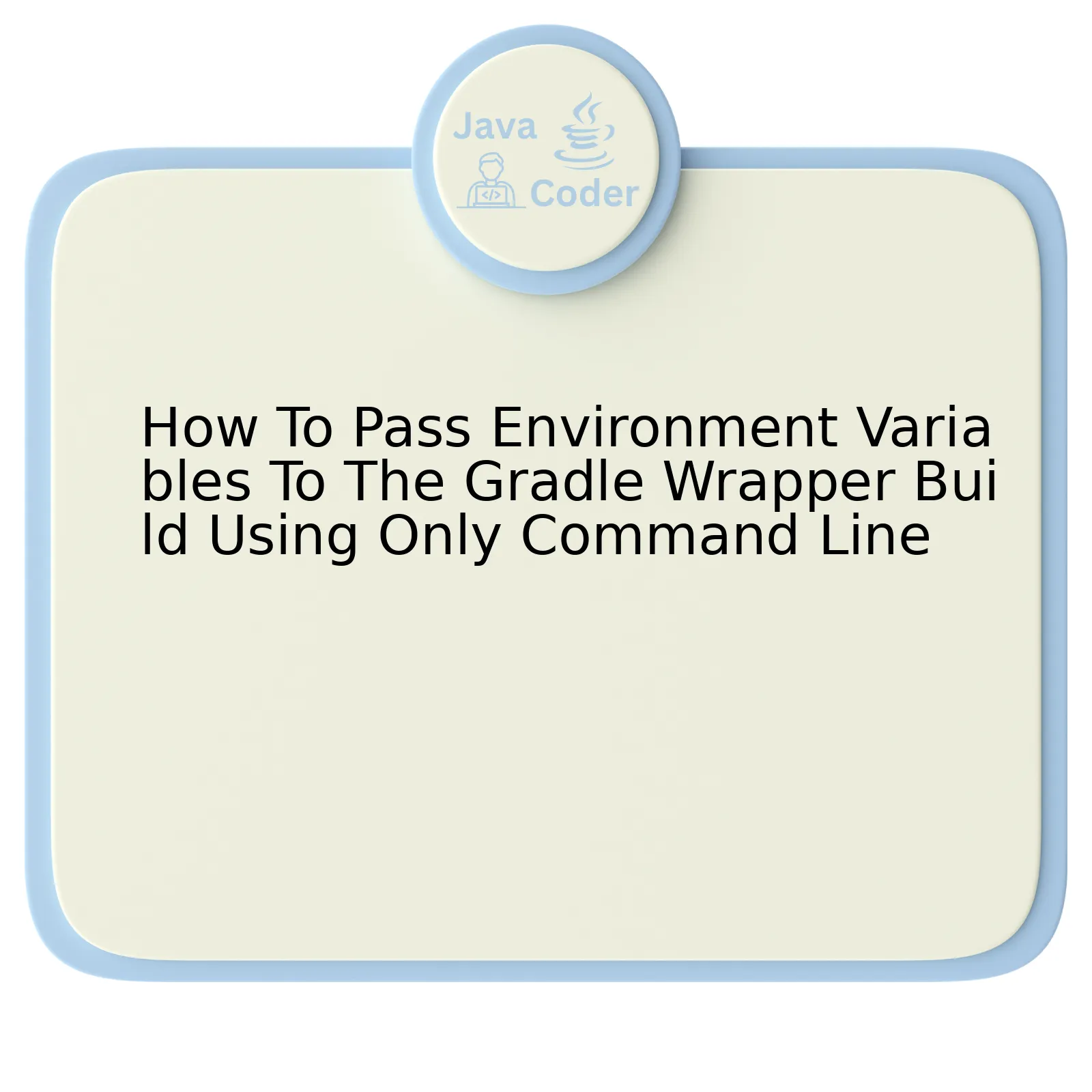
Passing environment variables to the Gradle Wrapper build using only the command line boils down to understanding and effectively utilizing two key components: Environment Variables and The Command Line. Below is a detailed walk-through, dividing the overall process into three distinct steps.
Firstly, let’s analyze the components through a simplified
table
:
Components | Description |
---|---|
Environment Variables | Variable values set in your system’s environment that can be used to customize the behaviour of your build. |
Command Line | A text-based interface that allows interaction with your computer via commands. |
1. **Setting up Environment Variables**
To pass an environment variable via command line to the gradle wrapper, you first need to define that variable in the system’s environment settings.
SET VAR_NAME=value
Replace `VAR_NAME` with your selected variable name (e.g., DATABASE_URL, SECRET_KEY), and replace `value` with the corresponding value for each variable.
2. **Passing Variables via Command Line**
Once done with setting up the variable, it’s crucial to express this newly created variable as a Java property in the command line while executing the Gradle Wrapper. Here’s how you can do it:
./gradlew -Dorg.gradle.java.home="%VAR_NAME%"
Replace `%VAR_NAME%` with your specific variable name to ensure effective implementation. This line tells the Gradle wrapper to run using the value of the variable as the Java home directory.
3. **Validating the Execution**
Ultimately, it’s good practice to ascertain whether the executed command produces the desired outcome. One such check would be to print the value of the Java Home directory.
./gradlew printJavaHome
Running the above command would confirm if the Java Home directory has been set to the value specified in the environment variable.
By employing the direct manipulation of the command line in tandem with environmental variables, you’re granted both increased control over your Gradle build process, and heightened adaptability within your development environment.
In words of Linus Torvalds, “[Software is] largely a service industry operating under the persistent but unfounded delusion that it is a manufacturing industry.” Hence, building your application with tools like the Gradle wrapper via command-line offers the versatility to efficiently manage different environments and configurations, thereby maximizing the service value of software development.
Maximizing Efficiency with Gradle Wrapper Build and Environment Variables: A Comprehensive Guide
The Gradle Wrapper is an indispensable tool for developers who use Gradle as an essential part of their Java application’s build process. Structured to ensure consistent builds, the Gradle Wrapper automates the management of Gradle versions and can also conveniently work with build environment variables. Here, let’s delve into the steps on how to pass environment variables to the Gradle Wrapper build using only command-line.
Process for Passing Environment Variables
Environment variables can be passed directly to the Gradle script in the execution phase via the command line. This is achieved by appending the variable (wrapped in -P) to the end part of the Gradle command.
For instance, assume that we have a variable named
ENV_VAR
which should take the value “Testing”. The following command line operation shows how to pass it:
./gradlew build -Penv=Testing
Here, the
-P
flag specifies that an additional project property needs to be set up, right before performing the build task.
ENV_VAR
becomes an available property within the build context and can be called upon via
project.property('env')
.
Simply put, whenever there’s a need to pass an environment variable, follow these steps:
- Combine the
-P
flag with your desired environment variable.
- Use the composed project property inside your build script key areas.
Dynamically Setting Up Environment Variables
For situations that involve setting multiple and different environment variables dynamically during runtime or build phases, this can be achieved using a properties file. Check out the following example:
def env = hasProperty('env') ? env : 'development' def filename = "src/main/resources/${env}.properties" project.ext.set('custom_env', new Properties().load(new FileInputStream(filename)))
The code snippet above demonstrates a scenario where the build script anticipates for ‘env’ property to be provided. In instances where this isn’t given, it reverts to a default setting – in our case, ‘development’. The chosen environment dictates which properties file to load. These property values are then accessed at any point in the build file using
project.custom_env.propertyName
.
Our day-to-day coding experiences are laden with tasks relating to building, testing, and deploying applications in various environments. To quote Kent Beck “Any program is only as good as it is useful.” Employing strategies such as passing environment variables to Gradle Wrapper ensures maximized efficiency not only in software development but also in fine-tuning the deployment process.
Always link back to online references like Gradle Docs for more comprehensive guides on variables declaration and usage in Gradle.
Beyond Technicalities: Passing Environment Variables to Your Gradle Wrapper Build
Running the Gradle Wrapper build command can sometimes necessitate modifying specific environment variables to achieve the desired results for your Java project. Achieving this task by interacting directly with the command line interface is very straightforward and conveniently adaptable.
One way you could pass environment variables to the Gradle Wrapper build using only the command line would be by setting them before invoking the gradlew command as shown in the code snippet below:
MY_ENV_VAR=desired_value ./gradlew build
In the above example, MY_ENV_VAR would be the name of your custom environment variable, and ‘desired_value’ would be the value that should be assigned to it. This command will result in the ‘gradlew build’ command being executed with an environment where MY_ENV_VAR has been set to ‘desired_value’. Note, however, that this change in the value of MY_ENV_VAR will not impact other commands issued in the same terminal session after the gradlew invocation.
If you have multiple environmental variables to assign values to, the format would just involve a series of assignments pre-pended to the gradlew build command as demonstrated in the following code snippet:
ENV_VAR1=value1 ENV_VAR2=value2 ./gradlew build
In circumstances where you need to employ these parameters frequently in your Java development routine, it would be viable to create a shell script or batch file (depending on the operating system you’re working with) that sets such environment variables consistently prior to each ‘gradlew build’ invocation.
As Matt Raible rightfully pointed out, “Variables are one of the major building blocks of programming.” Understanding how to manipulate them via the command line can significantly enhance the flexibility and adaptability of your software development practices. Adhering to good environment variable use convention can save a lot of development time and simplify troubleshooting when issues arise. It’s all about mastering standard practices and using them efficiently to bring your coding expertise to the next level.
Command Line Mastery: Simplifying Gradle Wrapper Builds with Environment Variables
As a proficient Java developer, the command-line environment is the mainstay of your day-to-day operations. Mastering this territory greatly simplifies your tasks and workflows. A quintessential tool that you would find handy is the Gradle Wrapper and how it blends with environment variables for seamless builds.
The Gradle Wrapper, an elemental part of your Gradle build, is designed to provide users with a frictionless method for executing builds. Bundled alongside your workspace repository, it equips all developers involved in a project with a standard way to invoke the build.
However, as you navigate projects, certain scenarios require entering a set of configurations – ideally during execution. How do we attain this while preserving our code’s sanctity?
This predicament finds a solution with environment variables. Environment variables are essentially dynamic, named variables on your system that can be used to store data that can be utilized by multiple applications. In this scenario, they carry the power to pass sensitive information to our Gradle Wrapper without touching the underlying code. Recall the wisdom in Brian Kernighan’s famous words: “Everyone knows that debugging is twice as hard as writing a program in the first place. So if you’re as clever as you can be when you write it, how will you ever debug it?”
Let’s say we want to inject an authorization token into a Gradle Wrapper build for an HTTP request. Here’s what your command line tug-of-war might look like:
$ export AUTH_TOKEN="your-token-here" $ ./gradlew run
In this instance, `AUTH_TOKEN` represents our environment variable. To leverage its value within the Gradle build, refer it in a manner aptly demonstrated below:
def token = System.getenv("AUTH_TOKEN") ?: "Token Unavailable"
`System.getenv()` method fetches the assigned value to the environment variable `AUTH_TOKEN`. If no value exists (null), `Token Unavailable` becomes the placeholder.
But remember, the purpose of environment variables is not only to maintain the robustness of the program but to help you manage confidential information without exposing them to any risk factor. The art lies in their ability to decouple security-critical details from your application and ensure their safe passage to necessary components.
You could further explore this topic by checking out Gradle Wrapper Documentation or other resources such as the comprehensive reserve of stack overflow discussions available online.
Unveiling the Power of Command Line for Enhanced Gradle Wrapper Builds
Leveraging the potency of the command line can greatly enhance Gradle Wrapper builds. This tool is particularly beneficial when we need to pass environment variables to the build without writing them directly into the scripts or configuration files.
Let’s elucidate this by discussing how to pass environment variables to the Gradle Wrapper build using only the command line.
Passing Environment Variables with Command Line
Environment variables are an efficient method of providing config values to your build. They are easy to change, they keep sensitive data safe, and they don’t necessitate changing source code or build script.
Passing them through the command line can be done implicitly or explicitly. For an implicit pass, you assign a value to an environment variable before running the gradle command:
export VAR_NAME=var_value ./gradlew task_name
However, a more explicit approach would be:
VAR_NAME=vale ./gradlew task_name
This ensures the Gradle build environment will have access to the VAR_NAME.
Rationale for Using the Command Line
Running scripts from the command line might initially seem daunting, but it holds several advantages that becomes apparent once you’ve acclimated yourself to it.
– Convenience: Variables like version numbers or file paths can be updated with ease without adjusting the actual code.
– Security: Confidential information such as API keys or passwords can be passed securely without revealing them in plain text.
– Adaptability: It allows developers to debug build problems on the server.
To quote Doug Gwyn, a notable figure in UNIX culture, “UNIX was not designed to stop its users from doing stupid things, as that would also stop them from doing clever things.” This encapsulates the incredible flexibility that mastering the command line environment bestows upon developers.
Enabling Effective Builds
In the context of Gradle, the chief advantage of employing the CLI as compared to GUI lies in automation and extensibility. Tasks that require user input become a bottleneck in any automated process. Utilisation of the command line obviates this issue, extending the potential of Gradle.
Furthermore, utilizing commands enables a finer control at a lower level over Gradle builds. Whether it is to declare project properties or configure Gradle properties, all such functionalities are at disposal via the command line.[source]
All of this empowers developers to create environments where the build system and the used resources can be tuned to their liking and requirements, drastically enhancing the potentiality of Gradlе Wrappеr builds.
To conclude, leveraging the power of the command line provides enhanced control over Gradle Wrapper builds and allows imperative parameters to be passed efficiently. It’s an essential skill for anyone seriously working with Java and Gradle.
Understanding how to pass environment variables to a Gradle wrapper build by utilizing only the command line is pivotal for any Java developer looking to integrate this approach into their development routine. By mastering this concept, you ramp up efficiency and align your projects to modern coding practices.
For those new to this, passing environment variables to the Gradle wrapper can be achieved by prepending them prior to executing the script in the command line. For instance, if we were to set the variable `VAR1` to `VALUE1`, it would look something like:
VAR1=VALUE1 ./gradlew task
This methodology paves the way toward a more streamlined project setup and it eliminates the need for having to manually update or change settings within the project or IDE. It’s an essential technique that bears weight in the realm of Java development.
Developers may also find value in rules files. Again, here’s what one such rule might look like:
systemProp.VAR2 = System.getenv("VAR2")
Such an environment variable would be used as follows:
VAR2=VALUE2 ./gradlew task
This demonstrates how to pass environment variables to the Gradle Wrapper from the command line, which stands as a testament to the agility, versatility, and power inherent in the world of Java programming.
While code-level know-how is indispensable, always remember: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” (Martin Fowler, British software developer and author).
As developers, while we create using machines, it is crucial not to forget that our ultimate consumers are humans too. A simplicity in code, just as demonstrated in passing environment variables via command-line, is a path leading towards developing understandable and robust programs.