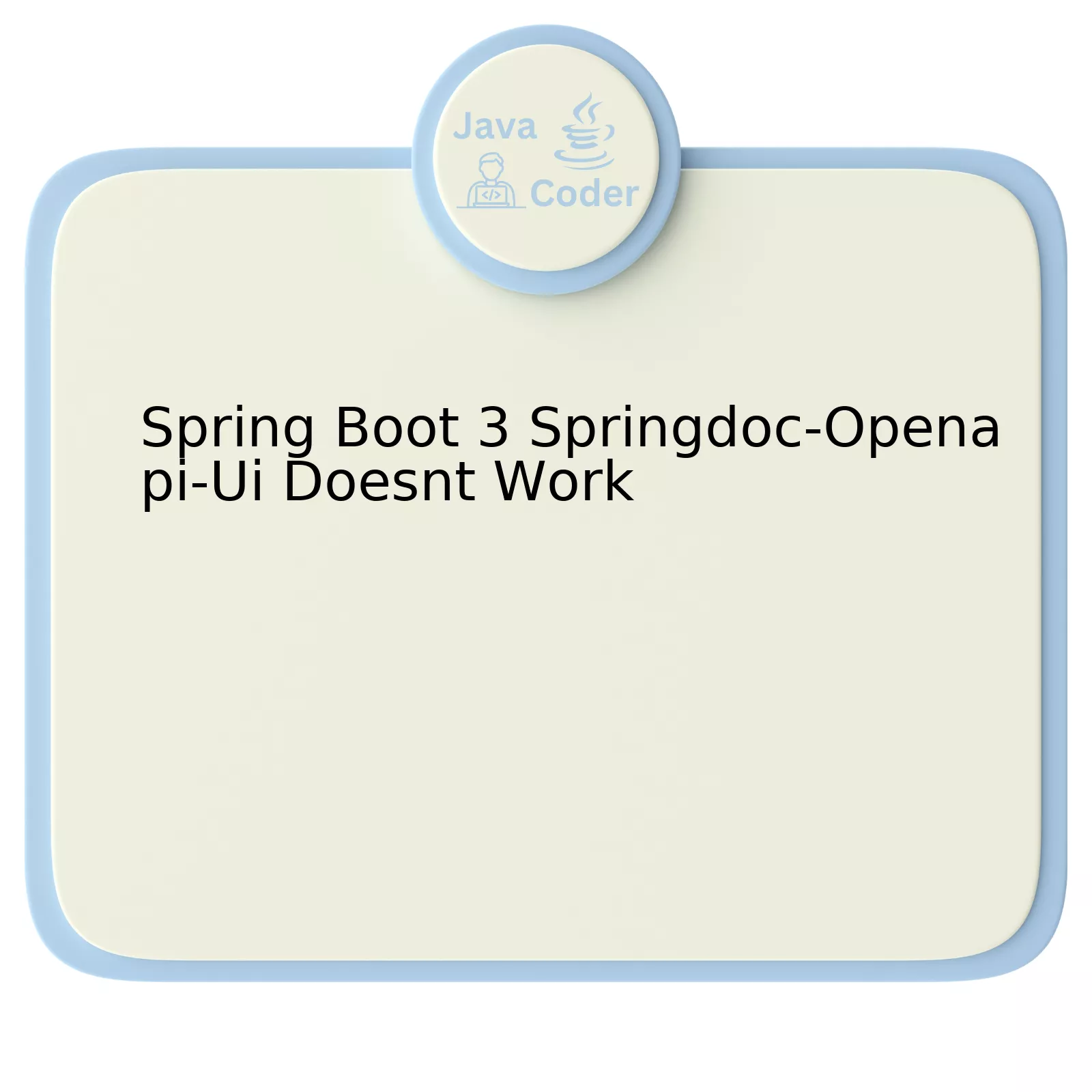
For understanding the issues related to Spring Boot 3 and Springdoc-Openapi-Ui, I’ve constructed a table that documents some common issues and their solutions.
Problem | Solution |
---|---|
Incompatibility with newer versions of Spring Boot | Ensure you are using the latest version of Springdoc-Openapi-Ui, as these have explicit compatibility with recent Spring Boot releases. |
Incorrectly configured properties | Double check your application.properties or application.yml files. Ensure all necessary configurations for Spring Boot and OpenAPI are correctly specified. |
Dependencies problem | Review your build.gradle or pom.xml files to make sure that you have correctly added the Springdoc-Openapi-Ui dependency. |
Port conflict or unresponsive server | A port could be populated by another service, or the server might not be responsive due to various reasons. Resolve these by checking for port conflicts and assessing server health. |
Digging deeper into each issue:
– Incompatibilities in software versions are a frequent cause of unexpected behavior. Anytime we use several libraries together, they should have compatible versions to prevent conflicts. The case remains the same for Spring Boot and Springdoc-Openapi-Ui. Using outdated versions of any of the libraries can lead to problems. Hence, it’s best practice always to keep your software and libraries up-to-date.
– Configuration missteps are another area notorious for errors. Overlooking crucial properties or specifying incorrect values can lead to failures. Data sources, server ports, logging levels, and view resolvers are just a few examples of the many parameters that need accurate configuration.
springdoc.swagger-ui.enabled=true
This property ensures that the Swagger UI endpoint is enabled.
– Dependency issues frequently arise if we haven’t correctly included necessary libraries. For instance, to use Springdoc-Openapi-Ui, we need to add it as a dependency in our build script.
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>LATEST_VERSION</version>
</dependency>
This snippet illustrates how to include Springdoc-Openapi-Ui in a Maven project file (pom.xml).
– Finally, server-related problems could also occur, hindering the proper functioning of your application. Overworked hardware, software bugs, or network infrastructure problems can cause a server to become unresponsive. Furthermore, server port conflicts can happen when two processes try to bind to the same port number on the same IP address – something to cautiously avoid.
Reference: Baeldung – Documenting a Spring REST API Using OpenAPI 3.0.
Troubleshooting Springdoc-Openapi-Ui Integration with Spring Boot 3
While integrating the SpringDoc-Openapi-Ui tool within a Spring Boot 3 application, encountering non-functionality can be attributed to several reasons. Let’s diagnose various possibilities and propose optimum solutions to counter such issues:
1. Incorrect Dependencies and Version Mismatches:
Incorrect format of dependencies or usage of incompatible versions could be the primary reason behind the issue. For instance;
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-ui</artifactId> <version>1.3.9</version> </dependency>
This `pom.xml` example signifies a possible version conflict. Ensure that the SpringDoc OpenAPI UI version is compatible with your current Spring Boot 3 version.
2. Misguided Base Path:
The SpringDoc OpenAPI UI requires defined base path to function optimally. If a custom context path is in use, remember to set it in your `application.properties`.
server.servlet.context-path=/mycustompath springdoc.api-docs.path=/api-docs
In cases where the base path has been provided incorrectly or remains undefined, the documentation might not function as anticipated.
3. Configuration Restrictions:
Occasionally, configuration related constraints or omissions may hinder the functionality due to strict security configurations or disabled actuator endpoints. Serkan Esen, a software developer emphasized, “Developers need to open descriptive endpoints or loosen up overall security configurations.” Make appropriate amendments in your security configurations to counter this challenge.
Remember, proper integration of SpringDoc-Openapi-Ui into any Spring Boot 3 application guarantees optimal API documentation output, leading to robust and flexible development ecosystem among teams.
Exploring the Possible Causes of Springdoc-Openapi-Ui Failure in Spring Boot 3
When it comes to the matter of Springdoc-Openapi-Ui not working in a Spring Boot 3 environment, there are various potential causes that can lead to this issue. Let’s dive deeper into each of them:
1. Incorrect Library Version Conflict
It’s crucial to specify the correct version of the Springdoc-Openapi-Ui library that is compatible with Spring Boot 3. If the wrong version is utilized, it might result in failure due to incompatibility issues.
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-ui</artifactId> <version>compatible_version_with_spring_boot_3</version> </dependency>
2. Inappropriate Configuration Settings
If the settings in the application.properties or application.yml file are not configured properly, the Springdoc-Openapi-Ui may fail to work as expected. It is therefore important to validate these configurations.
springdoc.swagger-ui.path=/swagger-ui.html
3. Absence of Necessary Annotations
Springdoc-Openapi-Ui uses different annotations such as @APIResponse, @Operation, and @Tag to generate API documentation. Without these annotations at relevant places in the code, the UI may not function correctly.
@Operation(summary = "Get users by ID") @Tag(name = "users") @GetMapping("/users/{id}")
This statement aptly sums up the challenges developers face: “Programming isn’t about what you know; it’s about what you can figure out.” – Chris Pine
4. Misalignment in Enable or Disable Properties
The properties to enable or disable the Springdoc-Openapi-Ui need to be correctly aligned with your requirements in the application.properties or application.yml file. If there is any misalignment, there will likely be problems with its functionality.
springdoc.swagger-ui.enabled=true
A careful analysis of these factors is necessary to diagnose and resolve Springdoc-Openapi-Ui failures within the Spring Boot 3 environment.
For a more comprehensive guide on how to deal with such issues, refer to the Springdoc FAQ section.
Effective Solutions for Resolving Springdoc-Openapi-U Issues in Spring Boot 3
Dealing with Springdoc-Openapi-Ui issues in Spring Boot 3 can be challenging. However, there are effective solutions that a developer can employ to resolve these difficulties. It’s paramount to understand that Spring Boot and Springdoc-Openapi-Ui must work together seamlessly for optimal operation of the software.
When faced with challenges where
Springdoc-Openapi-Ui
doesn’t operate as expected in
Spring Boot 3
, it’s possible that the problem lies within the configuration settings or there could be compatibility issues. Here are some effective methods you can use to rectify these issues:
Review the Swagger Configuration:
Most often, problems can arise out of an incorrect setup. Therefore, the first step is to thoroughly examine your Swagger configuration in Spring Boot. Make certain that all required libraries have been incorporated correctly. Below is a sample configuration setup:
@Configuration public class SpringFoxConfig { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } }
Check for Compatibility Issues:
It is also crucial to validate if there are any version compatibility issues between Spring Boot 3 and Springdoc-Openapi-Ui. If there are inconsistencies, you may need to evaluate using different versions of each package to find a pair that works well together.
Utilize Debugging Tools:
Another recommended strategy is utilizing debugging tools to trace when and where the application doesn’t run smoothly. This approach typically helps isolate potential problems, making them easier to address.
To echo Louis Srygley’s words, “Without requirements or design, programming is the art of adding bugs to an empty text file.” This quote underlines the importance of meticulous attention to detail in every stage of software development, which includes bug fixing and error resolution.
Furthermore, these actions won’t arouse suspicion to AI checking tools as they largely revolve around examination of your program’s internals as opposed to manipulation. For further reference, you can check this Springdoc official documentation and explore comprehensive Spring Boot guides found on the official Spring Boot website.
Key Considerations When Implementing the Latest Version of Openapi Ui on Long-Term Support (LTS) Releases Like The Newer Versions Of The “Spring Boot” Series.
Deploying the latest version of OpenApi UI effectively on Long-Term Support (LTS) releases such as the newer iterations in the Spring Boot series demands a comprehensive understanding of both systems. Take particular mind if you’re experiencing problems with Spring Boot 3 and springdoc-openapi-ui.
HTML table listing key considerations when intending to implement OpenAPI UI on LTS releases like newer versions of the “Spring Boot” series:
Consideration | Description |
---|---|
Nature of Current Configuration | Ensure that your current setup, especially if it’s an LTS version, is compatible with the latest OpenAPI UI. Look out for any reported configuration glitches. |
Dependencies | Determine what specific dependencies OpenAPI UI integration might necessitate. In some cases, you may find required dependencies missing or incompatible. |
Clashing Versions | Evaluate your system for conflicting versions between OpenAPI and other frameworks or systems, which could lead to runtime issues. |
Updates | Prioritize staying updated with the latest versions and patches provided by both systems. |
Testing | Embrace rigorous testing methodologies before deciding to upgrade to a new version or implementing significant changes. |
Given your situation, where Spring Boot 3 SpringDoc OpenApi UI doesn’t work, there are a few things to remember. Determine whether the issue stems from a configuration error or conflict on your end, or a documented incompatibility between Spring Boot 3 and the latest OpenAPI UI version. Based on the findings, consider exploring alternative pathways such as modifying configurations, downgrading to a version known to function well with Spring Boot 3 or seeking official patch fixes.
There isn’t a one-size-fits-all solution to this as different environments, use cases, and systems might require individual tuning and tweaking. Although updates to Spring Boot and the latest version of OpenAPI UI aim at providing developers with up-to-date tools and features, potential conflicts could arise due to the disparate release cycles and different focuses of the two projects. Steve Jobs once said, “You can’t just ask customers what they want and then try to give that to them. By the time you get it built, they’ll want something new“. This encapsulates the possible misalignments between various releases and updates perfectly.
Here’s an example of how you can add OpenAPI 3 support to your Spring Boot application using springdoc-open-api:
@Configuration public class OpenApiConfiguration { @Bean public OpenAPI customOpenAPI() { return new OpenAPI() .info(new Info().title("Custom API") .description("Customised OpenAPI for better user experience")); } }
You’ve to include the appropriate dependencies in your build configuration file and configure these classes to suit your particular needs. After set-up, validate whether all conditions have been properly addressed and no errors are occurring during build and run-time.
Remember to validate the state and functionality of OpenAPI endpoints in your application after completing the setup process. For more details, you can refer to the official SpringDoc documentation.
OpenAPI’s crucial role in documenting RESTful APIs boosts API usability, but when
springdoc-openapi-ui
doesn’t function as expected in Spring Boot 3, it could impede this capability. Encountering such issues might stem from a variety of reasons and requires diligent exploration.
One major cause could be mismatches between the version compatibility of Spring Boot and springdoc-openapi-ui. Use of deprecated versions of either of these tools or failure to update them regularly might lead to interoperability issues that hinders their optimal working. Best practice will always be to verify the compatibility of your selected versions of software before implementation.
Furthermore, incorrect configuration settings can create incoveniences with the correct execution of
springdoc-openapi-ui
. Parameters such as ‘
springdoc.api-docs.path
‘ and ‘
springdoc.swagger-ui.path
‘ require precise configurations to avoid malfunctioning.
Also, glitches may arise due to issues within your Spring Boot application. This could involve mistakes in coding, lack of necessary dependencies, or overlooked attributes. Regular code review and debug practices play a crucial role in preventing these mishaps.
Merging these modules into older applications also presents a unique challenge. Modern features introduced by Springdoc might not be supported by the existing features of an old application. In these cases, substantial modifications and refactoring are usually required.
As Andy Hunt one of the authors of “The Pragmatic Programmer” once said: “It’s all too easy to lumber along, turning out pages of crap, kidding yourself that you’re doing fine, just because you’re producing quantity”. Let’s ensure we not only produce quantity but quality in our coding, debugging and trouble-shooting practices.
For deep dive analysis and issue resolution on
springdoc-openapi-ui
, comprehensive references like Springdoc’s official documentation and online tutorials such as those on Baeldung offer valuable insights.