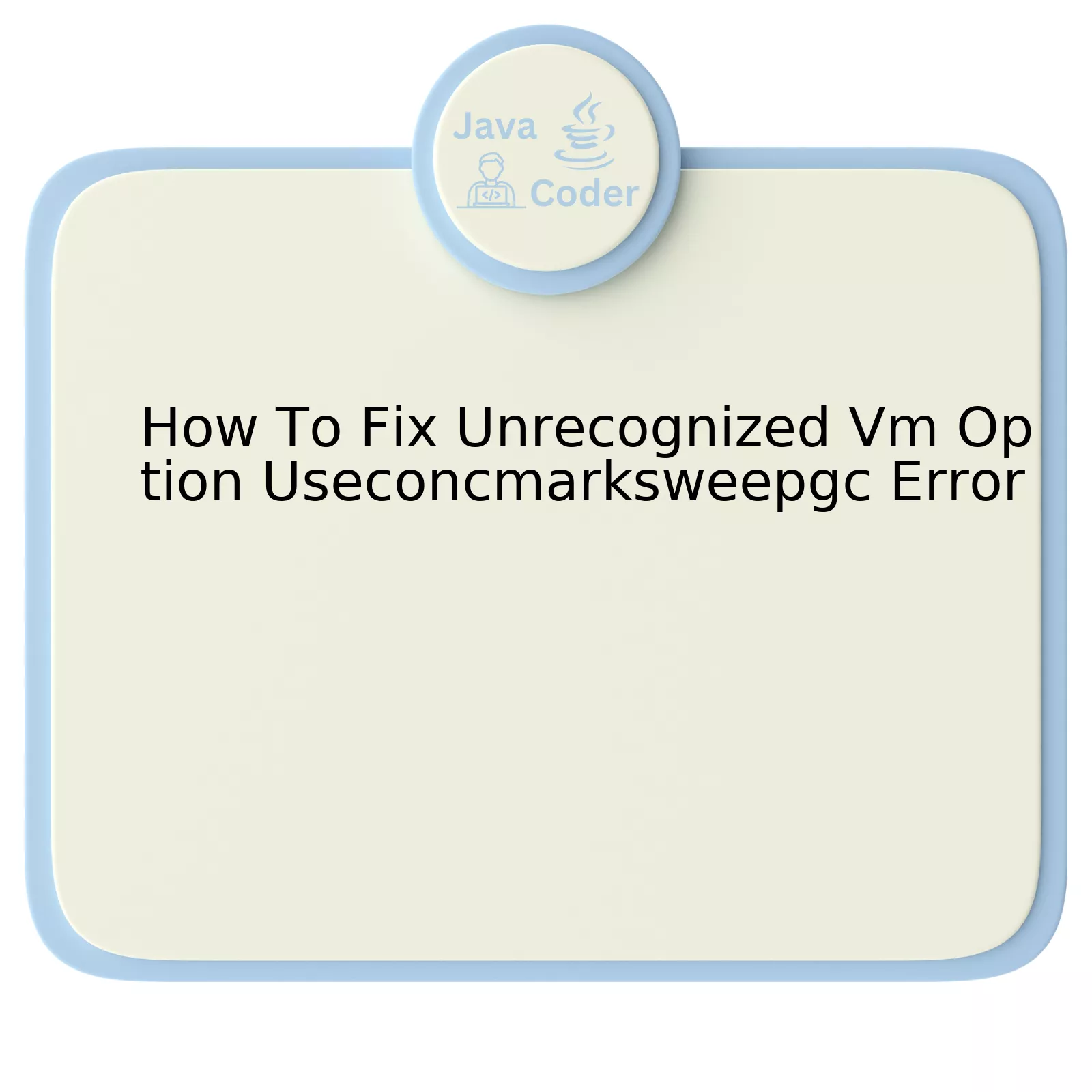
Before diving into the solution, you need to understand the root cause of the “Unrecognized VM option ‘UseConcMarkSweepGC'” error. This error arises due to the deprecation and eventual removal of CMS Garbage Collector from the Java Development Kit (JDK). Starting from JDK 9, the CMS Garbage Collector was deprecated and it was eventually removed in JDK 14.
This implicates that if your application is running with either -XX:+UseConcMarkSweepGC or -XX:-UseConcMarkSweepGC options on JDK 14 and later versions, the JVM will not start and you’ll be getting the Unrecognized VM Option ‘UseConcMarkSweepGC’ Error.
The table below shows possible solutions for this error depending on the JDK version:
Java Version | Fix |
---|---|
JDK 14 and Later Versions | Remove all the CMS options from the command line and use alternative Garbage Collectors like G1GC or ZGC. |
JDK 9 to JDK 13 | You have two options – either remove all the CMS options as recommended for JDK 14 and later, or suppress the depreciation warning by using -Xlint:-options |
JDK 8 and Earlier Versions | In these versions, the CMS options are still recognized and can be used without errors or warnings. |
As per the Oracle’s recommendation, you can replace the CMS GC (-XX:+UseConcMarkSweepGC) with G1GC (-XX:+UseG1GC) in JDK 9 and later versions.
For example, in case you have:
java -XX:+UseConcMarkSweepGC -jar my-application.jar
You can alter this to:
java -XX:+UseG1GC -jar my-application.jar
According to the creator of Java, James Gosling, “Java is to JavaScript what Car is to Carpet”. Referring lastly to any script based alternatives beyond Java is generally discouraged for decent system performance and feasible cross platform development. Hence it’s best to follow given solutions in their exactness to ensure the smooth working of your applications while using JDK.
Remember, the key here is to determine which JDK version you’re operating on and apply the correct fixing strategy accordingly.
Understanding the Unrecognized VM Option UseConcMarkSweepGC Error
The ‘Unrecognized VM option UseConcMarkSweepGC’ error often arises in the event of JVM arguments deprecation or removal. The Garbage Collection (GC) algorithm implied by this option, Concurrent Mark Sweep (CMS), was deprecated in JDK 9 and ultimately removed in JDK 14. When applications previously configured with CMS are operated on modern Java Development Kit iterations such as JDK 15 or 16, the stated error occurs.
To address this problem, it’s salient to identify feasible solutions that adapt to modern GCs application without compromising system performance. This approach will benefit from an improved developer understanding of memory management within JVM, alongside strategies for choosing suitable GC algorithms.
Consider these strategies:
1. Revert to Older JDK Version
It involves running your application with a JDK version that supports CMS. As mentioned earlier, CMS is available up to JDK 14. For example:
JAVA_HOME=path_to_your_jdk14_home java -XX:+UseConcMarkSweepGC -jar yourapp.jar
Notwithstanding, this strategy bears a token risk of security issues and missing out on new features inherent in newer JDK versions.
2. Transition to an Alternative GC Algorithm
There are several garbage collector supported by modern JDK versions which perform comparably to CMS. Notable mentions include:
– G1GC (Garbage-First Garbage Collector): Generational, parallel, multi-threaded, concurrent, and incremental GC. Optimized for systems with substantial memory space.
– Parallel GC: Provides static data structure sizing contingent upon one’s specific requirements to deduce raw throughput.
– ZGC (Z Garbage Collector): Low-latency GC wieldy for multi-terabyte heaps. Minimal pause times regardless of heap size.
Example setting G1GC:
JAVA_HOME=path_to_your_newer_jdk_version java -XX:+UseG1GC -jar yourapp.jar
In conclusion, making conscious GC choices extends beyond resolving the unrecognized VM option ‘UseConcMarkSweepGC’ issue. It encompasses a pattern for scaling performance on the back end of Java applications. A careful deliberation over alternatives, guided by insights on offered features, trade-offs and use cases, becomes essential.
Dave Thomas once said, “A good programmer is someone who always looks both ways before crossing a one-way street.” In similar vein, a seasoned Java developer always weighs options when tinkering with aspects as intricate as GC algorithm selection.reference
Exploring Causes Behind the VM UseConcMarkSweepGC Error
The error “Unrecognized VM option ‘UseConcMarkSweepGC'” frequently surfaces after upgrading JDK. Primarily, this message reveals that the operator has mandated to use Concurrent Mark Sweep (CMS) as a garbage collector. However, since CMS has been depreciated in the latest versions of Java, this causes the JVM to react with an unrecognized VM Option error.
public class MyApp { public static void main(String[] args) { System.out.println("Hello, World!"); }
This code begins a simple Java application; the “main” method signifies JVM’s point of entry into the app. When running the above code on a system using Java 14 or higher with CMS allocated as the garbage collector, you’ll receive the error at issue. Why? Because Java no longer regards ‘UseConcMarkSweepGC’ as a recognized VM option – hence, it throws an error.
Discard the UseConcMarkSweepGC
Removing the flag UseConcMarkSweepGC from your JVM options is a straightforward solution. You will now be utilizing the GC that comes by default i.e., G1.
Examine below for an example:
Replace:
java -XX:+UseConcMarkSweepGC -jar my-app.jar
With:
java -jar my-app.jar
G1 will take over as the default garbage collector if you don’t specify a different one.
Consider the Alternatives
Suppose you have specific reasons not to utilize G1.
You may wish to consider employing ZGC (Z Garbage Collector) or Shenandoah. These GCs are designed to minimize pause times without considerable overhead extensions.
Try Using a Lower Java Version
As an immediate workaround, you could fall back to an older Java version until you’re ready to make a more permanent adjustment. CMS is still accessible in Java 8 provided Oracle distributes it.
It’s essential to realize that software evolves and modifies over time. It influences each layer tied in the system stack we rely on while building applications, as Martin Fowler once said, “Any fool can write code that a computer can understand. Good programmers write code humans can understand.” Therefore, it becomes a practice to iterate updates/changes in a manner that does not disrupt the overall working.
In the same context, communities like O’Reilly Media provide thoughtful insights into garbage collection and JVM’s behavior, which you could consider in updating your JVM options.
Effective Strategies to Fix Unrecognized Vm Option UseConcMarkSweepGC Error
The “Unrecognized VM Option UseConcMarkSweepGC Error” is a Java-based error developers often encounter during the garbage collection process. It’s usually an aftermath of changes in recent Java Virtual Machine (JVM) versions, especially JDK 9 and beyond.
The error precipitates due to the ‘UseConcMarkSweepGC’ flag being deprecated in JDK 9 and subsequently, removed permanently in JDK 14. Thus, it no longer recognizes this flag, leading to the generation of your mentioned error. To rectify this issue, you will need to adopt different strategies ranging from JVM updating to utilizing alternative procedures. These are distinct ways known to counteract such a complication:
1. Update Your Java Version:
One workable solution may be upgrading your JDK. If you’re using JDK 8 or any earlier version, consider moving to JDK 9 or later iterations. Updating JDK could solve this problem as newer versions don’t recognize the ‘UseConcMarkSweepGC’ option.
2. Modify Your Garbage Collection Strategy:
Due to the ‘UseConcMarkSweepGC’ option becoming obsolete, altering your garbage collection strategy can be a productive approach. Reconsider changing it to algorithms compatible with JVM newer versions like Garbage-First (G1) collector. This algorithm helps manage heap space effectively and is gradually becoming a beacon for most Java developers.
To start utilizing G1 collector, replace:
-XX:+UseConcMarkSweepGC
with
-XX:+UseG1GC
Changing to the G1 collector should rectify your issue.
3. Use an Alternative Java Vendor:
An additional way of dealing with the error is by opting for other Java vendors which accommodate features unsupported by Oracle’s JDKs; for example, Red Hat’s Builds of OpenJDK.
As stated by one of Red Hat’s architects, Andrew Dinn,”it’s harder to predict which specific technology will prove essential to your business in the future.”source. Inducting the insight into our current context, swapping to alternative Development Kits can eventually solve your identified problem.
Preventing Future Occurrence of the ‘UseConcMarkSweepGC’ Error
The ‘UseConcMarkSweepGC’ error in Java often pops up when a developer tries utilizing the Concurrent Mark Sweep (CMS) garbage collector. The problem originates from the fact that this garbage collector has been deprecated since Java 9 and removed entirely in Java 14. So, if you are using these versions or any newer ones, the CMS garbage collector is unavailable resulting in the ‘Unrecognized VM Option UseConcMarkSweepGC’ error.
Now, let’s dive into ways to resolve this issue and prevent its future re-occurrence:
Option 1: Switch to a Different Garbage Collector
An effective method is to switch to a supported garbage collector. Java supports several other garbage collectors including:
- G1GC – Garbage First Garbage Collector
- Parallel GC – Parallel Garbage Collector
- ZGC – Z Garbage Collector
- Shenandoah GC – Shenandoah Garbage Collector
For example, switching to `G1GC` entails replacing `-XX:+UseConcMarkSweepGC` with `-XX:+UseG1GC` in your JVM options.
java -XX:+UseG1GC MyJavaApp
Option 2: Revert to an Older Version of Java
Another feasible option consists of reverting to an older version of Java. If your application requires the CMS garbage collector, you can use a JDK that still supports it. Oracle’s JDK 8 or OpenJDK 8, for instance, would be viable options in this case.
It’s crucial to bear in mind, however, that making use of outdated versions might expose your applications to security risks. Hence, adequately weigh this decision beforehand.
Option 3: Redesign Application to Work with a Current Garbage Collector
You could also invest time in redesigning your applications to work with a currently supported garbage collector. Although more time-consuming and labor-intensive, this move boasts of longer-term benefits, ensuring your application aligns with ongoing development best practices.
As Martin Fowler, the renowned software expert, said:
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Thus, adjusting to software changes positively contributes to creating efficient, future-proofed applications. This approach is a vital step in avoiding the re-emergent ‘Unrecognized VM Option UseConcMarkSweepGC’ error.
Consider integrating periodic system audits, upgrading to modern techniques, and adopting decisive strategies to avoid this error altogether. By doing so, you can minimize potential downtime, enhance efficiency, and build robust, scalable software.
Refer here for more information about different garbage collectors and their usage in Java.
Solving Unrecognized VM Option UseConcMarkSweepGC Error
Diving deep into one’s coding issues is a fundamental part of being a software engineer. Today, it seems we’ve touched upon a common error that many encounter while running Java-based applications – the unrecognized VM option ‘UseConcMarkSweepGC’. This particular error could be attributed to using deprecated options which existed in older JVM versions but no longer function in newer ones.
The most effective method to resolve this lies in replacing the deprecated option. Oracle’s transition from Concurrent Mark Sweep (CMS) garbage colllector to Here is the Removal of the Experimental Ahead-of-Time (AOT) and Just-in-Time (JIT) Compiler the Garbage-First (G1) collector since JDK 9 would mean you need to replace ‘-XX:+UseConcMarkSweepGC’ with ‘-XX:+UseG1GC’.
To demonstrate further, when we include the ‘-XX:+UseG1GC’ into our JAVA_OPTS:
JAVA_OPTS="-Xmx1024M -Xms1024M -XX:+UseG1GC -jar minecraft_server.jar nogui"
The change results in the system utilizing the G1 garbage collector instead of CMS, successfully eliminating the ‘Unrecognized VM option ‘UseConcMarkSweepGC” error.
“Errors are just opportunities for learning.” This quote resonates significantly with programmers worldwide; managing to navigate the ‘Unrecognized VM option ‘UseConcMarkSweepGC” error gives us an exemplar experience of this universal sentiment.
It’s pivotal to note that these continuous changes in JVM versions might present challenges initiating even common tasks. A proactive approach includes staying updated on Oracle’s release notes and prevalent programming blogs to understand depreciation and replacements beforehand.