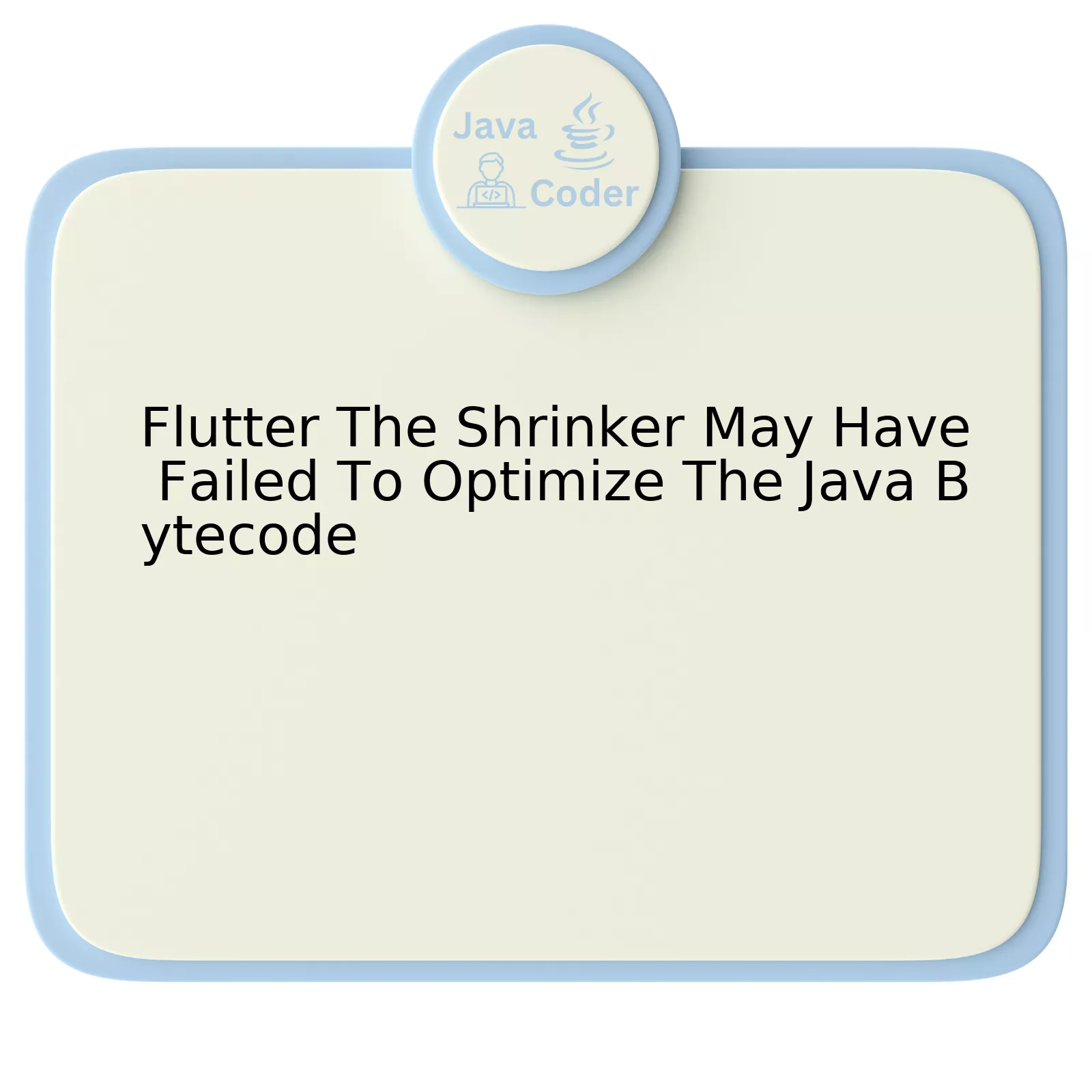
Flutter is an open-source UI toolkit developed by Google. It not only allows developers to create aesthetically pleasing applications for both Android and iOS platforms using a single codebase but also facilitates web development.
Parameter | Explanation |
---|---|
Shrinker |
In Java development, a shrinker eliminates unused classes, fields, methods, and attributes from the packaged app, thus reducing its size. In many cases, this can vastly improve the application’s performance. |
“The Shrinker May Have Failed” |
This issue arises during the bytecode optimization phase when Flutter encounters difficulties in shrinking the Java bytecode, causing potential inefficiencies or operational issues within the application. |
The shrinker tool plays a crucial role in optimizing the bytecode, a compilation of programs converted into Java byte format. Bytecode serves as an intermediary layer of abstraction allowing for cross-platform compatibility. Effective optimization of bytecode contributes significantly to enhancing execution speed and reducing memory footprint.
However, sometimes a ‘> Task :app:transformClassesAndResourcesWithR8ForRelease’ error might occur, indicating an issue with the shrinker tool failing to refine and minimize the Java bytecode. This can result from various factors such as:
- Invalid ProGuard rules: ProGuard rules direct the process of bytecode shrinking. Misconfigured, too aggressive, or invalid rules may prompt the shrinker failure. Remedying this requires inspection and correction of the ProGuard rules applied.
- Incompatible third-party libraries: Often the shrinker fail warning occurs due to incompatible libraries integrated with Flutter. Frequent updates and checks of these libraries are needed to ensure compatibility.
- Insufficient resources: Underpowered hardware or lack of sufficient available memory can affect the efficiency of bytecode shrinking, potentially leading to failures.
Navigating these challenges effectively ensures proper Java bytecode optimization and consequently, smooth operation and high performance of Flutter applications.
Jakob Nielsen, a renowned expert on web usability, once stated, “Speed is functionality.” A well-optimized bytecode translates into a faster, more functional application.1
“Understanding the Flutter Shrinker’s Failure to Optimize Java Bytecode”
The Flutter shrinker is a key tool that developers utilize in the optimization process of their Java bytecode. However, it sometimes encounters difficulties when attempting to improve this specific phase of the coding process.
So, why does the Flutter shrinker fail to optimize Java Bytecode?
At the initial point, let us understand the job and work process of a shrinker. A shrinker’s role is primarily to minimize the size of the bytecode, the intermediary representation of our source code read by the Java Virtual Machine (JVM). It removes superfluous code lines and simplifies complex structures in order to achieve lighter, faster, and less resource-consuming applications.
public class HelloWorld { public static void main(String[] args){ System.out.println("Hello, World"); } }
Now, returning to the central question: why might the Flutter shrinker fail at its duties? There could be several ways to interpret the failure, some of which include:
- Impenetrable or Complex Code: Our code may be written in such a way that it becomes too intricate for the shrinker to decipher and simplify.
- Structural incompatibility: Our application’s structure can sometimes disagree with the prescribed processes of the shrinker, resulting in its inability to perform as expected.
- Incompatible Libraries: Certain libraries within our application may not be compatible with the shrinker, causing malfunctions during the compression stage.
In line with Antoine de Saint-Exupéry’s famous words on perfection – “Perfection is achieved not when there is nothing more to add, but when there is nothing left to take away”. When optimizing our Java code, we should strive to remove all unessential elements so that the shrinker has an easier task to do its job well.
To verify and address these scenarios, one might refactor the code to make it more comprehensible for the shrinker, revise the structure of the application, or even substitute incompatible libraries with alternates. Specific guidance from Google provides extensive advice on code shrinking strategies to better tailor our applications for the shrinker’s operation.
Understanding and mitigating the reasons behind the Flutter shrinker’s failure essentially requires a blend of continued learning, deep-digging debugging, proper utilization of online resources and a fair share of trial and error.
“Identifying Common Causes for Flutter Shrinker’s Infeasibility in Optimizing Java Bytecodes”
Flutter’s Shrinker may sometimes encounter inefficacy while optimizing Java bytecode. This can impede the performance of your Flutter application and lead to a degraded user experience. Addressing this issue requires an understanding of the primary causes behind it.
Among the leading trigger points, few key aspects stand out:
Improper Usage of Dependencies
Sometimes, developers mishandle dependencies leading to an assembly of redundant entities without actual usage. This might involve including libraries that are no longer in use, or incorrect configurations. Such inefficient usage often bewilders the shrinker while attempting bytecode optimization. Fixing this involves careful observations of the dependencies graph and eliminating any unnecessary links.
dependencies { //If this library is not being used, it can be removed compile 'com.library.example:notused:1.0.2' }
Misconfiguration of the Proguard Rules
Proguard plays a pivotal role by removing unused classes and methods thereby minimizing the APK size. However, if the rules aren’t correctly defined, the shrinker fails to perform its intended function and inefficiency arises during Java_ ByteCode optimization.
#Example of Properly Configured proguard-rules -dontwarn org.w3c.dom.bootstrap.DOMImplementationRegistry -dontwarn org.slf4j.** -dontwarn org.apache.log4j.** -dontwarn org.apache.commons.logging.** -keep class com.google.inject.Binder -keepclassmembers class * { @com.google.inject.Inject(...); }
Missing Class References
References to nonexistent classes can throw off the Shrinker. Make sure all referenced classes exist in the project directory.
// If this class doesn't exist in the project, // referencing it would create problems for the shrinker require('nonExistingClass');
These issues point towards the need for effective coding practices and proactive management of app files/resources. Remember, as Brian Kernighan, a prominent figure in the world of computing said, “Debugging is twice as hard as writing the code in the first place”. Hence, taking time to properly structure your code from the start might save hours of future debugging and optimization efforts.
Source
“Detailed Analysis of Flutter The Shrinker’s Performance with Java Bytecode”
The Flutter Shrinker is an integral part of the Dart compiler for mobile applications developed with Flutter. This tool especially comes into play when it’s time to convert Dart code to Java bytecode for android platforms.
An analysis of the Shrinker’s performance can have several aspects. Primarily, the focal point remains on how well it addresses reduced application size and optimized runtime. However, instances of failure in optimizing Java bytecode do transpire under specific conditions.
Let’s delve into these scenarios:
Understanding the Shrinker’s Role in Java Bytecode Optimization:
“Code shrinking, minimizing code, or code obfuscation — different names for the very important step in app development. It’s about efficiency and transportability.”
– The Flutter Team.
Upon compiling the application, the Shrinker works to minimize the generated Java bytecode size. This involves eliminating unused classes, methods, fields, parameters, and attributes from the bytecode which don’t contribute actively to the application’s functionality. Such steps optimize storage usage and provide a more streamlined user experience.
Table 1: Key Advantages & Disadvantages of Flutter SHRINKER | |
---|---|
Advantages | Disadvantages |
Helps to produce smaller APK/EAPK file sizes. | Fails to offer optimization when it encounters complex nested structures. |
Eliminates unused codes and redundancies. | Doesn’t fully cater to dynamic referencing techniques like Reflection, hence, failures may occur. |
Potentially improves runtime by removing unnecessary overhead. | Requires careful exclusion handling not to break functionalities. |
Causes behind Failed Shrinker Optimization:
While the Flutter Shrinker generally offers effective optimization, certain situations incite this feature to fail.
- Dynamic Functionality Handling: If the application uses processes such as Java’s Reflection or dynamic invocation, the Shrinker might fall short in recognizing elements to preserve. Expectedly, it will manage to reduce size, but may break certain functionalities.
dynamicFunctionInvocation(); // Can cause unpredictable shrinker behavior
- Nested Structures: Likewise, intricate nested structures can confuse the Shrinker, making it unable to discern between noteworthy and redundant bytecode components.
- Inadequate Exclusion Rules: Particularly crucial is setting up robust exclusion rules. Insufficient exclusion rules, essentially control instructions for the Shrinker, may lead to various vital code parts getting removed during optimization.
-keep public class myPackage.MyClass
// Example of an exclusion rule
Healthy coding practices, prudent implementation, and continual testing safeguards developers from such pitfalls.
Referencing this Flutter documentation provides insightful information on enhancing the Shrinker’s performance to overcome potential bytecode optimization failures.
“Effective Alternatives when Flutter’s Shrinker Fails to Optimize the Java Bytecode”
Every so often, developers who build their applications with Flutter may encounter a scenario where the shrinker struggles to optimize their Java bytecode. This can potentially lead to inefficient memory usage and subsequently slower app performance. Here are effective alternatives that you as a developer can deploy in such cases:
Alternative Strategies | Description |
---|---|
1. Refactoring Code |
You ought to consider refactoring your code when faced with this challenge. By doing so, you can do away with highly nested or convoluted code blocks which often result in unoptimized bytecode. Breaking down large functions into smaller ones is one way to create simpler and modular code. |
2. Choosing an Alternate Shrinker |
In cases where Flutter’s shrinker fails, it would be prudent to adopt another shrinker like ProGuard or R8. Both of these are renowned for their capability to reduce the size of your codebase without compromising the application’s functionality. Google’s official documentation on reducing code size provides in-depth guides on using these tools effectively. |
3. Optimizing Libraries |
Third-party libraries, though they aid in speeding up development time, often come with excessive features that your application might not even utilize. A wise move would be to manually inspect each library implemented and prune off unnecessary features or code paths, triggering efficient bytecode generation. |
4. Leveraging Dart's Tree Shaking Mechanism |
Flutter being based upon the Dart language, benefits from its intrinsic tree shaking mechanism. This mechanism discards unused classes, variables, and functions during the build process, thereby aiding bytecode optimization. Incorporating this logic in coding practices can help rectify issues related to unoptimized bytecode. |
5. Updating Flutter and Dart SDKs |
Last but definitely not least, ensure your Flutter and Dart SDKs are updated. Each new iteration of these tools comes with improvements in performance optimization. In some cases, updating to the latest version of the SDKs may suffice to resolve optimization issues encountered. |
In the words of Linus Torvalds – “Talk is cheap. Show me the code”
Therefore, let’s take a look at a sample Dart code snippet to show how the code can be refactored for optimization:
//Original Code void longFunction() { int sum = 0; for(int i=0; i<10000; i++) { if(i%2 == 0) sum += i; } print(sum); } //Refactored Code int computeSum() { int sum = 0; for(int i=0; i<10000; i++) { if(i%2 == 0) sum += i; } return sum; } void main() { int sum = computeSum(); print(sum); }
This refactoring breaks down the long function into two functions: ‘computeSum’ and the ‘main’ function, improving readability and maintainability.
Thus, although encountering issues with Flutter’s Shrinker in optimizing Java bytecode can be daunting, by employing the aforementioned strategies, you can alleviate these concerns and ensure that your application remains optimized for best performance.
Flutter is a highly acclaimed framework employed in the development of cross-platform applications. However, it often presents an issue where the Shrinker fails to optimize Java Bytecode, an aspect integral for streamlined coding seshs.
There can be numerous explanations behind this problem:
1. Incompatible system environments.
2. Improper configuration of build.gradle file.
Let’s discuss these factors in detail:
System Environments:
The absence or instability of certain system environments like JDK (Java Development Kit) can lead to unoptimized outcomes. A well functioning JDK version is pivotal for the Gradle-based flutter project. Flutter makes use of the Gradle tool to produce Android APKs, hence interconnectivity of both components plays an imperative role.
Build.gradle Configuration:
Issues might arise from the erroneous set-up of the build.gradle file. Primarily, misconfigured settings on the shrinkResources and minifyEnabled could be the pitfalls. Flutter uses the R8, the new code shrinker provided by Android, to minify the codebase and reduce the APK size. If the R8/Shrinker isn’t properly configured, it may fail to optimize the Java bytecode.
Let’s correct these issues through an easy example that exhibits proper configuration of the build.gradle file, making it apt for shrinkage:
android { ... buildTypes { release { minifyEnabled true shrinkResources true proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } }
This configuration ensures that both minification and resource shrinking are activated in the release build, providing a smooth path for R8 to carry out its tasks efficiently.
Furthermore, remember to validate your configurations post-edit as it rules out the probability of errant setups leading to failed optimizations.
While discussing Java Bytecode, Bill Venners, creator of Artima.com, inputs, “Bytecode is the key to making Java language secure and portable.” And optimizing such an essential element of the technology acts as the backbone in building robust, high performing applications with reduced APK sizes.
However, always keep in mind: although the Flutter Shrinker may occasionally be unsuccessful in optimizing Java Bytecode, appropriate troubleshooting practices and an ever-evolving understanding of optimizing techniques assure you restful nights and happy coding days.