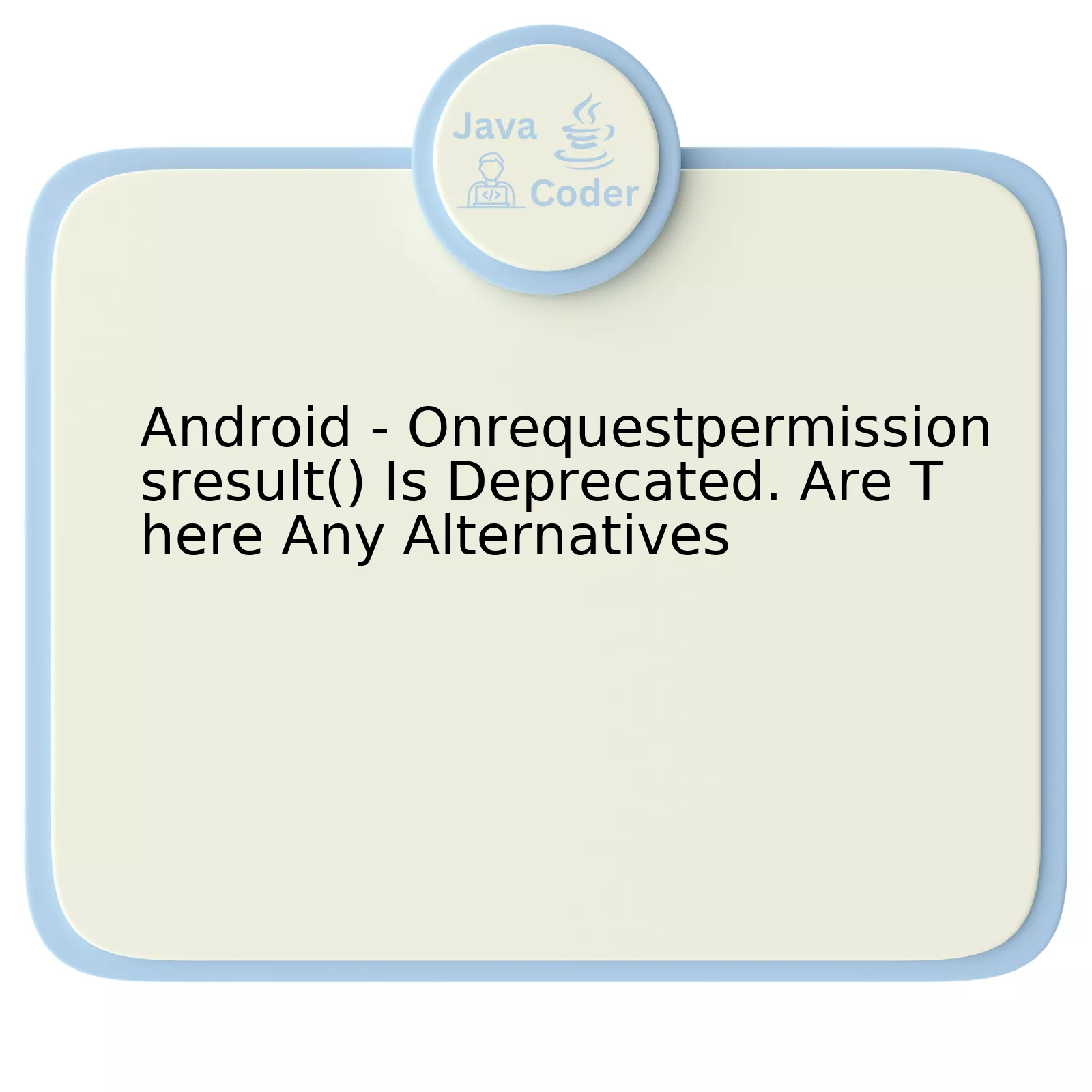
The Android –
onRequestPermissionsResult()
has been deprecated from API 30 (Android 11). It provided a way to manage runtime permissions, a security feature introduced in Android 6.0 (API 23). With the implementation of this method, developers were allowed to prompt for permission right at the point when it is needed during runtime, enhancing user trust and boosting app capabilities. However, the deprecation of
onRequestPermissionsResult()
is due to the advent of new permission handling mechanism introduced in API 23.
Let’s represent this scenario via an HTML table:
Feature | Android – onRequestPermissionsResult() | Android – ActivityResult API |
---|---|---|
Introduction Version | API 23 | API 23 |
Deprecation Status | Deprecated from API 30 | Current standard for requesting permissions |
Purpose | To handle runtime permissions | To manage runtime permissions with a more comprehensive and dynamic approach |
The updated and suggested alternative is to use the ActivityResult API instead of the deprecated
onRequestPermissionsResult()
. Compared to its predecessor, the ActivityResult API provides clearer and safer results through its request-response model, eliminating the need for problematic request codes. Furthermore, it reduces spaghetti code by keeping everything related to one task together rather than spread across different methods.
Here is a practical example showing how to request permission using the new API:
ActivityResultLauncherrequestPermissionLauncher = registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> { if (isGranted) { // Permission acknowledged. } else { // Explain to the user that the feature is unavailable because the features requires a permission that the user has denied. } }); ... private void checkAndRequestPermission() { if (ContextCompat.checkSelfPermission( CONTEXT, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { // Permission already granted. } else { // Requesting permission via new API requestPermissionLauncher.launch(Manifest.permission.ACCESS_FINE_LOCATION); } }
A common quote relating to the software development field might be Jim Highsmith’s words: “The best way to get a project done faster is to start sooner.” This can be applied here clearly indicating the urgency of aligning android application development with up-to-date API versions to ensure efficacy and safety of apps. By starting earlier to adopt the new APIs like ActivityResult API, we stand to reap the benefits of creating applications that are robust and optimized for the latest devices.
Exploring the Deprecation of onRequestPermissionsResult() in Android
In investigating the deprecation of
onRequestPermissionsResult()
in Android, one must likewise examine suitable alternatives. Deprecation is part and parcel of software evolution, intended to phase out methods or features that have been rendered obsolete due to more advanced solutions.
The
onRequestPermissionsResult()
is a method in Android development used as a callback for the results of permission requests. However, Google recently deprecated this method since the release of Android 11, seeking to
implement newer methodologies that encapsulate the complete lifecycle of asynchronous operations.
Recognizing the need to replace deprecated Android components is crucial for developers to keep their codebase updated, future-proofed, relevant, and devoid of issues caused by outdated methodologies. Thus, the following comprehensive guide should lead any develop to adapt their coding practices seamlessly with Android’s latest trends.
The Fragment Result API
This introduces the principal substitute for
onRequestPermissionsResult()
: The framework-provided
registerForActivityResult()
method from Fragments API in Android 11. This new method accepts an instance of an
ActivityResultContract
and a callback. It suits well when replacing the deprecated method due to its ability to register a request and handle the result as well.
Here is an example of how you might use it:
examplePermission.registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> { if (isGranted) { // Execute functionality depending on the permission } else { // Handle denial of permission } });
In this piece of code, an instance of
ActivityResultContracts.RequestPermission
allows your application to prompt the user to grant the appropriate permissions, while the lambda function handles the response accordingly.
Importance of Adapting to New Practices
Deprecating functions like
onRequestPermissionsResult()
is decided by the Android team to be beneficial for the community. Developers are encouraged to adhere to these changes because deprecated APIs might not be supported in future Android versions or could be removed entirely. Thus adhering to these updates, your applications become resistant to future vulnerability, improving application performance and user-experience.
Google I/O ’18 keynote speaker Dan Sandler states, “By willingly embracing the deprecation of outdated methods and transitioning to improved practices, developers unlock invaluable capabilities that enhance their product’s usability and versatility.”
Remember, always refer to the official Android Developers website for most recent information related to deprecations and their suitable alternatives.
Current Challenges Arising from the Deprecation of onRequestPermissionsResult()
In the rapidly evolving landscape of Android development, it’s common to encounter difficulties in adapting to changes that often occur, particularly when familiar methods are deprecated. A typical example of this is the deprecation of
onRequestPermissionsResult()
. Developers are compelled to grasp alternatives and adjust their code to ensure not only its effectiveness but also its compatibility with subsequent versions of Android.
First, let’s clarify what we mean by deprecation. In software development, deprecation refers to the discouragement of use of a particular software feature. Essentially, when a method or a class gets deprecated, it is discouraged from use, primarily because there’s an improved alternative or the feature is no longer relevant for some other reason. Importantly, a deprecated method is not immediately removed because it provides developers the necessary time until they adapt to the new method suggested.
The method
onRequestPermissionsResult()
followed this similar trajectory in recent times. As part of Android’s permission model, it was the standard way to handle users’ responses when their permission was requested at runtime. However, with the release of Android 11, the Android team announced its deprecation.
The primary alternative provided by Google for the situation where
onRequestPermissionsResult()
has been deprecated comes from the AndroidX Activity library, version 1.2.0-beta02 and Fragment library version 1.3.0-beta02. It involves using registerForActivityResult() with the new ActivityResultContracts.RequestPermission(). For instances where multiple permissions are needed, the RequestMultiplePermissions() class within ActivityResultContracts could be employed.
Consider the following code snippet as an example:
registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> { if (isGranted) { // Execute your functionality when permission granted } else { // Handle the case where the user denied the permission } });
Tim Berners-Lee, one of the pioneers in technology, once said, “The goal of the Web is to serve humanity. We build it now so that those who come to it later will be able to create things that we cannot ourselves imagine.” This rings true even now as technological advancements reshape how we approach problems, driving us to devise better solutions.
To reference this change, you can find more about it on the official Android developer’s website. Here, they offer a comprehensive guide addressing the shift from using
onRequestPermissionsResult()
to adopting
registerForActivityResult().
This significant shift in handling permissions in Android demonstrates how technology invariably advances to provide better, more sophisticated solutions, urging developers to adapt, learn, and grow continuously.
Emerging Alternatives for the Deprecated onRequestPermissionsResult()
The recent updates in Android has brought deprecation to the method
onRequestPermissionsResult()
. This function was used for managing app permissions before Android 11. There has been a finer grained permission model now, and some functions like
onRequestPermissionsResult()
have been deprecated.
Let’s dive into alternatives you can consider instead of the deprecated
onRequestPermissionsResult()
.
Activity Result API:
Google is encouraging use of the new Activity Result APIs. Instead of overriding
onActivityResult()
or its associated request codes, Android has announced a far more streamlined way to handle activity results. The key objective with these APIs is to provide a consolidated system that yields greater clarity and robustness when dealing with both activity and permission results. You get type safety, runtime safety and don’t need any request codes by using this approach.
Here is an example:
java
ActivityResultLauncher
registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> {
if (isGranted) {
// Permission granted
} else {
// Permission denied
}
});
if (ContextCompat.checkSelfPermission(this, Manifest.permission.RECORD_AUDIO)
== PackageManager.PERMISSION_GRANTED) {
// You can use the API directly
} else if (shouldShowRequestPermissionRationale(…)) {
// Explain to the user why you need the permission
} else {
requestPermissionLauncher.launch(Manifest.permission.RECORD_AUDIO);
}
DataStore Preferences:
With the DataStore library, you have two options: Proto DataStore and Preferences DataStore. For most cases of replacing SharedPreferences, Preferences DataStore will be adequate. However, Proto DataStore allows typed objects so complex data types can be stored with greater ease than JSON strings with SharedPreferences.
This should give you a head start to accommodate these changes in your android application. In the wise words of Mitchell Hashimoto, co-founder of infrastructure automation company HashiCorp, “In our world, we are on the bleeding edge. We’d rather not do things by the book.”
Notice that no matter how technology evolves, keep abreast of those changes and continually adapting our skills accordingly is imperative in the journey of software development. As mutable as technology is, one constant remains, the pursuit of improvement and advancement.
Innovation and Compatibility: Modern Replacements to onRequestPermissionsResult()
When Android users are confronted with the news that the method
onRequestPermissionsResult()
is deprecated as of API 30, it can make them feel unsure about their future development projects. Fortunately, this evolution of Android’s approach to requesting permission doesn’t mean developers are left without alternatives. With technology ever-evolving and innovating, there are new methodologies in place to handle the permissions in a better way.
Let’s consider the example of the Permissions API in Jetpack, which demonstrates that technology entities continually seek what is best for both developers and end-users. Relative to onRequestPermissionsResult(), the new API seems to offer resilient flexibility and practicality.
Consider how you use
onRequestPermissionsResult()
.
java
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
switch (requestCode) {
case PERMISSION_REQUEST_CODE:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
performAction();
}
return;
}
}
But onRequestPermissionsResult() becomes deprecated. Below is an alternative using registerForActivityResult and ActivityResultContracts.RequestMultiplePermissions().
java
ActivityResultLauncher
registerForActivityResult(new ActivityResultContracts.RequestMultiplePermissions()) {
boolean allGranted = !result.containsKey(Manifest.permission.CAMERA) ||
result.containsKey(Manifest.permission.ACCESS_FINE_LOCATION);
if (allGranted){
performAction();
}
};
requestPermissionLauncher.launch(new String[] { Manifest.permission.CAMERA,
Manifest.permission.ACCESS_FINE_LOCATION});
The frequently quoted phrase “the only constant in technology is change” comes to mind here. Notable is how this change aligns with what Google developer advocates have been emphasizing in recent years: encouraging developers to embrace up-to-date tools and APIs, focusing on those that improve reliability, enhance performance, and ensure productivity.
As developers adjust to these changes, it’s useful to remember that innovation often accompanies disruption. Technological progression affords us the opportunity to learn, grow, and adapt in our pursuit of creating top-tier applications.
In the grand scheme of things, the deprecation of
onRequestPermissionsResult()
in favor of more advanced mechanisms aligns with the broader objective of optimizing Android application development.
Just as Lisa Gansky, American Entrepreneur and author once observed, “Often, the most strikingly innovative solutions come from realizing that your concept of the problem was wrong.” This iterative journey of resolving deprecated APIs welcomes the unveiling of better, more efficient solutions.
For further reading into Android’s newer APIs and Libraries, visit the Android Developer Documentation’s page on Jetpack and its various features here.
As a Java developer, navigating the world of Android is no doubt familiar territory. However, Android’s evolution brings changes and deprecations that developers have to deal with. One such modification is the deprecation of the
onRequestPermissionsResult()
method. While it has served its purpose diligently over the years, the immutable cycle of technological growth suggests a need for more efficient solutions.
Onrequestpermissionsresult(), introduced in API level 23, formed a key element within the permissions protocol in Android applications. It was designed to handle the grant or denial of our permission requests. But the lifecycle complexities coupled with duplication problems made the Android team reconsider its approach.
With the commencement of Android Activity Result API (from AndroidX 1.2.0), we now have a better-suited substitute for onRequestPermissionsResult().
Introduced to us in Fragment 1.3.0 and Activity 1.2.0, the new permissions handling mechanism has simplified the process. It has minimized duplications while maintaining backward compatibility, thereby giving developers more capability and convenience.
Instead of using
onActivityResult()
or
onRequestPermissionsResult()
, you register an instance of an
ActivityResultContract
and receive a
ActivityResultLauncher
.
Here’s a small example:
Before: | Now: |
---|---|
int REQUEST_CODE = ...; void requestPermission() { ActivityCompat.requestPermissions(this, new String[] {Manifest.permission.CAMERA}, REQUEST_CODE); } @Override public void onRequestPermissionsResult(int requestCode, ...) { if (requestCode == REQUEST_CODE) {...} } |
ActivityResultLauncher<String> requestPermissionLauncher = registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> { if (isGranted) {...} else {...} }); void requestPermission() { requestPermissionLauncher.launch(Manifest.permission.CAMERA); } |
This upgrade, as John Papa rightfully quoted, “Code is like humor. When you have to explain it, it’s bad.” Thanks to this improvement, code readability is highly improved, it’s reduced, easier to understand, eliminating unnecessary boilerplate codes, which makes maintaining and debugging relatively easy. The replacement makes sure your code is cleaner, shorter and smoothly run across different Android versions.
The shift from
onRequestPermissionsResult()
towards
ActivityResultContracts.RequestPermission
marks another stride in the versatile progression of Android. As developers, adapting to these changes will not only enhance our coding efficiency but will also future proof our apps in the ever-evolving digital landscape. Plus, it is always good to stay updated with recent coding practices, helps us in continuously delivering quality products enhancing end-user experience.
Refer Google’s official documentation on Android app permissions for an in-depth understanding of the changes and how you can implement them in your current and future projects.