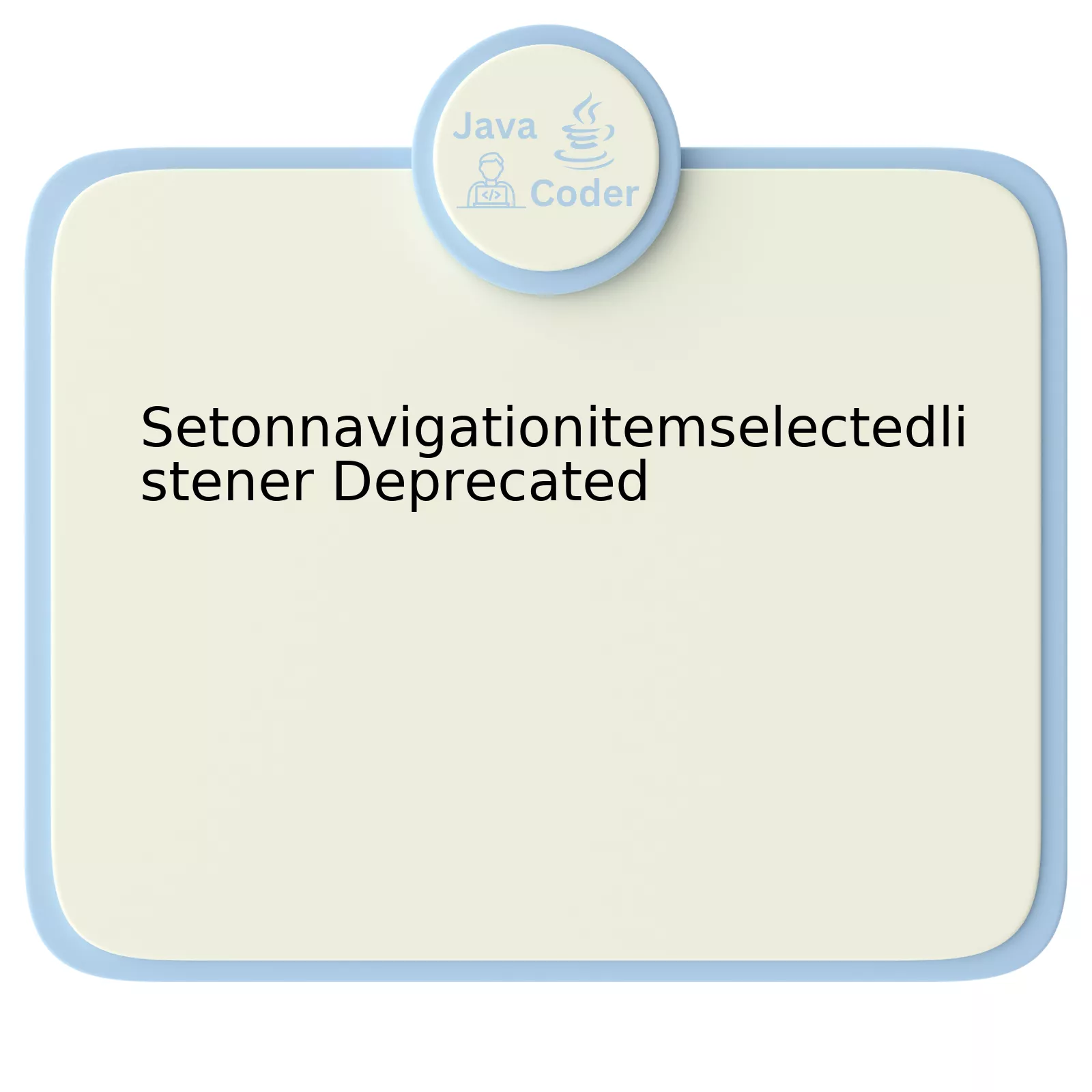
The `setOnNavigationItemSelectedListener` in Android programming has been deprecated. Earlier, it was used with `BottomNavigationView`, primarily for handling the clicks on navigation items within class. Here is an encapsulated detail which compares old method and suggested new approach.
Attribute | setOnNavigationItemSelectedListener | suggested replacement: setOnItemSelectedListener |
---|---|---|
Availability | No longer available after API version 29 (Deprecated) | Suggested to be used in newer implementations for all API levels |
Purpose | Handles item selection events | Mimics similar behavior with some additional benefits |
Compatibility | Not recommended in new developments | Has broader compatibility and optimization practices |
The newly introduced method `setOnItemSelectedListener` provides essentially the same functionality but with some additional benefits. Developers are encouraged to update their codebase to use this new method. The reason for the change can be attributed to optimization needs and improvements in conjunction with the release of new API versions.
Here’s how you might implement `setOnItemSelectedListener`:
bottomNavigationView.setOnItemSelectedListener(new NavigationBarView.OnItemSelectedListener() { @Override public boolean onNavigationItemSelected(@NonNull MenuItem item) { switch (item.getItemId()) { case R.id.action_home: // Handle the home click break; case R.id.action_settings: // Handle the settings click break; } return true; } });
As Jeff Atwood, a prominent figure in coding and co-founder of Stack Overflow, expressed, “The best way to prepare [to be a programmer] is to write programs, and to study great programs that other people have written probably no truer words can be applied when adapting to changes in a programming language.”
Understanding the Deprecation of SetOnNavigationItemSelectedListener
The deprecation of `SetOnNavigationItemSelectedListener` is a major topic in Java development, particularly for those working with the Android platform. Here’s a thorough look at what this deprecation means and how to handle it while keeping the specific focus on `setOnNavigationItemSelectedlistener` being deprecated.
Before delving into the understanding, let’s quickly cover what “deprecation” means in the programming world. Deprecation is a status applied to software features to indicate that they should not be used, usually because they have been replaced by newer features or functionalities.
“Deprecation is a language techiques to tell users: proceed with caution.” – Joel Spolsky, Co-founder of Stack Overflow.
In the context of Android UI development, Google introduced the Bottom Navigation View widget several years ago. Initially, the component’s navigation events were handled using the `setOnNavigationItemSelectedListener`. But, over time and with the evolution of different coding conventions and techniques, Google has decided to deprecate `setOnNavigationItemSelectedListener`. The primary reason being that there are better modern methods to handle these scenarios.
But what does this deprecation mean for you?
– It signifies that developers should divert from this method and adapt to another, more updated one.
– The method will still function as expected without causing your applications to break, but its future compatibility could be compromised, prompting developers to move away from it.
– The official replacement for `setOnNavigationItemSelectedListener` is seen to be more advanced while offering similar functionality e.g `setOnItemSelectedListener`.
Here’s an example of how to use the new method ‘setOnItemSelectedListener’:
bottomNavigationView.setOnItemSelectedListener( new OnItemSelectedListener() { public boolean onNavigationItemSelected(@NonNull MenuItem item) { // Handle item selection here return true; } } );
Link to [Official Android Documentation](https://developer.android.com/guide/navigation) for complete reference and practical examples.
It’s always important to keep up with the changes and updates in your chosen technologies to ensure that your codebase remains efficient, healthy, and compatible with future versions of the tools and libraries you’re using. Deprecating `setOnNavigationItemSelectedListener` represents one such change within the vast landscape of Android development.
Exploring Alternatives to Deprecated SetOnNavigationItemSelectedListener
With the introduction of Material Design’s BottomNavigationView, developers found an easier way to implement navigation within their applications. The method SetOnNavigationItemSelectedListener has been a long-time favorite for many coders. However, Android officially deprecated this method in favor of another approach, causing numerous developers to scramble for substitutes. Let’s delve into the potential alternatives to SwapOut BackSetOnNavigationItemSelectedListener without hindering your application’s usability and effectiveness.
One notable alternative is using OnOptionsItemSelected. This function comes built-in within Android Studio and affords developers the capability of handling selections of items on the system or application menu without needing to implement setOnNavigationItemSelectedListener.
Here’s an example of how you’d use it:
java
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.home_menu:
// handle home_menu click here.
return true;
default:
return super.onOptionsItemSelected(item);
}
}
Another viable alternative is to employ the Navigation Component. As Google’s official documentation states, the Navigation Component is a library that manages complex navigational structures and transitions, providing a navigational pattern significantly broader than what SetOnNavigationItemSelectedListener offers.
You can setup the Navigation Component with BottomNavigationView following these steps:
1. Add a dependency for the Navigation UI KTX:
java
implementation ‘androidx.navigation:navigation-ui-ktx:2.3.5’
2. Setup BottomNavigationView:
java
val navController = findNavController(R.id.nav_host_fragment)
findViewById
As Tim Berners-Lee, the inventor of WWW, once said: “The Web as I envisaged it, we have not seen it yet.” This statement can apply to software development – technology is everevolving, and developers should be adaptable. Therefore, recognizing when to retire deprecated functions like `setOnNavigationItemSelectedListener` and substitute them with newer ones is crucial in becoming a successful developer.
Adapting to new methods might seem overwhelming at first glance, but they offer a plethora of benefits such as increased productivity, simpler code management, and improved user experience. These advantages elevate them from being mere replacements to potent and progressive functions capable of shaping the future direction of app development.
Why Move Away from Using SetOnNavigationItemSelectedListener
The Android platform’s changes are characterized by constant evolution and deprecation is a part of this process. Recently, the traditional navigation method `setOnNavigationItemSelectedListener` for Bottom Navigation Views has been deprecated within new versions of the Material Design Library.[Reference]
Before we delve into the reasons and implications of this deprecation, you should know that deprecation doesn’t mean that the feature is removed immediately. Instead, it serves as a warning that there are better alternatives that should be used, so early adoption is beneficial.
The major reasons for moving away from `setOnNavigationItemSelectedListener` are:
- Emphasis on single-Activity architecture: Over time, Google’s guidance to Android developers has been shifting towards a preference for single-Activity applications using the Navigation component[Reference]. The previous approach might not combine smoothly with this structure, prompting the transition.
- Introduction of OnDestinationChangedListener: The new recommended way to listen for selection events in your Navigation component is to set an `OnDestinationChangedListener` on your NavController[Reference]. It offers more control over navigation and understanding the back stack.
Here’s a simplified code snippet that demonstrates how to utilize `OnDestinationChangedListener`.
java
navController.addOnDestinationChangedListener(new NavController.OnDestinationChangedListener() {
public void onDestinationChanged(@NonNull NavController controller, @NonNull NavDestination destination, @Nullable Bundle arguments) {
//your desired actions here
}
});
To quote Brian Goetz, ‘Innovation is never free.’ And it applies to software development as well. The introduction of newer APIs may initially require learning and adaptation. However, they ultimately lead to more efficient systems being deployed.
I hope this gives you a comprehensive understanding of why `setOnNavigationItemSelectedListener` has been deprecated and the benefits of adapting to the replacement.
Impacts and Considerations with Deprecating SetOnNavigationItemSelectedListener
Deprecating the method
setOnNavigationItemSelectedListener
found in Google’s Material Design Bottom Navigation View has sent ripples in the Java programming community, spawning numerous discussions and debates regarding its direct impact on applications and potential alternatives.
Impacts:
- Code Refactoring: Developers now necessitate investing time and resources to refactor their existing code. This move can be seen as both a boon or bane depending upon individual’s perspective.
- Ripple Effect in Libraries and Dependencies: Any library or dependency relying on this deprecated method might face issues and may need updates.
- Migrating Complexity: Transitioning to alternative options might induce certain complexities due to unfamiliarity with the nuances of new methods.
In Larry Wall’s insightful words, “The three chief virtues of a programmer are: Laziness, Impatience and Hubris.” The deprecation indeed presents certain challenges but it is also an opportunity for evolution of more efficient coding practices.
Considerations:
- Adopting the Replacement: It is crucial to adapt to the officially recommended alternative,
setOnItemSelectedListener
, which is believed to offer similar outcomes.
- Longevity of Codes: Instead of dwelling on the problem, it’s worth focusing efforts on making codes adaptable and flexible to changes.
- Active Learning: Embrace the change as an opportunity to learn something new and improve the longevity and efficiency of your application.
There is no denying that deprecated methods can pose challeneges in the maintenance phase. However, the overall motive behind such steps is typically improving the performance and effectiveness of the codebase.
For instance, consider the shift to the new
setOnItemSelectedListener
,
`Google Official Documentation`
Remember Hal Abelson’s wisdom, “Programs must be written for people to read, and only incidentally for machines to execute.” Through this deprecation, your approach towards efficient coding will not only enhance your skillsets but also align your work with the dynamic trends of programming.
The drive to keep updating our skills makes us bounce back even higher irrespective of the challenges faced.
Future-proofing our codes is often achievable by staying compliant with updated methods while actively adopting the newer methods arriving with updated versions.
SetOnNavigationItemSelectedListener Deprecated
The
setOnNavigationItemSelectedListener
method, commonly employed within Android development in regards to BottomNavigationView, has been declared deprecated with the advent of more recent versions of Material Design Libraries (Version 1.2.0-alpha03 and above). The rationale behind this decision mainly revolves around streamlining the process for easier and more efficient coding approach.
Deprecation does not signify immediate obsolescence but is rather a soft warning issued by developers signaling their intent to phase out such API components gradually in the future. It therefore behooves programmers to evolve flexibly by progressively embracing prevalent alternatives.
In lieu of the
setOnNavigationItemSelectedListener
method, modern design libraries have introduced the
OnItemSelectedListener
method to be used in connection with navigation components. Below is a simple Java example showcasing this switch:
BottomNavigationView bottomNavigationView = findViewById(R.id.bottom_navigation);
bottomNavigationView.setOnItemSelectedListener(new NavigationBarView.OnItemSelectedListener() {
@code@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()){
case R.id.navigation_home:
//
break;
case R.id.navigation_dashboard:
//
break;
case R.id.navigation_notifications:
//
break;
}
return true;
}
});
It’s worth noting that the transition from the deprecated
setOnNavigationItemSelectedListener
to the newer
OnItemSelectedListener
aims at ensuring the consistent handling of selections upon updating the selected state programmatically.
As a concluding thought, here is an apt quote by Jeff Atwood, co-founder of Stack Overflow – “Coding is not ‘fun’, it’s technically and ethically complex.” A clear testament to the dynamic nature of software advancements and the consequent need for technical adaptability amongst programmers.
References:
Android Documentation Deprecated SetOnNavigationItemSelectedListener
StackOverflow Discussion on Deprecation