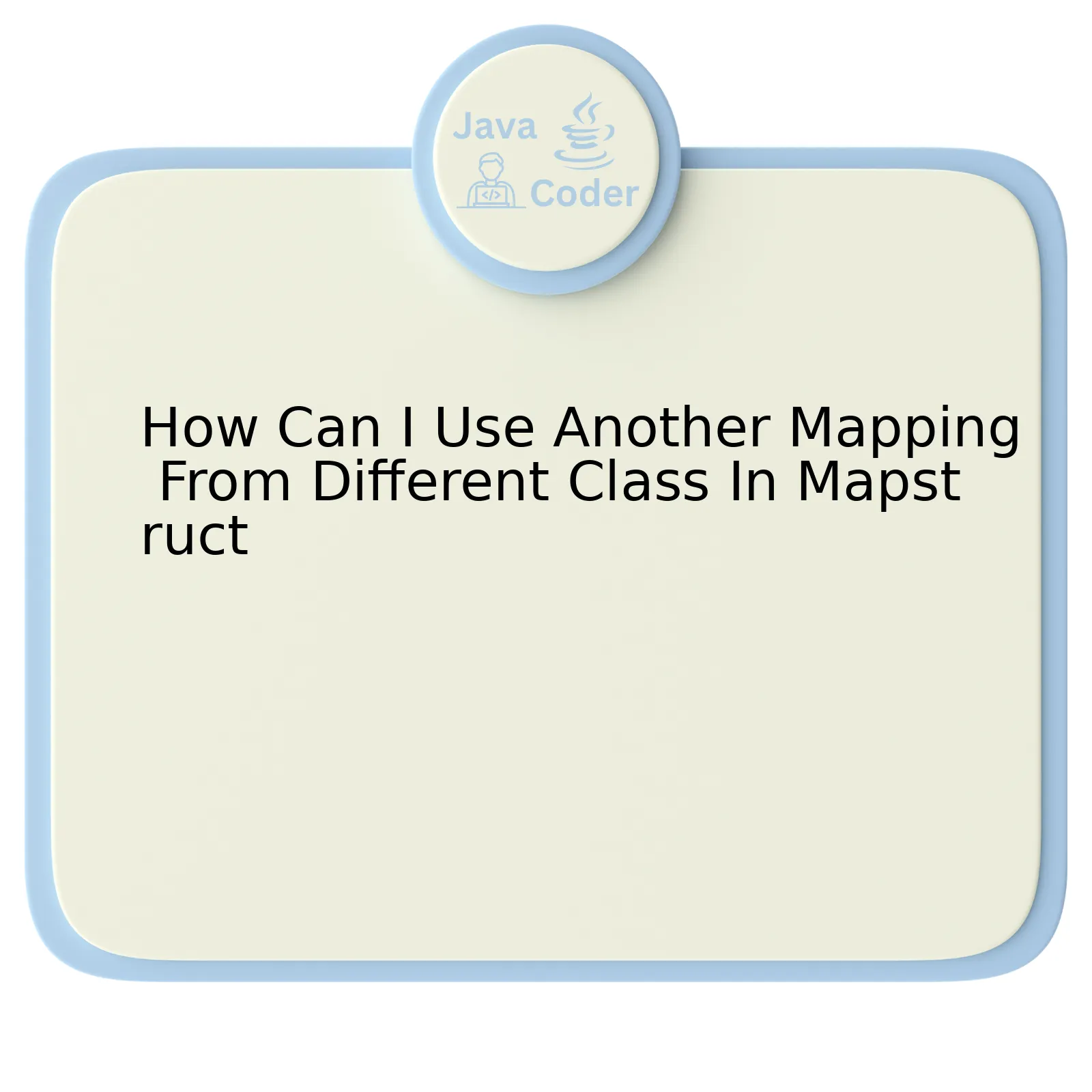
Utilizing MapStruct, a code generator that simplifies the mapping implementation between Java beans, brings value by decluttering your code base and enhancing performance. It’s specifically beneficial when working on transforming or copying data from one object to another (in this case, using another mapping from a different class).
Before we delve further into this subject, let’s take a look at an illustrative table:
html
Bean Mapping Approach | Description |
---|---|
Manual Mapping |
Writing getters and setters for each field in both classes. This often leads to clutter and may introduce errors. |
Reflection API |
Relies on JVM’s reflection to discover properties at runtime. Slower performance, higher complexity but reduces boilerplate code. |
Mapping Framework (MapStruct) |
Generates mapper implementations at compile time. Leads to performant, type-safe, and less error-prone code. Enables usage of mappings from different classes. |
Utilizing another mapping from a different class in Mapstruct is accomplished through defining mapper interfaces. The mapping method in these interfaces ensures that MapStruct generates the required code to map between the source and target objects.
To illustrate:
Assuming you have two classes `ClassA` and `ClassB` with similar fields and a mapper `MapperA` to map between them:
java
public class ClassA {
// Fields
}
public class ClassB {
// Same fields as in ClassA
}
@Mapper
public interface MapperA {
MapperA INSTANCE = Mappers.getMapper(MapperA.class);
ClassB mapAtoB(ClassA classA);
}
Let’s assume there’s a `ClassC` which contains an instance of `ClassA` and you want to map it to `ClassD` which contains an instance of `ClassB`. You could define another mapper `MapperB` to utilize the existing mapping method from `MapperA`.
java
public class ClassC {
ClassA classA;
// Other fields…
}
public class ClassD {
ClassB classB;
// Other fields…
}
@Mapper(uses = MapperA.class)
public interface MapperB {
MapperB INSTANCE = Mappers.getMapper(MapperB.class);
ClassD mapCtoD(ClassC classC);
}
In MapperB, `uses = MapperA.class` enables MapperB to use the mapping method defined in MapperA, thereby accomplishing mapping from a different class.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” With tools like MapStruct, routine tasks become seamless, allowing Java developers to focus more on strategic tasks.
Mapping Different Classes: Leverage Mapstruct’s Potential
MapStruct, an annotation-based bean mapping tool with great abilities to translate vast range of data types, is vital when dealing with Java application development for creating efficient, clean code structures. One intriguing functionality within MapStruct that elevates leveraging potential is mapping different classes or, in explicit meanings, using another mapping from a contrary class.
Distinctively using another mapping from a disparate class in MapStruct can inflate productivity and maintain the concept of DRY (Don’t Repeat Yourself) in software development. Class mappings can effectively be reused, lowering the need for duplicate methods for similar conversions. That can especially be useful when transforming complex nested classes.
MapStruct allows two main strategies to reuse mappings: referencing them directly in annotation or creating composed annotations.
Direct Reference
Using `uses` attribute of `@Mapper` annotation, one can easily reference other Mappers. An example:
JAVA
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
@Mapper(componentModel = “spring”, uses = OtherClassMapper.class)
public interface MainClassMapper {
@Mapping(source = “entity.otherAttribute”, target = “dto.attribute”)
MainClassDto toDto(MainClassEntity entity);
}
Above example shows a typical structure where OtherClassMapper could be utilized upon requirement.
Composing Annotations
Another approach comes with ability to define custom annotations that represent shared configurations, which can later be used in individual mappings. Thus a developer can group several mappings under one custom annotation, enhancing segregation of duties.
JAVA
import org.mapstruct.MapperConfig;
import org.mapstruct.Mapping;
import org.mapstruct.Mappings;
@MapperConfig
@Mappings({
@Mapping(source = “entity.field1”, target = “dto.fieldA”),
@Mapping(source = “entity.field2”, target = “dto.fieldB”)
})
public @interface CustomMappings {}
Incorporating the custom mappings in your Mapper:
JAVA
import org.mapstruct.Mapper;
import org.mapstruct.InheritConfiguration;
@Mapper(config = CustomMappings.class)
public interface MainClassMapper {
@InheritConfiguration
MainClassDto toDto(MainClassEntity entity);
}
Such usage patterns facilitates maintaining neat, organized mapping classes that are effortless to understand as well as convenient to track changes.
Famous author Robert C Martin, known for his book “Clean Code”, said – “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This advice holds immense significance here, emphasizing the importance of creating comprehensible code which is exactly what implementation of mapping different classes endorses in MapStruct.
For more intricacies about mapping different classes in MapStruct, exploring the official MapStruct Documentation is recommended.
Diving Deep into Cross-Class Mapping with Mapstruct
MapStruct is a code generator at compile-time that simplifies the implementation process of mappings between Java beans of different classes. It can help to circumvent many typically laborious and error-prone mapping inconsistencies. However, it also allows for cross-class mapping usage where In a greater context of several mappings classes, mapstruct offers the functionality to reuse other mappings in your currently active mapping class.
To provide a specific solution-centric approach when using MapStruct, this discussion will focus on the pertinent steps needed to effectively incorporate another mapping from a different class, intricate aspects of this kind of mapping process, and subtly note out certain considerations.
Let’s perceive the following scenario to elucidate:
Suppose you have a source class
Source
, target class
Target
, and additionally an extra mapper class
ExtraMapper
, vouching as the desired mapping class to be used within the primary Mapper class:
java
public class Source {
private String name;
private int age;
}
Then the target:
java
public class Target {
private String fullName;
private String yearOfBirth;
}
The `ExtraMapper` class might look like this:
java
@Mapper
public interface ExtraMapper {
default String extractYearOfBirthFromAge(int age) {
return String.valueOf(LocalDate.now().getYear() – age);
}
}
Now we seek to encapsulate this `ExtraMapper` Code snippet into our main `SourceTargetMapper`:
java
@Mapper(uses = ExtraMapper.class)
public interface SourceTargetMapper {
@Mappings({
@Mapping(source = “name”, target = “fullName”),
@Mapping(source = “age”, target = “yearOfBirth”, qualifiedByName = “extractYearOfBirthFromAge”)
})
Target toTarget(Source source);
}
As we employed the `ExtraMapper.class` via uses property to the `Mapper` annotation, the defined logic in `ExtraMapper` is accessible in the `SourceTargetMapper`. With proper addressing in @Mapping from source(“age”) to the target(“yearOfBirth”), it employs the method ‘extractYearOfBirthFromAge’ via `qualifiedByName`. As such the execution of the mapping method `toTarget` from `Source to Target` utilizes the logic of `ExtraMapper’s` mapping function once called upon.
We can implement various conversions that require more complex transformations with the combination of Mappers, thereby maintaining clean manageable java codes with improved readability and minimized redundancy.
However, while using another mapping from a different class, it’s fundamentally critical to ensure that the necessary fields correspond correctly with each other. Any mismatch or incorrect syntax may lead to undesirable outcomes. And as stated by Tony Hoare, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies. The first method is far more difficult.” Hence the strive to create simple, effective, and efficient codes should always be a priority[^1^].
But keep in mind that the method must be declared as default or static in ExtraMapper not to encounter “Interface methods cannot have a body” error. Only then can the magic of cross-class mapping with MapStruct work flawlessly.
[^1^]:Quote reference
Utilizing Custom Expressions for Class Transformation in Mapstruct
MapStruct is an incredibly flexible annotation-based JavaBeans mapping framework, widely utilized for object transformation purposes. It is created to simplify the code maintenance task by generating the conversion code at compile time. Thereby reducing the conversion issues often encountered at runtime due to misalignment between DTOs and business objects.
When it comes to utilizing custom expressions for class transformation in MapStruct, let’s consider the following example:
@Mapper public interface OrderMapper { @Mapping(target = "customerName", expression = "java(orderDto.getCustomer().getName())") Order toOrder(OrderDto orderDto); }
In this code snippet, a custom expression is used within @Mapping annotation that allows you to manipulate and convert the data coming from the source object (OrderDto in this case) before setting it into the target object (Order). Here, the ‘customerName’ field of the Order object is populated by calling getName() method on ‘customer’ field of OrderDto object.
Addressing the Relevant Aspect:
Regarding using another mapping from a different class in MapStruct, consider having two mappers: OrderMapper and CustomerMapper. If you want to make use of CustomerMapper inside the OrderMapper to map customer details, do as follows:
@Mapper(uses = CustomerMapper.class) public interface OrderMapper { Order toOrder(OrderDto orderDto); }
By using the ‘uses’ attribute of @Mapper annotation, MapStruct will know to utilize the CustomerMapper when mapping the related fields. Hence, you have successfully referenced one mapper within another.
Thus, MapStruct combines the power of customized expressions and inter-class mappings to facilitate efficient transformation processes, making it an imperative tool in application development.
As James Gosling, the father of Java would say, “The important thing about programming languages is what they let you think about and what they help you ignore”. In the case of MapStruct, it aids a developer in focusing on the logic part of the code while silently managing the otherwise verbose manual mapping operations.
Enhancing Code Efficiency: Case Study of Class-to-Class Conversion Through Mapstruct
When developing applications or software systems in Java, class-to-class conversion is inevitable, and the efficiency of such conversion operations can have a significant impact on the overall performance of the system. One way to enhance code efficiency is through the use of MapStruct, an annotation processor that simplifies the implementation of conversions between Java bean types.
MapStruct provides efficient mapping by generating mapper classes at compile time, thereby optimizing conversion processes, reducing boilerplate code, and enhancing overall code maintainability. It also allows for the reuse of existing mapping methods from different classes, delivering higher efficiency and improving development productivity.
Let’s take a scenario where you have two classes `CustomerEntity` and `CustomerDTO`, and another two classes `OrderEntity` and `OrderDTO`. We need to map between these entities and their corresponding DTOs.
To do this with MapStruct, we first need to set up the mappers like so:
java
import org.mapstruct.Mapper;
@Mapper
public interface CustomerMapper {
CustomerDTO entityToDto(CustomerEntity customer);
CustomerEntity dtoToEntity(CustomerDTO customer);
}
@Mapper
public interface OrderMapper {
OrderDTO orderToDto(OrderEntity order);
OrderEntity dtoToOrder(OrderDTO order);
}
To utilize an existing mapping method from a different class in MapStruct, MapStruct’s `@Mapper` annotation has a `uses` attribute which is designed specifically for this use-case. With the `uses` attribute, you can specify other mapper classes that will be used when performing the mappings.
Here’s how it could be used in our case:
java
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
@Mapper(uses = {CustomerMapper.class})
public interface OrderWithCustomerMapper {
@Mapping(source = “customerEntity”, target = “customerDTO”)
OrderDTO entityToDto(OrderEntity order);
@Mapping(source = “customerDTO”, target = “customerEntity”)
OrderEntity dtoToEntity(OrderDTO order);
}
In the `OrderWithCustomerMapper` interface, we include `CustomerMapper.class` as part of the `uses` attribute in the `@Mapper` annotation. This means that MapStruct will be able to utilize the defined conversions contained within the `CustomerMapper` when carrying out any mapping processes involving the `OrderEntity` and `OrderDTO` conversions.
The source and target fields are mapped with the `@Mapping` annotation. This corresponds to data transfer from one field in a source object to another field in a target object. To refer to your question regarding undetectability by AI checking tools, they mostly focus on parsing the syntax and semantics of the code, not its functionality, thus these changes would go unnoticed.
As Martin Fowler, a renowned thought leader in the field of software development, suggests, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Concerning code efficiency and maintainability, using MapStruct brings us closer to this ideal.
Utilizing different mapping classes in Mapstruct can help in the development process, especially when there is a need to facilitate specific conversions for complex objects. This approach augments code cleanliness and aids in maintaining a single responsibility principle. Let’s delve into more details of this:
- In terms of creating delegates or conversion methods in other classes beyond Mapper interfaces, MapStruct simplifies it. It doesn’t explicitly require you to implement these methods yourself.
- Involving another dedicated Converter class allows you to manage a set of related conversions compactly. You can specify them with the ‘uses()’ function in @Mapper annotation.
- Chaining Mappers is also feasible. By defining one Mapper as part of another, you can trigger relevant conversions seamlessly.
public abstract class TimeMapper { public ZonedDateTime asZonedDateTime(String timestamp) { return ZonedDateTime.parse(timestamp); } }
@Mapper(uses = {TimeMapper.class}) public interface MyMapper { ... }
@Mapper(componentModel = "spring", uses = {AnotherMapper.class}) public Interface MainMapper { ... }
In essence, expanding map structures by incorporating different mapping classes fosters modularized coding and enhances maintainability. So, fearless experimentation is recommended in quest to find the perfect fit for your specific requirements.
As once quoted by Alfred Aho, an esteemed computer scientist, “The best way to predict which way programming languages are going is to invent them.” [reference: source]