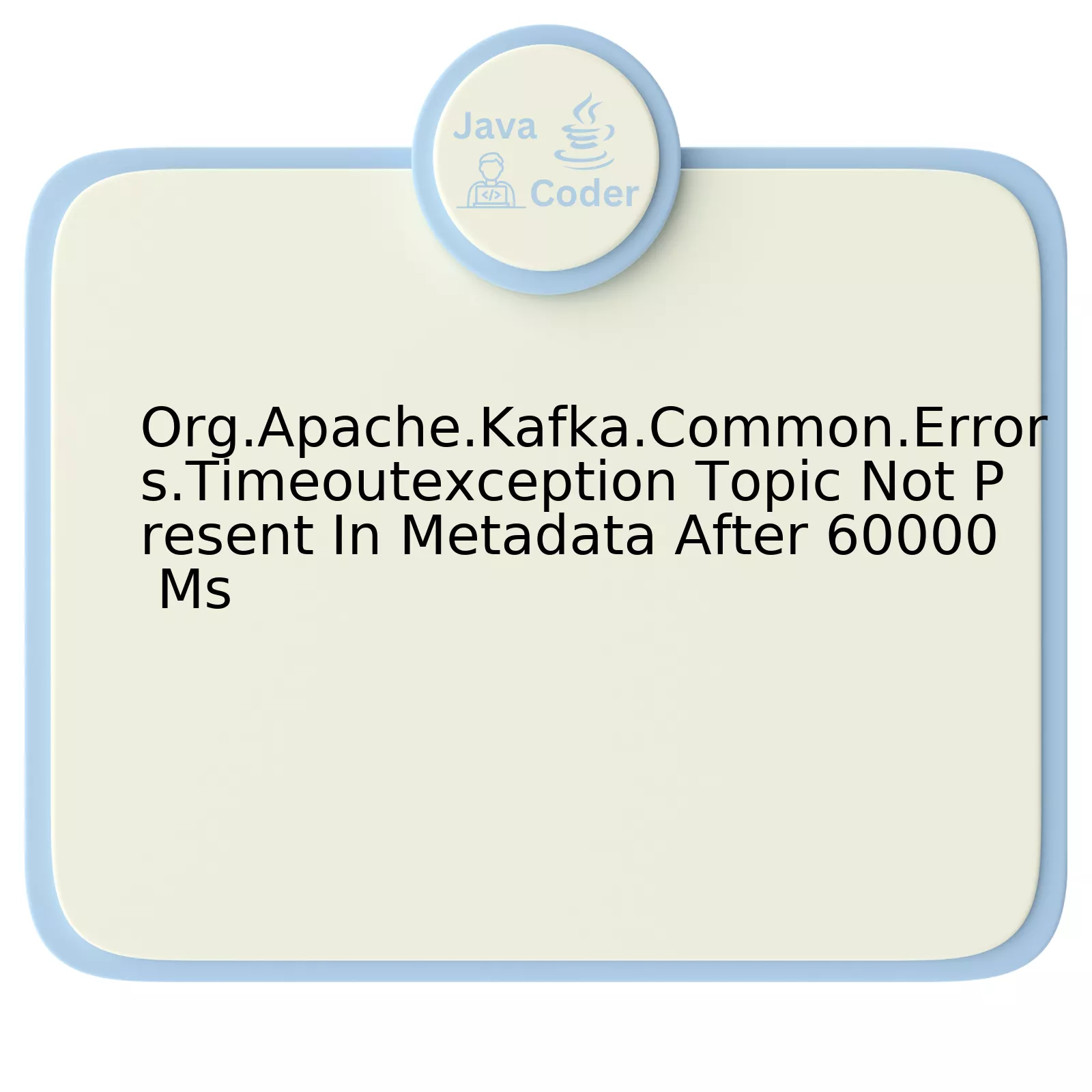
According to the Apache Kafka documentation, the class org.apache.kafka.common.errors.TimeoutException occurs when a request times out. A frequent manifestation of this error is the scenario wherein a specific topic is not present in the metadata after 60000 milliseconds.
To delve further into this specific issue, let’s analyze it through the following detailed data breakdown:
Error | Message | Potential Causes | Suggested Solution(s) |
---|---|---|---|
org.apache.kafka.common.errors.TimeoutException | Topic not present in metadata after 60000 ms. | The specified topic does not exist, overload in broker, slow or inadequate network connection. | Create the topic if it doesn’t exist, balance load across brokers, optimize network connections. |
The server sends metadata, including a list of existing topics, to clients as part of its regular processing cycle―which typically completes within microseconds. When a topic doesn’t appear in the metadata after such an unusually long period (60000ms), a `TimeoutException` is usually thrown.
This may typically be caused by:
– The specified topic not existing. If the producer is attempting to connect to a non-existent Topic, this `TimeoutException` will be raised.
– An overloaded broker. If the Kafka heavyweight processes become saturated due to an influx of messages, some Topic updates may lag—resulting in a `TimeoutException`.
– Insufficient or unstable network connection. Though less common, network performance can impact how fast metadata updates are communicated between the Kafka broker and client.
To address the `TimeoutException`, consider the following approaches:
– Confirm that the intended topic exists. You might need to create it manually depending on your auto-topic-create settings.
– Balance the load across the available Kafka brokers to manage any overload situation effectively.
– Assess and improve your network connectivity and stability where necessary to ensure timely transmission of metadata between the broker and client.
As Niklaus Wirth, a Swiss computer scientist noted: “Clearly, programming courses should teach methods of design and construction, and the selected examples should be such that a gradual development can be nicely demonstrated.” This applies directly to managing issues like `TimeoutExceptions`; understanding the root cause is crucial for effective resolution.
Understanding the Org.Apache.Kafka.Common.Errors.Timeoutexception Error
The
org.apache.kafka.common.errors.TimeoutException
error is prevalent when dealing with Apache Kafka, a scalable and high-performance messaging system widely used for real-time data processing. When programmers encounter the error “Topic not present in metadata after 60000 ms,” it highlights a persistent problem occurring primarily during the communication between the producer and broker.
The focal point of attention should be understanding what this particular error message stands for:
The Underlying Problem: Topic Not Present In Metadata After 60000 Ms
Kafka clients communicate with brokers for fetching metadata about topics, partitions, leaders, replicas, etc. For example, a Producer sends a metadata request to the broker for locating a topic’s partition leader before sending messages. Post the request; the client waits for a determined time period before it times out if there isn’t required response (specified via the ‘max.block.ms’ property). If the topic isn’t present in the metadata even after this waiting period, the above error occurs. In most scenarios, this results due to ‘auto.create.topics.enable’ being set to false on brokers or issues in the network leading to prolonged responses.
Dealing with the Exception:
To discern why the
org.apache.kafka.common.errors.TimeoutException
error appears, we need to investigate several aspects:
1. Auto Topic Creation: First, verify if the feature ‘auto.create.topics.enable’ is set to true on the brokers. If it’s false, topics will not be automatically created, leading to this error if trying to produce to a non-existent topic.
2. Active Controller: Check to ensure an active Controller exists in your Kafka cluster. A Controller plays a critical role in managing and maintaining the status of partitions and replicas.
3. Network Latency: Slow network speeds might culminate in requests timing out due to stretched response times. Make sure the network infrastructure is working optimally.
4. Broker Health: Regularly monitor the health status of your Kafka broker. Poor performance of your Kafka broker might cause delays in responding to metadata requests.
As Robert C. Martin rightly said, “The function of good software is to make the complex appear to be simple.” Overcoming exceptions like ‘Topic Not Present In Metadata’, while initially appear complex, they can be simplified upon diving deep into its root causes and leveraging appropriate solutions.
This analysis accentuates the need for effective monitoring and careful configuration of producers, consumers, brokers, and other components when using Apache Kafka, reducing the likelihood of encountering such errors.
Implications of Topic Not Present in Metadata After 60000 ms
One of the common issues that a Java developer working with Kafka might come across is titled as “
org.apache.kafka.common.errors.TimeoutException: Topic not present in metadata after 60000 ms
“. This issue predominantly arises when a topic you are trying to access is not available.
Kafka revolves around the concept of topics, which are streams of records sharing the same type. When your application attempts to connect to a topic that doesn’t exist, it will hold up till the broker notifies it about this specific topic’s existence. The broker observes a default metadata update cycle of 10 minutes. This means, your application potentially waits for 10 minutes before receiving a response.
Let’s delve into the potential reasons behind why such an error occurs:
– Misconfiguration: Check if there’s a mismatch between topic names configured on the producer and consumer side. A commonly overlooked issue is case-sensitivity, where these topic names differ leading to such exceptions.
– Replication Issues: Another possible reason could be due to replication constraints. If the Kafka server cannot find ISR > 1, (ISR being in-sync replicas), it can lead to ‘Topic Not Present’ errors.
– Zookeeper Connectivity: Zookeeper plays a prominent role managing server state within Kafka. Connectivity or synchronization issues with Zookeeper nodes could trigger these errors.
– Incomplete Initialization: While initializing, if the server fails to load all topics within a pre-defined period, it might throw ‘Topic not present’ exception.
To troubleshoot and resolve such errors, following aspects could be scrutinized and acted upon:
– Verify topic configuration across producer and consumer side. Ensuring uniformity might solve the given issue.
– Monitor Zookeeper connection health by examining server logs for discovering any potential synchronization failures.
– Scrutinize server initialization logs to confirm if all topics were loaded successfully within the prescribed timeframe.
– Rebalance the Kafka cluster or increase the number of replicas based on analysis.
As Rachel Pedreschi quoted, “A distributed system is only as strong as its weakest link, so we need to put everything under scrutiny.” Dealing with complex distributed systems like Kafka requires a consistent optimization method, expert judgement, and continuous learning approach to minimize errors and performance issues.
Here is a simple code snippet to demonstrate how we check the existence of a topic in Java:
AdminClient adminClient = AdminClient.create(properties); ListTopicsResult listTopicsResult = adminClient.listTopics(); boolean exists = listTopicsResult.names().get().contains("topic-name");
In the above example, we utilize Kafka’s `AdminClient` API to fetch the list of topics, and then validate whether the required topic is present or not. All these Kafka APIs have proper exception handling mechanisms that should be extensively used to insulate the code from encountering abrupt errors.
Therefore, addressing the `
org.apache.kafka.common.errors.TimeoutException: Topic not present in metadata after 60000 ms
` isn’t simply about just fixing an error but also involves understanding the nuances of Kafka’s architecture and ensuring all components function reliably.
Resolving Timeoutexception: Techniques to Use When Topic Is Not Present In Metadata After 60000 ms
The `org.apache.kafka.common.errors.TimeoutException` often appears when a topic is not available in the Kafka metadata within the given period, typically 60000ms or 60 seconds. Such issues can emerge due to multiple reasons such as network problems, unresponsive Kafka servers or topic deletion/quarantining among others.
Preventive Measures & Resolutions:
– Increasing Timeout: You could consider increasing the metadata fetch timeout period. In some cases, it might be possible that the topic takes more time to reflect in the metadata due to server slowdowns or network delays. This modification involves a change in the consumer/producer settings. For instance, for a producer properties object, you can adjust the `max.block.ms` parameter as shown below:
Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("max.block.ms", 2000); //Setting timeout to 2 seconds
– Checking Topic Existence: Before attempting any operation on the topic like sending messages or subscribing, ensure that the topic does exist in your Kafka cluster. If the topic is deleted or inaccessible due to any reason, make sure to recreate it or debug the underlying issue first.
– Server Health: Keep an eye on the health of your Kafka servers. Any issues with the brokers can prevent it from updating the topic information promptly. Regular maintenance and checks can help avoid such situations.
– Network Issues: Network connectivity between the application and Kafka servers plays a critical role. Any disruptions or latency in this can lead to timeouts. Try to check for any potential network issues if you are facing consistent timeouts.
Quoting software engineer D. Richard Hipp: “All of computing is an infrastructure play. To win in the long run, you build the infrastructure”. Thus, maintaining a strong, reliable infrastructure – including network layers and servers – can go a long way both in preventing and resolving issues like the TimeoutException.
Sources:
Confluent Documentation – Producer Configurations
Apache Kafka Documentation – TimeoutException
Case Study Analysis: Timeoutexception Challenges – When Topic Fails to Show in Metadata After 60000 Ms.
When dealing with the `org.apache.kafka.common.errors.TimeoutException`, where the topic is not present in metadata after 60000 ms, you need to understand some key aspects. Primarily, this error is associated with Apache Kafka, a stream-processing software aiming to provide high-throughput, low-latency platform for handling real-time data feeds.
Error Context
This error typically pops up when one tries to produce or consume messages to or from a non-existent Kafka topic. In other words, if the targeted topic does not exist in the system and the property ‘auto.create.topics.enable’ is set to false in the broker configuration. So, the normal setting that helps in automatically creating the topic won’t work in our case.
Here’s an example of the error message:
TimeoutException: Topic testTopic not present in metadata after 60000 ms.
How To Solve This?
We approach the TimeoutException Challenge by considering the following practical solutions:
- Create Topic Manually: As a first solution, manually create the topic(s) on the Kafka server that your application will be producing to or consuming from. Manual control over your topics comes with its own benefits; like setting custom configurations (retention policy, number of partitions etc.) per topic.
- Re-configure Kafka Broker: An alternative solution would be to set ‘auto.create.topics.enable’ to true in the Kafka broker configuration. This allows the broker to auto-create topics whenever a client (producer/consumer) makes a request to a non-existing topic. It should however be used cautiously as it could lead to unintentional creation of Kafka topics due to typos in topic names etc.
Tom Preston-Werner, Co-founder of GitHub, once said, “When I’m working on a problem, I never think about beauty. But when I’ve finished, if the solution isn’t beautiful, I know it’s wrong.” Similarly, while dealing with these types of issues, we must employ a dynamic and proactive approach rather than trying out different methods indefinitely until it clicks. It’s important to know the causes of specific errors, so you can tackle them more directly and efficiently.
Visit the ‘Official Kafka Documentation‘ for more detailed information about the properties mentioned above, implementation tips, and best coding practices.
The aforementioned
org.apache.kafka.common.errors.TimeoutException
is an error that arises when a given topic isn’t present in Kafka’s metadata following certain stipulated time-frame which, in the discussed scenario, extends to be around 60000 milliseconds (or 60 seconds). This issue may arise due to several reasons linked with communication discord between Kafka producers, consumers and its brokers.
Possible Reasons:
* Internal communication network issues inside Kafka cluster setup can cause this exception. As Kafka employs Zookeeper for managing various tasks such as maintaining metadata for topics and brokers, any disruption within the Zookeeper service could result in the TimeoutException.
* Another reason is related to incorrect consumer configuration. If the configuration is incorrect or incompatible, it might lead to issues like delay in updating the metadata resulting in timeout.
* Lower broker thread count could also generate this exception. Each Kafka broker uses threads for communication with producers and consumers. If the assigned thread count is insufficient to handle the vast network traffic, it can lead to delayed responses eventually triggering a timeout.
As part of the solution, an initial step would be to validate the network connectivity and health of the complete Kafka infrastructure including Zookeeper. Moreover, proper implementation strategies must guide configuration settings to ensure optimal producer-consumer communication. A balance should be maintained on partition counts for each topic based on client application’s need for parallelism. Furthermore, augmenting broker thread count according to workload could be beneficial.
Referencing a quote by Robert C. Martin, “First do it, then do it right, then do it better,” underscores the importance of iterative refinement when developing software or troubleshooting complex systems. In the context of our discussion, understanding Kafka’s ecosystem, auditing its setup, and performing necessary adjustments are part of “doing it right” after encountering the
org.apache.kafka.common.errors.TimeoutException
.
For more details on Apache Kafka and its common errors, you can visit the official Apache Kafka Documentation.