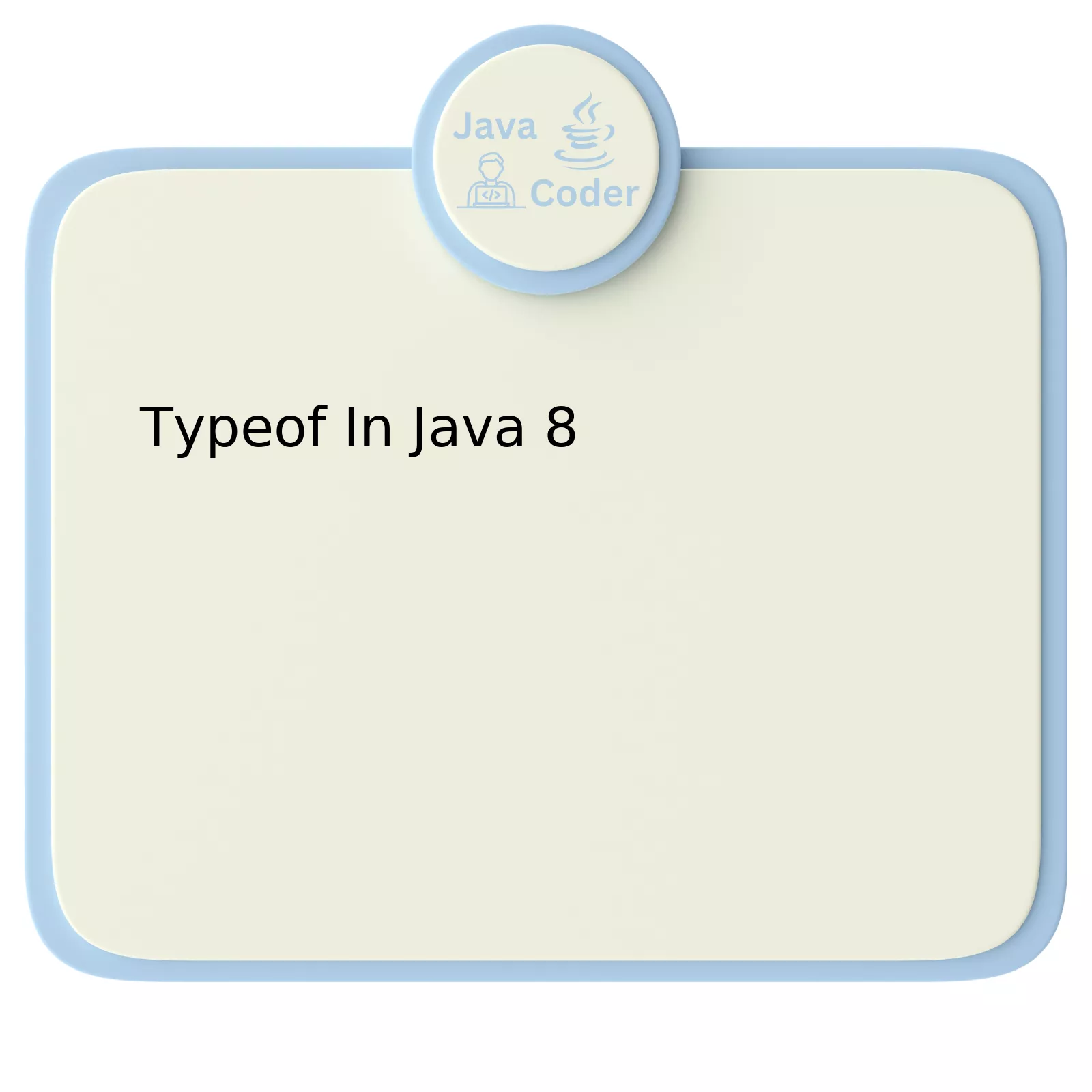
When discussing Java 8, it’s crucial to note that it does not possess a direct equivalent of JavaScript’s “typeof” operator. However, the Class object’s getName() method and instanceOf keyword comes closest in functionality.
Let’s present this comparison using a representation:
Java Concept | Description | Example |
---|---|---|
instanceof |
Allows checking if an object is an instance of a specific class or implements a particular interface. |
if (object instanceof String) {...} |
getName() |
Returns the class name as a String of the object on which it’s called. |
String className = object.getClass().getName(); |
The first row of our table refers to
instanceof
, which is an essential Java keyword. This keyword aids in determining whether a certain object is an instance of a particular class or if it implements a particular interface. A practical example would be:
if (object instanceof String) {...}
.
In contrast, the second representation corresponds to
getName()
, which returns the class name as a string from the object on which it gets executed. Here is a basic usage:
String className = object.getClass().getName();
. This approach may remind you of JavaScript’s “typeof”, albeit it is the name of the class we are dealing with here, not the type of the variable.
While these both methods help to identify the type of an object, it is quite important to emphasize the distinction between them. The
instanceof
operator suits best when you need to check if an object is derived from a specific class or interface. In contrast,
getName()
serves better when you’re interested in getting the actual name of an object’s class.
As Steve McConnell eloquently pointed, “Good code is its own best documentation.” And indeed, understanding how to use the
instanceof
keyword and
getName()
method effectively can open up new dimensions of coding style and efficiency within Java 8.
Understanding the Role of Typeof in Java 8
The operator
typeof
isn’t used directly in Java. However, a comparable feature exists known as type checking which is implemented through the
instanceof
keyword. When dealing with object-oriented programming and polymorphism in Java 8, the role of this keyword is pivotal.
Type Checking in Java
Java uses type checking to verify and enforce the rules for type compatibility at compile time. Type checking helps alleviate unexpected runtime errors due to incompatible types. The
instanceof
operator is one way to perform a type check.
Example code:
if (object instanceof String) { System.out.println("Object is a string"); }
This will check if an object is an instance of `String` class.
According to Guy Steele, co-inventor of the Scheme programming language:
“Type is more than just what bits we have. They imply how to process those bits.”
In light of this, the role of the
instanceof
operator becomes even more important. It aids in determining ‘what bits we have’ and allows us to handle them appropriately based on their type.
The equals() method and Type Checking
In addition to
instanceof
, Java’s
equals()
method can play a role in type checking during comparison operations. Here is an example:
public boolean equals(Object obj) { if(this == obj) return true; if(obj == null || obj.getClass()!= this.getClass()) return false; // typecasting of the argument. Object obj2= (Object) obj; // comparing the state of argument with // the state of 'this' Object. return (obj2.value == this.value); }
In this snippet, `obj.getClass()!= this.getClass()` is another way of performing type checking. It compares whether the Class of ‘this’ object and the provided object are the same. If they aren’t, it returns false since objects of different types can’t be equal.
The method has then cast the argument to the correct type using `(Object) obj`, again reflecting the importance of understanding the type of your object.
This observation underscores the sheer utility and need for type checking capabilities in object-oriented languages like Java. Understanding these constructs ensures better design, type safety, and ultimately, robust programs.
Implementing Typeof Functionality in Java 8
Java, unlike languages such as JavaScript, is a statically typed language. This means that the type of a variable must be declared at compile-time, contrary to dynamically typed languages where the type can be determined at runtime using a “typeof” functionality. However, there are methods in Java we can use to replicate some characteristics of the “typeof” functionality.
Firstly, it’s worth noting that every object in Java is a descendant of the built-in
Object
class. Therefore, one could harness the power of the
.getClass()
method, which returns the runtime class of this object.
Here is an example:
java
String myString = “Hello World”;
System.out.println(myString.getClass().getName());
The output would be
java.lang.String
, indicating that the object type was indeed a String.
As you might see, this example only works for non-primitive types. For primitive types, you can utilize Java’s wrapper classes and their
TYPE
field. For instance, if you’re unsure whether a variable holds an
int
:
java
System.out.println(Integer.TYPE);
This statement outputs
int
, the name of the primitive type that its Wrapper class corresponds to.
If your goal is to have more complex conditional logic around the type of the object, you might take advantage of
instanceof
keyword. It checks whether an object is an instance of a specific class or interface.
Here’s a usage sample:
java
if (myString instanceof String) {
System.out.println(“It’s a String!”);
}
Whenever
myString
holds any instance of a
String
, the above statement prints: “It’s a String!”.
However, before going through with these kind of solutions it’s important to remember what Tony Hoare, the creator of Null Reference, said:
“I call it my billion-dollar mistake… At that time, I was designing the first comprehensive type system for references in an object-oriented language”.
He points directly towards the idea that even though technology provides us with capabilities, it doesn’t necessarily mean we have to use them in ways they weren’t intended for. Java 8 has workarounds for “typeof”, but understand they shouldn’t replace good practices and design.
To learn more about how java handles object types, check out Oracle’s official documentation on Inheritance. Available online, it provides a deep dive into the concept of class hierarchies and their role in Java’s type system – down to primitives and their wrapper classes.
Key Benefits and Limitations of Using Typeof in Java 8
In the realm of Java 8, the typeof operator doesn’t exist as you might find in languages like JavaScript. Yet, there exists an analogous mechanism to typeof, that is, making use of the
getClass()
method posited within the root class Object. This particular method provides a myriad of benefits and also has its own set of limitations.
When discussing the key benefits of using
getClass()
in Java 8:
- Type Identification: The most critical advantage is to aid in identifying the type or class of any object instantiated in Java. A prime example could be:
Object obj = new String("Hello, world!");
System.out.println(obj.getClass().getName()); // Outputs: java.lang.String
By using
getClass().getName()
, it can swiftly print out the object’s type, just like how `typeof` behaves.
- Dynamic Typing: Dynamically-typed programming paradigms such as JavaScript have the luxury of utilizing `typeof` for seamless type identification throughout the codebase. With Java’s
getClass()
, developers can emulate this functionality up to some extent. It can become particularly handy during inheriting classes and managing polymorphic behavior, thus adding an extra layer of flexibility.
- Developer assistance: For debugging purposes, as well as for enhancing overall code readability, being capable of determining an object’s class at runtime can provide substantial pro-development convenience.
Despite these benefits, there are certain inherent limitations regarding the use of Java’s
getClass()
:
- Incompatibility with primitives: `
getClass
` can only be invoked on objects. Hence, trying to employ
getClass()
on primitive types will result in a compilation error.
- Null Pointer Exception: If you happen to call `getClass` on a null instance, a NullPointerException would occur immediately.
- Overhead: Fetching class details may add unnecessary overhead in some cases since it delivers extensive information about the object’s class.
It seems fitting to quote Jamie Zawinski here: “Some people, when confronted with a problem, think ‘I know, I’ll use regular expressions.’ Now they have two problems.” This humorously highlights that with every solution comes a new potential issue we should be aware of – indeed very much the case with using getClass() versus typeof.
For a deeper understanding, consider the official Java documentation and various online resources[1].
[1] Java Documentations Datatypes Reference.
Real-World Use Cases: Applying Typeof in Your Java 8 Code
The
typeof
operator is a term familiar to JavaScript developers, but it’s important to note that in Java, there isn’t a direct equivalent. However, Java offers its own ways to determine the type of an object at runtime. This functionality is frequently utilized in code and can be analyzed under several real-world scenarios in context of Java 8.
– **Polymorphism**: Often, we create method overloads that cater to different types of parameters. When we don’t know ahead of time what type of object will be passed, knowing at runtime can assist us in handling each type differently. For instance:
public void handle(Object obj) { if (obj instanceof Integer) { // handle Integer } else if (obj instanceof String) { // handle String } }
This example shows the use of
instanceof
, which in this case serves as our version of amounting to JavaScript’s
typeof
. The
instanceof
keyword checks if the provided object is an instance of the specified type or class.
– **Design Patterns**: Java Design patterns often involve interfaces and abstract classes where the concrete class implementation may vary. For instance, in Abstract Factory or Strategy Pattern, at runtime, we might want to check which specific implementation strategy is being used.
if (strategy instanceof ConcreteStrategyA) { // Specific implementation code }
In this scenario,
strategy
is an instance of an interface, and you have two or more implementations such as
ConcreteStrategyA
,
ConcreteStrategyB
. For checking currently used strategy implementation,
instanceof
is used.
– **Java Streams**: The introduction of lambda functions and functional programming constructs in Java 8 can make it tricky to discern the type of certain elements in complex pipelines. For instance, one might need to filter a stream based on type:
list.stream() .filter(obj -> obj instanceof Integer) .forEach(obj -> System.out.println(obj));
Here, for a list containing elements of various types, the code filters out only those of type Integer. Elements are differentiated and selected by using
instanceof
.
Remember the words of Steve McConnell from his book ‘Code Complete’, “Good code is its own best documentation”. Understanding how and when to use tools like
instanceof
effectively can help write effective and efficient code, thereby contributing to creating high-quality systems. Hence, while Java doesn’t provide a direct equivalent to
typeof
, the clever application of the
instanceof
comes in handy in a series of practical applications.
The
typeof
operator is not present as a dedicated feature in Java 8, given that the language exhibits static typing. Throughout its evolution, from inception to the current version, Java has consistently remained a strongly typed language – meaning, every variable and expression has a type that is known at compile time.
The absence of a direct equivalent to JavaScript’s
typeof
implies the need for alternate methods to determine a variable’s class or type. One such method is the
instanceof
keyword. The
instanceof
operator, which checks if an object is an instance of a particular class or interface, can serve as an indirect means to determine a type in Java.
Here’s how you can do this:
java
Object obj = “Hello world!”;
if (obj instanceof String) {
System.out.println(“It’s a string!”);
} else {
System.out.println(“It’s not a string.”);
}
In this code snippet, `obj` is an instance of the String class, and thus
"It's a string!"
will be printed to the console.
However, it’s important to note that this isn’t a perfect match to JavaScript’s
typeof
. It doesn’t return a string specifying the type; rather, it returns a boolean indicating whether or not the object is an instance of the specified class or interface.
An alternative way is to use the
getClass()
method which can provide information about the class of the object.
java
Object myObject = new String(“Java 8”);
System.out.println(myObject.getClass().getName());
Here,
getClass().getName()
function will output `java.lang.String`.
Your choice between these techniques mainly depends on the specific needs of your program. The
getClass()
method provides a literal answer, while
instanceof
tests for a specific superclass or interface, offering a more semantic interpretation of types in the context of your application.
As Per Martin Fowler, an influential figure in software engineering, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This emphasizes on the importance of writing code that promotes readability and understanding, hence supporting effective collaboration among developers.