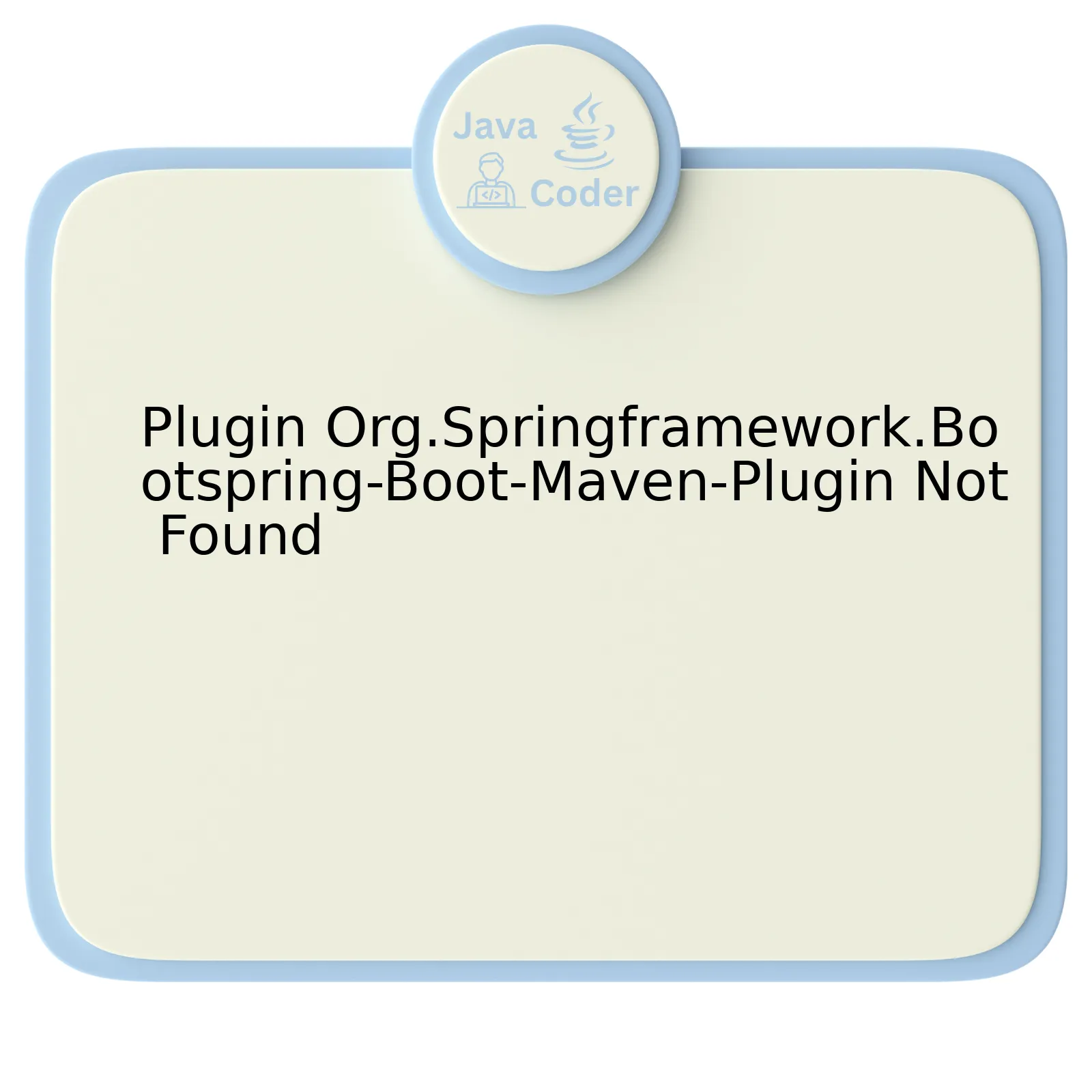
Issue | Possible Causes | Solutions |
---|---|---|
Plugin org.springframework.boot:spring-boot-maven-plugin not found |
|
|
(connectivity)[https://docs.spring.io/spring-boot/docs/current/reference/html/getting-started.html#getting-started-first-application]
This problem may occur if you encounter network issues that prevent Maven from communicating with the necessary repositories to download the plugin, or the settings.xml in your .m2 directory might be misconfigured.
Also, inaccurate Project Object Model (POM) file configurations is another common cause – probably an issue of specifying the wrong version of the Spring Boot parent or failing to specify it at all.
To fix this:
– First, ensure that your Internet connection is working well as Maven needs to access its repositories to download the plugin. If there’s a breakage or issue with your networking, this could be the main reason why the plugin can’t be found.
(settings.xml)[https://maven.apache.org/settings.html]
Second, verify your settings.xml file in your .m2 repository. Ensure that the file gets correctly configured with the proper repositories. A misconfiguration in this file could cut off Maven from accessing the necessary repositories to find the spring-boot-maven-plugin.
(POM)[https://maven.apache.org/pom.html]
Finally, check and rectify your POM file. If there’s a typographical error or some config syntax issues within the POM file, it could lead to this problem. Make sure that it’s accurately configured, particularly if you are inheriting from the spring-boot-starter-parent or importing the spring-boot-dependency. Specific attention to the accurate versions helps prevent such problems.
In words of Robert C. Martin, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.”
Understanding the Org.Springframework.Boot-Spring-Boot-Maven-Plugin Issue
The
org.springframework.boot
:
spring-boot-maven-plugin
is a quite prominent and an invaluable tool in the Java programming world, particularly for projects built with Maven and Spring Boot. This plugin plays a crucial role due to its unique capabilities to package executable JAR or WAR archives, perform an Opinionated ‘spring-boot:run’ Mojos and manage various dependencies.
Functionality | Description |
---|---|
Manage Dependencies | The plugin helps in attenuating dependencies management issues, relieving developers from the intricate task of controlling every individual version of dependency they use. |
Package Executable Archives | This functionality allows for creating standalone Java applications that can be run from the command line, without missing any additional jars on the classpath. |
Opinionated ‘spring-boot:run’ Mojos | It includes an opinionated set of ‘Mojos’, paving the way for straightforward configuration experience. |
In a typical project build, if you observe the issue “Plugin
org.springframework.boot:spring-boot-maven-plugin
not found”, it’s usually due to one or more of these primary reasons:
Misconfiguration in the Project POM File
This might be due to incorrect or incomplete configuration of the plugin inside your project’s Maven ‘pom.xml’ file. It’s essential to ensure that the declaration for this plugin contains necessary elements and is properly nested within the ‘plugins’ tag under the ‘build’ section. Markus Eisele, a well-known Java developer, has rightly mentioned, “Maven is not a magic wand – it’s only as good as your pom.xml.”
An example of correct configuration would look like:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.5.0</version>
</plugin>
</plugins>
</build>
Lack of Proper Dependency Resolution
Occasionally, problems with resolving the plugin can occur when using Maven. These could be related to network connectivity issues preventing Maven from reaching the necessary repositories, or it could stem from local repository corruption. Running
mvn clean install
should normally resolve these issues.
Outdated Plugin Version
An out-dated version of the plugin can be another potential cause. A simple update to a newer version which is compatible with the current Spring Boot version used in your project could possibly solve the problem.
The official documentation of the Spring Boot Maven Plugin provides an elaborative guide on troubleshooting issues specifics to its workings. Employing these guidelines, we can rectify common issues that arise concerning the said problem, thereby optimizing productivity in working with Spring Boot and Maven.
Potential Causes for Plugin Not Found Error in Spring Boot Maven
This enigmatic error "Plugin org.springframework.boot:spring-boot-maven-plugin not found" when working on a Spring Boot project with Maven, is a matter that many Java developers have to deal with. Understanding the ramifications requires an insight into some of the potential causes.
Possible Cause #1: Incorrect Syntax or Typos
A very common occurrence which often gets overlooked is incorrect syntax or subtle typographical errors in your
pom.xml
file. This can cause Maven to misinterpret the requested plugin. Therefore, ensure that you've entered the correct artifact name -
spring-boot-maven-plugin
and the exact group ID -
org.springframework.boot
.
Possible Cause #2: Network Issues
In certain instances, network issues such as firewalls, unstable internet connections, or proxy configurations might prevent Maven from accessing the central repository, preventing it from finding and downloading the necessary plugins. Ensuring stable network connectivity is hence essential.
Possible Cause #3: Local Repository Corruption
Corruption within your local "
.m2
" repository is another significant aspect to consider. Deleted or corrupted files within this repository can cause Maven to fail to locate the indicated plugin. A great way to fix this is by deleting the potentially corrupt local repository, as Maven will generate a new one during the next build process.
Possible Cause #4: Outdated Maven or Spring Boot Version
At times, having outdated versions of Maven or Spring Boot can also lead to this issue. Incompatibilities between versions may arise so it is always recommended to regularly update to the latest version to mitigate these errors.
Possible Causes |
---|
Incorrect Syntax or Typos |
Network Issues |
Local Repository Corruption |
Outdated Maven or Spring Boot Version |
Consequently, looking at the possible causes and understanding how to address them provides a fitting solution to the issue. As renowned programmer Grace Hopper said, "The most damaging phrase in the language is ‘we’ve always done it this way’." This can serve as a good mindset while addressing these issues, approaching every problem from a fresh perspective, irrespective of the issue being encountered.
Ways to Resolve the Spring Boot Maven Plugin Not Found Problem
The problem of "Plugin org.springframework.boot:spring-boot-maven-plugin not found" is common amongst developers, but there are reliable ways to address it. While diving into this issue, we need to discuss how the Spring Boot Maven Plugin works, what might be causing the problem and some solutions that can be taken to resolve it.
The Importance of Spring Boot Maven:
The Spring Boot Maven Plugin is a critical tool for any Java developer using the Spring Framework. It provides first-class support to the Spring Boot applications in Maven Environment. The plugin allows developers to build standalone Spring applications that you can “just run”.
Causes of The Problem:
There could be different reasons why you are running into the "Plugin org.springframework.boot:spring-boot-maven-plugin not found" problem.
- One common cause is an incorrect project setup or configuration.
- Another potential reason could be because of your Maven settings or connectivity issues preventing you from accessing Maven central repository.
- Dependency problems could also trigger this issue. Incorrectly defined pom.xml file can equally contribute to this problem
Solutions:
If you encounter this problem, here are some steps you might want to consider:
Firstly, ensure that your pom.xml file is correctly set. Your pom.xml should include the spring boot starter parent and the correct format of spring-boot-maven-plugin as shown below:
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.4.RELEASE</version> <relativePath/> </parent> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
Secondly, check the network connection and proxy settings. Sometimes, a poor network connection can cause Maven to fail in fetching necessary plugins or dependencies, which may appear as if they are not found. A similar problem can occur when behind a corporate proxy, so ensure your Maven settings include the correct proxy configuration.
Lastly, make sure your local maven repository is not corrupted. It's recommended to perform a clean install to repopulate your local repository.
As Linus Torvalds once said,"
Software is like entropy: It is difficult to grasp, weighs nothing, and obeys the Second Law of Thermodynamics; i.e., it always increases." So does the complexity of troubleshooting, yet the solution can often be simple tweaks with thoughtful consideration.
References:
You can visit these references for more understanding and possible solutions to this issue:
Baeldung - Resolve Spring Boot Issues
Stackoverflow - Plugin org.springframework...
Exploring Alternate Methods and Workarounds When Faced with Plugin Issues
Facing the issue of not finding the Spring Boot Maven Plugin,
org.springframework.boot:spring-boot-maven-plugin
, suggests a potential problem in your project configuration or the way Maven is set up. Here is an analytic exploration of possible alternatives and workarounds to address this predicament:
Revisit Project's pom.xml File
A simple yet often overlooked aspect is the project’s pom.xml file. One must ensure that the correct coordinates for the plugin are specified. The correct identifier should be
org.springframework.boot:spring-boot-maven-plugin
.
The following represents what a standard incorporation of the Spring Boot Maven Plugin looks like:
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
Ensuring this resides correctly within your pom.xml file might aid in rectifying the 'Plugin Not Found' anomaly.
Cross-verify Maven Version and Setup
Ensure that the Maven version you employ supports the Spring Boot Maven Plugin. For instance, Spring Boot 2.3.0.RELEASE requires Maven 3.3 or later.
Additionally, validate that Maven has been configured accurately, both globally and for your specific user. It includes verifying the Maven Home, Java version, and other environment settings.
Incorporate Explicit Plugin Version in Project Pom
Notwithstanding Maven's capability to fetch the latest available versions of plugins from the repository, explicitly specifying the plugin version in your project's pom.xml file can alleviate potential version-related conflicts or miscues. That might be the solution if the plugin version that Maven is attempting to retrieve does not exist.
This could look something like this:
<plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>2.3.0.RELEASE</version> </plugin>
"Microservices require a lot of error handling as distributed systems have lots of things that can go wrong at any moment". This statement by Henn Idan implies that like with microservices, Maven plugins would need constant checking and troubleshooting too.
Maven Repo Connectivity
Are there any connectivity or networking issues preventing Maven from accessing the plugin from the remote repository? Firewall constraints or network proxies may limit access to the internet, causing plugin retrieval problems.
Remember, Maven retrieves plugins and dependencies over the internet from repositories, like the Central Maven Repository. Therefore, ensuring unhindered internet access for Maven is indeed crucial. You may also want to investigate whether temporary network glitches perturbed Maven's download process.
Delete and Refresh Local Repository
Lastly, corrupted data in your local repository could lead to an inability to find plugins. In such a scenario, it may be beneficial to delete the contents of your local .m2 repository and enforce a fresh download of all necessary artifacts upon your next build.
To familiarize yourself further with plugin execution issues, it's highly advised to check out the "Introduction to Plugin Diagnostics" offered by official Maven Guide.
Deep Diving into the Issue: Plugin Org.Springframework.Bootspring-Boot-Maven-Plugin Not Found
The commonly encountered error of
Plugin org.springframework.boot:spring-boot-maven-plugin not found
, triggers when the Maven project fails to locate the vital Spring Boot Maven Plugin. This plugin is instrumental for packaging executable jars or war archives. Inadequate configurations in the
pom.xml
file often lead to this issue, and demands a thoughtful debugging strategy.
Causes | Solutions |
---|---|
Incorrect version of
spring-boot-starter-parent in pom.xml |
Ensure that the correct Spring Boot starter parent version is specified. |
Inadequately configured corporate firewall/proxy server | Contact network administration or configure proxy settings in Maven's
settings.xml |
.m2 directory corruption |
Remove the impacted artifacts from the local
.m2 repository. |
Online dependency repositories are down/unavailable | Check status of dependency repositories or try again later. |
While analyzing the problem at hand, it’s worth bearing in mind the immortal words of Martin Fowler - "Any fool can write code that a computer can understand. Good programmers write code that humans can understand." Approaching it with clear, systematic debugging steps can make the process more manageable.
A precise way to resolve the error is by verifying the alignment of the Spring Boot application's
pom.xml
content with the official Spring Boot documentation. Configuring online dependency repositories correctly also plays a crucial role.
It's pivotal to note, the resolution of this error can differ based on the specific circumstances and configurations of each system. Aiming to be a good programmer, as Martin Fowler suggests, involves crafting our solutions in a tailored manner, comprehending every aspect of the problem. This mindset influences the ways we deal with errors like
Plugin org.springframework.boot:spring-boot-maven-plugin not found
adequately and effectively.
For additional insights into handling similar Maven-related issues, take a glance at some official Maven documentation and Apache Maven forums1. Here one can discover various potential solutions proposed by a vibrant community of developers confronting similar challenges.