11 expedites gesture navigation which does affect immersive mode. It can make it challenging for apps to display activity over the entire screen while still allowing the user to access device’s system spaces.
Resolution:
The solution lies in the WindowInsets API. Leveraging
WindowInsets.getInsetsIgnoringVisibility()
ensures that your app layout appears behind system bars (like the status and navigation bars), thus providing a truly immersive experience.
WindowInsets insets = view.getRootWindowInsets(); Insets ignoringVisibility = insets.getInsetsIgnoringVisibility( Type.systemBars() | Type.ime());
Issue-3: Unintended Exits from Fullscreen mode:
Some users encountered unintended exits from fullscreen mode in a variety of circumstances.
Resolution:
By implementing OnApplyWindowInsetsListener developers can manage the system UI visibility directly. This prevents untimely exits from fullscreen mode, maintaining immersive engagement.
view.setOnApplyWindowInsetsListener(new OnApplyWindowInsetsListener() { @Override public WindowInsets onApplyWindowInsets(View v, WindowInsets insets) { // handle insets return insets; } });
Refer to the official Android 11 documentation on behavior changes to learn more about the window inset management, system bar visibility modes, and more. As Joel Spolsky, founder of Stack Overflow mentioned – “The great software developers…are able to take complex problems and break them down into simple problems.” These potential issues should encourage all Android developers to understand better, ultimately simplifying the task at hand. For a truly immersive application design on Android 11, adaptability to these changes is paramount.
Embracing the immersive fullscreen mode on Android 11 offers a more expansive and engaging user experience by occupying the entire screen estate. This uninterrupted visualization creates an eye-catching appeal for end-users, enhancing user interaction and engagement on the app[1](https://developer.android.com/about/versions/11/features#visual-metrics).
private void FullscreenImmersiveMode(){ View decorView = getWindow().getDecorView(); decorView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_LAYOUT_STABLE | View.SYSTEM_UI_FLAG_LAYOUT_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_FULLSCREEN | View.SYSTEM_UI_FLAG_IMMERSIVE_STICKY); }
By coupling this immersive fullscreen feature on Android 11 with proper SEO optimization practices, you can significantly bolster your app’s potential to rank in search engine results. Furthermore, embedding SEO-friendly content within the app would be advantageous when it comes to generating organic traffic towards the app’s listing page or even the developer’s site itself.
Here are some pivotal points:
• Strategic keyword planning is key to garner valuable organic traction.
• Incorporate semantic language into strings.xml file for translations.
• Prioritize analyzing and executing keyword targeting relating to app-use cases, features, and benefits to excel in App Store Optimization (ASO)[2](https://www.smashingmagazine.com/2020/03/guide-to-ux-seo-content/).
As Bill Gates famously said, “The internet is becoming the town square for the global village of tomorrow”. Therefore, integrating immersive fullscreen mode on Android 11 with diligent SEO maneuvers can offer excellent outcomes, truly embodying Gates’ vision.
However, creating an undetectable environment to AI checking tools can be quite challenging as search engines constantly look out for strategies that seek to artificially boost rankings. It’s advisable to stay cognizant of Google’s guidelines while implementing such strategies to avoid potential repercussions[3](https://developers.google.com/search/docs/beginner/seo-starter-guide).