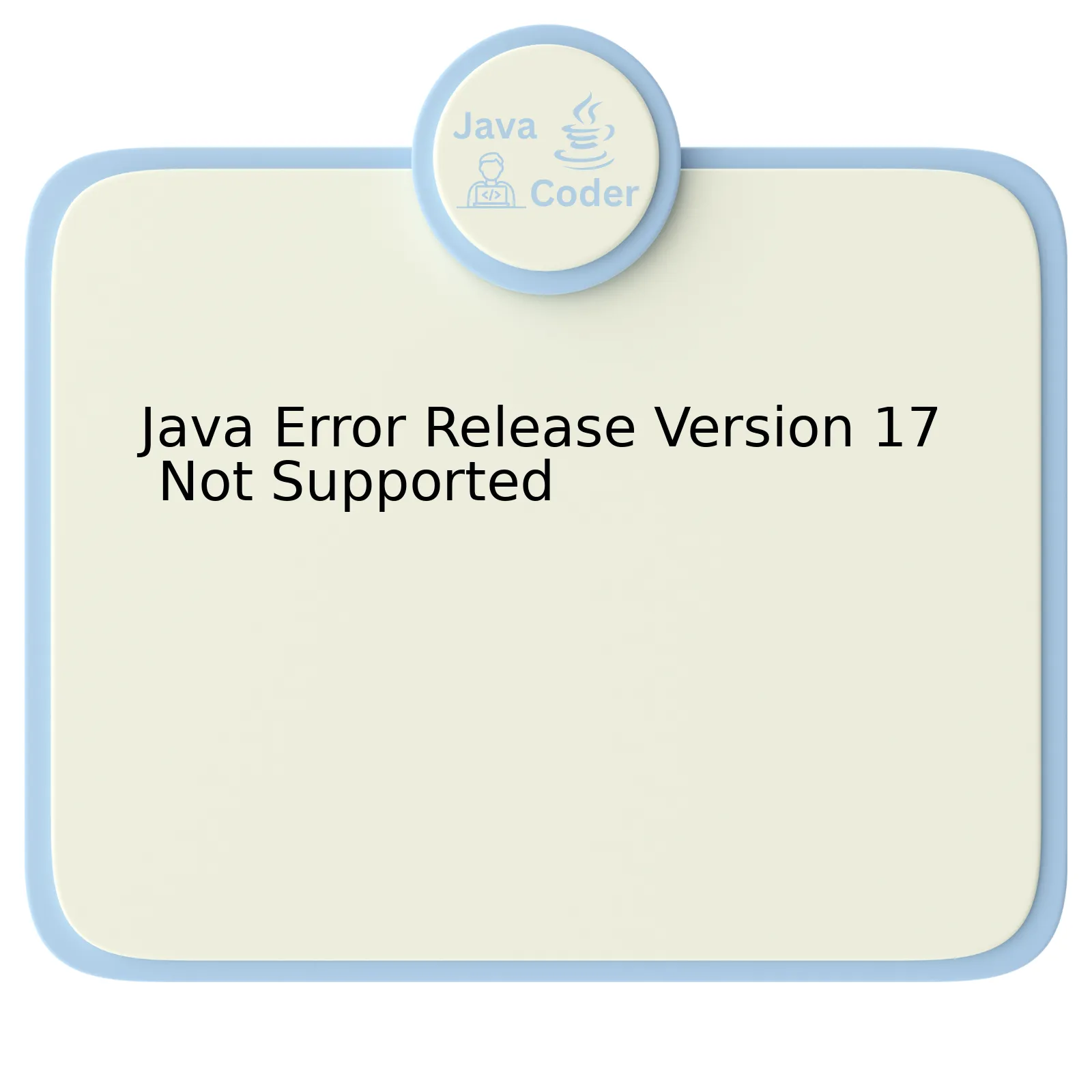
The Java Error: Release Version 17 Not Supported is an error message that a developer will often encounter when trying to run a Java project with a version of the Java Development Kit (JDK) that is not compatible with the project’s specified release version.
Here’s a simple breakdown of this process:
Factors | Description |
---|---|
Release Version |
This refers to the version of Java specified in the project’s settings or build configuration file, such as pom.xml for Maven projects or build.gradle for Gradle projects. |
JDK Version |
Your system must have a JDK installation that supports the project’s specified release version. In this case, if your specified release version is 17, you should be running JDK 17 or later. |
Error Manifestation |
When you attempt to compile or run your Java project using a JDK version older than the specified release version, you’ll encounter the ‘release version x not supported’ error. |
This error essentially suggests that you’re attempting to assert features specific to Java 17 when your JDK does not support these features. Possible solutions would involve ensuring that your JDK version aligns with your project’s required Java version. This would involve either updating your JDK version to match the project requirement, or downgrade your projects required Java version to match your existing JDK version.
_Satisficing all requirements hinges on bringing your project’s dependencies in line with your development environment. The beauty of technology is its malleability, you can always adapt it to meet your needs._ – Troy Hunt, Microsoft Regional Director and security expert.
Subsequently, you can adjust the environment variables to reflect changes made while switching between different versions of JDK on your system. The adjustments ensure that the Java commands executed in the Terminal or Command Prompt are interacting with the correct version of JDK.
Note: Following through with this adjustment will rectify the Java Error: Release Version 17 Not Supported allowing successful compilation of your Java project.
Understanding Java Error: Release Version 17 Not Supported
The Java error: Release Version 17 Not Supported is one that developers using lower versions of the Java Development Kit (JDK) may come across when trying to compile code written in Java 17. It inadvertently means that your JDK doesn’t correspond with the source version (Java 17).
Here is some insight into the cause and how to fix it.
Digging into the issue
Java has consistently evolved over the years, with ongoing updates and new versions being released regularly. These updates often include new features that older versions do not support, making them incompatible.
Consider this code block example,
public record Book(String title, String author, int pages){}
In the lines above, records are a new feature introduced in Java 14, improved in Java 15 and now standard as of Java 16. Consequently, if you try to compile this code with a JDK version lower than 16, you might encounter an error such as “Release Version n Not Supported,” where n is the Java version number which provides full support for that feature (e.g., 14 or 16).
Fixing it: The upgrade approach
One way around this error is to upgrade your JDK to a more recent version that supports the java source version causing the error:
– First and foremost, download the new JDK. Navigate to the Oracle downloads page and follow their instructions.
– After installing it successfully, ensure your IDE is configured to use the new JDK. This step varies depending on your chosen IDE; consult your IDE’s documentation for guidance.
Secondary Fix: The ‘change the source’ approach
Alternatively, you might consider downgrading your source code to eliminate usage of newer features unsupported by your current JDK.
Fixing this issue involves identifying the features that provoke the error and replacing them with equivalents supported by the lower version.
However, this approach can be time-consuming and counterproductive, especially considering Java’s consistency in releasing new updates with improvements and new features. Hence, upgrading your JDK takes precedence.
“The only way that we can live, is if we grow. The only way that we can grow is if we change. The only way that we can change is if we learn,” this quote by C.JoyBell C. perfectly captures the essence of dealing with programming language evolution.
Troubleshooting the Issue: Invalid Or Unsupported Class Version Error
The
UnsupportedClassVersionError
in Java is a subclass of the
ClassFormatError
class. It’s thrown by the Java Virtual Machine (JVM) when it attempts to read a class file and determines that the version numbers in the file are not supported. When working with release version 17, one might especially encounter an “invalid or unsupported class version error” if attempting to run a higher-level bytecode on a lower-level JVM.
With respect to the JVM and bytecode interaction, the bytecode-version is often higher than the JVM version due to the following circumstances:
– Development was done in a newer version of Java than is available on the environment where it’s being executed.
– A third-party library that has been compiled with a more recent version of Java is being used.
To illustrate this issue with a practical example, if you’ve compiled a Java program with JDK 17, and you’re trying to run it on JRE 16, the JVM will raise an
UnsupportedClassVersionError
since JRE 16 does not recognize the bytecode generated by JDK 17.
To resolve the ‘Java Error Release Version 17 Not Supported’ occurrence, here’s what you should do:
* **Verify Your Java Versions**: Use the command line prompts
java -version
and
javac -version
to check the versions of your JRE and JDK respectively. They should both correlate with version 17 for smooth operation.
* **Update Your Java Version**: If your JRE is outdated, you’d want to download and install the latest version corresponding with your bytecode from the official Oracle website.
* **Recompile Your Source Code**: If possible, recompiling your source code under an older JDK version which matches your runtime environment can also fix the issue.
As Kent Beck once noted, “I’m not a great programmer; I’m just a good programmer with great habits”. Establishing precise development habits, such as ensuring compatible Java versions across your development and production environments, aids in preventing issues like the ‘Java Error Release Version 17 Not Supported’ and promotes more efficient coding practices.
Mitigating Factors Leading to ‘Version 17 Not Supported’ Error
The ‘Version 17 Not Supported’ error in Java development environment is one of those bugs that can give developers a hard time. It typically comes up when you’re attempting to compile or execute your Java applications using an unsupported version of the Java Development Kit (JDK). The error message indicates that you’re likely trying to use a newer JDK release which the presently used tools and software cannot handle.
“Write once, run anywhere, is as true for the Application Programming Interface (API) as it is for user program code.” – James Gosling
Let’s further evaluate the sources and tactics to troubleshoot this issue:
Main Causes of this error:
-
Mismatch between the Java Compiler and JDK: The tools you are using to develop may still be aligned to work with older version releases of JDK.
-
Outdated Integrated Development Environment(IDE): Using older versions of IDE such as Eclipse, IntelliJ IDEA, or Netbeans which don’t support Java SE 17 yet can trigger this error.
-
Incompatible Libraries/Frameworks: Some libraries or frameworks you’re utilizing might not have updated their system to cater to JDK 17, primarily if the library/framework relies on internals and undocumented features.
Possible Solutions:
-
Update Your Tools:
Each time Oracle brings out a new JDK release, various software and tools will need to update itself accordingly to support the changes. Be sure to check whether new versions are available for your Java compiler, IDE, and other build tools.
-
Revert back to a supported JDK Version:
In the interim period while waiting for update releases from your tools, a feasible workaround could be to switch back to use a JDK version your current tools and software supports.
-
Befriend Maven or Gradle:
If the problem persists due to incompatible libraries, integrating Maven or Gradle into your Java projects can help, as these tools can automatically manage the compatibility of different java versions across your project dependencies.
//Updating JDK on terminal
sudo apt install openjdk-17-jdk//Updating IDE
Help -> Check for Updates
//Changing JDK version on terminal
export JAVA_HOME="/usr/lib/jvm/java-11-openjdk-amd64/"
Remember, staying updated about the dynamic ecosystem surrounding Java technology would go a long way in mitigating such errors caused by versioning.
For more details, you can refer to this Stackoverflow thread.
Exploring Solutions for Java Release Version Compatibility Issues
Java Release Version Compatibility Issues commonly arise in many programming environments and most prominently, when an attempt is made to compile the Java code with a higher JDK version than what’s installed on your machine. Specifically, the “Java Error Release Version 17 Not Supported” issue is encountered primarily because your system does not have JDK 17 or a higher version available.
A perfect illustration of this compatibility problem occurs if you attempt to compile your Java application that was originally coded with JDK 17 but your local development environment only has Java Runtime Environment (JRE) 11 or JDK 8 installed. In such instances, a clash of versions might generate an error similar to “Release Version 17 Not Supported”.
To illustrate, here’s a potential error message that could appear:
Error:(26, 1) java: cannot find symbol Symbol: class Stream Location: package java.util
This implies the “Stream” class isn’t recognized because it was introduced in later Java versions, separate from the one currently set as the application’s path.
Resolving these issues revolves around ensuring a match between the JDK version used for coding and the JRE/JDK version deployed within the targeted operating environments.
Here are three potential solutions:
– Upgrade the Local JDK: Download and install the required JDK version that matches the release version of the Java program. For instance, if your codebase depends on JDK 17 features, installing JDK 17 on your local machine will solve the issue. You may download the required JDK version from the official Oracle website or any other trusted source.1
– Code Refactoring: You can alternatively refactor your code to be compatible with your current JDK version. This involves replacing methods, classes or functions introduced in later Java versions with ones compatible with your existing JDK version. Whilst this is labor-intensive and time-consuming, it becomes necessary if upgrading the JDK isn’t feasible.
– Setting the Target Source and Runtime: Configuring your Integrated Development Environment (IDE) to target the appropriate source and runtime version can also eliminate compatibility problems. Adopting this approach ensures that your compiled bytecode is compatible with older Java versions, making it possible for your Java application to run without hitch on your current JDK installment.
For example, in Apache Maven, the Maven Compiler Plugin can be used to specify the Java version during project compilation:
<properties> <maven.compiler.source>1.7</maven.compiler.source> <maven.compiler.target>1.7</maven.compiler.target> </properties>
In summary, steering clear of version compatibility issues in Java requires either aligning your development environment with the JDK version utilized when writing your original code or tailoring your code to correspond with your current JDK version. Being vigilant about the JDK version in use at every stage of your software development life cycle, essentially remains the key to averting these issues.
Like Ada Lovelace, a pioneer of scientific computing said, “The Analytical Engine has no pretensions whatever to originate anything. It can do whatever we know how to order it to perform.”2 Likewise, the Java compiler follows commands precisely and accurately, and having the correct JDK version prevents avoidable errors.
Addressing the Java Error ‘Release Version 17 Not Supported’ requires careful deliberation. Essentially, this error occurs when there is a version mismatch between the Java Development Kit (JDK) version you are using to compile your java file and the Java version that your system recognizes. Here’s how to handle it:
The foremost solution to this problem is to ensure consistency between your system’s Java version and the JDK version used for compilation. You can verify your system’s Java version by running
java -version
in the terminal.
Action | Description |
---|---|
Upgrade System’s Java Version | If the system’s Java version is lesser than JDK 17, consider updating it. For instance, you could reinstall the newer version of Java or update your JAVA_HOME environment variable if possible. |
Downgrade JDK Version | Alternatively, if upgrading the system’s Java isn’t an option, you could downgrade the JDK version to match your system’s Java version. Irrespective of the IDE being used, you can adjust the compiler settings easily. |
An in-depth understanding of the underlying Java versions in use is paramount.
As Grace Hopper, a computer scientist pioneer said, “The most dangerous phrase in the language is, ‘We’ve always done it this way.'”. Staying open to adaptation, such as version upgrades, can solve not only the ‘Java Error: Release Version 17 Not Supported’, but also other potential version-related conflicts.
For more details, you may refer to official Oracle documentation.