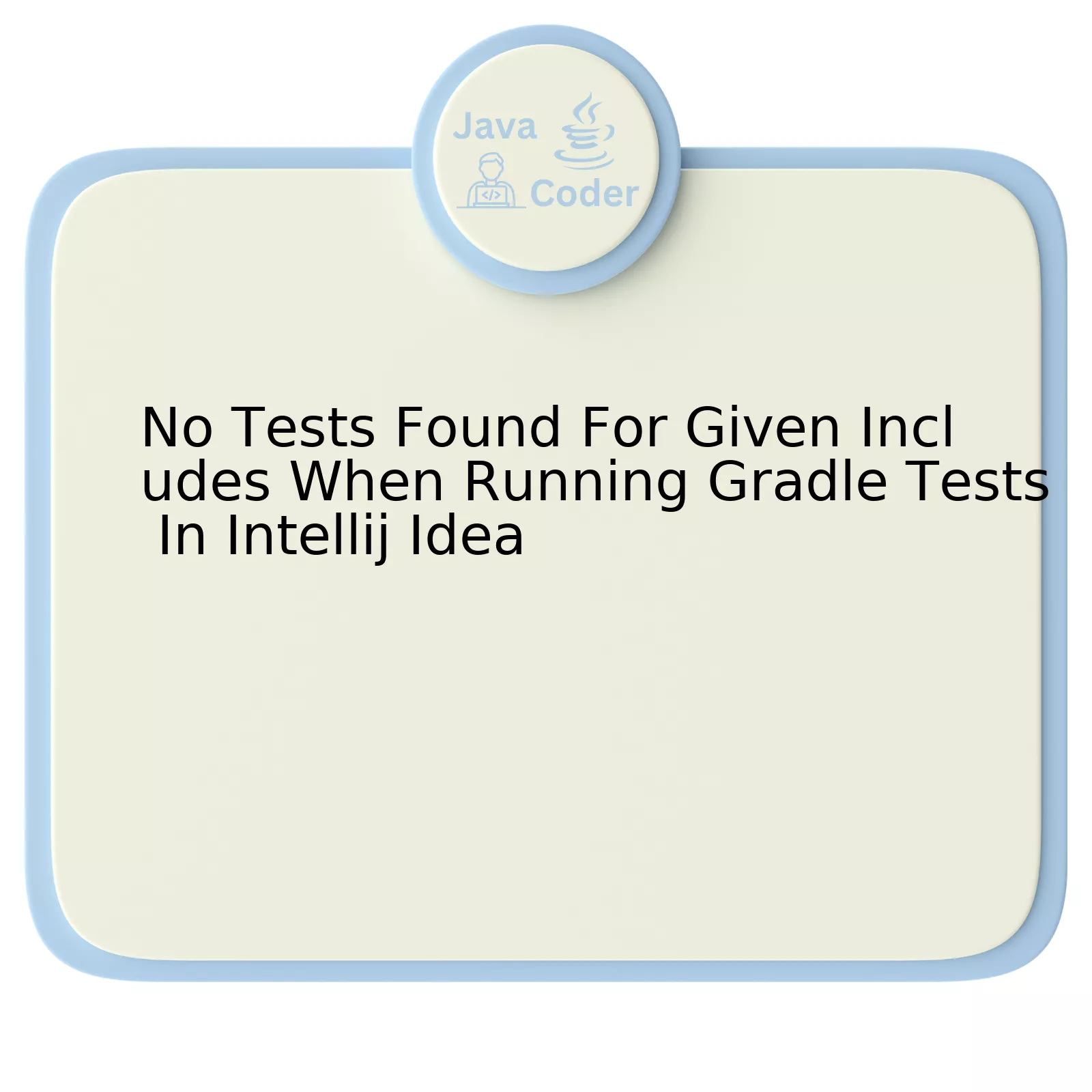
Issue | Causes | Solutions |
---|---|---|
No Tests Found For Given Includes |
|
|
This tabular arrangement helps to isolate the problem – “No Tests Found For Given Includes”, when running Gradle tests. Listed alongside are potential causes and corresponding solutions which could aid in resolving this prevalent issue.
Among the common causes is misconfiguration of the Gradle build script. In spare words, the building and testing rules written within the `build.gradle` file may not be articulating correctly, the set of tests to run. To circumvent this, the solution would involve adjusting the Gradle build script for including the right patterns, conforming to what the test suite contains.
Another cause could be related to the usage of parameterized tests not supported by the IntelliJ IDEA test runner. Parameterized tests are commanding as they allow developers to run the same test over different inputs without code duplication. However, these need specific runners to perform optimally. Navigating out of this bottleneck would mean either switching to a plain JUnit or TestNG setup that doesn’t use parameterized tests, or use a different test runner in IntelliJ IDEA that supports them.
The last potential cause lies within outdated or incompatible software versions. The IntelliJ IDEA IDE, Gradle build tool, or the JUnit testing framework if not seamlessly incorporated, might run into compatibility issues leading to the “No tests found” prompt. Keeping these systems updated with stable releases can majorly alleviate such hitches.
As quoted by tech-guru Robert C. Martin, “When you find a bug, the very first thing you should do is write a test that exposes it.”. So let’s focus on having our tests runnable, because indeed, testing goes beyond finding and exterminating bugs, it assures quality and saves time and costs in the long-run.
Understanding the Issue: No Tests Found For Given Includes
When you run Gradle tests in IntelliJ IDEA, you may occasionally encounter an error message that reads “No Tests Found For Given Includes.” This issue essentially arises due to mismatches between the test classes or methods and the included patterns defined in your configuration.
Before diving into the specific causes and solutions, it’s important to clarify what the term “Given Includes” means. It pertains to a set of patterns that define which test classes or test methods should be involved in the testing process.
Causes
The essential cause of the “No Tests Found For Given Includes” error would generally fall into three categories:
- Configuration Issues: This refers to the situation where the specified test class or method does not match with the ‘includes’ pattern listed in the configuration file.
- Naming Convention: Java, as well as many IDEs, follows certain naming conventions for test classes and methods. If these are not adhered to, test discovery can fail, leading to this error.
- Test Dependencies: If your project is missing necessary test dependencies, such as JUnit or TestNG, the tests may not be discovered properly.
Solutions
Understanding the potential causes enables us to apply appropriate solutions:
- Verify Configuration: Review your configuration settings and ensure that the ‘includes’ pattern matches your test classes or methods structure.
- Check Naming Conventions: Make sure that your test classes and methods follow standard Java naming convention. Typically, test classes have names ending with ‘Test’, and test methods are annotated with @Test.
- Add Necessary Dependencies: Ensure all necessary dependencies like JUnit or TestNG are added in your Gradle build file.
This quote from Linus Torvalds, the developer of the Linux kernel encapsulates it perfectly:
“Talk is cheap. Show me the code.”
To demonstrate a basic configuration for running JUnit, let’s consider this trivial snippet:
dependencies { testImplementation 'junit:junit:4.13.1' } test { useJUnit() include '**/*Test.java' }
If your test classes are following the “*Test” naming convention, and are within directories covered by `**/*Test.java` pattern, then Gradle should find them without error.
Remember to use UTF-8 encoding for all your Gradle files and test files. Actually, IntelliJ IDEA normally uses UTF-8 by default; but as a double check, do verify under File > Settings > Editor > File Encodings.
Please refer to JetBrains official documentation for more detailed information on configuring testing libraries in IntelliJ IDEA.
Remember, correct configuration is critical in resolving the “No Tests Found For Given Includes” error when running Gradle tests in IntelliJ IDEA.
Revisiting Gradle Configuration in IntelliJ IDEA
While discussing Gradle configuration in IntelliJ IDEA, it’s important to understand its relation with test discovery problem, specifically addressing the issue of “No tests found for given includes” when running Gradle tests in IntelliJ IDEA. This is a common error that developers may encounter and generally stems from configuration or setup issues.
Gradle’s powerful capabilities for managing project dependencies and coordinating builds can sometimes generate complexities during integration with IDE like IntelliJ IDEA. Among these complexities lies the test discovery – not locating JUnit tests appropriately. IntelliJ interacts with Gradle to generate and manage project settings, hence any discrepancies may result in failed test discovery.
Finding the root cause
The first step involves diagnosing the exact issue leading to the error. Some culprits might include:
* Mismatched names between the testing class and test method name in the Gradle configuration file
* Incorrectly configured build.gradle file, often missing apply plugin: ‘java’ or apply plugin: ‘jacoco’
* Problems arising from a multi-module project where each module lacks an individual Gradle file
Strategies for solutions
Once the root cause has been identified, next comes implementing the solution. Here are some strategies to solve the test discovery problem:
task test(type: Test) { include '**/*MyTestClass.*' }
apply plugin: 'java' apply plugin: 'jacoco'
Always update IntelliJ Idea
Ensuring IntelliJ IDEA is up-to-date proves essential too. Multiple versions of IntelliJ IDEA have introduced better support for Gradle projects and enhanced provisioning for linked Gradle tasks. Updating your IntelliJ IDEA could resolve the issue automatically, as newer versions come with bug fixes and improvements.
Alternative … Run Gradle Tasks within IntelliJ IDEA
If the problem persists despite these adjustments, try running Gradle task within IntelliJ IDEA instead of CLI (Command Line Interface). You can access this through:
View -> Tool Windows -> Gradle -> Tasks -> application -> run
As Martin Fowler, a renowned software developer stated, “When you’re dealing with automation, it’s paramount to get fast feedback, comprehensible feedback, and thorough feedback.” Through such measures, it ensures your development process works smoothly and effectively by avoiding errors like “No tests found for given includes”. It reiterates the role Java dependencies and project configurations play in shaping the application build and execution phase.
Resolving “No Tests Found” Error During Gradle Execution
The “No Tests Found” error during Gradle execution, specifically in context to IntelliJ IDEA, can be a hurdle particularly when you are trying to conduct precise tests for specific includes. Ferry Biggelaar, the well-known software developer and open-source advocate, once said: “To solve a problem, you need to understand it from its roots.” That’s why we first need to comprehend the core of the matter.
Gradle is acknowledged globally for its adaptability when it comes to scripting tests. However, there are scenarios where an individual might stumble upon a ‘No Tests Found For Given Includes’ error while running Gradle tests in IntelliJ IDEA. This usually results due to configuration mishaps or discrepancies caused due to version inconsistencies.
Let’s glance through crucial factors impacting this situation and effective solutions for each scenario.
Scenarios | Solutions |
Mismatching JUnit Version | Make sure that the JUnit version used by Gradle and IntelliJ IDEA alike. Inconsistencies among versions may lead to the failure in recognizing test cases. Synchronize them to a similar variant. Update them in your build.gradle file as needed. |
Wrong Test Configuration | Ensure that the Test kind is set correctly in Run/Debug Configurations. By default, IntelliJ should pick up the ‘Gradle Test’ runner. If not, you may manually set it to run with ‘Gradle Test’, ensuring that IntelliJ directly uses the Gradle runner to operate the tests. |
Inaccurate Test Directory Structure | Verify that the project follows a standard directory layout that complies with Gradle’s default settings. The typical path should resemble
src/test/java/YourTest.java . If the test cases do not follow the Maven or Gradle conventions, you will need to configure the sourceSets in the build.gradle file. |
Improper Annotation of Test Method | Certify that all test methods are accurately annotated with the
@Test directive. Unannotated test methods might not be identified by IntelliJ, which could lead to a ‘No Tests Found’ message being displayed. An example of an adequately annotated test method would look like this: @Test |
Incorrect Usage of Gradle Wrapper | Ascertain the application of the correct Gradle wrapper within your project. Navigate to `File -> Settings -> Build, Execution, Deployment -> Build Tools -> Gradle`, knocking down to the ‘Gradle Home’. Here, mark the checkbox ‘Use Gradle from’ and select ‘wrapper task in Gradle build script’. |
Keep in mind these potential circumstances and their resolutions. Yet sometimes, ‘No Tests Found’ errors might be due to multiple factors intertwined together. Therefore, analyze your unique instance, observe what matches your case best from the scenarios mentioned above and follow the advised solutions concurrently.
For additional materials on the subject, you may visit resources such as official Gradle [docs] or IntelliJ IDEA [support pages].
Best Practices for Running Gradle Tests Successfully in IntelliJ IDEA
IntelliJ IDEA is a sophisticated integrated development environment (IDE), beloved by many Java developers for its robust capabilities and friendly interface. But as with any tool, effective use requires knowledge of best practices. In this case, encountering “No Tests Found For Given Includes” when running Gradle tests can be particularly challenging. Here are key practices you should adopt to avoid this error:
1. Ensuring Correct Project Structure
Your IntelliJ project structure needs to accurately reflect your desired test environment. It should be consistent with the Gradle standard directory layout. Without this consistency, the IDE may not locate your tests.
For instance, your source code should be in
/src/main/java/
, while tests should reside in
/src/test/java/
.
project
├── src
│ ├── main
│ │ └── java
│ │ └── yourpackage
│ └── test
│ └── java
│ └── yourpackage
This way IntelliJ knows where your tests are – crucial to prevent “No Tests Found” errors.
Synchronize Intellij IDEA and Gradle Settings
Another common issue arises from discrepancies in configurations between IntelliJ and Gradle. To ensure smooth operation, synchronize settings in both environments regularly:
-
TASKS—>build—>build
on the Gradle menu to recompile.
- Upon completion, use
Create Test...
option to create a new test file.
- After creating your test, run
./gradlew clean build -i
in the terminal to rebuild using Gradle and check everything runs correctly.
If IntelliJ still presents trouble running tests inside the IDE, an effective hack is circumventing it altogether. Running tests outside IntelliJ IDEA is achievable via Terminal or Command Prompt:
./gradlew cleanTest test --info
Keeping Gradle and IntelliJ Up to date
Always maintain an updated version of both Gradley and IntelliJ IDEA. Both organizations continually make improvements to their products, which often includes solving bugs that might affect test discovery and execution.
- In Gradle, use the wrapper and specify the version in your
gradle-wrapper.properties
file.
- In IntelliJ IDEA, always update to the latest version through
Help--> Check for Updates...
.
These steps enhance communication between the two platforms, likely preventing the issue at hand.
Scott Hanselman, a prominent technologist once said, “The only way to go fast is to go well.”
It emphasizes the importance of adhering to best practices in software development and using your tools effectively – they help you ‘go well’ and ultimately, ‘go fast’. So, following these practices will surely enhance your efficiency when running Gradle tests in IntelliJ IDEA.
When working with Intellij IDEA and utilizing Gradle for your builds, it’s not uncommon to encounter the ‘No Tests Found For Given Includes’ error. This situation usually arises when there’s a discrepancy between the test environments or due to specific configuration issues.
For starters, let’s understand that
Gradle
is an open-source build automation system. It addresses several significant aspects of modern software development practices such as convention-over-configuration, flexibility, and performance.
Addressing the issue requires keen attention to how your tests are configured within the IntelliJ IDE.
- The first thing to do is verify if your project modules are correctly marked as Test Source Folders. These should rightfully be colored green in your file structure. Misconfiguration at this level could lead to IntelliJ not being able to find your tests.
- Another point to examine is your test class names. If they don’t follow the convention of ending with the word “Test”, then InteilliJ might disregard them during the test discovery process.
- Ensuring you have properly defined paths in your SDK setup could also improve the situation. Your Gradle JVM setting needs to align with your project SDK.
- If you still can’t figure out why your tests aren’t running, it may be worth trying to rebuild your project or check your setup compared with another similar project that works.
Adopting these strategies should mitigate most cases of the ‘No Tests Found For Given Includes’ issue.
According to Kent Beck, who is one of the pioneers in software development and particularly known for his work on Extreme Programming, says: “I get paid for code that works, not for tests, so my philosophy is to test as little as possible to reach a given level of confidence”.
Action Points |
---|
Verify if your project modules are correctly marked as Test Source Folders |
Check your naming conventions for test classes |
Ensure proper definition of paths in your SDK setup |
Consider doing a complete rebuild of your project if all else fails |
Nevertheless, remember that sometimes the anomaly might not be with IntelliJ IDEA or Gradle, but with the coding practices. The goal is to maintain resilient, efficient, and seamless operations, and this underscores the need to familiarize oneself with the basic principles of unit testing and integration testing. You can read more about Gradle from its official website here and IntelliJ IDEA here.
Understanding these considerations provides affirmations that even in the face of challenges, developers possess versatile tools in IntelliJ IDEA and Gradle to troubleshoot hitches such as the ‘No Tests Found For Given Includes’.