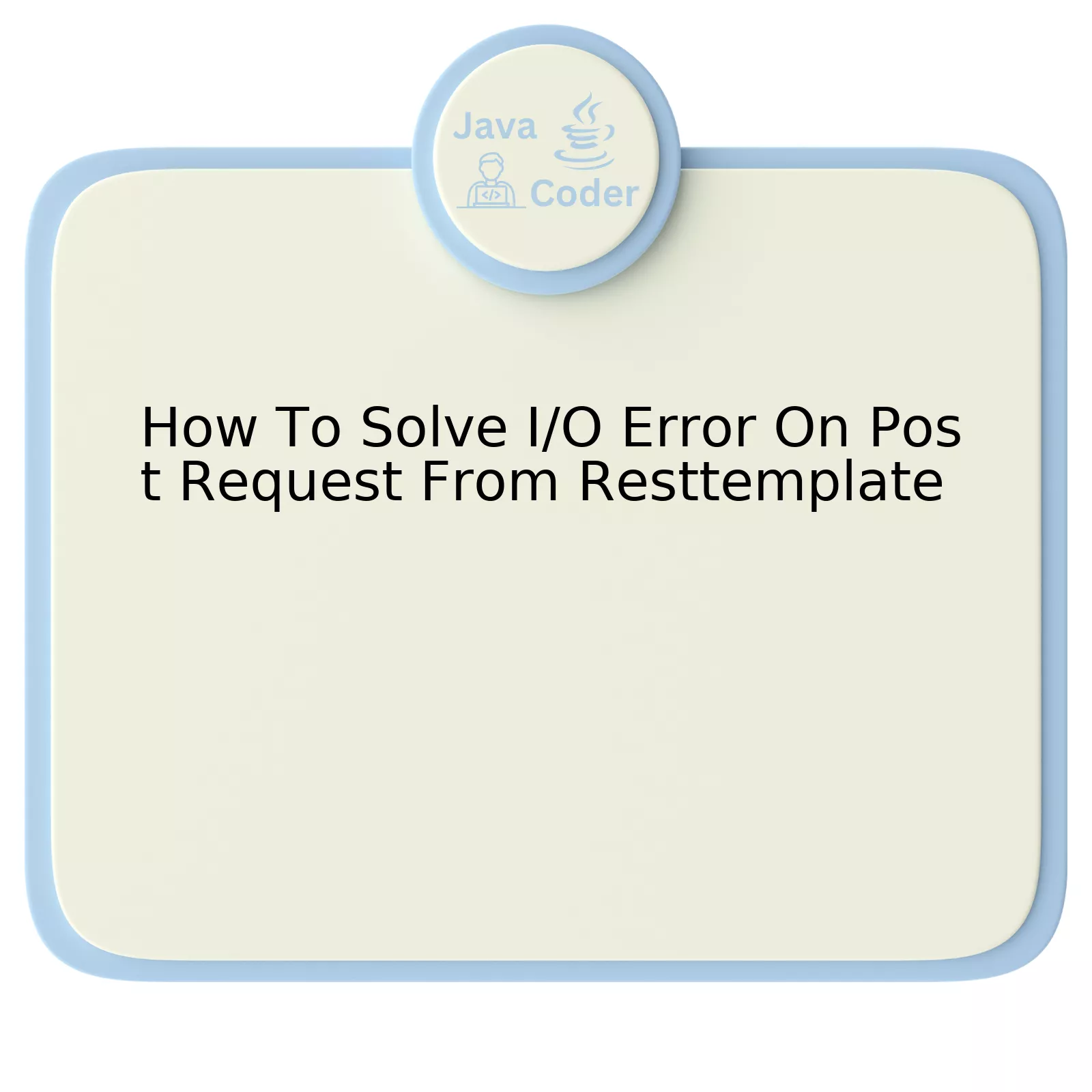
Resolving I/O errors when executing a POST request using RestTemplate fundamentally requires following several key steps. Include system diagnostics checks, pinpointing problem roots, and implementing solutions for the discovered issues.
Below is a structured representation encapsulating these crucial steps:
Steps | Description |
---|---|
Diagnose System Health | Initially, ascertain that your machine is operating optimally with adequate resources to prevent unexpected system I/O complications. |
Review Code Implementation | Check if the RestTemplate usage in your code adheres to best practices. Incorrect implementation can easily result in an Input / Output (I/O) error. |
Timeout Parameters | Consider adjusting timeout parameters for your RestTemplate instance. I/O errors might arise from network latency or prolonged response times, and adjusting the parameters can help manage such scenarios better. |
Check Network Connectivity | Ensure there’s secure and stable internet connectivity. Interruptions or poorly optimized networks can initiate I/O problems. |
ExceptionHandler | If you employ a global ExceptionHandler with an exception resolution strategy, it is important to make sure it can address I/O exceptions too. This acts as a safety net and resolves some potential issues. |
In the overall process of solving I/O errors during a POST request using RestTemplate, first and foremost is evaluating the health of the system to eliminate possibilities of the error originating from there. The deployment platform must be optimized to ensure good performance in handling requests.
Secondly, reviewing code usage is crucial to find out if RestTemplate has been implemented correctly. Errors can originate from incorrect implementations, such as failure to handle CloseableHttpResponse properly, which may lead to resource leaks resulting in I/O errors. Thus, proper implementation of RestTemplate is fundamental.
Adjusting timeout settings could also provide a solution. If your application is wired to high-latency networks, slowing down processes means the timeout is reached quicker, leading to erroneous behavior. By setting restTemplate.setRequestFactory() using HttpComponentsClientHttpRequestFactory and increasing timeouts with setConnectTimeout() and setReadTimeout(), the error can be corrected.
Confirming a strong network connection is vital – spotty, intermittent connections are likely to affect data transfer and result in I/O complications. A steady, reliable network should minimize interruptions thereby mitigating related errors.
One final point is having a robust exception handling mechanism – for instance, a global ExceptionHandler that can potentially deal with I/O exceptions. Implement well-rounded error resolution strategies in your application, so it becomes resilient in the face of such challenges.
As Linus Torvalds said: “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.” Exactify this philosophy while creating an error-free, strong, yet flexible software environment.
Understanding the Basics of I/O Error on Post Request
Understanding the basics of an I/O (Input/Output) error during a POST request in Java requires grasping several aspects of how client-server communication works, particularly within a RESTful architecture. The `RestTemplate` class is often used to execute HTTP requests on a server and when you experience an I/O error during a POST request, it essentially means there’s some problem with the way your client application is connecting to, communicating with or reading from the server.
Let’s divulge further into the relevant steps required to solve an I/O Error on a POST request using RestTemplate:
Identify the Specific I/O Error
Start by identifying the specific type of I/O error you’re experiencing. Here are some common examples:
“I/O error on POST request for…”: This usually occurs due to disruptions in network connections or inaccessible server endpoints.
“No suitable HttpMessageConverter found for response type…”: Occurs if there’s no appropriate converter to decode the server’s response into readable data for your client application.
Analyze the Stack Trace
A detailed analysis of the stack trace thrown at runtime gives valuable insight into what led to the error. Look out for which method calls initiated the error, check for any identifiable patterns.
Validate Request Headers and Body
Ensure that the HTTP headers and body in your POST request are correctly defined. If these parameters don’t comply with what the server expects, this could lead to miscommunication between your client and the server leading to I/O errors.
Try Different RestTemplate Configurations
If your I/O error stems from unexpected server responses, try altering the settings of your client’s `RestTemplate`. For instance, if you’re struggling with message conversion problems, you could add different `HttpMessageConverters` to your `RestTemplate`.
Here is a sample code for adding a converter `RestTemplate restTemplate = new RestTemplate();`:
restTemplate.getMessageConverters().add(new MappingJackson2HttpMessageConverter());
Consult Documentation or Online Resources
The importance of documentation like the official Oracle JavaDoc page for IOException cannot be overstated. Other instances where you’ll find useful assistance include API documentation of any third-party libraries that your system may be relying upon.
For many Java developers, one invaluable piece of wisdom from Brian Kernighan has been:
“Everyone knows that debugging is twice as hard as writing a program in the first place. So if you’re as clever as you can be when you write it, how will you ever debug it?”
Keeping that quote in mind, being prudent in planning your code structure, anticipating possible exceptions and learning about different debugging techniques could significantly mitigate the occurrence of these puzzling I/O errors.
Exploring RestTemplate: A Practical Tool for Communication
RestTemplate, as a primary class in Spring Framework, is widely recognized for its efficient solution in consuming web services. This client-side HTTP access gateway provides excellent tools for communication, making it an invaluable resource for developers working on Spring-based applications.
However, one common issue that has often been encountered by Java developers with RestTemplate involves the Input/Output (I/O) error during Post requests. To effectively troubleshoot this particular challenge, we need to take a methodical and hands-on approach that includes a certain level of understanding and proficiency about RestTemplate’s architecture and capabilities.
“Focusing on problems and solutions becomes a chore when you love what you do. That love brings focus, determination and all the energy you need”. – Carlos Santana, Web Developer.
To effectively detect I/O errors during Post requests from RestTemplate, let’s consider the code snippet scenario below:
RestTemplate restTemplate = new RestTemplate(); String uriTemplate = "https://example.com/api/resource"; HttpEntity<MyObject> request = new HttpEntity<>(new MyObject(), headers); try { ResponseEntity<String> response = restTemplate.postForEntity(uriTemplate, request, String.class); } catch (RestClientException e) { e.printStackTrace(); }
Here, a standard POST request is sent by RestTemplate, and a RestClientException is caught, along with its stack trace. This RestClientException can encapsulate I/O-related issues such as ResourceAccessException or HttpClientErrorException, providing relevant insights into what might have gone wrong.
Viewing the stack trace details of the caught exception could indicate whether it’s an SSL handshake exception indicative of certification issues, a ConnectTimeoutException reflecting network or firewall issues, or an UnknownHostException pointing towards DNS resolution issues.
The table below details some potential remedies based on common error types:
—
- Review the server’s SSL certificate status.
- Consider switching HTTPS to HTTP during testing if acceptable.
- Verify the correctness of the endpoint URL.
- Check if your system’s firewalls are blocking outgoing requests.
- Make sure DNS resolution is working properly for the specific endpoint host.
- Try using the server’s IP address directly instead of hostname.
—
Addressing these common exceptions not only resolves the immediate I/O error but also potentially avoids future REST API communication challenges with RestTemplate.
Reference:
Guide to RestTemplate | Baeldung
Solving I/O Errors in Post Requests: Detailed Techniques and Approaches
When dealing with I/O errors in Post requests specifically when using Resttemplate, this is often indicative of issues ranging from communication problems between the client and server, to unforeseen exceptions thrown due to the non-availability of resources or incorrect configurations. Here are some techniques and approaches for handling such scenarios:
Exception Handling and Logging
It’s important to pursue detailed exception handling and logging to get a good understanding of what may be causing the issue. Java exception stack traces are invaluable here. For example;
try { // your Resttemplate post request } catch (RestClientException e) { System.out.println(e.getMessage()); }
This approach provides you with the explicit details about the error.
Checking Server Status
I/O Errors could arise if there’s a problem with the server you’re trying to reach. So, it’s highly beneficial to check the status of the remote server. In case the server is down or unresponsive, I/O errors could occur while making the POST request.
Auditing Network Parameters
These errors can also stem from incorrect network parameters such as base URL, port number, or even protocol scheme (http vs https). You should always verify these values before making the request.
Verifying Request Body Format
Using an incorrect format for the request body can trigger an I/O error. Ensure that the request body aligns with what the server-side API expects to receive.
Here’s a correct example of setting the request body;
HttpEntityrequest = new HttpEntity (new JSONObject(map).toString(), headers);
Use of Timeouts
Another common cause of these errors is a timeout by the client waiting for the server response. Adjusting timeouts set for connection and read time might resolve the problem. Resttemplate provides these options;
restTemplate.setRequestFactory(getClientHttpRequestFactory()); private ClientHttpRequestFactory getClientHttpRequestFactory() { int timeout = 5000; HttpComponentsClientHttpRequestFactory clientHttpRequestFactory = new HttpComponentsClientHttpRequestFactory(); clientHttpRequestFactory.setConnectTimeout(timeout); return clientHttpRequestFactory; }
As said by Andrew Hunt and David Thomas, “Debugging is like being the detective in a crime movie where you are also the murderer.” It encapsulates the essence of addressing I/O errors; it’s all about investigating the issue contextually, tracking down potential culprits, and then methodically eliminating each one.
RestTemplate’s Common Pitfalls and Proven Solutions to I/O Error Issues
When dealing with RestTemplate, two common issues or pitfalls developers regularly face are:
– I/O errors on POST requests.
– Difficulty in marshalling and unmarshalling request/response objects.
Now, let’s delineate a solution to the first problem of ‘I/O error on Post request from RestTemplate’.
An I/O Error essentially raises an alarm that something went wrong during the execution of an Input/Output operation, specifically when making HTTP requests. In Java, these are often represented as `java.net.SocketTimeoutException`. This particular exception is thrown when a timeout occurs during a read or acceptance message within a socket connection.
Problem | Solution |
---|---|
I/O error on Post request from RestTemplate | Adjust Timeout settings or Use WebClient where applicable |
Taking a closer look at the Post request issue, it seems the RESTful service being requested took too long to respond, there could be slow network issues, or the request may have significant latency.
Here is how you can adjust timeout setting in Java with `RestTemplate`:
RestTemplate restTemplate = new RestTemplate(); HttpComponentsClientHttpRequestFactory requestFactory = new HttpComponentsClientHttpRequestFactory(); requestFactory.setConnectTimeout(5000); // set short connection timeout requestFactory.setReadTimeout(5000); // set short read timeout restTemplate.setRequestFactory(requestFactory);
By customizing your Request Factory, you can directly set your application’s Connection and Read timeouts. The timeouts above are just placeholders, your specific use case might require larger values. Please adjust accordingly.
In the latest versions of Spring’s WebFlux Framework, RestTemplate has been deprecated in favor of WebClient, which is touted for more advanced features such as streaming scenarios and zero dependency on the Servlet API. Hence, another good practice would be to switch to WebClient.
Our coding world evolves quickly. As Brian Kernighan famously noted, “Controlling complexity is the essence of computer programming”. While solving complex tasks with neatly written code, also keep an eye out for any updates or changes that could make these tasks simpler. For further reading, Baeldung’s post offers a comprehensive guide to transition from RestTemplate to WebClient.
The challenge of overcoming I/O errors on post request from Resttemplate is an aspect that any Java developer might encounter at some stage. Let’s explore possible ways to solve this issue:
Solution 1: Check for Network Connection Issues
Firstly, ensure there are no network or connectivity issues. Sometimes, a simple connectivity glitch could lead to such problems. Make sure to verify your internet connection, proxy settings, and server availability.
Solution 2: Validation of Correct Request Parameters
A common reason for I/O errors can be incorrect request parameters. It’s important to double check whether the correct parameters are being sent with the request.
For example:
HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_JSON); HttpEntityentity = new HttpEntity ("parameters", headers); RestTemplate restTemplate = new RestTemplate(); restTemplate.postForEntity(url, entity, String.class);
Solution 3: Server-Side Error Handling
Another common reason is server-side error handling. You need to handle exceptions properly on the server side application so that it sends a meaningful response whenever there is an exception.
Solution 4: Update RestTemplate Instance
Sometimes, outdated versions can cause unknown errors. Hence, updating or modifying your RestTemaplate instance according to your needs should ideally fix the issue.
To iron out these error instances within your programming journey Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it”. Hence, always stay alert for smart solutions.
Implementing these guidelines helps ensure smooth interaction between client-server communication through RestTemplate. Learning to troubleshoot and resolve problems by yourself enhances your competency as a professional developer.