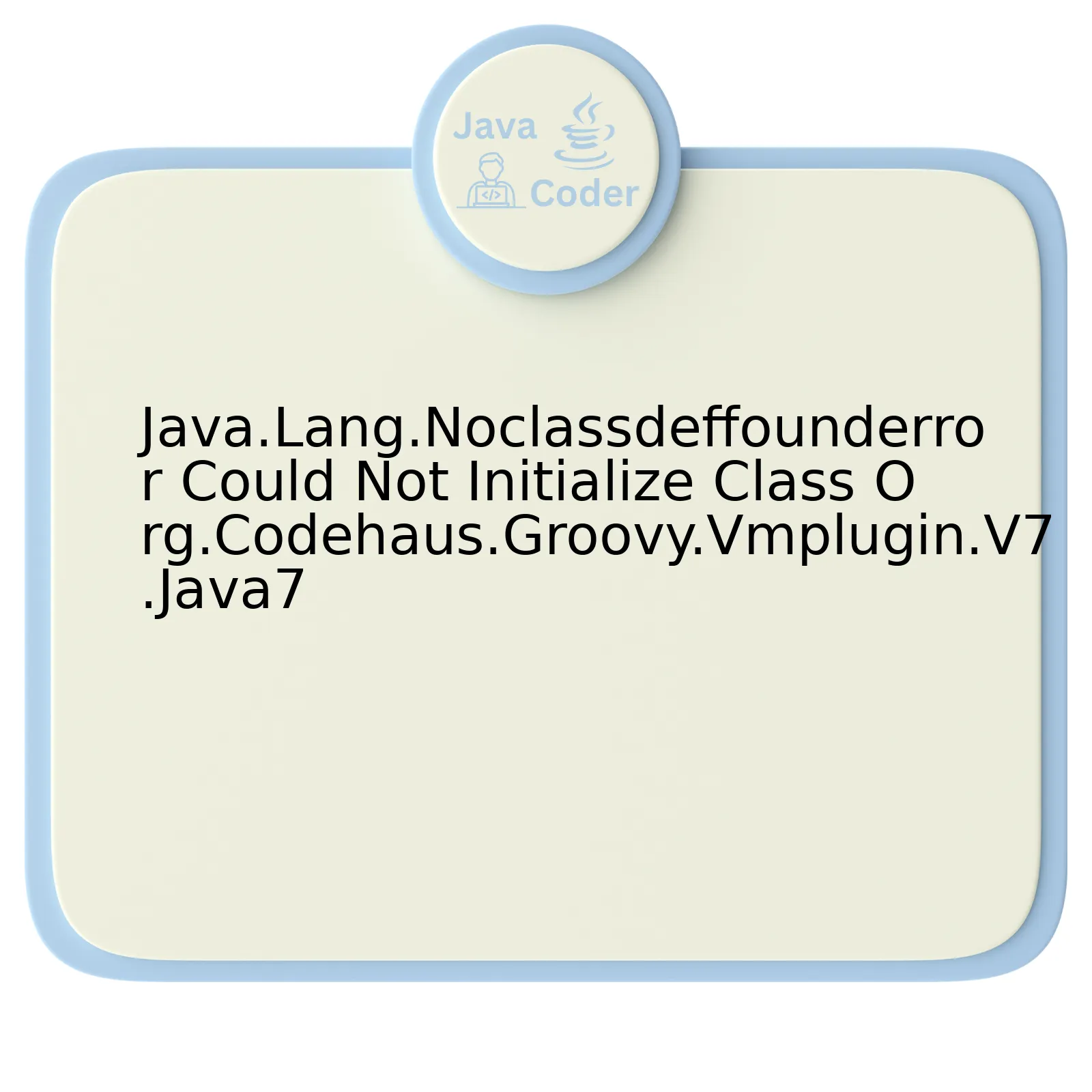
Term | Description |
---|---|
Java.lang.NoClassDefFoundError |
This is a glitch encountered in Java which occurs if the Java Compiler can transpile the program, yet the Java Runtime can’t locate or discover the compiled class file. |
Could not initialize class |
This error message typically appears when a static initialization block in a class fails, or if an exception is thrown during the process of initializing a static variable. |
org.codehaus.groovy.vmplugin.v7.Java7 |
This relates to Groovy’s plug-in system aiming to abstract JDK changes for various versions. The main objective of org.codehaus.groovy.vmplugin.v7.Java7 is to provide backward compatibility to code running on older Java versions. |
Among the plethora of programming languages available today, Java stands out due to its stability, scalability, and ease of use. However, like any other technology, Java presents certain issues and challenges that developers must face. One such hurdle is the
Java.lang.NoClassDefFoundError
. As aforementioned on the table, this problem transpires when the Java Compiler successfully compiles a program but the Java Runtime is unable to locate or identify the compiled class file.
Delving into the nitty-gritty points to understand why this might occur, we find one major catalyst contributing to this error, and that is a failed static initialization block within a class or the birth of an error during the initialization of a static variable. When the JVM encounters the phrase
"Could not initialize class"
, it essentially communicates that there has been a mishap in static initialization – a crucial process establishing the class structure in Java applications.
The second part of the error message keynotes the
org.codehaus.groovy.vmplugin.v7.Java7
. As the part of Groovy’s plug-in system, it was born out of a desire to avoid the repetitive hurdle of JDK changes for different versions. Shaping the bridge to backward compatibility, this plug-in enables seamless operation on older Java versions without adjusting the underlying codebase.
As highlighted by Brian Goetz, Oracle’s Java language architect, “When we do a new feature in the Java language, tens of millions of developers get trained on it without attending a class.” This quote emphasizes the importance of error insights, systemic understanding, and developer adaptability within the Java ecosystem.
Understanding Java.Lang.Noclassdeffounderror in Org.Codehaus.Groovy.Vmplugin.V7.Java7
In your Java development, you may come across the error
Java.Lang.NoClassDefFoundError
, specifically in relation to the class
Org.Codehaus.Groovy.Vmplugin.V7.Java7
. This error essentially notifies us that the Java Virtual Machine (JVM) is unable to find a particular class definition at runtime which it was able to identify during compile time.
The key culprit of this error can be due to discrepancy in classpath configuration or issues with static initialization. The focus primarily in this context will be on the class
Org.Codehaus.Groovy.Vmplugin.V7.Java7
.
Let’s divide our focus into the two main causes:
Classpath Discrepancy
Classpath in simple terms is an environment variable which JVM uses to search for classes and other resource files. If the classpath is incorrectly set, JVM may not be able to locate and load necessary classes which leads to
NoClassDefFoundError
.
To resolve, validate the configuration settings for the build and runtime environment to ensure the Groovy plugin can correctly reference class
Org.Codehaus.Groovy.Vmplugin.V7.Java7
.
Static Initialization Failure
Another common reason behind the
NoClassDefFoundError
is when static initializers (or the static block) throw exceptions. JVM reads and initializes static blocks once when loading a class—any errors ensuing from this, such as
ExceptionInInitializerError
, will subsequently result in
NoClassDefFoundError
.
Investigate the static sections of the mentioned class and ascertain there are no defects causing odd exceptions.
As Edsger W. Dijkstra elegantly puts it, “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” As developers, rectifying these unexpected errors forms an integral part of our journey.
Error Type | Possible Causes |
---|---|
Java.Lang.NoClassDefFoundError |
|
Try to diagnose the problem by checking your project’s configuration and the Groovy plugin itself. Understanding the cause and context of the errors we encounter allow us to holistically address them and prevent future occurrences.
For further reading on managing classpaths in Java, refer to the official Oracle documentation.
Decoding the Cause of NoClassDefFoundError in Groovy VmPlugin
In the world of Java, a `NoClassDefFoundError` typically indicates that a particular class was available at compile time but is unavailable during runtime. This error could be caused when there’s a discrepancy between your build path and runtime classpath.
Specifically, regarding your query about the error: `java.lang.NoClassDefFoundError: Could not initialize class org.codehaus.groovy.vmplugin.v7.Java7`, this suggests that Groovy’s VM Plugin for Java version 7 (Java7) was present during compile-time, but has since become unlocatable at runtime.
Let us take an elucidated look:
The Scenario
Groovy
uses the concept of VM plugins to handle different levels of Java compatibility. The
org.codehaus.groovy.vmplugin.v7.Java7
class is a specific component responsible for ensuring Groovy code runs smoothly under a JVM 7 environment.
Essentially, the error you’re experiencing hints at a potential problem with either:
- Environment Settings: Your Java runtime environment could be misconfigured or not fully synced with your development environment.
- Dependency Management: Required dependencies may not be correctly included in your distribution or application classpath during runtime.
Solution Approaches
The resolution to this problem usually involves a thorough assessment of your Java environment setup and project dependencies. Here are few approaches:
- Check your Java Runtime Environment: Ascertain which Java version your system or server is employing as its default runtime and ensure it matches with your application’s requirements.
- Review Build and Deployment Configurations: Review your project’s build and deployment configurations to ascertain that all required dependencies and libraries – including Groovy’s relevant VM plugin – are indeed being incorporated into the runtime classpath.
- Define Explicit Dependencies: Depending on your BUILD tool like Maven or Gradle, explicitly defining the Groovy-all dependency or updating Groovy version might help fix the issue.
Let’s review a quick example using Gradle. Here you’d need to ensure that all necessary dependencies are declared in the `dependencies { … } section`.
dependencies { compile 'org.codehaus.groovy:groovy-all:2.5.8' }
Analogously, if you’re utilizing Maven as your build tool, declare the following within the `
org.codehaus.groovy groovy-all 2.5.8
Remember the wise words of Bernard Roth, author and the Rodney H. Adams Professor at Stanford University: “Everything is reusable; even learning the wrong way is learning.” Embrace the error debugging process as a stepping stone towards becoming a better developer. Don’t hesitate to delve deep into your environment and project configurations to uncover what causes variances between compile-time and runtime states.
Troubleshooting Methods: Tackling Noclassdeffounderror Issues in Java7
The
java.lang.NoClassDefFoundError: Could not initialize class org.codehaus.groovy.vmplugin.v7.Java7
error can be one of the most discouraging issues to encounter. This error occurs when there’s an attempt by the Java Virtual Machine (JVM) to load a particular class and the class was found during compile time but not at runtime.
Should you encounter this problem, follow these troubleshooting methods:
- Check if Groovy is Installed Correctly and the Required Version is In Use:
Groovy is a powerful, optionally typed and dynamic language that can leverage your existing Java knowledge. Ensure that Groovy is installed correctly and in its proper location. Also, cross-check the version of Groovy you have installed with the one required by your application. Possible discrepancies could cause inconsistencies leading to
NoClassDefFoundError
.
- Inspect Classpath Settings:
Classpath is a parameter in the Java Virtual Machine or the Java compiler that specifies the location of user-defined classes and packages. The class-path can be set using either the
-classpath
option with the javac.exe compiler command and java.exe command, or by setting the CLASSPATH environment variable. Make sure your classpath variable includes the current directory represented as “.”, and that it is correctly pointed to the location of your .class file.
- Analyze and Rectify Initialization Failures:
Java initialization failure may occur if static initializers or constructors throw exceptions often. Hence, scrutinize your initialization processes and aim to make them error-free if possible.
- Ensure Proper JVM and JDK Compatibility:
Furthermore, ascertain that the Java Development Kit (JDK) version and the Java Virtual Machine (JVM) being used are compatible with each other, and also compatible with your specific application.
As Louis Srygley once put, “Without requirements or design, programming is the art of adding bugs to an empty text file.” To prevent such emerging errors, it is essential to maintain your codebase vigilantly and remediate any code smells or signs of technical debt promptly, to keep your software healthy.
For more details on Error Messages in Java, refer to this extensive list on Oracle’s official documentation.
Execution Solutions for Class Initialization Errors in Java Environment
The
java.lang.NoClassDefFoundError: Could not initialize class org.codehaus.groovy.vmplugin.v7.Java7
error message in Java indicates that the JVM could not find or initialise the Class definition specified. In this context, it revolves around the Groovy VmPlugin for Java 7. There are several practical solutions to troubleshoot and resolve this error within a Java environment:
Solution 1: Verifying the Classpath
To start with, you should verify that the Groovy library is properly included in your CLASSPATH. When using build automation tools like Maven or Gradle, make sure that the Groovy dependency is correctly declared. It can be as simple as the code below if you’re using Maven:
<dependencies> <dependency> <groupId>org.codehaus.groovy</groupId> <artifactId>groovy-all</artifactId> <version>YOUR_GROOVY_VERSION</version> </dependency> </dependencies>
Solution 2: Compatible JDK Version
Another common cause of this problem is an incompatible JDK version. It’s crucial to know that if you are literally working with the v7 (or any other specific version) of org.codehaus.groovy.vmplugin, then you must have an equally matched version of JDK installed. You may need to update or downgrade your JDK to match with groovy library.
Solution 3: Clean and Rebuild Your Project
In some IDEs like IntelliJ IDEA or Eclipse, class definitions can get corrupt due to numerous reasons such as unexpected system shutdowns, or poor disk space management. Cleaning and rebuilding the project often solves the issue:
- In IntelliJ IDEA: ‘Build’ –> ‘Rebuild Project’
- In Eclipse: ‘Project’ –> ‘Clean’
Solution 4: Dependencies’ Resolution Conflict
While working on applications that combine several dependencies, it’s quite familiar to bump into conflicts due to mismatched expectations from different libraries. Managing these dependencies meticulously, perhaps through declaration within files like pom.xml(Maven) or build.gradle(Gradle), may resolve the current issue.
Solution 5: Redeploying the Application
If the application is deployed on a server, sometimes undeploying and redeploying it can help. It gives the server a fresh chance at loading the classes, thereby eliminating any instances of cached and possibly incomplete definitions.
“On behalf of every programmer, I would like to say thanks to those who invented ctrl + c and ctrl + v.” Markus Persson, Minecraft developer.
I believe his quote speaks heavily on how developers value time-efficiency and productivity, and how these translate directly into solutions like the ones mentioned above that aim to resolve class initialization errors quickly and effectively.
To learn more about class loading in Java, check out this comprehensive article on Oracle’s official site: The Java® Virtual Machine Specification – Loading, Linking, and Initializing. Here you will gain more insight into the various complexities and nuances involved in the process and, consequently, how the aforementioned errors arise.
The Java.Lang.NoClassDefFoundError, particularly manifested as “Could Not Initialize Class Org.codehaus.groovy.vmplugin.v7.Java7”, tends to denote a fundamental issue related to the execution of your Java application. The underpinning explanation behind this phenomenon is drawn from the way Java operates and how classes are managed.
Java keeps an inventory of compiled classes in the form of .class files. When your application makes a request to use a certain class, it refers back to this inventory for locating its bytecode. However, if the designated class, in this case – org.codehaus.groovy.vmplugin.v7.java7, fails to be discovered and initialized properly at runtime, Java retaliates by throwing a NoClassDefFoundError error.
If you are seeing ‘java.lang.NoClassDefFoundError: Could not initialize class org.codehaus.groovy.vmplugin.v7.Java7’ error message, it indicates that the JVM (Java Virtual Machine) has found the class during compile time but not during runtime. This disparity usually happens when the class has been compiled successfully, but encountered issues during initialization, for instance:
- Exceptions thrown at static initializers or constructors
- JVM limitations related to size or memory resulting in unsuccessful initialization
- Anomalies in the physical existence, traceability, or access levels for the requested .class file
To remedy such scenario viz-a-viz the ‘org.codehaus.groovy.vmplugin.v7.Java7’ error:
- Revisit any modifications made in your build and deployment process that could have disrupted the bytecode allocation for the respective Groovy Plugin.
- Delve into the potential changes associated with environment variables, most importantly CLASSPATH and PATH.
- Consider upgrading or downgrading Groovy depending on the precise compatibility prospects tied to your application.
While handling errors like NoClassDefFoundError, it becomes essential to be methodical with your debugging steps. Remember, every detail counts, starting from checking your basic syntax to understanding the logistics of your complete development pipeline.
Lastly, recall renowned software engineer Linus Torvalds’ thoughts on debugging: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”source. Hence, systematic approach coupled with simplified code can help you grapple with comprehensive errors like Java.Lang.NoClassDefFoundError efficiently.