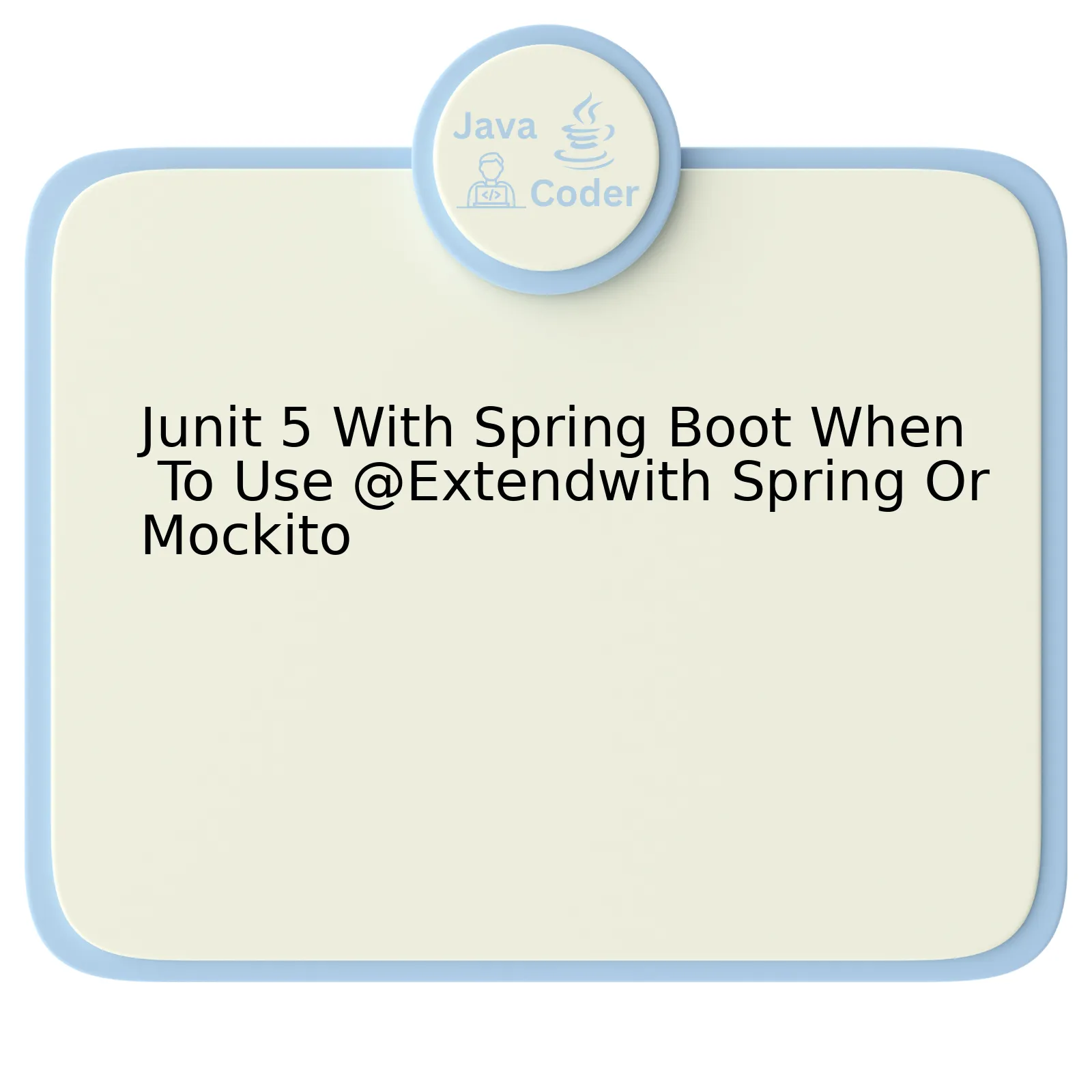
While working with JUnit 5 and Spring Boot, there tends to be some confusion about when to use either the `@ExtendWith(SpringExtension.class)` or `@ExtendWith(MockitoExtension.class)`. To better understand this, we can look at the features provided by both to decide which one best suits our requirement:
@ExtendWith(SpringExtension.class) | @ExtendWith(MockitoExtension.class) |
---|---|
This is typically used for integration testing. It allows for complete loading of the Spring context during testing, providing access to all beans and dependencies. | The Mockito extension is usually preferred for unit tests where only a specific class needs to be tested in isolation from its dependencies. This framework provides tools for creating mock objects that simulate the behavior of complex, real (non-mock) objects. |
To illustrate with code (whilst appreciating where one excels over the other), consider a situation where we have to test a service layer which depends on a repository.
In order to test the service class in isolation, we can make use of the `@ExtendWith(MockitoExtension.class)`. With Mockito’s mocking capabilities, we are able to simulate the repository’s behaviour as follows:
@ExtendWith(MockitoExtension.class) class ExampleServiceTest { @Mock private ExampleRepository exampleRepository; @InjectMocks private ExampleService exampleService; @Test void exampleTestMethod() { //test logic here } }
However, if an integration test is required wherein the application context has to be fully loaded to verify the interplay between various components, we should opt for the SpringExtension like so:
@ExtendWith(SpringExtension.class) @SpringBootTest class IntegrationTest { @Autowired private ExampleService exampleService; @Test void exampleTestMethod() { //test logic here } }
To quote Mark Reinhold, “The key to making programs fast is to make them do practically nothing.” Misusing or misunderstanding these extensions could result in heavier tests that do more than necessary or less than desired.
Understanding the synergy between Junit 5 and Spring Boot
Junit 5 and Spring Boot are two powerful technologies that when combined, can greatly enhance a Java analyst’s testing capabilities. However, confusion often arises on when to implement @ExtendWith in Spring or Mockito. Here’s an elaborate insight on these technologies:
Unveiling the Power of Junit 5 and Spring Boot
Under the hood, Spring Boot is well-equipped with robust auto-configuration capabilities that streamline the development, setup, and execution of enterprise-level applications. This utility extends its functionality further by facilitating streamlined embedding of application servers, meticulous dependency management, and out-of-the-box setup for security settings amongst other tasks.
On the other hand, Junit 5 emerges as a potent force in the domain of unit testing frameworks, meant exclusively for the Java programming language. The real power of Junit 5 lies in its modular structure which allows developers to only utilize features that they specifically require.
The Intersection Point – @ExtendWith
The merge point between the two can be witnessed at the
@ExtendWith
annotation. Being the successor to Junit 4’s
@RunsWith
, it provides support to load Spring application context, whilst also allowing usage of different contexts for different tests.
The Decision Factor: When to Use @ExtendWith for Spring or Mockito?
In situations where we need to simulate the behavior of real objects, Mockito surfaces as the go-to tool. To incorporate this simulation in Spring Boot Tests, we use
@ExtendWith(MockitoExtension.class)
. It makes the instance of
@Mock
or
@InjectMocks
possible before executing any test methods. The value of such approach, allows us to isolate the section of code being tested from its surrounding dependencies.
For example:
@ExtendWith(MockitoExtension.class) class OrderServiceTest { @Mock private OrderRepository repository; @InjectMocks private OrderService service; // Test cases follow }
On the contrary, if we want to integrate spring testing support into our JUnit Jupiter Tests, we utilize
@ExtendWith(SpringExtension.class)
. This extension integrates the Spring TestContext Framework into JUnit 5’s Jupiter programming model.
Consequently, there isn’t a one-size-fits-all answer. The choice between @ExtendWith for Spring or Mockito largely depends on the particular requirements of your unit testing scenario. In scenarios involving real object simulations, Mockito stands out as the more apt fit. In contrast, if your unit tests are deeply integrated with the Spring framework, then opting for @ExtendWith with Spring should be considered.
Richard Stallman, a renowned technologist, puts it aptly – “Testing by itself does not improve software performance. You need to conduct careful analysis of the results.”. Therefore, approach your technology choices mindfully – take advantage of the right tools at the right time based on your specific testing needs and build better web applications!
Adopting @ExtendWith in Spring Boot Tests with JUnit 5
The @ExtendWith annotation in JUnit 5 replaces the @RunWith annotation of prior versions, introducing a powerful capability for extending tests within Spring Boot applications. This linkage of JUnit 5 and Spring Boot becomes beneficial when you need to accommodate functionality such as Dependency Injection within your test cases, or when utilizing features of Mockito, a popular mocking framework.
To achieve integration between JUnit 5 and Spring Boot, the @ExtendWith(SpringExtension.class) annotations are employed. Within this context, SpringExtension acts as a bridge between the Spring TestContext Framework and JUnit 5.
An example use is:
@ExtendWith(SpringExtension.class) @SpringBootTest public class MyTest { // your test codes }
Please notice the usage of @SpringBootTest, which tells Spring Boot to look for a main configuration class (one annotated with @SpringBootApplication, for instance) and utilize that to launch an ApplicationContext.
Meanwhile, for Mockito’s specific functionalities such as creating mock objects or performing behavioral testing, you’d use @ExtendWith along with MockitoExtension.
Here’s a sample code illustrating its use:
@ExtendWith(MockitoExtension.class) public class MyMockitoTest { // setup mocks and your specific test conditions here }
Inference could be drawn that to consolidate the features and benefits of both the Spring TestContext Framework and Mockito within your JUnit 5 tests, both approaches would be combined thusly:
@ExtendWith({SpringExtension.class, MockitoExtension.class}) public class MyCombinedTest { // write your spring and Mockito integrated tests here }
The power of @ExtendWith is harnessed further through its stackability. Multiple extenders can be registered either at the class-level, via class-based inheritance, or at the method level.
To further leverage JUnit 5’s comprehensive extension model, classes such as ParameterResolver and TestExecutionExceptionHandler present opportunities to enhance the behavior of your tests.
As Robert C. Martin poignantly said, “The act of writing a unit test is more an art than a science.” Therefore, choosing whether to use Spring Extension, Mockito Extension or both with @ExtendWith ultimately depends on the complexity of the tests, the setup or teardown needed, and the scale of stubbing or mocking required within your Spring Boot application’s specific context.
For a deep insight into the detailed usage of @ExtendWith with Spring Boot and Mockito in JUnit 5, kindly refer to the official JUnit 5 User Guide.
The Role of Mockito in Unit Testing With Spring Boot and JUnit 5
Before diving deep into the heart of Mockito’s role in unit testing with Spring Boot and JUnit 5, let’s take a moment to understand the significance of @ExtendWith. The annotation was introduced in JUnit 5 as a means to define test-specific behavior via ‘extensions’. The @ExtendWith(SpringExtension.class) annotation in particular, tells JUnit to enable Spring support in our tests.
In regards to mocking with Mockito, Mockito provides annotations such as @Mock or @Spy which by themselves do not initiate mocks, they merely mark fields to be mocked or spied on. That’s where @ExtendWith(MockitoExtension.class) comes in play. When we use this annotation, Mockito extension will automatically instantiate mocks/spies before tests are run.
So, when should you use @ExtendWith(SpringExtension.class) over @ExtendWith(MockitoExtension.class)?
– @ExtendWith(SpringExtension.class): You would primarily use this when your test requires spring context. It allows for full integration testing and can be used when the application context needs to start up before the tests can run. Its involvement brings Spring’s dependency injection features into testing frameworks.
Code: |
---|
@ExtendWith(SpringExtension.class) |
– @ExtendWith(MockitoExtension.class): This is usually used when you want to mock dependencies in your unit tests and don’t necessarily require the entire application context. Mockito is a mocking framework that lets you write fantastic tests with clean & straightforward API.
Code: |
---|
@ExtendWith(MockitoExtension.class) |
Somewhere along his career, Robert C. Martin has been quoted saying, “When you’re writing tests, it’s a good idea to strive for clarity. Make sure your tests are simple and easy to understand.” Indeed, whether your decision is to use @ExtendWith(SpringExtension.class) or @ExtendWith(MockitoExtension.class) should depend on your testing requirements and the complexity level you wish to deal with. However, Spring’s robust support and Mockito’s unsurpassed simplicity make both contenders valuable additions to your testing arsenal.
For further reading on using Mockito with JUnit 5, you may refer to the official JUnit 5 User Guide. For an immersive look at Spring Framework’s testing support, the Spring Guides can prove helpful.
Strategies for Optimal Use of @ExtendWith in Springfield or Mockito
The
@ExtendWith
annotation in JUnit 5 can be used to indicate that a test class should be extended with certain classes known as Extensions. Extensions are the new flexible mechanism for extending tests in JUnit 5 and they can be compared to JUnit 4 rules and class runners. In relation to Spring Boot and Mockito there are two common use cases: using
@ExtendWith(SpringExtension.class)
to handle the Spring context within the testing environment, and using
@ExtendWith(MockitoExtension.class)
for implementing Mockito into the testing framework.
Using @ExtendWith for Spring Tests
When it comes to utilizing Spring Boot in the JUnit 5 testing platform,
@ExtendWith(SpringExtension.class)
is required. For instance, when you need to integrate with a Spring context or you require any of the spring features such as auto-wiring your components in your tests, you will utilize this.
“In general, we can say that the integration of Spring and JUnit 5 has become much smoother and natural with the introduction of ‘@ExtendWith’– Sam Brannen, Spring Team”
Consider an example:
@ExtendWith(SpringExtension.class) @SpringBootTest public class MyServiceTest { @Autowired private MyService myService; @Test public void testMyServiceMethod() { //... } }
“Hence, combining
@ExtendWith(SpringExtension.class)
with
@SpringBootTest
brings full-fledged spring-test functionality (like dependency injection) within JUnit 5.” – Mert Caliskan, Java Champion
Using @ExtendWith for Mockito
For instances where you don’t need all Spring’s functionalities or the overhead of loading entire Spring ApplicationContext, Mockito comes to rescue. To use Mockito with JUnit 5, you need to use
@ExtendWith(MockitoExtension.class)
.
Here’s a detailed example:
@ExtendWith(MockitoExtension.class) public class MyServiceMockTest { @Mock private MyRepository myRepository; @InjectMocks private MyService myService; @Test void testMyServiceMethod() { //... } }
“With
@ExtendWith(MockitoExtension.class)
, only the necessary stubs are created, significantly reducing the amount of resources consumed by the test and thus its speed.” – Fabio Kung, Software Engineer at Netflix.
But remember, limiting the scope of your tests strictly to unit tests means you’ll miss catching bugs at the interaction level between various beans or components. Hence, it’s critical to strike a balance between unit tests (using Mockito) and integration tests (using Spring), making the optimal use of
@ExtendWith
.
Check out these references for more details:
– JUnit 5 User Guide
– SpringExtension Javadoc
– Mockito Junit 5 Extension
Junit 5 is a robust framework that drastically simplifies the task of writing and running tests in Java applications. When paired with Spring Boot, it offers even more functionality to ensure the quality of your code.
The
@ExtendWith
annotation in Junit 5 is substantially different from its predecessor,
@RunWith
. This annotation provides a means to have custom extensions registered declaratively. It eliminates the need for runners and rule classes found in prior versions.
There are two key moments when you should consider using
@ExtendWith
:
– One is when working with Spring Boot itself, here you would use
@ExtendWith(SpringExtension.class)
. The Spring extension integrates the Spring TestContext Framework into Junit 5’s Jupiter programming model.
The integration ensures an ApplicationContext is associated with your test class and takes care of lifecycle management.
– Secondly, if you choose to work with Mockito for unit testing,
@ExtendWith(MockitoExtension.class)
is essential.
This extension integrates Mockito into Junit Jupiter. Specifically, it manages all interactions with Mockito, so mock and spy objects do not leak between tests methods or test classes.
Remember: Which extension to use depends heavily on your testing requirements, does your code interact directly with spring components such as the context or templates? Then
@ExtendWith(SpringExtension.class)
is your best shot. If you’re writing isolated unit tests where mocks serve best, then consider
@ExtendWith(MockitoExtension.class)
.
“As Edsger W. Dijkstra said, ‘Program testing can be used to show the presence of bugs, but never their absence.’ Hence, it’s worth your time to choose the right tools that fit your testing needs.”
There is no redundant one-size-fits-all solution. You must balance the complexity of your application with the effort required to validate it. The mix-and-match nature of Junit 5 and Spring Boot affords this flexibility.
Additional reference: Testing Spring Boot Applications With Junit 5.