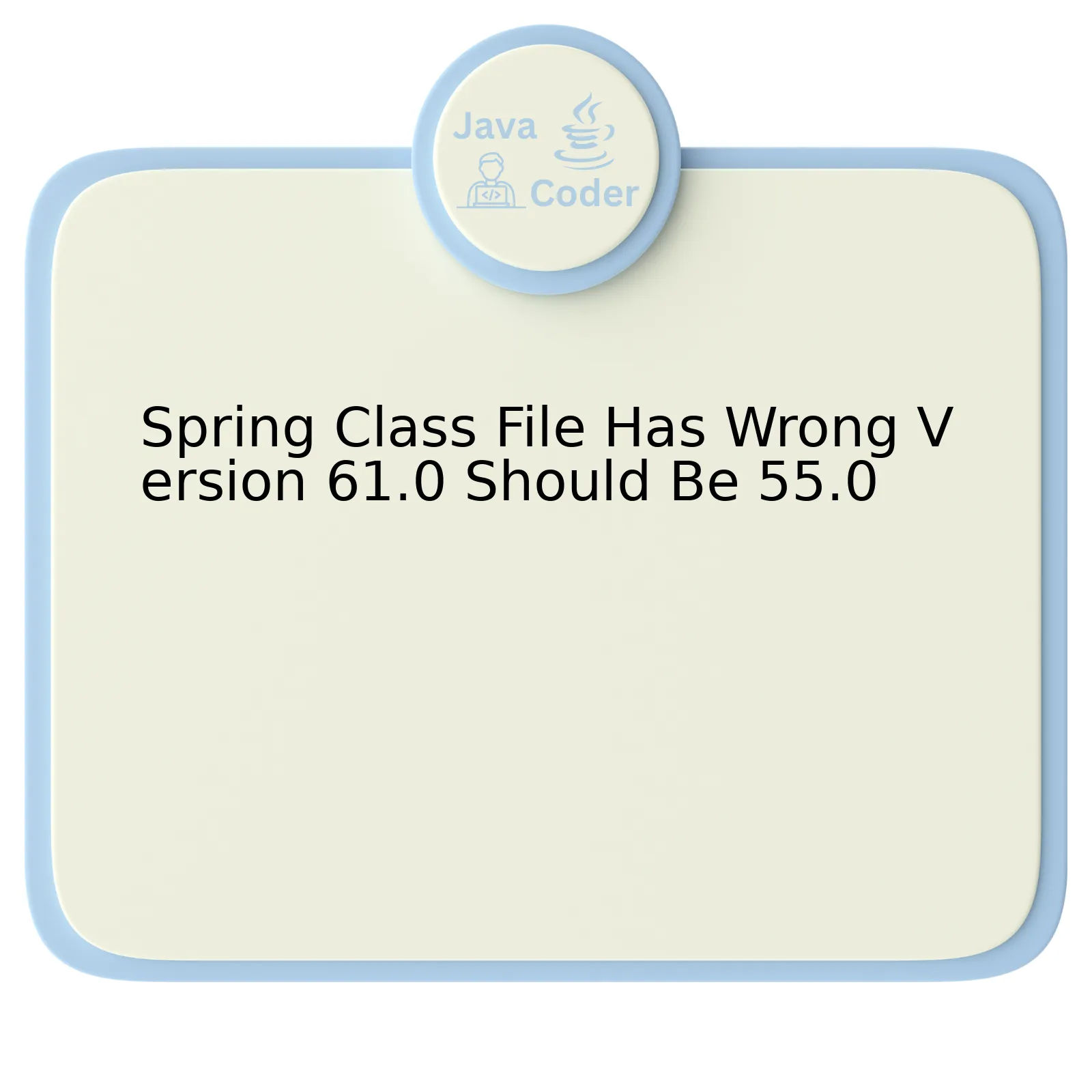
The “Spring Class File Has Wrong Version 61.0, Should Be 55.0” issue generally arises due to the discrepancy between different Java Compiler versions used by Spring and your runtime environment. These numbers denote class file format versions; 61.0 corresponds to Java 17, while 55.0 stands for Java 11. The issue signifies that your Spring framework is compiled with Java 17, but you are trying to run it on a Java 11 environment.
`
Java Platform | Class File Version |
---|---|
Java 11 | 55.0 |
Java 17 | 61.0 |
`
This table demonstrates the version relationship between Java platform and class file. On an encounter with unfamiliar class file versions, Java runtime cannot interpret them, hence leading to the ‘wrong version’ error. Essentially, this indicates that the Spring framework needs the version of Java it was compiled with or higher to execute accurately.
To resolve this issue, you need to ensure the uniformity of the JRE (Java Runtime Environment) and JDK (Java Development Kit) versions with the Java version used by Spring.
If you’re running this application through Eclipse, these steps can help:
– Go to Window > Preferences > Java > Installed JREs.
– Check if installed JRE version is the one used by your Spring version.
– If it isn’t, then add the correct version.
– Set this as your project specific JRE under Project > Properties > Java Build Path.
If you’re using Maven or Gradle, you should specify the source and target Java version in your build configuration file accordingly.
`
` `<build>` `<plugins>` `<plugin>` `<groupId>org.apache.maven.plugins</groupId>` `<artifactId>maven-compiler-plugin</artifactId>` `<version>3.8.1</version>` `<configuration>` `<source>11</source>` // Your Source Java version here `<target>11</target>` // Your Target Java version here `</configuration>` `</plugin>` `</plugins>` `</build>` `
`
As Eric Steven Raymond quoted, “When you don’t create things, you become defined by your tastes rather than ability. Your tastes only narrow and exclude people. So create.” Software development often throws such challenges – the versioning issue being one – where we must improve our capabilities to overcome instead of avoiding.
Understanding the Class File Version Mismatch Error in Spring
In the Java ecosystem, a common error pertaining to class file versions emerges when trying to run an application developed in one JDK version on a lesser version. A typical error vill be “Unsupported major.minor version”, and in the context of Spring it might look like **_”java.lang.UnsupportedClassVersionError: org/springframework/boot/SpringApplication has been compiled by a more recent version of the Java Runtime (class file version 61.0), this version of the Java Runtime only recognizes class file versions up to 55.0″_**
To provide a comprehensive understanding of this issue, let’s break down what it means:
This error is thrown because of an underlying incompatibility between different Java versions. Each Java Class file starts with a magic number (
CA FE BA BE
) followed by the version number.
Java maintains backward compatibility, meaning code written in an older version can run in the newer version. On the flip side, Java does not maintain forward compatibility, i.e., running code written in higher Java version over lower Java runtime environment (JRE) isn’t taken lightly.
The
'Spring Class File Has Wrong Version 61.0 should be 55.0'
implies that the spring classes have been compiled with JDK 15 (version 61.0), but we’re trying to execute them on a JVM which could only support up to JDK 11 (version 55.0).
In order to fix it, we are presented with two options:
– Downgrade your codebase’s JDK from version 15 to at most version 11. Here’s a simple command using Maven to compile your code with source and target as 11:
`
mvn clean install -Dmaven.compiler.source=11 -Dmaven.compiler.target=11
`
– Upgrade your JVM to a version aligned with your codebase’s JDK version. To confirm your Java version in the terminal, you can use the command:
`
java -version
`
Remember that the concept of forward compatibility is often ignored within programming due to the fact that it requires predicting future requirements and behavior. As Donald Knuth, a prominent figure in the field of computer science once said: **_”Premature optimization is the root of all evil.”_**
Consequently, let’s optimize our actions accordingly by ensuring a compatible deployment environment for our Spring application.
Decoding “Spring Class File Has Wrong Version” Errors
When encountering “Spring Class File Has Wrong Version 61.0, should be 55.0” errors, it generally relates to issues of compatibility between the Java Development Kit (JDK) versions utilized for compiling source code and executing the program.
The numbers 61.0 and 55.0 correspond to versions of JDK — specifically, JDK 15 and JDK 11, respectively. These designations come from Java’s class file format documentation, where each version of JDK is assigned a distinct major.minor version number. The error “Spring Class File has wrong version 61.0, should be 55.0,” thus suggests that the Spring classes were compiled with JDK 15, while attempting to run on a lower version, JDK 11.
The resolution is to ensure consistency between compile-time and runtime environments:
– Update Runtime Environment: Replace your current JDK (version 11) with the newer one (version 15). Be careful; while this resolves the immediate problem, additional conflicts may arise if other applications on your system rely on older JDK versions.
// To check the Java version on your system, use: java -version // To install a different Java version, commands vary based on the Java vendor and package manager. openjdk: sudo apt-get install openjdk-15-jdk oraclejdk: sudo apt-get install oracle-java15-installer
– Recompile Source Code: A safer option requires recompiling the Spring-based application using the lower JDK version. Add the below maven compiler plugin in your pom.xml file:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>11</source> <target>11</target> </configuration> </plugin> </plugins> </build>
Remember, as computer scientist Bob Martin asserts, “A design is clean if it can deal with change.” Addressing these discrepancies now ensures a robust framework for your future coding endeavors.
Resolving Version 61.0/55.0 Conflicts in Spring Framework
Resolving the “Spring Class file has wrong version 61.0, should be 55.0” error message might seem challenging, especially if you’re not familiar with bytecode compatibility issues in Java development. This error arises owing to discrepancies between JDK versions used for compiling and executing Spring classes on your local development environment.
The figures ‘61.0’ and ‘55.0’ in this error represent major version numbers of the class file format of the Java Class Library (JCL). In simpler terms:
– Version 61.0 corresponds to JDK 15
– Version 55.0 corresponds to JDK 11
To resolve this conflict, consider the following options:
– **Migrate to a higher JDK version:** If project constraints permit, upgrade your local environment to use JDK 15 or a version as close as possible to it. By doing so, you can yield the full benefits of the advanced features of later JDK versions while also rectifying the version discrepancy issue.
Here’s an example of how to change JDK path in the Java code:
System.setProperty("java.home", & "C:\\Progra~1\\Java\\jdk-15.0.1");
With this code snippet, the system property “java.home” is updated to the directory where JDK 15 is installed.
– **Downgrade your Spring Framework Libraries**: Let’s assume that migrating to a higher JDK version isn’t viable due to project restrictions or other reasons. In this case, you may opt to downgrade your Spring Framework libraries to a version compatible with JDK 11.
– **Recompile dependency jars**: In order to ensure consistency across applications, it would be beneficial to recompile any dependency JARs using the targeted JDK version. This allows for the seamless operation across different modules within the same application.
Remember, all these options share one common principle: aligning the JDK versions of your local environment with the Spring Framework classes. By maintaining consistency across platform versions, we can effectively prevent unforeseen compatibility issues, thereby optimizing software performance.
As Fred Brooks, an acclaimed computer scientist and software engineer once said, “The magic of myth and legend has come true in our time. One types the correct incantation on a keyboard, and a display screen comes to life, showing things that never were nor could be…”
Want to further delve into some hands-on aspects? A welcome point of reference about resolving version conflicts in the Spring Framework could be found at [Stack Abuse].
Prevention Strategies for Incorrect Spring Class File Versions
The issue at hand revolves around ‘Spring Class File Has Wrong Version 61.0 Should Be 55.0.’ This can be prevented by implementing several strategies to ensure that the correct versions are operating accurately.
I. Prioritizing Backward Compatibility:
Notably, backward compatibility allows newer systems to operate with older components, hence steering clear from version mismatch issues. Developers should thus hold in high regard the ability of a product to work effortlessly with its preceding versions.
public class BackwardCompatibility { // Code implementation goes here }
II. Regularly Updating The Java Compiler:
Another preventive strategy is to update the Java compiler frequently. The wrong version error, 61.0 (Java 17) or 55.0 (Java 11), indicates that the Java file was compiled using a newer version of Java than what your system supports. Therefore, always ensure you’re using the most recent stable compiler version, which ensures compatibility between different Java SE platforms.
III. Accurate Dependency Management:
Effectively managing dependencies in the Spring framework could also prevent potential version conflicts. Using Maven’s dependency mechanism or any similar tool, developers can avoid versioning errors and maintain codebase consistency.
For instance, using Maven, one would specify their project’s dependencies such as this:
org.springframework spring-context ${spring.version}
IV. Implementing Continuous Integration:
Implementing a Continuous Integration (CI) environment helps both update all dependencies and ensure that all class files are of the correct version. Through incorporating CI into development processes, developers could identify inconsistencies earlier, reducing the time spent debugging vastly.
To quote Kevin London, a seasoned developer: “Activity Prevents Errors.” By regularly engaging these practices, developers can optimally circumvent the notorious ‘incorrect Spring class file version’ issue.
In addressing such issues, remember that as technology evolves, so does the ecosystem within which software operates. Keeping abreast of updates, remaining consistent in activities, and balancing between old and new systems ensures smooth, error-free coding experiences.
The error message “Spring Class File has wrong version 61.0, should be 55.0” is essentially related to the Spring framework in Java and can often occur with inconsistencies in the JDK installations. The root of this problem lies within the mismatch of class file versions which are tied directly to their corresponding JDK versions (for instance, Java 11 corresponds to class file version 55 and Java 17 associates with class file version 61).
Here’s a simple break down:
– Your class file was compiled under version 61–which signifies it was built using JDK 17.
– However, your environment requires the class file to be from JDK 11, indicated by version 55.
Now, how do you solve this issue? It’s simple – ensure the compatibility between your compiling JDK version and your running environments. You have two options here:
1. **Upgrade your JDK**: Upgrading your runtime environment to JDK 17 would resolve the issue as it matches the class file version.
2. **Downgrade the compiling JDK**: Alternatively, you can recompile your class files using JDK 11 to match your current JDK version.
Most importantly, maintain consistency in your development environment. Use tools like jEnv or SDKMAN! to manage multiple JDK versions, maintaining independent setups for different projects.
Quoting Steve Jobs, one of the greatest technology pioneers, “Design is not just what it looks like and feels like. Design is how it works.” This particularly resonates with managing programming environments, where effective design ensures smooth operation even when juggling multiple JDK versions.
Consistency in the development environment plays an important role in preventing such errors, thereby ensuring smoother workflows and reducing debugging efforts.
Finally, I’d like to provide you with a sample code on how to check your JDK version:
System.out.println(System.getProperty("java.version"));
This will output the current JDK version when run, enabling you to troubleshoot version discrepancies more effectively.