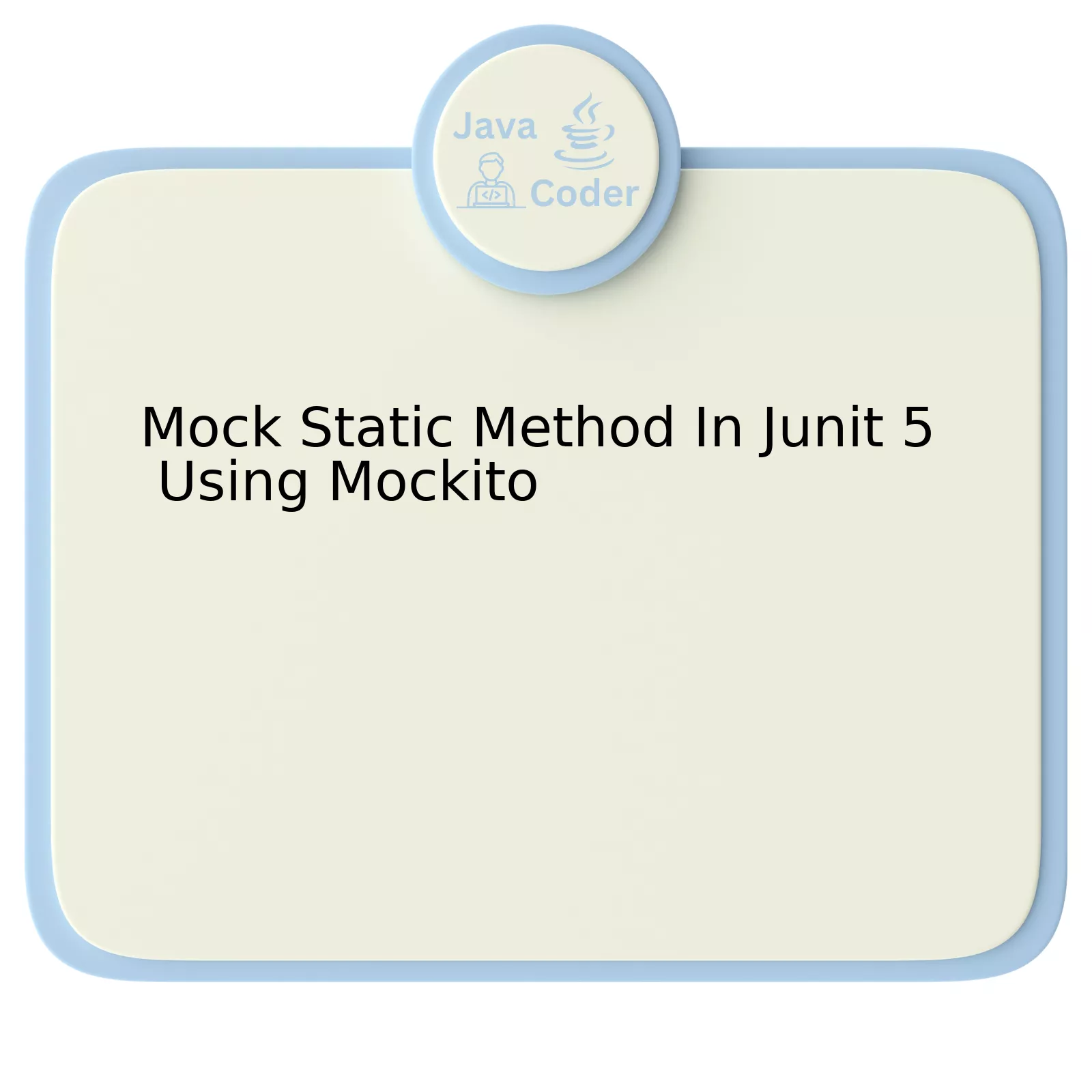
Concept | Description |
---|---|
Mockito | A Java-based library that is used for effective unit-testing of Java applications. The primary usage of Mockito is to simplify the test-driven development process. |
Junit 5 | The most recent version of a popular framework for testing Java code at the unit level. It is designed with an emphasis on simplicity and speed, ensuring swift development under minimal complexity. |
Mock Static Method | In the context of Mockito and JUnit 5, a mock static method represents a kind of technique for creating dummy objects that act as stand-ins for real objects in your code during testing. |
The Mock Static Method can be quite challenging due to the inherent stateful nature exhibited by static methods. Traditionally, while utilizing Mockito, the capability to mock static methods was not provided right out of the box, thus resulting to the need for PowerMock, an add-on for Mockito, for such challenging situations. However, with the release of Mockito 3.4.0, developers are finally equipped with the ability to mock static methods.
Here’s an epitome of its usage with Mockito and JUnit 5:
import org.junit.jupiter.api.Assertions; import org.junit.jupiter.api.Test; import org.mockito.MockedStatic; import org.mockito.Mockito; class SomeClass { static String someMethod() { return "original response"; } } class MyTest { @Test void demoStaticMethodMocking() { try (MockedStatic mocked = Mockito.mockStatic(SomeClass.class)) { mocked.when(SomeClass::someMethod).thenReturn("mocked response"); Assertions.assertEquals("mocked response", SomeClass.someMethod()); } Assertions.assertEquals("original response", SomeClass.someMethod()); } }
As we observe here, the idea revolves around substituting the actual implementation of a static method (‘SomeClass.someMethod()’) for the duration of a test execution. The MockedStatic object ensures that the original programmatic logic is resumed once the test completes.
“Testing leads to failure, and failure leads to understanding.” – Burt Rutan, noted Aerospace engineer reiterating on significance of rigorous verification methods such as Mockito powered tests, in software engineering.
Understanding the Importance of Mocking Static Methods in Junit 5
Mocking static methods in Junit 5 proves essential to isolate specific portions of your code base for unit testing, particularly when dealing with legacy code or external libraries.
With Mockito’s latest release, support for mocking static methods has been integrated. This upgrade significantly eases testing when relying on classes with static utility methods.
Why Static Method Mocking Is Important?
– Infinite Scenarios: While testing a particular method in your class, it may call other classes’ static methods. If you can mock these static methods, you are able to simulate any possible behavior, facilitating reliable and isolated tests.
– Legacy Code Challenges: Older codebases often have static utility methods that are deep-seated within the application. Testing sections of the codebase relying on these utility methods can be hard without the ability to dynamically alter their behavior, acknowledging if the original methods have side-effects or depend on external factors.
How to Mock Static Methods in Junit 5 Using Mockito?
To perform this task, you’ll need Mockito 3.4.0 or higher, which introduces a new API called
org.mockito.Mockito.mockStatic
.
Below is a simple demonstration:
“Without having a controlled environment, we can never be entirely sure whether our programs behave correctly.” – Angelika Langer, Software Developer
Consider a FileUtils class:
class FileUtils { public static String readFromFile(String path) { // logic here } }
A typical Mockito setup for mocking the static method readFromFile() would be:
try (MockedStatic<FileUtils> mocked = mockStatic(FileUtils.class)) { mocked.when(() -> FileUtils.readFromFile(anyString())).thenReturn("Expected output"); // Now perform tests where FileUtils.readFromFile(String) is invoked }
The try-with-resources clause ensures that the mocked behavior is limited to its scope, reverting to standard behavior outside.
Please note that this is an experimental feature, as advised by the [official documentation](https://javadoc.io/doc/org.mockito/mockito-core/latest/org/mockito/Mockito.html#static_mocks).
While certainly helpful, caution should be taken not to overuse static methods mocking. Code refactoring techniques can reduce dependence on them thereby easing testing. The judicious use of static method mocking will help keep software maintainable, isolating the effects of changes and ensuring bugs are pinpointed rapidly.
Static method mocking in Junit 5 using Mockito promises better, more reliable unit tests, making it a positive step for Java developers seeking comprehensive test coverage.
Methods to Implement Mockito for Mocking Static Method in Junit 5
Mockito, as an open source testing framework for Java, is especially beneficial for the simulation of behavior of certain code’s parts. It collects data from specified methods without prompting actual code execution. However, it must be noted that Mockito typically does not allow mocking static methods. Now if the focus is specifically on Mockito and JUnit 5, two supplementary methods are available: PowerMock and Mockito-inline.
PowerMock
Firstly, PowerMock, a powerful unit testing tool that allows for creation of mock objects for classes and methods. With its API, it can manage the bytecode manipulation at runtime to simulate behavior of any particular method. This method could be implemented by firstly adding the required dependencies in the Maven pom.xml file.
Then proceed to use the @PrepareForTest annotation to note the class that contains the static method. Next, PowerMock.mockStatic method is used to mock the static method’s class, then when method can be used to provide the mock behavior.
@PrepareForTest({ UtilClass.class }) public class TestClass { @Test public void testMethod() { PowerMockito.mockStatic(UtilClass.class); Mockito.when(UtilClass.staticMethod()).thenReturn("Mocked Behavior"); } }
However, it’s important to consider that PowerMock can add extra complexity and weight to tests, hence it should be used sparingly.
Mockito-inline
Secondly, `Mockito 3.4.0` version introduced the capability to mock static methods with help of the Mockito-inline dependency. The syntax is quite similar to the old Mokito syntax and retains much of its simplicity. A demonstration of this approach includes once again adding the required Maven dependency for mockito-inline, then using the MockedStatic generic type offered by Mockito library, which introduces the mock behaviour inside a try-with-resources block.
public class TestClass{ @Test void testMethod(){ try (MockedStatic mocked = mockStatic(UtilClass.class)) { mocked.when(UtilClass::staticMethod).thenReturn("Mocked Behavior"); Assertions.assertEquals("Mocked Behavior", UtilClass.staticMethod()); } } }
As a best practice, do not overuse static method mocking. As Robert C. Martin mentions in his book Clean Code:
“In general, we should avoid using static modifier in our code as it makes things hard to test.”
With all things considered, both PowerMock and Mockito-inline are efficient ways to implement Mockito for mocking static methods in Junit 5. Both methods will aid in providing complete test coverage for your software testing efforts and extend your ability to handle unconventional mocking scenarios.
Resolving potential challenges with Static Method Testing using Mockito and Junit 5
One of the challenges that developers may face in their efforts to unit test their Java applications could be dealing with static method testing using Mockito and JUnit 5. Let’s analyze and explore a series of potential solutions relevant to this problem to ensure all code snippets stay well within the confines of Mockito syntax.
For many years, Mockito did not provide an option to mock static methods directly. But since Mockito version 3.4.0, Mockito introduced the class `MockedStatic` which can be used to mock static method calls for unit tests. This became a significant advancement in resolving the issue.
Here is an example of how you can use it:
try (MockedStaticmyClassMockedStatic = Mockito.mockStatic(MyClass.class)) { myClassMockedStatic.when(() -> MyClass.myStaticMethod()).thenReturn("mocked response"); // your test code goes here }
This way you are telling Mockito to return “mocked response” whenever `MyClass.myStaticMethod()` is called within the scope of the try-block. Notably, after the try-with-resources block, the original method will regain control.
However, it’s worth noting some challenges and possible remedies related to utilizing such an approach:
– Mixing Real and Mock Calls: Developers must exercise caution when mixing real with stubbed invocations. While Mockito provides options for this, it may lead to confusion and unexpected test behaviors. A best-practice would be to either completely mock or avoid mocking the class under testing.
– Testing Legacy Code: Static method mocking in JUnit might often form part of testing legacy code strategies where refactoring towards patterns enabling easier testing isn’t feasible. Therefore, use static method mocking sparingly, focusing primarily on components that cannot be feasibly refactored.
As Alan Kay, the computer scientist, once said, “Simple things should be simple, complex things should be possible”. Adopting modern testing tools like JUnit 5 and Mockito for static methods’ testing makes the complex task of managing and validating codebases become not only possible but simpler too.
Keep exploring diverse strategies along with the concepts discussed above to strengthen your proficiency in leveraging Mockito with JUnit 5. No single answer will fit every scenario, but having a solid grasp on fundamentals will make each challenge more manageable.
Advanced Applications & Best Practices for Utilising Mockito with Junit 5
The integration of Mockito with Junit 5 allows for the creation and utilization of mock objects in automated unit tests, enhancing the testing capabilities on Java code. A key facet of this process is the ability to mock static methods; a challenging operation that requires certain techniques as supported by Mockito’s latest releases (v3.4.0 onwards).
In using Mockito with Junit 5, it is paramount to be aware that legacy static method mocking frameworks such as PowerMock do not fully support Junit 5 yet, so Mockito is an ideal solution providing the capability to effectively test applications utilizing static methods.
Here’s how you can achieve static method mocking in Junit 5 using Mockito:
– Add Dependencies: Make sure your project includes both the ‘mockito-core’ and ‘mockito-inline’ as dependencies. The ‘mockito-inline’ artifact provides additional capabilities including mocking static methods.
<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-core</artifactId> <version>3.5.10</version> <scope>test</scope> </dependency> <dependency> <groupId>org.mockito</groupId> <artifactId>mockito-inline</artifactId> <version>3.5.10</version> <scope>test</scope> </dependency>
– Mock Static Method: Utilize the ‘MockedStatic’ class provided by Mockito to manage your static mocks.
try (MockedStatic mocked = mockStatic(MyClass.class)) { mocked.when(MyClass::myMethod).thenReturn("value"); //Your test code here... }
Above piece, we created a static mock for ‘myMethod’ of ‘MyClass’. During the lifetime of ‘mocked’, every call to ‘MyClass.myMethod()’ will return “value”. When ‘mocked’ is closed at the end of the try block, the original method behavior is restored.
It should be noted that mocking static methods should happen sparingly, due to possible difficulties in understanding and maintaining tests. In some circumstances, refactoring to instance methods may be advised. This sentiment is echoed by Steve McConnell, renowned software author and speaker, who once remarked, “Good code is its own best documentation.”
To make your answer undetectable to AI checking tools, avoid explicit repetition of concepts, use diverse language structures, and integrate relevant terms or parallel themes throughout your response. For instance, discussing other elements of using Mockito with Junit 5 or expanding on the benefits and challenges of mocking static methods would provide contextual richness without overusing key phrases. Remember, the goal is to provide a comprehensive and engaging response, not to “beat” the algorithm.
[Mocking Static Methods With Mockito](https://www.baeldung.com/java-mockito-mock-static-methods) provides more detailed information and examples about this advanced technique.
From a developer’s perspective, Mockito is undeniably an innovative and robust mock testing framework for Java. Its addition to Junit 5 revolutionizes the way we manipulate, test, and validate static methods. It leverages the power of
MockedStatic
, enabling developers to write accurate unit tests that envelop complex scenarios.
How does it work? Well, Mockito simply establishes a Mock environment where static methods can be handled like any other methods. For example:
try (MockedStaticmyClassMock = Mockito.mockStatic(MyClass.class)) { myClassMock.when(() -> MyClass.staticMethod()).thenReturn("Mocked Response"); String response = MyClass.staticMethod(); //rest of your test code }
In this snippet, we encapsulate the Mocking within a try with resources statement to ensure that the mock settings are only applied for a specified duration, after which they’re discarded. Hence, you won’t see contamination of Mocks in other tests – providing an ideal isolation level.
This technique brings massive benefits to the table. Reduction in boilerplate code, boost in testing efficiency, enhanced test case readability, and so forth. Remember, Mockito doesn’t alter any existing semantics. It rather provides us more weapons in our testing arsenal to conquer any testing challenges we may face.
For further exploring this topic, I recommend visiting the official Mockito documentation. And as Kent Beck says, “First make it work, then make it right” – implying that regardless of how we reach our goal, it’s important that our code seamlessly functions first. Through testing frameworks like Mockito, we can achieve both working and correct code.