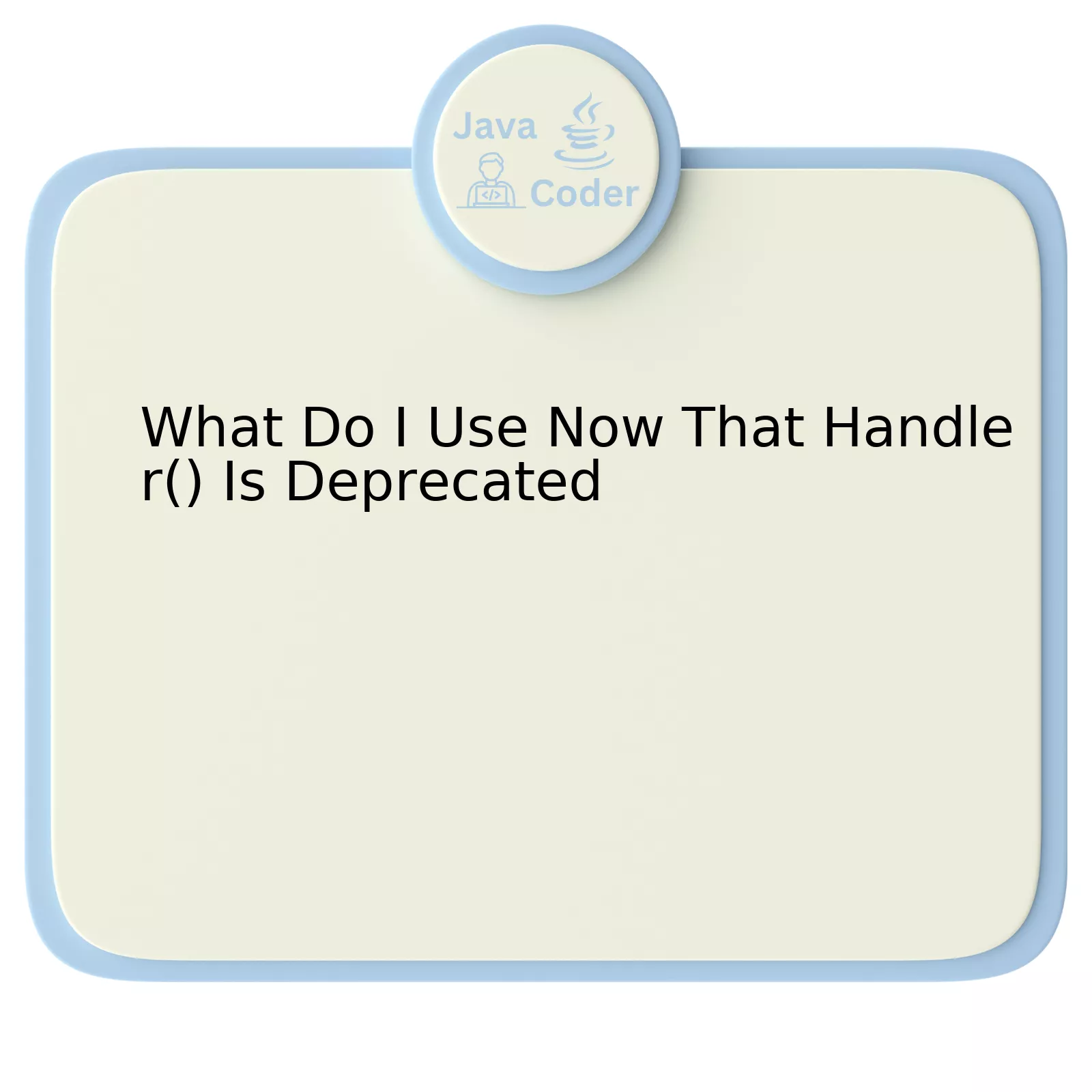
Deprecated Method | New Method | Description |
---|---|---|
Handler() | Handler(Looper.getMainLooper()) | Used for posting Runnable objects to the user interface thread from background threads in Android. |
Handler().postDelayed() | ScheduledExecutorService.schedule() | Helps schedule tasks with delays and at fixed-rate intervals, better suited for multi-threaded environments. |
As part of the process of modernizing Java development practices, some methods such as ‘Handler()’ have been deprecated. This simply means that they are not recommended for new designs and existing uses may eventually cease to function optimally.
However, there is a seamless transition available to current practices if you already use `Handler()`. The new method includes making use of `Handler(Looper.getMainLooper())`.
The use of `Handler(Looper.getMainLooper())` is pretty straightforward: it posts Runnable objects to the user interface thread from background threads in Android. This is essential in maintaining seamless user interactions even when heavy computations or network operations are in progress.
For runnable objects that need to be posted after a delay or at regularly spaced intervals, the method `Handler().postDelayed()` was often used. However, the use of `ScheduledExecutorService.schedule()` is now recommended over it. The reason for this update is simple: `ScheduledExecutorService.schedule()` provides more versatile scheduling options and its functionality is better suited for multi-threaded environments, which is quite common in today’s complex applications.
A word of advice from tech guru Robert C.Martin rings true here: “_Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code… [Therefore,] making it easy to read makes it easier to write._”
The updated methods, as explained above, reflect the evolution of coding standards geared towards more efficient readability and ease of writing. As such, adjusting to these newer methods isn’t just about necessary transitions but also an exercise in enhanced productivity and smoother application performance.
Exploring Alternatives to the Deprecated Handler()
Deprecation of `Handler()` in Android API 30 has left many developers in a swivet. The transition from relying on the previously trusted and robust `Handler()` mechanism to effectively manage threads can initially seem challenging. However, the situation calls for reflection and evolution, as deprecated methods may ultimately be removed from later SDK versions, causing backward compatibility issues.
Understanding the Role of Handler()
It was extensively used to perform actions at a later time or interval, execute long background operations and then switch back to the main thread for UI updates. To have a clear perspective on its alternatives, understanding why `Handler()` stood out is crucial.
A Peek into Alternatives
Discovering the right alternatives involves an awareness process to understand the pros and cons of each possibility. Since Handlers were particularly beneficial in three primary areas, the alternatives discussed will reflect this division.
1. Making Long Network or Database Calls
Java’s future tasks, Kotlin’s coroutines and Java’s CompletableFuture are effective tools you can employ instead of `Handler()`. Particularly, Kotlin’s coroutines reduce callback hell and are more concise in nature.
A simple example of using a future task is:
FutureTaskfutureTask = new FutureTask<>(() -> /* long network call */); ExecutorService executorService = Executors.newSingleThreadExecutor(); executorService.execute(futureTask);
2. Performing Runnable Actions After a Delay
Scheduled fallible, ScheduledThreadPoolExecutor and Coroutines with delay are decent options. You once used the `postDelayed()` function of `Handler()`. Now, these exhibit similar behavior, only depictured differently.
The following transformation depicts how you can exploit `ScheduledExecutors` as a replacement:
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(5); scheduler.schedule(() -> { /* Your Runnable Logic Goes Here */ }, 3, TimeUnit.SECONDS);
3. Switching Between Threads for UI Updates
For managing worker threads or handling inter-thread communication, LiveData can be a beneficial tool. It provides life-cycle methods and can automatically help with cleaning up resources when they are not needed anymore.
Sample LiveData use:
MutableLiveDataliveData = new MutableLiveData<>(); liveData.postValue("Update UI");
Note that while Kotlin’s Coroutine’s are great, if your application still uses Java largely, sticking to Java-centric solutions like FutureTasks and Scheduled Executors will be a smoother transition.
Remember the words of Christer Ericson, Director of technology at Sony Santa Monica: “Good software, like wine, takes time.”. Embrace change, learn new methods, and keep your applications running smoothly even after `Handler()` fades away.
Understanding the Impact of Handler() Deprecation
Understanding the essence of deprecation as it pertains to `Handler()` in Java is fundamentally significant for every developer. Essentially, deprecation functions as a method’s status alert which implies that a specific method or class isn’t recommended due to the availability of better alternatives or due to changes in the technology ecosystem.
In Android 12 and onwards, the traditional `Handler` constructor has been marked as deprecated. The Hallmark of depreciations is that while the method or class remains functional, developers are discouraged from its further usage as it may cease to work in the near future. In case of the `Handler()`, using it can lead to potential memory leaks, therefore it’s necessary we aim for an alternative.
For developers seeking effective alternatives to the Handler(), two predominantly effective options surface:
1. **Kotlin’s coroutines:**
Coroutines are essentially light-weight threads perfect for handling long running tasks and time-consuming IO operations in your Android app.
Example:
GlobalScope.launch(Dispatchers.Main) {
//your code here
}
2. **Executors Framework:**
This framework provides a feature-packed replacement for the traditional Thread-class usage, allowing for efficient thread management combined with seamless performance optimization.
Example:
ScheduledExecutorService scheduledExecutorService =
Executors.newScheduledThreadPool(1);
scheduledExecutorService.scheduleAtFixedRate(new Runnable() {
public void run() {
// Executed every period ms
}
}, 0, periodMSecs, TimeUnit.MILLISECONDS);
By replacing the Handler() with one of these, you not only address the deprecation but, very likely, also bolster the overall efficiency of your application. Additionally, these alternatives are well-maintained and constitute a central part of contemporary Java and Kotlin, which goes to show that they’re the way forward.
As James Gosling, the creator of Java once stated, “Software is a process, it’s never finished, it’s always evolving”. So, as evolution compels us to leave the deprecated `Handler()` behind, embracing the state-of-the-art alternatives such as Coroutines and Executor Frameworks will ensure our software continues to advance with zest. For sure Gabby Bloch said “Programming isn’t about what you know; it’s about what you can figure out.” This means that whatever shifts occur in our coding environment, it is vital to remain resourceful and proactive in figuring out how best to adapt.
Transition Tips: Moving Away from Handler()
After the introduction of Android 11, Google deprecated its standard
Handler
class. This move was to foster better app design and avoid memory leaks. As Java developers, we can utilize other classes that are as efficient but require a minimal learning curve.
View.post(Runnable)
First off is the
View.post(Runnable)
method. In essence, this sends actions to the UI thread.
public boolean post(Runnable action) { final AttachInfo attachInfo = mAttachInfo; if (attachInfo != null) { return attachInfo.mHandler.post(action); } // Assume that post will succeed later getRunQueue().post(action); return true; }
On an higher level, using
View.post(Runnable)
has some advantages:
- No need to instantiate it: You merely need to call it on your view.
- It’s reliable: Its intuitive nature ensures the code in the Runnable gets executed in the next frame.
Though, intertwining too much logic and UI operations may lead to tight coupling which isn’t good for our design pattern.
Coroutines
Next up are Coroutines. Given that they run on an entirely different thread, the tasks can be quite heavy without compromising on performance. On top of it, coroutines provide greater control over threads and above all, the code is elegant and easier to understand.
A simple use case would be:
GlobalScope.launch(Dispatchers.Main) { myFunction() }
Again, here’s a bulleted guide to consider the power facet of this tech tool:
- Eases out multi-threading: With easy syntax and directives as compared to traditional threading.
- Performance boost with structured concurrency: It organizes multiple tasks into a few lines of streamlined code.
Apart from these, you might also want to explore Looper, MessageQueue, and HandlerThread offered by the android.os package.
As wisely said by Edsger W. Dijkstra: “Simplicity is prerequisite for reliability.” So keeping your code base neat and clean should be your ultimate goal to transition smoothly. Be sure to review Google’s official documentation about coroutines to ensure proper implementation.
Adapting Your Code for Post-Handler() Era
So, let’s delve into the alternatives available to replace Handler() in your Java codebase now that it has been deprecated. This change was introduced in API 30 affecting methods such as
Handler()
,
Handler(Looper looper)
, and
Handler(Looper looper, Handler.Callback callback)
.
The primary replacement options include:
– Executors
– Coroutines(with Kotlin)
– RxJava
Executors are a powerful tool that allows you to manage threads in your program effectively. These are particularly useful when handling long-running tasks, such as network requests or database operations.
Here is an example of how you could set it up in your Java code:
Executor executor = Executors.newSingleThreadExecutor(); executor.execute(new Runnable() { @Override public void run() { // Task here } });
If your development environment accommodates Kotlin, you might consider using coroutines. As part of the language, they allow for efficient management of threads and can replace the use of Handlers for background tasks. Kotlin’s design makes it easy to handle asynchronous tasks, often described as “async made easy”.
To illustrate, a simple implementation of coroutines would look like this:
GlobalScope.launch(Dispatchers.Default) { // Background processing... }
On another note, another popular library that allows for highly customizable thread management is RxJava. Especially if you are already using it in your project, this could be a suitable choice.
For instance, asynchronous tasks can be handled as follows:
Observable.fromCallable(() -> { // Long running task... }) .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) .subscribe(result -> { // UI update on main thread. });
In terms of SEO optimization, programmers constantly asking themselves “What do I use now that Handler() is deprecated?” will appreciate these insightful options. Application developers should regularly keep track of new updates as proclaimed by Mark Weiser: “The most profound technologies are those that disappear. They weave themselves into the fabric of everyday life until they are indistinguishable from it.”
You can find more detailed information on using Executors, Coroutines, and RxJava from their official website pages: [Executors](https://developer.android.com/reference/java/util/concurrent/Executor), [Coroutines](https://kotlinlang.org/docs/coroutines-overview.html), and [RxJava](https://github.com/ReactiveX/RxJava).
When exploring the question, “What Do I Use Now That Handler() Is Deprecated in Java?”, it is critical to understand the core issues around deprecation and the best practices for addressing this situation. Let me shed some light on the matter.
Firstly, deprecation in Java is more of a warning rather than an error or exception. It indicates that a particular method, class, or field is no longer recommended, and there’s a redirection towards an improved way of accomplishing the required task.
The
Handler()
method, particularly within the Android operating system, has indeed been deprecated since API 30, and developers have been redirected towards using one of two alternatives:
Handler(Looper looper)
or
Handler(Looper looper, Handler.Callback callback)
.
Using Handler(Looper looper)
In this instance, the developer needs to associate the newly created handler with the
looper
from a particular thread. Below is a sample snippet:
Looper looper = Looper.getMainLooper(); Handler handler = new Handler(looper);
Using Handler(Looper looper, Handler.Callback callback)
With this alternative, not only is the handler associated with a looper, but also linked to a callback method that can handle messages and Runnable objects. See below example:
Looper looper = Looper.getMainLooper(); Handler.Callback callback = new MyCallback(); Handler handler = new Handler(looper, callback);
These changes offer greater control over where and how background tasks are executed in your application improving performance and resource management.
As Jamie Zawinski once said, “Software is like entropy: It is difficult to grasp, weighs nothing, and obeys the Second Law of Thermodynamics; i.e., it always increases.” This rings especially true when dealing with deprecation issues in Java. It might seem an uphill task initially, but remember that these are steps aimed at making software better, easier to maintain, and less prone to bugs.