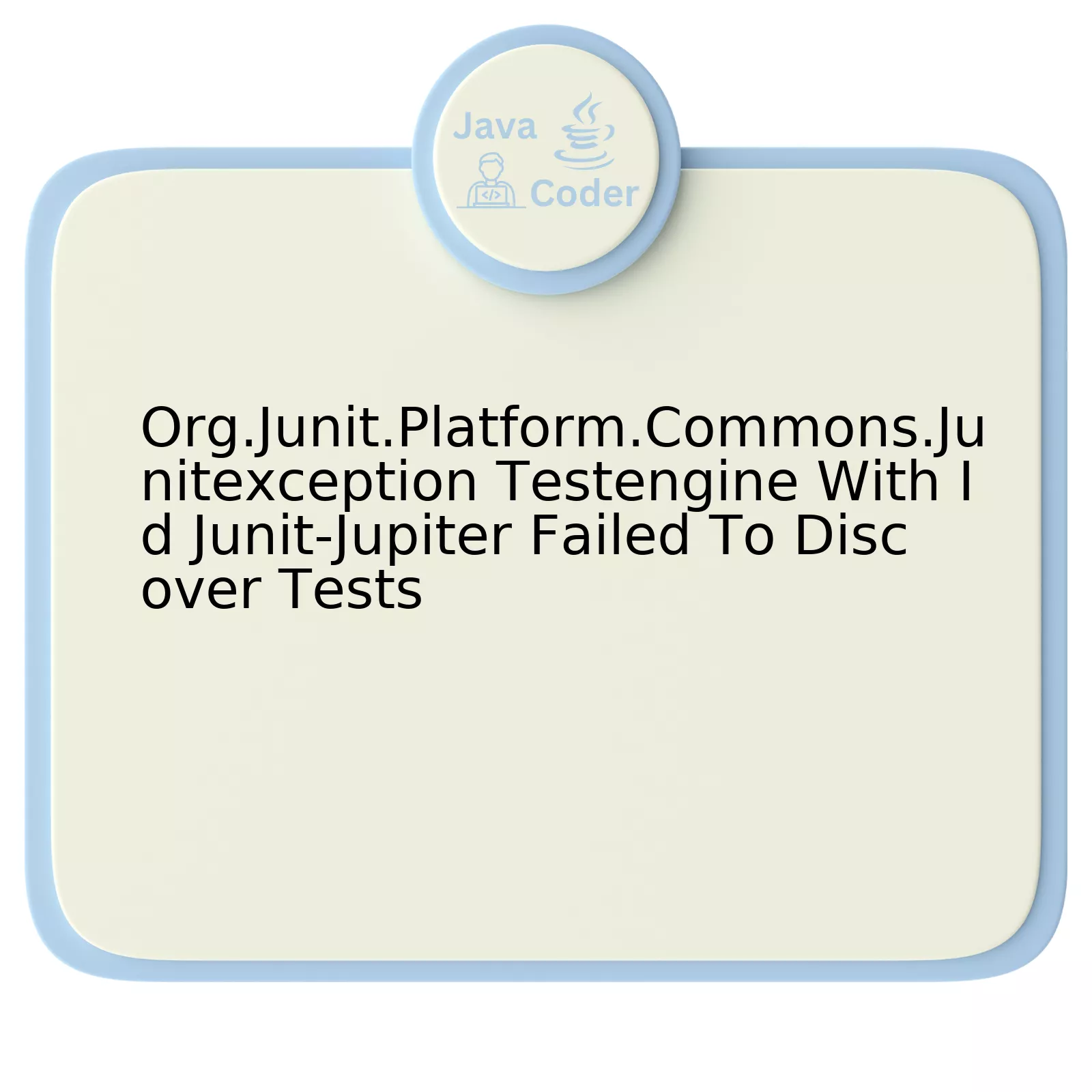
The `org.junit.platform.commons.JUnitException` is typically thrown when the JUnit test engine, designated as ‘junit-jupiter’, encounters difficulties in discovering or reading tests. The error message indicates that the test engine with id ‘junit-jupiter’ failed to discover tests.
This exception often occurs due to various reasons:
Causes | Solution |
---|---|
Issue with classpath | Ensure correct classpath configuration either manually or using build tools like Maven or Gradle. |
Mismatched JUnit version | Update to the latest JUnit 5 version that includes Platform, Jupiter and Vintage. |
No Test classes/methods found | Verify that at least one class contains methods annotated with @Test. |
Incorrect usage of annotations | Use @Test annotation on public non-static methods, not on the class itself. |
Moving just a bit deeper into this scenario, when tasks request for test discovery from the JUnit platform, it’s usually performed by a set of engines and each engine has a unique ID. Here, the engine with the ID, ‘junit-jupiter’, is essentially the one responsible for running the JUnit 5 tests. However, the said error might arise if there are no executable tests available or due to a faulty classpath configuration mentioning a wrong or non-existing directory.
Addressing the potential solutions as noted above, they generally involve making sure your classpath points to the correct locations of your compiled test classes and that you’re appropriately using the JUnit annotations. Complementary to this, one must ensure that their project’s dependencies align with JUnit5 Verses Junit4. These solutions, provided they are implemented meticulously, should resolve the exceptions generated.
“As long as your strategizing depends on what others can understand, you’ll find Java delivers. It’s easier to think through problems in Java. It’s easier to review code.” – Daniel Asharov, Software Developer
Understanding the JUnitException: TestEngine with ID junit-jupiter Failure
Understanding the
JunitException: TestEngine with ID 'junit-jupiter' failed to discover tests
requires familiarizing yourself with the nuances of JUnit, a simple open-source framework for writing and running repeatable tests in Java.
The error message signifies that the JUnit Jupiter engine was not able to discover any tests. This is generally due to incorrect configuration or problematic test setup or simply because there are no viable tests to be found by the JUnit Jupiter engine.
There could be several reasons why this exception occurs:
– No valid tests
– The existence of valid tests in your project makes sure that the JUnit Jupiter engine has something to scan and thus, perform its functions. If there aren’t any, it would certainly throw an exception regarding the failure of discovery.
– For instance, in JUnit 5 (which incorporates the JUnit Jupiter testing engine), tests must be annotated with
@Test
. Without this, or if the classes do not comply with JUnit 5 structure, the discovery process fails.
Java Class File Structure |
---|
public class ExampleTest { |
– Incorrect Configuration:
– Configuring your IDE build path properly, setting up the required dependencies (like the junit-jupiter-api and junit-jupiter-engine) using tools like Maven/Gradle is crucial.
– Ensure you are using a version of the java compiler compatible with the JUnit Jupiter version you’re employing. In general, JUnit 5 requires Java 8, and attempting to use an older version might result in the aforementioned issue.
In the words of Linus Torvalds, “Good programmers worry about data structures and their relationships”. Thus, understanding the intricate relationship between your tests and JUnit’s way of operating helps prevent errors like these.
For resolving this issue, cross-check your tests and configurations. Make sure the tests defined are structured properly following all the necessary JUnit guidelines. Furthermore, ensure your project’s dependencies are correctly set up. More on these topics can be found in the JUnit 5 User Guide.
Remember, proper debugging and elimination of potential scenarios can effectively foresee this situation during development, thereby making your software robust and reliable from inception.
The Cause and Implications of Failed Test Discoveries in Junit-Jupiter
The cause and implications of failed test discoveries in Junit-Jupiter are typically a result of complex configurations or underlying code issues. If we view this problem in the context of the error “org.junit.platform.commons.JUnitException: TestEngine with ID ‘junit-jupiter’ failed to discover tests”, it becomes evident that discovery errors often stem from certain common conditions.
Cause | Implication |
---|---|
Misconfigurations in Junit-Jupiter setup |
If the testing environment is not configured correctly, Junit may fail to locate and run your tests, leading to this error. These setups can include markers, filters, or selectors that do not align with your test classes. |
Incompatibility between Junit Jupiter's version and other libraries |
Sometimes, the Junit-Jupiter engine might be incompatible with the version of Spring Boot or any other testing library you use. In such cases, you observe this error. |
Underlying coding issues |
Incorrect usage of annotations, access modifiers of test methods or classes, and invalid method signatures can also lead to failed test discoveries. Using private visibility on testing methods, for example, can interfere with Junit’s capability to identify and execute those classes. |
External factors including but not limited to JVM bugs |
Though quite rare, issues with your Java Virtual Machine (JVM) can contribute to this error. |
By understanding the root cause behind such errors, swift and precise action can be taken to correct these flaws and maintain a well-functioning, efficient development workflow. Solutions vary by cause, but generally involve inspecting and configuring your testing setup carefully, ensuring compatibility of your tools, applying appropriate coding practices, or making sure your JVM functions properly. The debugging process can be made more effective by employing the Gradle’s
--debug
or Maven’s
-X
option to get detailed logs about the issue[1].
It is also important to consider that detecting and rectifying failing test discoveries promptly can prevent potential project pitfalls which might delay delivery timelines, causing increased costs and overheads. As software developer Jeff Atwood wisely stated, “Software testing is a sport like hunting, it’s bughunting.”
[1] Debugging Junit errors on Stackoverflow
Resolving the Common Issues Leading to JUnit Jupiter Test Discovery Failures
The `org.junit.platform.commons.JUnitException TestEngine` with ID ‘junit-jupiter’ failed to discover tests is a commonly encountered issue when using JUnit Jupiter for testing in Java. There are numerous reasons why this previously working setup may begin to fail, hindering the discovery and execution of your unit tests. These include but aren’t limited to classpath issues, compatibility problems between JUnit versions, incorrect test annotations, and improper use of assertion methods.
Correcting Classpath Issues
A typical scenario that can lead to this exception occurs when the classpath isn’t configured correctly. Be sure all necessary dependencies including JUnit Jupiter Engine, JUnit Jupiter API and JUnit Platform Runner are added and are accessible in the classpath. Here is an example of a correct Maven dependency:
<!-- JUnit 5 --> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.7.0</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.7.0</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.platform</groupId> <artifactId>junit-platform-runner</artifactId> <version>1.7.0</version> <scope>test</scope> </dependency>
Addressing Compatibility Issues
Another significant cause of the aforementioned exception is conflict or incompatible version among JUnit platform libraries. While resolving these, ensure that you’re using compatible versions of JUnit Jupiter and JUnit Platform Runner. It’s often best to use the latest stable release of all your JUnit components.
Inappropriate Use of Test Annotations
You may encounter this issue if you’re unknowingly using explicit or residual JUnit 4 test annotations (e.g.,
@RunWith
,
@Test
) instead of their JUnit Jupiter counterparts such as
@ExtendWith
and
@Test
. Replace any old annotations with those supported by JUnit Jupiter.
Incorrect use of Assertion Methods
Finally, similar compatibility issues can arise if you use JUnit 4 style assert methods (like
assertEquals(condition)
) instead of JUnit Jupiter’s assert methods (like
Assertions.assertEquals(condition)
). A shift towards using JUnit Jupiter’s preferred methods can alleviate this problem.
The wisdom from Linus Torvalds, the principal developer of Linux, serves us well here: “Good programmers know what to write. Great ones know what to rewrite (and reuse).” Remembering this, revisit problematic code sections for potential revisions that lean into JUnit Jupiter’s capabilities better. In addition, continue to explore resources like [JUnit 5 User Guide], [Baeldung’s JUnit 5 Guide] or StackOverflow to further your understanding and troubleshoot these perplexing issues.
Best Practices for Avoiding junit-platform-commons-JUnitException during Testing
When dealing with the error “
org.junit.platform.commons.JUnitException: TestEngine with id 'junit-jupiter' failed to discover tests
“, it emanates from a myriad of reasons. This error might occur if the testing frameworks in use are incompatible, or code obfuscation is causing conflicts among others. To mitigate the risk of encountering this error message during your testing process, consider applying the following best practices:
Ensuring Compatibility of the Testing Frameworks
This error could be due to incompatible versions of JUnit 5 (Jupiter) and other testing frameworks in use, such as Mockito or Spring Boot. You must ensure that all critical dependencies in your test classpath are compatible with each other. Check the versions you are using and potentially upgrade or downgrade to have synchronized versions that are known to work well together. For instance, Baeldung offers an excellent guide on how to make sure everything is compatible.
Avoiding Code Obfuscation During Unit Test Execution
Consider disabling any code obfuscation layers such as ProGuard while executing unit tests. Code obfuscation can cause renaming or removal of essential properties utilized in reflections like field names and method names leading to
JUnitException
errors.
Importing The Correct Assertions and Annotations Library
There’s always a possibility of importing incorrect libraries, especially when your IDE auto-imports classes. Make sure that you are importing Assert and related annotations from “
org.junit.jupiter.api.Assertions
” and not from other similar but different libraries.
Avoid Using Static Methods in Your Test Classes
By nature, static methods cannot be overridden. If your test classes contain static methods, this could potentially lead to JUnit failing to discover or execute the tests correctly.
Applying SOLID Principles in Your Tests
Adhering to SOLID principles in your tests can also help avoid common errors in JUnit. Ensure every test class has a single responsibility, they are open for extension but closed for modification, and dependencies between them can be substituted without affecting your tests.
As Martin Fowler,a British software developer, author and international public speaker on software development, specializing in object-oriented analysis and design, UML, patterns, and agile software development methodologies, including extreme programming, states “Whenever you are tempted to type something into a print statement or a debugger expression, write it as a test instead.”
This does not only arm the developer but the entire testing phase with useful clues to prevent any failures beforehand rather than trying to decipher what went wrong after the fact.
The occurrence of the Org.Junit.Platform.Commons.Junitexception isn’t a random incident but corresponds to certain specific conditions or setup issues. This exception generally occurs when JUnit fails to discover tests in an application under test configured with the engine ID ‘junit-jupiter.’
Investigations into this issue have shown that it can frequently be attributed to:
– An incompatible version of JUnit being used
– Misconfiguration of the testing suite, particularly in various IDE platforms such as Eclipse or IntelliJ.
Understanding this problem requires understanding some specific terms:
public class SomeClass
{
// Class logic here
}
“JUnit” is an open-source testing framework for Java which provides assertions to identify test method, test-cases containing fixture methods (i.e setUp() and tearDown()). It accentuates simplicity and efficiency in writing and testing code.(JUnit official Documentation)
“Junit-Jupiter” refers to the combination of the new programming and extension models provided by JUnit 5. When properly configured and used with compatible versions, it effectively aids software developers by identifying potential issues early in the development lifecycle.
Resolving the `Org.Junit.Platform.Commons.Junitexception Testengine With Id Junit-Jupiter Failed To Discover Tests` issue necessitates troubleshooting along these lines:
1. Check if JUnit is correctly set up and ensure that the correct version compatible with your Java version is appropriately referenced within your project dependencies.
2. Inspect your Project Setup – Are there any unmet or mismatched dependencies?
3. Validate the configuration of your testing suite.
By taking these steps, software developers can avoid or resolve the common Junit exception, ensuring a smooth unit testing process which is integral for high-quality software development. Veering off slightly onto another topic, Elon Musk once said – “If you get up in the morning and think the future is going to be better, it is a bright day. Otherwise, it’s not.” This inspires us to continuously strive towards resolving coding challenges and develop better software solutions each day.