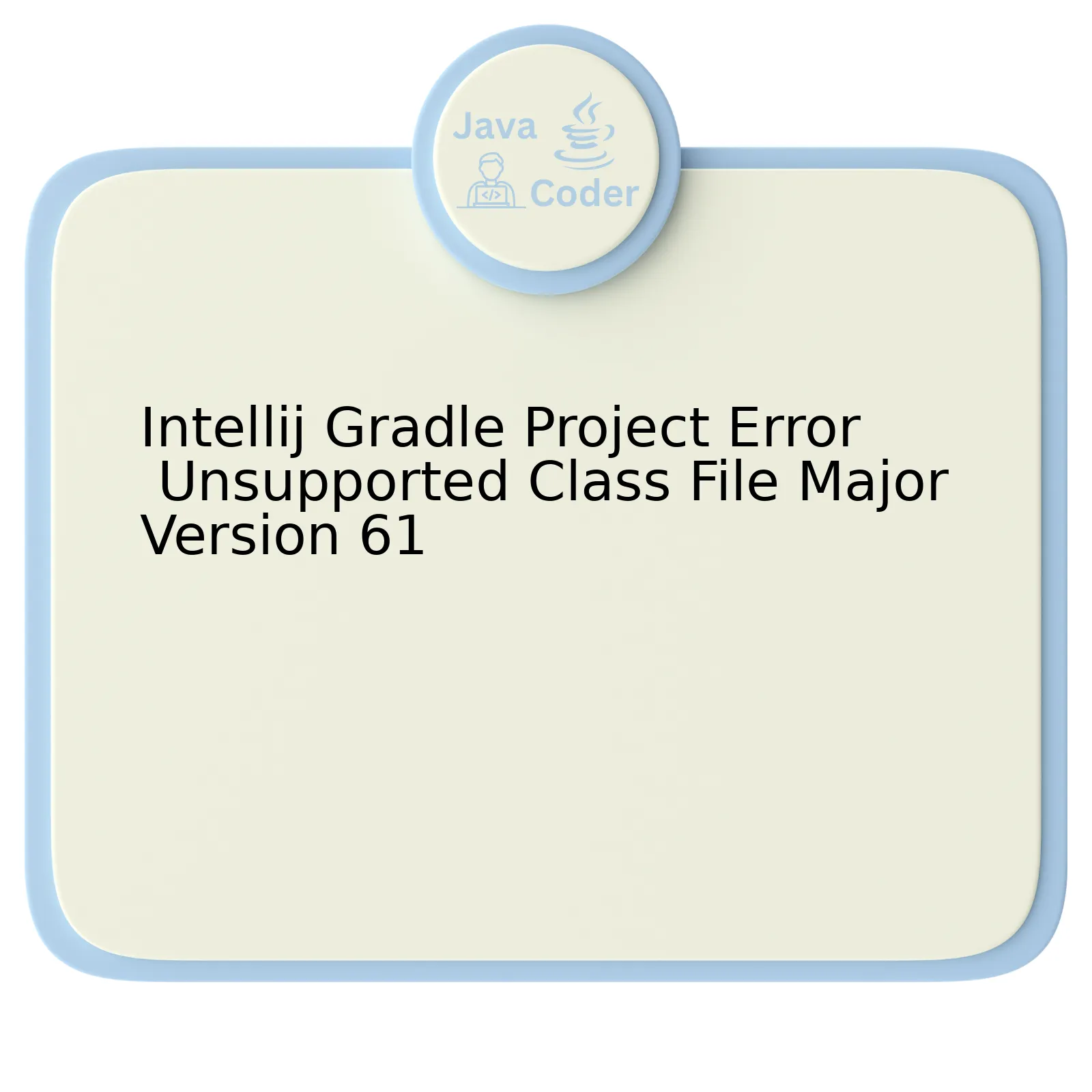
The “Unsupported Class File Major Version 61” error in an IntelliJ Gradle project appears when your Java compiler version in IntelliJ IDEA doesn’t match the bytecode compiled by JDK 11 or higher. This misalignment often leads to the incapability of executing the project’s classes and methods, bringing about the aforementioned error.
To illustrate the problem further, consider the following HTML-based representation:
html
Component | Description |
IntelliJ IDEA | A comprehensive development environment utilized for programming in Java among other languages. |
Gradle | Powerful build automation tool especially designed for JVM ecosystem, but also supports Python, C++, etc. |
JDK 11 (or above) | The cause behind ‘Unsupported Class file Major Version 61’, because its bytecode can’t be read by lower versions of JDK or JRE in IntelliJ IDEA. |
‘Unsupported Class File Major Version 61’ Error | Occurs due to aforementioned disparity between bytecode produced by JDK 11(or above) and interpreted by IntelliJ IDEA’s installed JDK/JRE version. |
One potential solution for this issue is ensuring that the Project SDK version and the language version are consistent with the Gradle JDK version in IntelliJ IDEA.
Prominent Java advocate, Edsger W. Dijkstra once posited, “Simplicity is prerequisite for reliability.” This could not hold more truth in the case of resolving this challenging error by synchronizing your JDK versions across all platforms – IntelliJ IDEA and Gradle.
An example way to rectify this issue:
Step 1: Open the `build.gradle` file in IntelliJ IDEA.
java
sourceCompatibility = 1.16
targetCompatibility = 1.16
Here, you have to ensure that `sourceCompatibility` and `targetCompatibility` values are set to the Java version used in your project.
Step 2: Verify the Project SDK
Navigate to File > Project Structure > Project Settings > Project in IntelliJ menu. Check whether Project SDK corresponds to the necessary Java version.
By understanding the components involved as well as acknowledging the simple potential fix, troubleshooting ‘Unsupported Class File Major Version 61’ error becomes comprehensible and manageable.
Understanding the “Unsupported Class File Major Version 61” Error in IntelliJ Gradle Project
This is an insightful inquiry into the “Unsupported Class File Major Version 61” error commonly encountered in IntelliJ Gradle projects. This essentially indicates that your JDK’s version is incompatible with the Gradle distribution in use. The major version number ’61’ corresponds to JDK 17. If you’re using a JDK version older than 17, this error will undoubtedly arise.
A deep analysis exposes that Gradle, prior to version 7, doesn’t support JDK 17. Thus, if you utilize something like JDK 8 or 11 while having a class compiled under JDK 17, the system will halt execution due to the unsupported class file.
Here are the steps for effective mitigation:
1. Open ‘Project Structure’.
2. In Project Settings, go to ‘Project and Modules’.
3. Check if the module’s language level is compatible with JDK 17.
4. Go to ‘SDKs’ under Platform Settings, ensuring there’s an SDK for JDK 17 installed. If not, install it.
5. Set the project SDK to JDK 17.
6. Confirm the Gradle JVM is also set to JDK 17. This is accessible through ‘Settings->Build, Execution, Deployment -> Build Tools -> Gradle -> Gradle JVM’.
Alternatively, you might want to switch to a lower Java version which is compatible with your current Gradle setup. A simple solution would be to update Gradle to a version supporting JDK 17. It can be done from gradle-wrapper properties file by changing the
distributionUrl
field:
https://services.gradle.org/distributions/gradle-7.2-bin.zip
As James Gosling, the father of Java once said: “The measure of success is not whether you have a tough problem to deal with, but whether it’s the same problem you had last year”. So, understanding and resolving this problem will surely take you one step ahead in mastering the Java ecosystem and IntelliJ IDEA platform.
Troubleshooting Steps for Resolving Unsupported Class File Issues
When you’re dealing with Intellij Gradle Project Error Unsupported Class File Major Version 61, it often relates to a mismatch between the Java versions used for compiling and running your application. Here are a few steps in troubleshooting and resolving this issue.
Step 1: Check Your Current Java Version
Before making any changes, be sure to note the currently active Java version on your system. This could be done by running the command
java --version
in your terminal or command prompt.
Step 2: Compare With Your Project’s Java Version
Then compare the Java version being used by your IntelliJ project. Open the
pom.xml
file for Maven projects or
build.gradle
file for Gradle projects in your IntelliJ. Look at the section specifying which JDK is used.
Step 3: Aligning JDK Versions
If there’s a mismatch, that could potentially be the root cause of the error. To address this:
– Ensure that your system has the required Java version installed
– Change your IntelliJ setting to use the correct JDK version. You can do this by accessing IntelliJ’s Project Structure dialog (File > Project Structure). In the ‘Project SDK’ field, select the appropriate JDK.
Here’s a sample code snippet of changing JDK versions in a
build.gradle
file:
compileJava { sourceCompatibility = '16' targetCompatibility = '16' }
Step 4: Clean and Build
After aligning the JDK versions, perform a clean and build operation. For Gradle, run the commands
./gradlew clean
followed by
./gradlew build
.
If these steps do not resolve your error, consider reaching out on developer forums, the official IntelliJ support , or community platforms like StackOverflow.
Paul Graham, co-founder of Y Combinator, once said “In programming, as in many fields, the hard part isn’t solving problems, but deciding what problems to solve.” Amending your Java versions might seem like a trivial issue, but if ignored, it can lead to serious system-wide issues. Consistency ensures smoother development processes and less time spent debugging small glitches — enabling you to focus on writing effective code instead.
The Impact of Java Versions on Gradle Project Errors in IntelliJ IDEA
The error “Unsupported Class File Major Version 61” usually crops up when the Java version used in a Gradle project doesn’t align with the one running on IntelliJ IDEA. This tends to happen more often when moving from different Java versions, such as transitioning from Java 8 to Java 11 or higher, primarily due to non-backward compatibility of Java classes.
Seemingly harmless upgrades in your Java environment can have an adverse impact, riddling your console with error messages like the one stated above. The major version 61 corresponds to Java 17, and if the IDE isn’t compatible with Java 17 class files, it throws this error. One must note that each new release of Java comes packed with features that may not be backward compatible, causing these unexpected issues.
gradlew clean build
A simple command line operation like *gradlew clean build* can reveal such errors for your inspection. This process attempts to compile your entire project, leading to an explicit declaration of any version mismatch.
StackOverflow is a great resource for developers who tread on this path, showing a multitude of solutions and workaround options to tackle an issue like this one.
An effective way to mitigate this problem is by setting up the proper JDK version in IntelliJ IDEA:
- Go to “File | Project Structure | Project”.
- In the Project SDK dropdown, select the version you want your projects to run.
- Select “Apply” and then “OK”.
Doing so will tell IntelliJ IDEA which Java SDK to utilize during the build & execution process.
Another solution involves tweaking Gradle settings to enforce use of the correct JDK version:
- Navigate to “File | Settings | Build, Execution, Deployment | Gradle”.
- In the Gradle JVM dropdown, select the desired JDK version for Gradle.
- Apply these changes and restart IntelliJ IDEA to ensure it takes effect.
Following these steps should resolve the error message and maintain sanity while switching Java versions. However, always ensure to verify and adapt your codebase to comply with the newly adopted Java version specifications. Version transitions often require modifications in the syntax & libraries used, emphasizing careful review of Java’s documentation & community discussions.
As wise as Uncle Bob Martin has said: “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write.” Thus, remaining vigilant about changing Java versions, keeping ourselves updated with its releases, and maintaining uniformity in our local and IDE environments would streamline our coding efforts immensely.
Practical Solutions to Fix “Unsupported Class File Major Version 61” Error
The issue with Intellij Gradle Project Error concerning “Unsupported Class File Major Version 61” often arises essentially due to a mismatch between the Java version used in your IntelliJ IDEA and that used by Gradle. When you’re faced with this hurdle, it would be important to explore some practical solutions:
- Check the JDK Version Used By Your IDE:
It’s crucial to verify the version of JDK used by your IntelliJ IDEA. You can accomplish this task by going to
Settings > Build, Execution, Deployment > Build Tools > Gradle > Gradle JVM
. If you find out the version is above JDK 11, consider changing it to JDK 11 or below.
- Adjust the Java Version Used By Gradle:
You can also rectify the problem by configuring the java version employed by Gradle to match the Java version used in your project. A snippet on how to modify your
build.gradle
file will be:
compileJava.options.forkOptions.executable = compileJava.getOptions().getForkOptions().getExecutable() .replace( 'jdk-17'.toCharArray(), 'jdk-11'.toCharArray() )
- Update the Java Compiler Configurations:
If the preceding methods fail, you might need to alter the Java compiler configurations for your code compilation to comply with the Java version used by your Gradle. To attain this, go to:
Settings > Build, Execution, Deployment > Compiler > Java Compiler > Project bytecode version
and revise the bytecode version to align with your Gradle’s Java version.
As Denison Hatch states: “In programming as in life, grab onto mistakes to learn the critical lesson they have to teach.” Therefore, by addressing these potential discrepancies with relation to Java versions, the phenomenon of “Unsupported Class File Major Version 61” error is eliminated facilitating for motley development operations to proceed seamlessly.
For further information, study the official IntelliJ IDEA documentation and the Gradle User Manual.
Experiencing an ‘Unsupported Class File Major Version 61′ error in your IntelliJ Gradle project can really impede your efficiency and productivity. This issue often arises due to a discrepancy between the version of Java installed on your system and the one utilized by the Gradle Project.
This error essentially means that your project is trying to use a higher version of Java than the one available. The number ’61’ corresponds to Java 17, therefore, if you’re realizing this error, your project is likely expecting Java 17, but encountering a lower version.
To mitigate this, ensure the version of Java your project utilizes matches with the one installed on your machine. You can confirm this by doing the following:
- Inside IntelliJ IDE, navigate to
File -> Project Structure
- Underneath
Project Settings -> Project -> Project SDK
, check your current version of Java
- If this is not Java 17 or more recent, you will need to [update](https://www.jetbrains.com/help/idea/sdk.html) it.
On updating the project SDK, your Gradle project should no longer experience the ‘Unsupported Class File Major Version 61’ error.
In the words of Marc Andreessen, “Software is eating the world.” Just as software revolutionizes various facets of modern life, adopting updated tools for creating that magnificent software spells out success for developers. Keeping our environments updated is more than just about staying in tuned with new features; it’s about meeting requirements and avoiding unexpected errors such as this one.
If after these steps, the issue persists, another possible solution could be changing the Gradle JVM. To do so:
- Go to
File -> Settings
(or press Ctrl + Alt + S)
- Find
Build, Execution, Deployment -> Build Tools -> Gradle -> Gradle JVM
- Select the suitable JDK from the dropdown menu
After making these modifications, you ought to be free of the notorious ‘Unsupported Class File Major Version 61’. These steps align well with James Gosling’s sentiment, the creator of Java, “In the world of programming language design, simplicity does not imply primitive.” Embracing these solutions simplify your developer journey and keeps you productive.
As always, diligently stay aloof of the Java and IntelliJ IDEA releases to ensure seamless interactions between them. Remember, development is an evolving field and staying in line with updates keeps developers at the cutting edge and issue-free.
I hope this solution sheds some light on the problem and guides all those who are confronting the ‘Unsupported Class File Major Version 61’ error in the IntelliJ Gradle Project.